一、SSH整合-实现登录以及在线列表展示
1.该项目实现用户登录在线列表展示功能。
2.用户只能单点登录
1.1 创建数据库的SQL
CREATE TABLE `admin` (
`id` int(11) DEFAULT NULL,
`account` varchar(50) DEFAULT NULL,
`pwd` varchar(255) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8
CREATE TABLE `dept` (
`id` int(11) DEFAULT NULL,
`name` varchar(255) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8
CREATE TABLE `emp` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`dept_id` int(11) DEFAULT NULL,
`name` varchar(50) DEFAULT NULL,
`age` int(11) DEFAULT NULL,
`birth` date DEFAULT NULL,
`content` text,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=23 DEFAULT CHARSET=utf8
1.2 代码
1.2.2 实体层
AdminEntity .java
public class AdminEntity {
private int id;
private String account;
private String pwd;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
}
DeptEntity .java
public class DeptEntity {
private int id;
private String name;
private Set<EmpEntity> emps;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set<EmpEntity> getEmps() {
return emps;
}
public void setEmps(Set<EmpEntity> emps) {
this.emps = emps;
}
}
EmpEntity .java
public class EmpEntity {
private Integer id;
private String name;
private Integer age ;
private Date birth;
private String content;
private DeptEntity deptEntity;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public DeptEntity getDeptEntity() {
return deptEntity;
}
public void setDeptEntity(DeptEntity deptEntity) {
this.deptEntity = deptEntity;
}
}
1.2.2 mapper层
AdminEntity.hbm.xml
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="org.jsoft.entity" >
<class name="AdminEntity" table="admin">
<!-- 主键 ,映射 -->
<id name="id" column="id" type="int" >
<generator class="native"></generator>
</id>
<!-- 非主键,映射 -->
<property name="account" column="account"></property>
<property name="pwd" column="pwd"></property>
</class>
</hibernate-mapping>
DeptEntity.hbm.xml
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="org.jsoft.entity" >
<class name="DeptEntity" table="dept">
<!-- 主键 ,映射 -->
<id name="id" column="id" type="int" >
<generator class="native"></generator>
</id>
<!-- 非主键,映射 -->
<property name="name" column="name"></property>
<set name="emps" table="emp">
<key column="id"></key>
<!-- 映射外键表。class指定外键表对应的java类型。由于package指定了包,所以可以简写类名。否则,写完整类名 -->
<one-to-many class="EmpEntity"></one-to-many>
</set>
</class>
</hibernate-mapping>
EmpEntity.hbm.xml
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="org.jsoft.entity" >
<class name="EmpEntity" table="emp">
<!-- 主键 ,映射 -->
<id name="id" column="id" type="java.lang.Integer" >
<generator class="native"></generator>
</id>
<!-- 非主键,映射 -->
<property name="name" column="name"></property>
<property name="age" column="age" type="java.lang.Integer"></property>
<property name="birth" column="birth" type="java.util.Date"></property>
<property name="content" column="content" ></property>
<many-to-one name="deptEntity" column="dept_id" class="DeptEntity" lazy="false"></many-to-one>
</class>
</hibernate-mapping>
1.2.3 dao层
BaseDao.java
public class BaseDao<T> {
private Class clazz;
public BaseDao() {
Type type = this.getClass().getGenericSuperclass();
ParameterizedType t=(ParameterizedType) type;
