目录
1 . 顺序栈
#include <iostream>
using namespace std;
#define MaxSize 50
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//最大容量
int top;//栈顶指针
} SqStack;
//初始化栈
void InitStack(SqStack &S) {
S.top = -1;//初始化栈顶指针
}
//判断栈空
bool StackEmpty(SqStack S) {
if(S.top == -1) {
return true;
} else {
return false;
}
}
//新元素入栈
bool Push(SqStack &S, ElemType x) {
if(S.top == MaxSize - 1) { //栈满 报错
return false;
}
S.top += 1;
S.data[S.top] = x;
// S.data[++S.top] = x;
return true;
}
//元素出栈
bool Pop(SqStack &S, ElemType &x) {
if(S.top == -1) { //栈空
return false;
}
x = S.data[S.top];
S.top -= 1;
// x = S.data[S.top--];
return true;
}
//读取栈顶元素
bool GetPop(SqStack &S, ElemType &x) {
if(S.top == -1) { //栈空
return false;
}
x = S.data[S.top];
return true;
}
void test() {
SqStack S;
InitStack(S);
for (int i = 0; i < 10; i++) {
Push(S, i);
}
if(!StackEmpty(S)) {
cout << "Not Empty!" << endl;
} else {
cout << "Is Empty!" << endl;
}
ElemType k;
while(!StackEmpty(S)) {
Pop(S, k);
cout << k << " ";
}
cout << endl;
}
int main() {
test();
return 0;
}
代码运行结果: 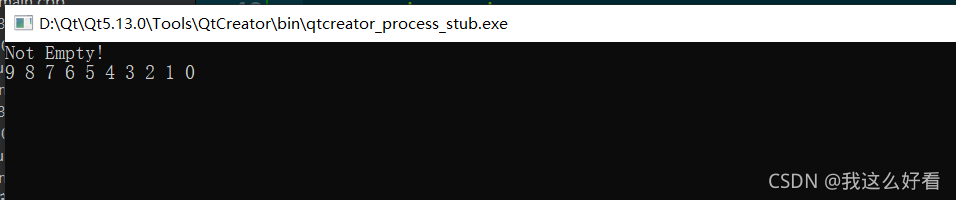
2 . 共享栈
#include <iostream>
using namespace std;
#define MaxSize 50
typedef int ElemType;
typedef struct {
ElemType data[MaxSize];//最大容量
//栈顶指针
int top0;
int top1;
} ShStack;
//初始化栈
void InitStack(ShStack &S) {
//初始化栈顶指针
S.top0 = -1;
S.top1 = MaxSize;
}
//判断栈空
bool StackEmpty(ShStack S) {
if(S.top0 == -1 && S.top1 == MaxSize) {
return true;
} else {
return false;
}
}
//判断栈满
bool StackFull(ShStack S) {
if(S.top0 + 1 == S.top1) {
return true;
} else {
return false;
}
}
//新元素入栈
bool Push0(ShStack &S, ElemType x) {
if(StackFull(S)) { //栈满 报错
return false;
}
S.data[++S.top0] = x;
return true;
}
bool Push1(ShStack &S, ElemType x) {
if(StackFull(S)) { //栈满 报错
return false;
}
S.data[--S.top1] = x;
return true;
}
//元素出栈
bool Pop0(ShStack &S, ElemType &x) {
if(S.top0 == -1) { //栈空
return false;
}
x = S.data[S.top0--];
return true;
}
bool Pop1(ShStack &S, ElemType &x) {
if(S.top1 == MaxSize) { //栈空
return false;
}
x = S.data[S.top1++];
return true;
}
//读取栈顶元素
bool GetPop0(ShStack &S, ElemType &x) {
if(S.top0 == -1) { //栈空
return false;
}
x = S.data[S.top0];
return true;
}
bool GetPop1(ShStack &S, ElemType &x) {
if(S.top1 == MaxSize) { //栈空
return false;
}
x = S.data[S.top1];
return true;
}
void test() {
ShStack S;
InitStack(S);
for (int i = 0; i < 10; i++) {
Push0(S, i);
}
for (int i = 0; i < 10; i++) {
Push1(S, i);
}
ElemType x;
if(StackEmpty(S)) {
cout << "Is Empty!" << endl;
} else {
cout << "Not Empty!" << endl;
}
while(GetPop0(S, x)) {
Pop0(S, x);
cout << x << " ";
}
cout << endl;
GetPop1(S, x);
if(StackEmpty(S)) {
cout << "Is Empty!" << endl;
} else {
cout << "Not Empty!" << endl;
}
while(GetPop1(S, x)) {
Pop1(S, x);
cout << x << " ";
}
cout << endl;
if(StackEmpty(S)) {
cout << "Is Empty!" << endl;
} else {
cout << "Not Empty!" << endl;
}
}
