会话
:用户打开一个浏览器,点击了很多超链接,访问多个
web
资源,关闭浏览器,这个过程可以称之为会话;
一个网站,怎么证明你来过?
客户端
服务端
1.
服务端给客户端一个 信件,客户端下次访问服务端带上信件就可以了;
cookie
2.
服务器登记你来过了,下次你来的时候我来匹配你;
seesion
保存会话的两种技术
cookie
客户端技术
(响应,请求)
session
服务器技术,利用这个技术,可以保存用户的会话信息? 我们可以把信息或者数据放在
Session
中!
Cookie
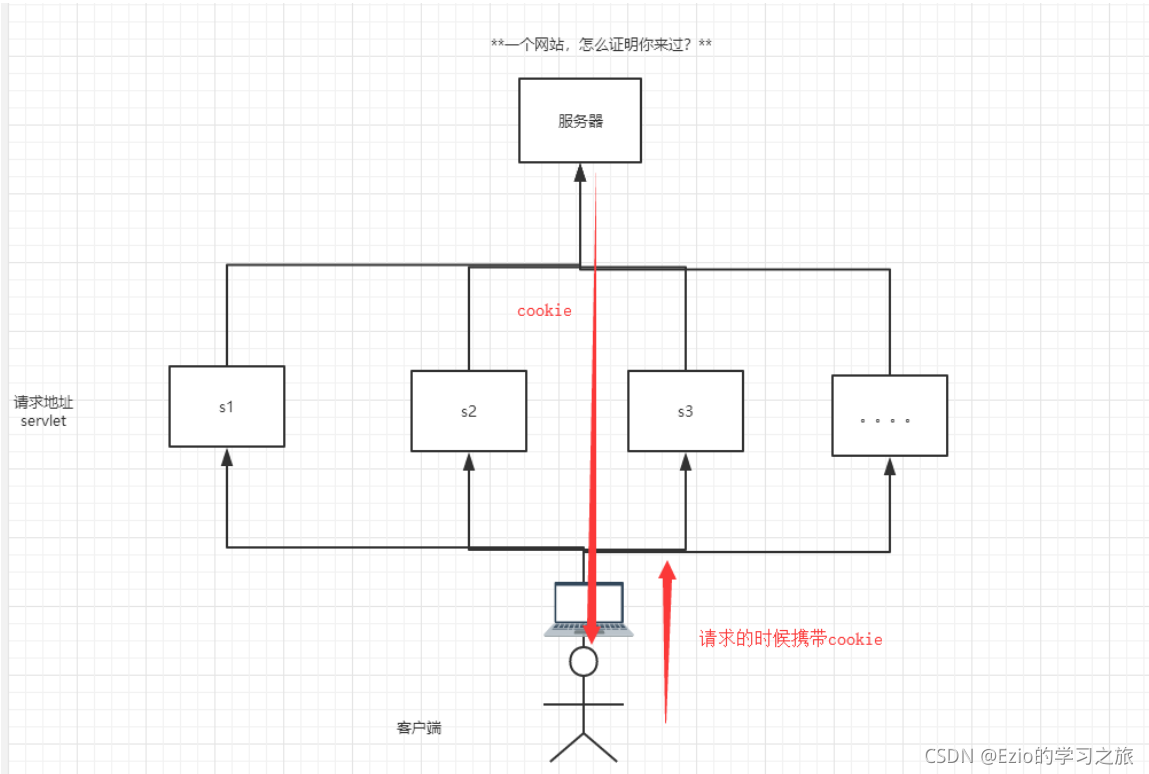
1.
从请求中拿到
cookie
信息
2.
服务器响应给客户端
cookie
Cookie[] cookies = req.getCookies(); //获得Cookie
cookie.getName(); //获得cookie中的key
cookie.getValue(); //获得cookie中的vlaue
new Cookie("lastLoginTime", System.currentTimeMillis()+""); //新建一个cookie
cookie.setMaxAge(24*60*60); //设置cookie的有效期
resp.addCookie(cookie); //响应给客户端一个cookie
一个网站
cookie
是否存在上限!