clazz=(Class) t.getActualTypeArguments()[0];
}
private SessionFactory sessionFactory;
public SessionFactory getSessionFactory() {
return sessionFactory;
}
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public Session getSession() {
return sessionFactory.getCurrentSession();
}
public int save(T t){
return (int) getSession().save(t);
}
public void update(T t){
getSession().update(t);
}
public int delete(Object [] ids) {
if(ids==null||ids.length==0) {
throw new RuntimeException("删除集合为空");
}
String delIds="";
for(int i=0;i<ids.length;i++) {
delIds+=ids[i].toString();
}
String hql=String.format("delete from %s where id in (%s)",clazz.getSimpleName(),delIds);
return getSession().createQuery(hql).executeUpdate();
}
public List<T> queryAll(){
return getSession().createQuery(String.format("from %s",clazz.getSimpleName())).list();
}
public T queryById(Object id) {
return (T) getSession().get(clazz, (Serializable) id);
}
public List<T> queryByParams(String hql,String ...parmas) {
Query query = getSession().createQuery(hql);
if(parmas!=null) {
for(int i=0;i<parmas.length;i++) {
query.setParameter(i, parmas[i]);
}
}
return query.list();
}
}
AdminDao .java
public class AdminDao extends BaseDao<AdminEntity> {
}
DeptDao .java
public class DeptDao extends BaseDao<DeptEntity> {
}
EmpDao .java
public class EmpDao extends BaseDao<EmpEntity> {
}
1.2.4 service层
AdminService .java
public class AdminService {
private AdminDao adminDao;
public AdminDao getAdminDao() {
return adminDao;
}
public void setAdminDao(AdminDao adminDao) {
this.adminDao = adminDao;
}
public AdminEntity queryByAccount(AdminEntity admin) {
List<AdminEntity> result = adminDao.queryByParams("from AdminEntity where account =? and pwd=?", admin.getAccount(),admin.getPwd());
if(result==null ||result.size()==0) {
return null;
}
return result.get(0);
}
}
DeptSeivice .java
public class DeptSeivice {
private DeptDao deptDao;
public DeptDao getDeptDao() {
return deptDao;
}
public void setDeptDao(DeptDao deptDao) {
this.deptDao = deptDao;
}
public List<DeptEntity> list(){
return deptDao.queryAll();
}
public DeptEntity getById(int id) {
return deptDao.queryById(id);
}
}
EmpSerivce .java
public class EmpSerivce {
private EmpDao empDao;
public EmpDao getEmpDao() {
return empDao;
}
public void setEmpDao(EmpDao empDao) {
this.empDao = empDao;
}
public int save(EmpEntity emp) {
return empDao.save(emp);
}
public void update(EmpEntity emp) {
empDao.update(emp);
}
public int delete(int id) {
return empDao.delete(new Object[] {id});
}
public List<EmpEntity> queryList(){
return empDao.queryAll();
}
public EmpEntity getById(int id) {
return empDao.queryById(id);
}
}
1.2.4 controller层
AdminController .java
public class AdminController extends ActionSupport implements ModelDriven<AdminEntity> {
private AdminService adminService;
public AdminService getAdminService() {
return adminService;
}
public void setAdminService(AdminService adminService) {
this.adminService = adminService;
}
private AdminEntity admin=new AdminEntity();
@Override
public AdminEntity getModel() {
return admin;
}
@SuppressWarnings("unchecked")
public String login(){
AdminEntity admin2 = adminService.queryByAccount(admin);
