C# winform 移动绘制圆形
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace DrawImage
{
public partial class Form1 : Form
{
private float c_cx;
private float c_cy;
private List<OffsetPoint> pointLst;
private int indexSelect = 0;
public Form1()
{
SetStyle(ControlStyles.UserPaint, true);
SetStyle(ControlStyles.AllPaintingInWmPaint, true);
SetStyle(ControlStyles.DoubleBuffer, true);
InitializeComponent();
this.c_cx = this.Width / 2;
this.c_cy = this.Height / 2;
pointLst = new List<OffsetPoint>();
pointLst.Add(new OffsetPoint(100, -100));
pointLst.Add(new OffsetPoint(0, 0));
pointLst.Add(new OffsetPoint(0, 50));
pointLst.Add(new OffsetPoint(0, -50));
pointLst.Add(new OffsetPoint(-100, 100));
pointLst.Add(new OffsetPoint(-100, -100));
pointLst.Add(new OffsetPoint(100, 100));
}
private void button1_Click(object sender, EventArgs e)
{
if (this.indexSelect % 7 == 0)
this.indexSelect = 0;
OffsetPoint curPoint = this.pointLst[indexSelect];
int nTgCx= this.Width / 2 + curPoint.o_x;
int nTgCy = this.Height / 2 + curPoint.o_y;
System.Diagnostics.Debug.WriteLine(nTgCx.ToString(), nTgCy.ToString() );
ThreadPool.QueueUserWorkItem(state => movePoint(nTgCx, nTgCy));
this.indexSelect += 1;
}
private void movePoint(int nTgCx, int nTgCy)
{
float all_diff_x = System.Math.Abs(this.c_cx - nTgCx) * 0.01f;
float all_diff_y = System.Math.Abs(this.c_cy - nTgCy) * 0.01f;
while (true)
{
float cx_diff = System.Math.Abs(this.c_cx - nTgCx);
float cy_diff = System.Math.Abs(this.c_cy - nTgCy);
if (cx_diff <= 0.001 && cy_diff <= 0.001) break;
Thread.Sleep(5);
this.c_cx = this.c_cx > nTgCx ? this.c_cx - all_diff_x : this.c_cx + all_diff_x;
this.c_cy = this.c_cy > nTgCy ? this.c_cy - all_diff_y : this.c_cy + all_diff_y;
this.Invalidate();
}
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
e.Graphics.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.AntiAlias;
e.Graphics.SmoothingMode = SmoothingMode.HighQuality;
e.Graphics.InterpolationMode = InterpolationMode.HighQualityBicubic;
e.Graphics.CompositingQuality = CompositingQuality.HighQuality;
Color drawColor = Color.FromArgb(0, 0, 255);
int cirR = 10;
int cx = Convert.ToInt32(System.Math.Round(this.c_cx));
int cy = Convert.ToInt32(System.Math.Round(this.c_cy));
e.Graphics.DrawEllipse(new Pen(drawColor, 10), cx - cirR, cy - cirR, cirR * 2, cirR * 2);
}
}
public class OffsetPoint
{
public int o_x;
public int o_y;
public OffsetPoint(int o_x, int o_y)
{
this.o_x = o_x;
this.o_y = o_y;
}
}
}
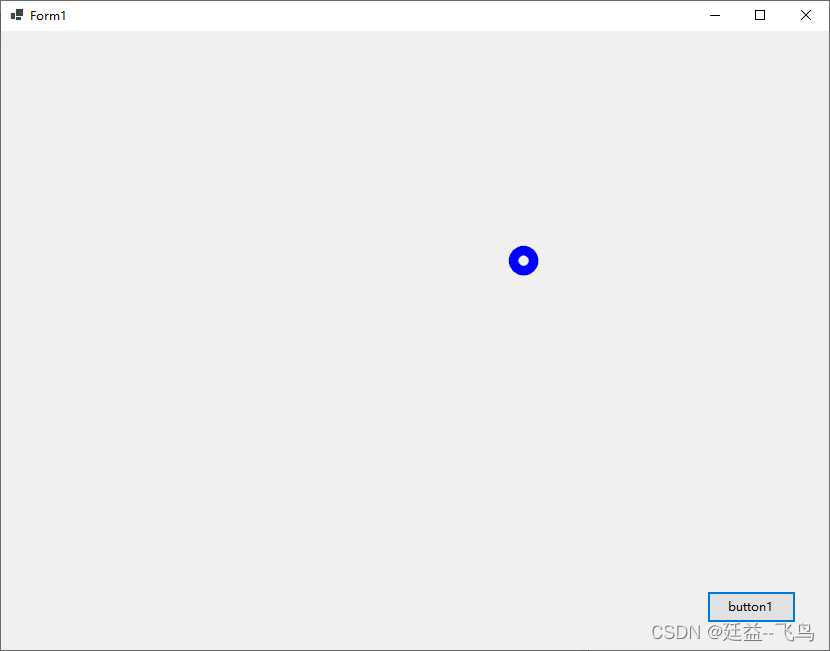