Python代码
import os
import csv
def convert_csv_to_yolo(csv_file, output_dir):
with open(csv_file, 'r') as file:
reader = csv.DictReader(file)
for row in reader:
filename = row['filename']
width = int(row['width'])
height = int(row['height'])
class_label = row['class']
xmin = int(row['xmin'])
ymin = int(row['ymin'])
xmax = int(row['xmax'])
ymax = int(row['ymax'])
x_center = (xmin + xmax) / (2 * width)
y_center = (ymin + ymax) / (2 * height)
bbox_width = (xmax - xmin) / width
bbox_height = (ymax - ymin) / height
yolo_line = f"{class_label} {x_center} {y_center} {bbox_width} {bbox_height}\n"
output_file = os.path.join(output_dir, filename + '.txt')
with open(output_file, 'a') as output:
output.write(yolo_line)
def batch_convert_csv_to_yolo(csv_folder, output_dir):
csv_files = [f for f in os.listdir(csv_folder) if f.endswith('.csv')]
for csv_file in csv_files:
csv_file_path = os.path.join(csv_folder, csv_file)
print(f"Converting {csv_file} to YOLO format:")
convert_csv_to_yolo(csv_file_path, output_dir)
print()
csv_folder_path = ''
output_folder_path = ''
batch_convert_csv_to_yolo(csv_folder_path, output_folder_path)
示例图
转换前
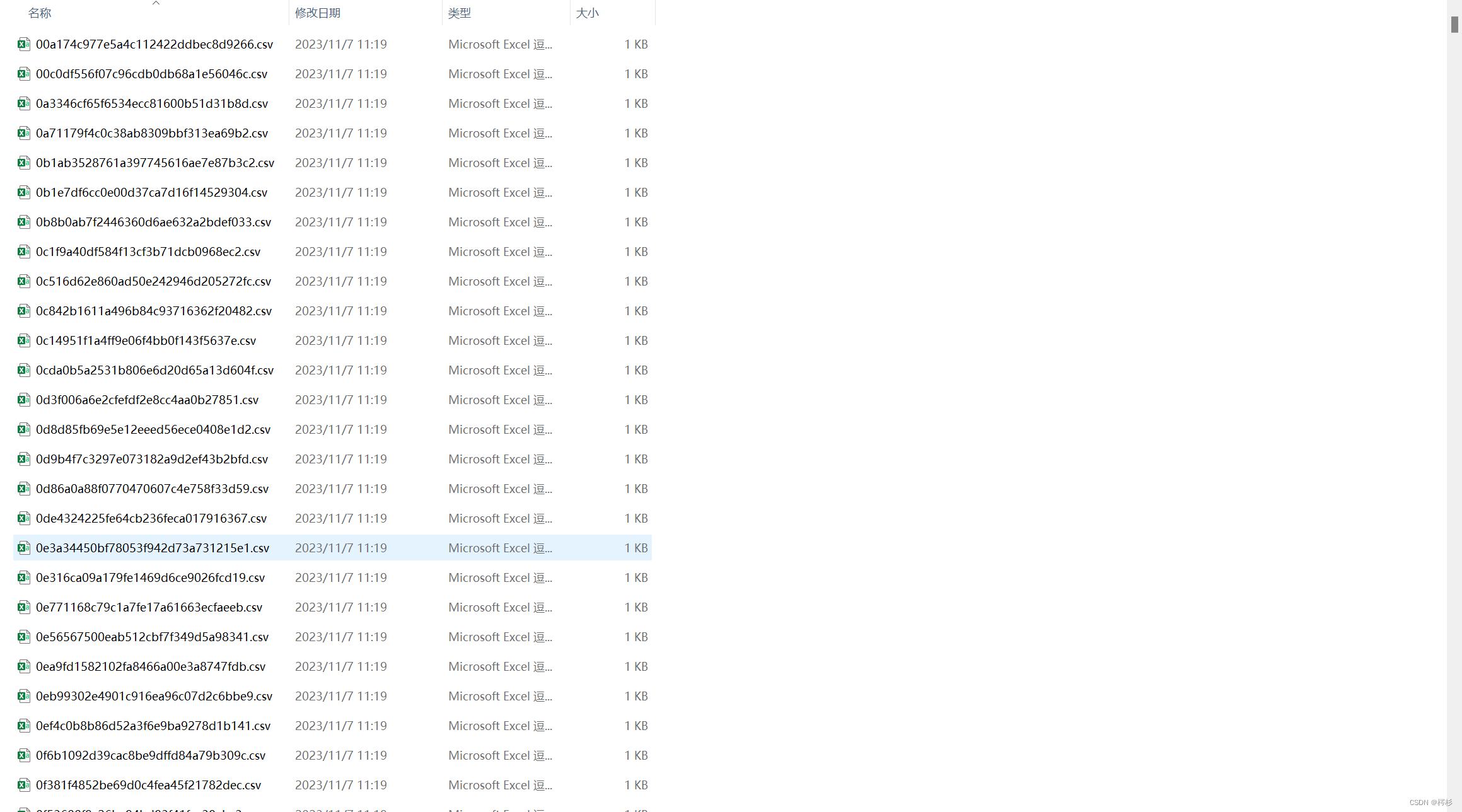
转换后
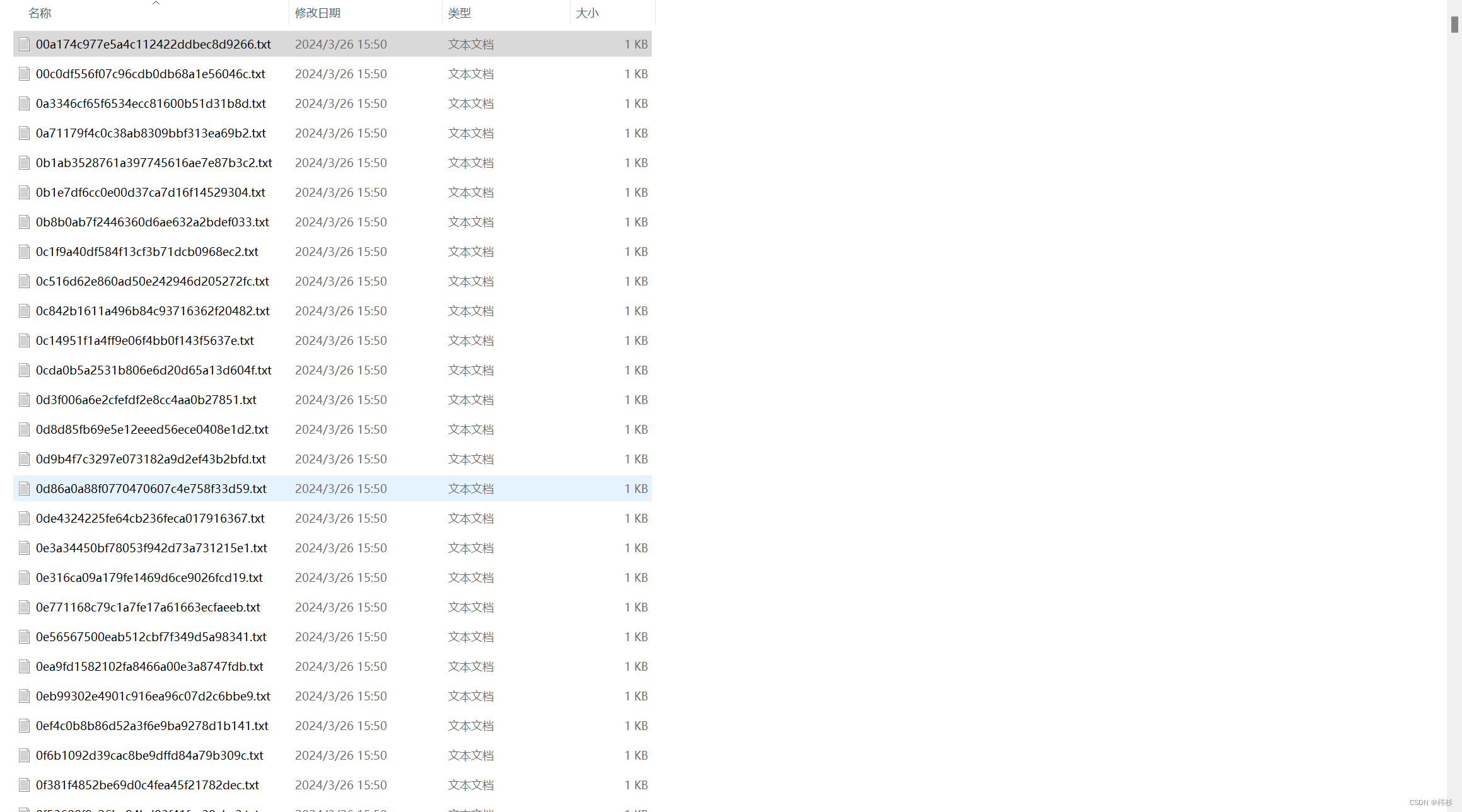