if(admin2==null) {
ServletActionContext.getRequest().setAttribute("msg", "用户名不存在或密码错误!!");
return "loginFail";
}
ActionContext.getContext().getSession().put("userInfo", admin2);
Object obj = ServletActionContext.getServletContext().getAttribute("onlineUsers");
if(obj==null) {
Map<String,HttpSession> sessionMap=new HashMap<String,HttpSession> ();
sessionMap.put(admin2.getAccount(), ServletActionContext.getRequest().getSession());
ServletActionContext.getServletContext().setAttribute("onlineUsers", sessionMap);
}else {
Map<String,HttpSession> sessionMap=(Map<String, HttpSession>) obj;
if(sessionMap.containsKey(admin2.getAccount())) {
sessionMap.get(admin2.getAccount()).invalidate();
}
sessionMap.put(admin2.getAccount(), ServletActionContext.getRequest().getSession());
}
return "loginSuccess";
}
public String loginOut(){
ActionContext.getContext().getSession().remove("userInfo");
ServletActionContext.getRequest().setAttribute("msg", "退出成功!!");
return "loginFail";
}
}
EmpController .java
public class EmpController extends ActionSupport implements ModelDriven<EmpEntity> {
private EmpSerivce empSerivce;
private DeptSeivice deptSeivice;
public EmpSerivce getEmpSerivce() {
return empSerivce;
}
public void setEmpSerivce(EmpSerivce empSerivce) {
this.empSerivce = empSerivce;
}
public DeptSeivice getDeptSeivice() {
return deptSeivice;
}
public void setDeptSeivice(DeptSeivice deptSeivice) {
this.deptSeivice = deptSeivice;
}
public String list() {
List<EmpEntity> result = empSerivce.queryList();
Hibernate.initialize(result);
HttpServletRequest req = ServletActionContext.getRequest();
req.setAttribute("result", result);
return "list";
}
public String addView() {
List<DeptEntity> result = deptSeivice.list();
HttpServletRequest request = ServletActionContext.getRequest();
request.setAttribute("depts", result);
return "addView";
}
public String updateView() {
List<DeptEntity> depts = deptSeivice.list();
ActionContext context = ActionContext.getContext();
context.put("depts", depts);
EmpEntity emp2 = empSerivce.getById(emp.getId());
ValueStack vs = ActionContext.getContext().getValueStack();
vs.push(emp2);
return "updateView";
}
private EmpEntity emp;
private Integer deptId;
@Override
public EmpEntity getModel() {
emp=new EmpEntity();
return emp;
}
public Integer getDeptId() {
return deptId;
}
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
public String add() {
DeptEntity dept = deptSeivice.getById(deptId);
emp.setDeptEntity(dept);
empSerivce.save(emp);
return "listAction";
}
public String update() {
DeptEntity dept = deptSeivice.getById(deptId);
emp.setDeptEntity(dept);
empSerivce.update(emp);
return "listAction";
}
public String delete() {
empSerivce.delete(emp.getId());
return "listAction";
}
}
1.2.6 interceptor
UserInterceptor .java
public class UserInterceptor extends AbstractInterceptor{
private static final long serialVersionUID = 1L;
@Override
public String intercept(ActionInvocation ac) throws Exception {
String method = ac.getProxy().getMethod();
if(method.equals("login")) {
return ac.invoke();
}
Object admin = ActionContext.getContext().getSession().get("userInfo");
if(admin==null) {
return "loginFail";
}
