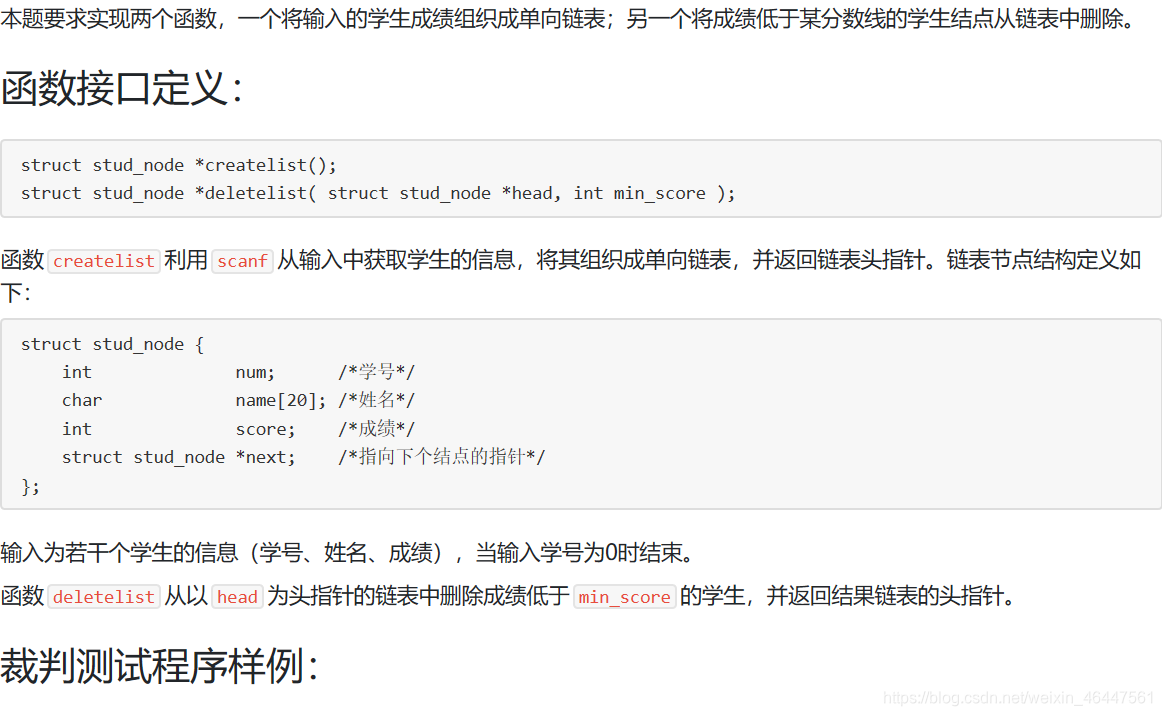
#include <stdio.h>
#include <stdlib.h>
struct stud_node {
int num;
char name[20];
int score;
struct stud_node *next;
};
struct stud_node *createlist();
struct stud_node *deletelist( struct stud_node *head, int min_score );
int main()
{
int min_score;
struct stud_node *p, *head = NULL;
head = createlist();
scanf("%d", &min_score);
head = deletelist(head, min_score);
for ( p = head; p != NULL; p = p->next )
printf("%d %s %d\n", p->num, p->name, p->score);
return 0;
}
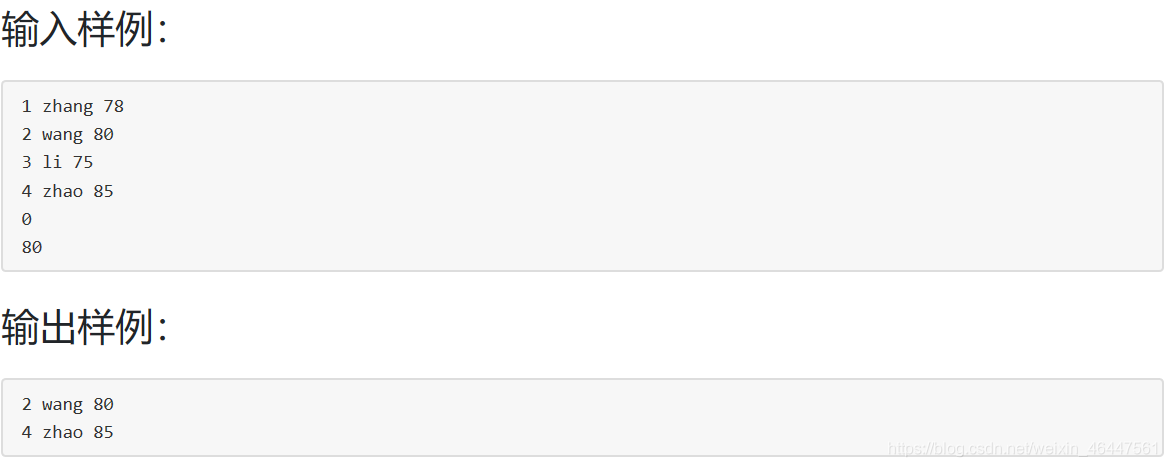
struct stud_node *createlist()
{
struct stud_node *p, *head = NULL,*tail=NULL;
int num;
while(scanf("%d",&num) && num){
p=(struct stud_node *)malloc(sizeof(struct stud_node));
p->num=num;
scanf("%s%d",p->name,&p->score);
p->next=NULL;
if(head==NULL && tail==NULL){
head=tail=p;
}
else{
tail->next=p;
tail=p;
}
}
return head;
}
struct stud_node *deletelist( struct stud_node *head, int min_score )
{
struct stud_node *p,*q;
while(head!=NULL && head->score<min_score){
q=head;
head=head->next;
free(q);
}
if(head==NULL){
return NULL;
}
p=head;
q=head->next;
while(q){
if(q->score<min_score){
p->next=q->next;
free(q);
}
else{
p=q;
}
q=p->next;
}
return head;
}