int main() {
test();
return 0;
}
代码运行结果: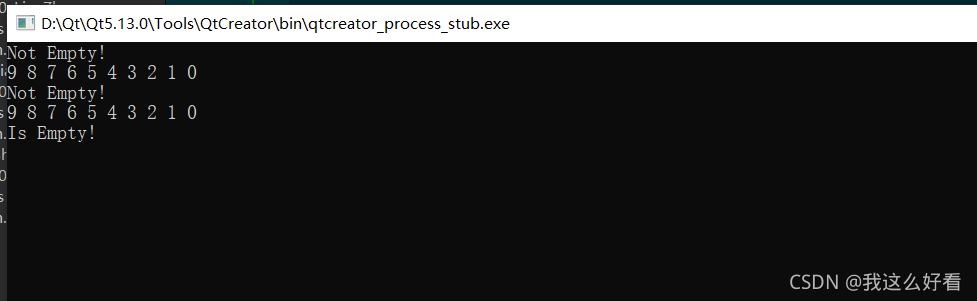
3 . 链栈(不带头结点)
#include <iostream>
using namespace std;
//不带头结点(推荐)
typedef int ElemType;
typedef struct LinkNode {
ElemType data;//数据域
struct LinkNode *next;//指针域
} *LiStack, LinkNode; //栈类型定义
//初始化链栈(不带头结点)
bool InitStack(LiStack &S) {
S = NULL;
return true;
}
//判断链栈是否为空
bool StackEmpty(LiStack S) {
if(S == NULL) {
return true;
}
return false;
}
//入栈操作
bool Push(LiStack &S, ElemType x) {
LinkNode *p = (LinkNode *)malloc(sizeof(LinkNode));
if(!p) { //申请失败
return false;
}
p->data = x;
p->next = S;
S = p;
return true;
}
//弹栈操作
bool Pop(LiStack &S, ElemType &x) {
if(StackEmpty(S)) { //空栈
return false;
}
LinkNode *p = S;
S = S->next;
x = p->data;
free(p);
return true;
}
//读取栈顶元素
bool GetTop(LiStack S, ElemType &x) {
if(StackEmpty(S)) { //空栈
return false;
}
x = S->data;
return true;
}
//销毁链栈
bool DestoryStack(LiStack &S) {
while(S != NULL) {
LinkNode *p = S;
S = S->next;
free(p);
}
free(S);
S = NULL;
return true;
}
void test() {
LiStack S;
InitStack(S);
// 入栈
for (int i = 0; i < 10; i++) {
Push(S, i);
}
// 栈顶元素
ElemType x;
if(GetTop(S, x)) {
cout << "GetTop:" << x << endl;
}
while(!StackEmpty(S)) {
Pop(S, x);
cout << x << " ";
}
cout << endl;
// 判空
if(StackEmpty(S)) {
cout << "Is Empty!" << endl;
} else {
cout << "Not Empty!" << endl;
}
// 销毁
DestoryStack(S);
}
int main() {
test();
return 0;
}
代码运行结果: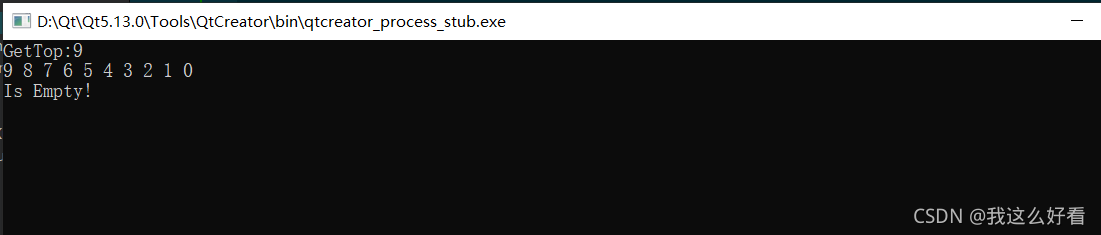
4 . 链栈(带头结点)
#include <iostream>
using namespace std;
//带头结点
typedef int ElemType;
typedef struct LinkNode {
ElemType data;//数据域
struct LinkNode *next;//指针域
} *LiStack, LinkNode; //栈类型定义
//初始化链栈(带头结点)
bool InitStack(LiStack &S) {
S = (LinkNode *)malloc(sizeof(LinkNode));
if(!S) { //申请失败
return false;
}
S->next = NULL;
return true;
}
//判断链栈是否为空
bool StackEmpty(LiStack S) {
if(S->next == NULL) {
return true;
}
return false;
}
//入栈操作
bool Push(LiStack &S, ElemType x) {
LinkNode *p = (LinkNode *)malloc(sizeof(LinkNode));
if(!p) { //申请失败
return false;
}
p->data = x;
p->next = S->next;
S->next = p;
return true;
}
//弹栈操作
bool Pop(LiStack &S, ElemType &x) {
if(StackEmpty(S)) { //空栈
return false;
}
LinkNode *p = S->next;
x = p->data;
S->next = p->next;
free(p);
return true;
}
//读取栈顶元素
bool GetTop(LiStack S, ElemType &x) {
if(StackEmpty(S)) { //空栈
return false;
}
x = S->next->data;
return true;
}
//销毁链栈
bool DestoryStack(LiStack &S) {
while(S->next != NULL) {
LinkNode *p = S->next;
S->next = p->next;
free(p);
}
free(S);
return true;
}
void test() {
LiStack S;
InitStack(S);
// 入栈
for (int i = 0; i < 10; i++) {
Push(S, i);
}
// 栈顶元素
ElemType x;
if(GetTop(S, x)) {
cout << "GetTop:" << x << endl;
}
while(!StackEmpty(S)) {
Pop(S, x);
cout << x << " ";
}
cout << endl;
// 判空
if(StackEmpty(S)) {
cout << "Is Empty!" << endl;
} else {
cout << "Not Empty!" << endl;
}
// 销毁
DestoryStack(S);
}
int main() {
test();
return 0;
}