目录
下一篇(监听器)的链接https://blog.csdn.net/weixin_46919419/article/details/112390091
GUI编程
一些大概的组件:
1、窗口
2、弹窗
3、面板
4、文本框
5、列表框
6、图片
7、监听事件
8、鼠标事件
9、键盘事件
GUI核心技术Swing AWT
AWT介绍
AWT介绍:AWT是Swing的前身
是一个窗口工具
包含了很多类和接口
元素:窗口、按钮、文本框
一些类的结构
Frame窗口
public static void main(String[] args) {
//Frame对象
Frame frame = new Frame("我的第一个java图形界面窗口");
//需要设置可见性
frame.setVisible(true);//可见
//设置窗口大小
frame.setSize(400,400);
//设置背景颜色
Color color = new Color(160,160,106);
frame.setBackground(color);
//弹出的初始位置,注意0,0点在屏幕左上角往下也是正数
frame.setLocation(200,200);
//设置大小固定
frame.setResizable(false);//大小不可变
}
效果:
出现的问题:窗口关闭不了
一次弹出多个窗口的练习
public class TestFrame2 {
public static void main(String[] args) {
//展示多个窗口
MyFrame myFrame1 = new MyFrame(100, 100, 200, 200, Color.blue);
MyFrame myFrame2 = new MyFrame(300, 100, 200, 200, Color.yellow);
MyFrame myFrame3 = new MyFrame(100, 300, 200, 200, Color.red);
MyFrame myFrame4 = new MyFrame(300, 300, 200, 200, Color.MAGENTA);
}
}
class MyFrame extends Frame{
static int id=0;//可能存在多个窗口,需要一个计数器
public MyFrame(int x,int y,int w,int h,Color color){
super("MyFrame"+(++id));
setBackground(color);
setBounds(x,y,w,h);
setVisible(true);
}
}
面板Panel
//panel,可以看做是一个空间,但是单独不能单独存在
public class TestPanel {
public static void main(String[] args) {
Frame frame=new Frame();
//布局的概念
Panel panel = new Panel();
//设置布局,先暂时设置为null
frame.setLayout(null);
//坐标
frame.setBounds(300,300,500,500);
frame.setBackground(new Color(40,161,35));
//panel设置,相对于frame
panel.setBounds(50,50,400,400);
panel.setBackground(new Color(193, 40, 10));
frame.add(panel);
frame.setVisible(true);
//监听事件,监听窗口关闭事件System.exit(0);
/*设计模式之:适配器模式,先使用一个实现类例如WindowAdapter实现WindowListener,
再调用实现好了的方法,避免了每次都要实现那么多接口中的函数*/
frame.addWindowListener(new WindowAdapter() {
//窗口点击关闭的时候需要做的事情
@Override
public void windowClosing(WindowEvent e) {
//结束程序
System.exit(0);
}
});
}
}
这次可以关闭的
布局管理器
流式布局
public static void main(String[] args) {
Frame frame = new Frame();
//组件-按钮
Button button1 = new Button("button1");
Button button2 = new Button("button2");
Button button3 = new Button("button3");
//设置为流式布局
//默认,居中
// frame.setLayout(new FlowLayout());
//设置靠左
// frame.setLayout(new FlowLayout(FlowLayout.LEFT));
//设置靠右
frame.setLayout(new FlowLayout(FlowLayout.RIGHT));
frame.setSize(200,200);
//把按钮添加上去
frame.add(button1);
frame.add(button2);
frame.add(button3);
frame.setVisible(true);
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
东南南北中
public static void main(String[] args) {
Frame frame = new Frame("TestBorderLayout");
Button east = new Button("east");
Button west = new Button("west");
Button south = new Button("south");
Button north = new Button("north");
Button center = new Button("center");
frame.add(east,BorderLayout.EAST);
frame.add(west,BorderLayout.WEST);
frame.add(south,BorderLayout.SOUTH);
frame.add(north,BorderLayout.NORTH);
frame.add(center,BorderLayout.CENTER);
frame.setSize(200,200);
frame.setVisible(true);
}
表格布局Grid
public static void main(String[] args) {
Frame frame = new Frame("TestBorderLayout");
Button btn1 = new Button("btn1");
Button btn2 = new Button("btn2");
Button btn3 = new Button("btn3");
Button btn4 = new Button("btn4");
Button btn5 = new Button("btn5");
Button btn6 = new Button("btn6");
frame.setLayout(new GridLayout(3,2));
frame.add(btn1);
frame.add(btn2);
frame.add(btn3);
frame.add(btn4);
frame.add(btn5);
frame.add(btn6);
frame.pack();//java函数,相当于自动布局
frame.setVisible(true);
frame.setSize(200,200);
}
布局练习
public static void main(String[] args) {
Frame frame = new Frame();
frame.setLayout(new GridLayout(2,1));
//4个面板
Panel p1 = new Panel(new BorderLayout());
Panel p2 = new Panel(new GridLayout(2,1));
Panel p3 = new Panel(new BorderLayout());
Panel p4 = new Panel(new GridLayout(2,2));
frame.setSize(400,300);
frame.setLocation(300,400);
frame.setBackground(Color.black);
frame.setVisible(true);
//上面4个
p1.add(new Button("East-1"),BorderLayout.EAST);
p1.add(new Button("West-1"),BorderLayout.WEST);
p2.add(new Button("p2-btn-1"));
p2.add(new Button("p2-btn-2"));
p1.add(p2,BorderLayout.CENTER);
//下面
p3.add(new Button("East-2"),BorderLayout.EAST);
p3.add(new Button("West-2"),BorderLayout.WEST);
//中间4个
for (int i = 0; i < 4; i++) {
p4.add(new Button("for-"+i));
}
p3.add(p4);
frame.add(p1);
frame.add(p3);
}
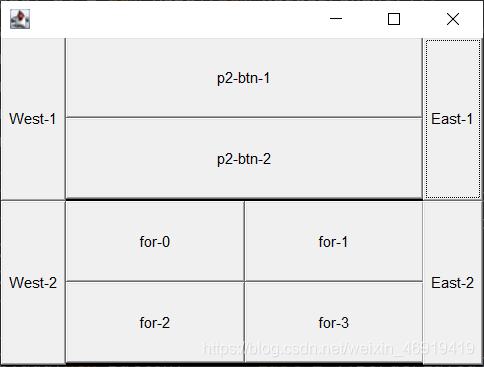
小结
1、Frame是一个顶级窗口
2、Panel无法单独显示,必须添加到某个容器中
3、布局管理器
1、流式
2、东西南北中
3、表格
4、大小,定位,背景颜色,可见性,监听