return ac.invoke();
}
}
1.2.7 struts类型转化器
DateConvert .java
public class DateConvert extends StrutsTypeConverter {
@Override
public Object convertFromString(Map map, String[] values, Class toClass) {
if(values==null||values.length==0||values[0].length()==0) {return null ;}
if(!toClass.equals(Date.class)){return null;}
System.out.println(">>>> 日期转换");
try {
SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd");
return sdf.parse(values[0]);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
@Override
public String convertToString(Map arg0, Object value) {
if(value==null) {
return null;
}
if(value instanceof Date) {
SimpleDateFormat sd=new SimpleDateFormat("yyyy-MM-dd");
return sd.format((Date)value);
}
return null;
}
}
src/xwork-conversion.properties
java.util.Date=org.jsoft.convert.DateConvert
1.2.8 配置文件
src/resource/bean-base.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/base"></property>
<property name="driverClass" value="com.mysql.jdbc.Driver"></property>
<property name="user" value="root"></property>
<property name="password" value="root"></property>
<property name="initialPoolSize" value="3"></property>
<property name="maxPoolSize" value="6"></property>
<property name="maxIdleTime" value="1000"></property>
</bean>
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource"></property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialect</prop>
<prop key="hibernate.show_sql">true</prop>
<prop key="hibernate.hbm2ddl.auto">update</prop>
</props>
</property>
<property name="mappingDirectoryLocations">
<list>
<value>classpath:org/jsoft/entity/mapper</value>
</list>
</property>
</bean>
<bean id="txManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory"></property>
</bean>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="get*" read-only="true"/>
<tx:method name="find*" read-only="true"/>
<tx:method name="*" read-only="false"/>
</tx:attributes>
</tx:advice>
<aop:config proxy-target-class="true">
<aop:pointcut expression="execution(* org.jsoft.service.*.*(..))" id="pt"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="pt"/>
</aop:config>
</beans>
src/resource/bean-controller.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="empAction" class="org.jsoft.controller.EmpController">
<property name="empSerivce" ref="empSerivce"></property>
<property name="deptSeivice" ref="deptSeivice"></property>
</bean>
<bean id="adminAction" class="org.jsoft.controller.AdminController">
<property name="adminService" ref="adminService"></property>
</bean>
</beans>
src/resource/bean-service.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="deptSeivice" class="org.jsoft.service.DeptSeivice">
<property name="deptDao" ref="deptDao"></property>
</bean>
<bean id="empSerivce" class="org.jsoft.service.EmpSerivce">
<property name="empDao" ref="empDao"></property>
</bean>
<bean id="adminService" class="org.jsoft.service.AdminService">
<property name="adminDao" ref="adminDao"></property>
</bean>
</beans>
src/resource/bean-dao.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="empDao" class="org.jsoft.dao.EmpDao">
<property name="sessionFactory" ref="sessionFactory"></property>
</bean>
<bean id="deptDao" class="org.jsoft.dao.DeptDao">
<property name="sessionFactory" ref="sessionFactory"></property>
</bean>
<bean id="adminDao" class="org.jsoft.dao.AdminDao">
<property name="sessionFactory" ref="sessionFactory"></property>
</bean>
</beans>
src/struts.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name="struts.i18n.encoding" value="UTF-8"></constant>
<constant name="struts.multipart.maxSize" value="1000000"></constant>
<package name="xxxx" extends="struts-default">
<interceptors>
<interceptor name="userInterceptor" class="org.jsoft.interceptor.UserInterceptor"></interceptor>
<interceptor-stack name="my">
<interceptor-ref name="defaultStack"></interceptor-ref>
<interceptor-ref name="userInterceptor"></interceptor-ref>
</interceptor-stack>
</interceptors>
<default-interceptor-ref name="my"></default-interceptor-ref>
<global-results>
<result name="success">/index.jsp</result>
<result name="err1">/index.jsp</result>
<result name="null">/index.jsp</result>
<result name="loginFail">/login.jsp</result>
</global-results>
<global-exception-mappings>
<exception-mapping exception="java.lang.NullPointerException" result="null" ></exception-mapping>
<exception-mapping exception="java.lang.Exception" result="err1" ></exception-mapping>
<exception-mapping exception="java.lang.NoSuchMethodException" result="err1"></exception-mapping>
</global-exception-mappings>
<action name="empAction_*" class="empAction" method="{1}">
<result name="list">/WEB-INF/view/list.jsp</result>
<result name="addView" >/WEB-INF/view/add.jsp</result>
<result name="updateView">/WEB-INF/view/update.jsp</result>
<result name="listAction" type="redirectAction">empAction_list</result>
</action>
<action name="adminAction_*" class="adminAction" method="{1}">
<result name="loginFail">/login.jsp</result>
<result name="loginSuccess" type="redirectAction">empAction_list</result>
</action>
</package>
</struts>
src/WEB-INF/web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<!-- 打开spring的SessionInView模式,作用是JSP页面访问懒加载数据 -->
<!-- 该配置一定放在struts前面 -->
<filter>
<filter-name>OpenSessionInView</filter-name>
<filter-class>org.springframework.orm.hibernate3.support.OpenSessionInViewFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>OpenSessionInView</filter-name>
<url-pattern>*.action</url-pattern>
</filter-mapping>
<!-- 1.struts配置 :引入struts核心过滤器 -->
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>
1.2.9 前端
index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>员工列表</title>
</head>
<body>
<c:redirect url="./empAction_list"></c:redirect>
</body>
</html>
login.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录</title>
</head>
<body>
<form action="./adminAction_login" method="post">
<table>
<tr>
<td>用户名</td>
<td><input type="text" name="account"></td>
</tr>
<tr>
<td>密码</td>
<td><input type="text" name="pwd"></td>
</tr>
<tr>
<td colspan="2" ><input type="submit" value="提交"> ${msg}</td>
</tr>
</table>
</form>
</body>
</html>
/WEB-INF/view/list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>员工列表</title>
</head>
<body>
<div>
<jsp:include page="./head.jsp"></jsp:include>
<table border="1" cellpading="0" cellspacing="0" style="margin:20px auto;width:500px;+">
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>备注</th>
<th>出生日期</th>
<th>部门</th>
<th>操作</th>
</tr>
<c:forEach items="${result}" var="emp" >
<tr>
<td>${emp.id}</td>
<td>${emp.name}</td>
<td>${emp.age}</td>
<td>${emp.content}</td>
<td>${emp.birth}</td>
<td>${emp.deptEntity.name}</td>
<td>
<a href="./empAction_updateView?id=${emp.id}">修改</a>
<a href="./empAction_delete?id=${emp.id}">删除</a>
</td>
</tr>
</c:forEach>
</table>
</div>
</body>
</html>
/WEB-INF/view/add.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>新增员工</title>
</head>
<style type="text/css">
td{
padding:2px;
text-align:center;
}
</style>
<body>
<form action="./empAction_add" method="post">
<table border="1" cellspacing="0" style="margin:20px auto;">
<caption>新增员工</caption>
<tr>
<td>姓名</td>
<td><input type="text" name="name" /></td>
</tr>
<tr>
<td>年龄</td>
<td><input type="text" name="age" /></td>
</tr>
<tr>
<td>备注</td>
<td><input type="text" name="content" /></td>
</tr>
<tr>
<td>出生日期</td>
<td><input type="date" name="birth" /></td>
</tr>
<tr>
<td>部门</td>
<td>
<select style="width:90%;" name="deptId" >
<option value="-1">请选择部门</option>
<c:forEach items="${depts}" var="item">
<option value="${item.id}"> ${item.name}</option>
</c:forEach>
</select>
</td>
</tr>
<tr>
<td colspan="2"> <input type="submit" value="提交" /> <input type="reset" value="重置" /></td>
</tr>
</table>
</form>
</body>
</html>
/WEB-INF/view/update.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@taglib uri="/struts-tags" prefix="s"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>修改员工</title>
</head>
<body>
<s:form action="./empAction_update" theme="simple">
<table border="1" cellspacing="0" cellpadding="0" style="margin:20px auto;">
<s:hidden name="id" value="%{id}"></s:hidden>
<tr>
<td>姓名</td>
<td><s:textfield name="name" value="%{name}" ></s:textfield></td>
</tr>
<tr>
<td>年龄</td>
<td><s:textfield name="age" value="%{age}" ></s:textfield></td>
</tr>
<tr>
<td>备注</td>
<td><s:textarea name="content" value="%{content}" ></s:textarea></td>
</tr>
<tr>
<td>出生日期</td>
<td><s:textfield name="birth" value="%{birth}" ></s:textfield></td>
</tr>
<tr>
<td>部门</td>
<td>
<s:select
name="deptId"
list="%{#depts}"
headerKey="-1"
headerValue="请选择"
listKey="id"
listValue="name"
value="%{deptEntity.id}"
></s:select>
</td>
</tr>
<tr>
<td><s:submit>修改员工</s:submit></td>
<td><s:reset >重置</s:reset></td>
</tr>
</table>
</s:form>
</body>
</html>
/WEB-INF/view/head.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="/struts-tags" prefix="s"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
<!-- 如果用户登录,显示 欢迎你 -->
<!-- 如果用户没有登录,显示 没有登录 -->
<s:if test="#session.userInfo != null">
<div style="display:inline-block;margin:0px auto;">
欢迎你,${userInfo.account}
<a href="./adminAction_loginOut">退出</a>
<a href="./empAction_addView">新增</a>
</div>
</s:if>
<s:else>
<div style="display:inline-block;margin:0px auto;">
没有登录!!!
<s:a href="./login.jsp">登录</s:a>
<s:a href="./register">注册</s:a>
</div>
</s:else>
</body>
</html>
1.3 实现效果
登录界面
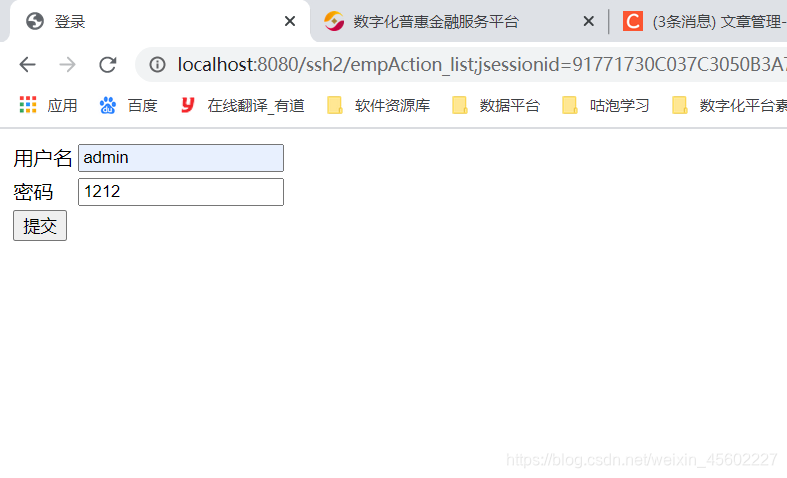
在线列表展示界面
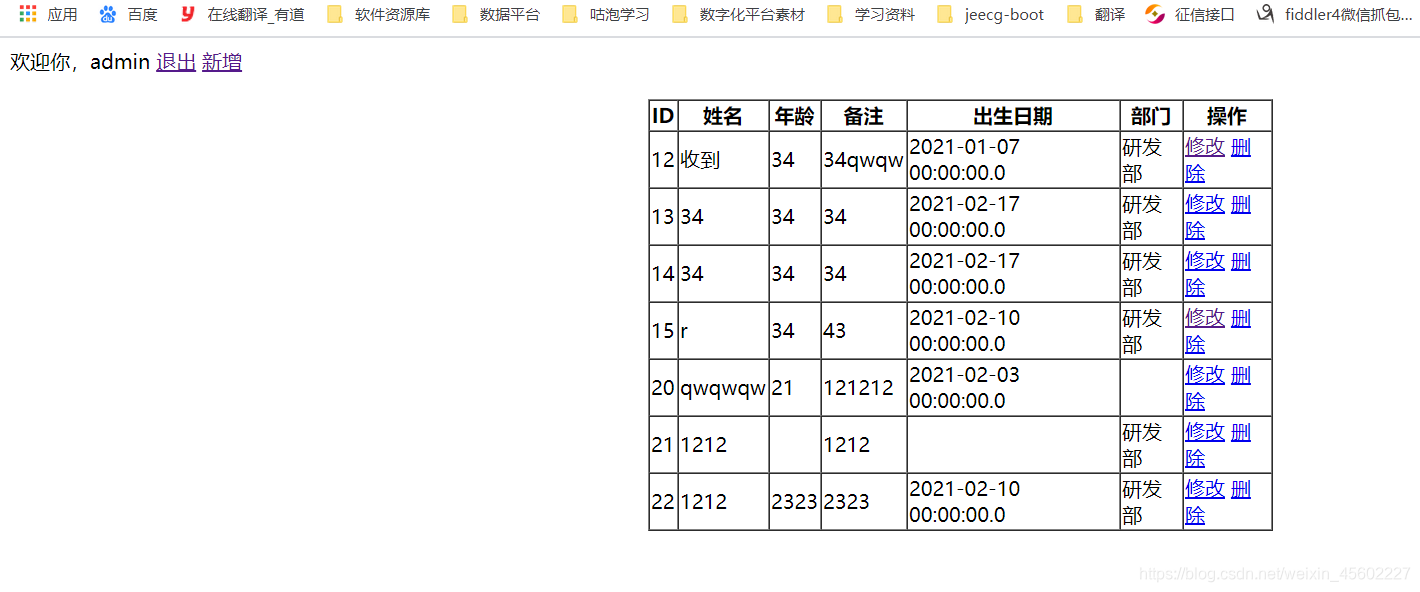
新增界面
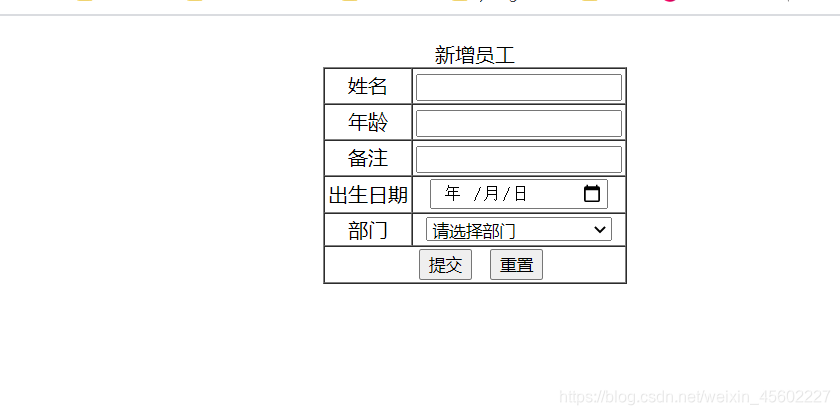
修改界面
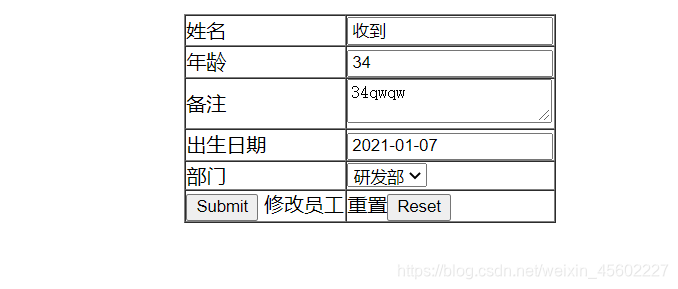