聊聊细节问题
一个
Cookie
只能保存一个信息;
一个
web
站点可以给浏览器发送多个
cookie
,最多存放
20
个
cookie
;
Cookie
大小有限制
4kb
;
300
个
cookie
浏览器上限
删除
Cookie
;
不设置有效期,关闭浏览器,自动失效;
设置有效期时间为
0
;
Cookie源码 定义了很多方法
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by Fernflower decompiler)
//
package javax.servlet.http;
import java.io.Serializable;
import java.text.MessageFormat;
import java.util.ResourceBundle;
public class Cookie implements Cloneable, Serializable {
private static final long serialVersionUID = -6454587001725327448L;
private static final String TSPECIALS;
private static final String LSTRING_FILE = "javax.servlet.http.LocalStrings";
private static ResourceBundle lStrings = ResourceBundle.getBundle("javax.servlet.http.LocalStrings");
private String name;
private String value;
private String comment;
private String domain;
private int maxAge = -1;
private String path;
private boolean secure;
private int version = 0;
private boolean isHttpOnly = false;
public Cookie(String name, String value) {
if (name != null && name.length() != 0) {
if (this.isToken(name) && !name.equalsIgnoreCase("Comment") && !name.equalsIgnoreCase("Discard") && !name.equalsIgnoreCase("Domain") && !name.equalsIgnoreCase("Expires") && !name.equalsIgnoreCase("Max-Age") && !name.equalsIgnoreCase("Path") && !name.equalsIgnoreCase("Secure") && !name.equalsIgnoreCase("Version") && !name.startsWith("$")) {
this.name = name;
this.value = value;
} else {
String errMsg = lStrings.getString("err.cookie_name_is_token");
Object[] errArgs = new Object[]{name};
errMsg = MessageFormat.format(errMsg, errArgs);
throw new IllegalArgumentException(errMsg);
}
} else {
throw new IllegalArgumentException(lStrings.getString("err.cookie_name_blank"));
}
}
public void setComment(String purpose) {
this.comment = purpose;
}
public String getComment() {
return this.comment;
}
public void setDomain(String domain) {
this.domain = domain.toLowerCase();
}
public String getDomain() {
return this.domain;
}
public void setMaxAge(int expiry) {
this.maxAge = expiry;
}
public int getMaxAge() {
return this.maxAge;
}
public void setPath(String uri) {
this.path = uri;
}
public String getPath() {
return this.path;
}
public void setSecure(boolean flag) {
this.secure = flag;
}
public boolean getSecure() {
return this.secure;
}
public String getName() {
return this.name;
}
public void setValue(String newValue) {
this.value = newValue;
}
public String getValue() {
return this.value;
}
public int getVersion() {
return this.version;
}
public void setVersion(int v) {
this.version = v;
}
private boolean isToken(String value) {
int len = value.length();
for(int i = 0; i < len; ++i) {
char c = value.charAt(i);
if (c < ' ' || c >= 127 || TSPECIALS.indexOf(c) != -1) {
return false;
}
}
return true;
}
public Object clone() {
try {
return super.clone();
} catch (CloneNotSupportedException var2) {
throw new RuntimeException(var2.getMessage());
}
}
public void setHttpOnly(boolean isHttpOnly) {
this.isHttpOnly = isHttpOnly;
}
public boolean isHttpOnly() {
return this.isHttpOnly;
}
static {
if (Boolean.valueOf(System.getProperty("org.glassfish.web.rfc2109_cookie_names_enforced", "true"))) {
TSPECIALS = "/()<>@,;:\\\"[]?={} \t";
} else {
TSPECIALS = ",; ";
}
}
}
Cookie案例,获取上次访问时间、
package com.mi.servletdemo;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.crypto.Data;
import java.io.IOException;
import java.net.URLEncoder;
import java.text.SimpleDateFormat;
import java.util.Date;
@WebServlet("/ServletDemo2")
public class ServletDemo2 extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=utf-8");
Cookie[] cos = request.getCookies();
boolean flag = false;
if (cos!=null && cos.length>0) {
for (Cookie c:cos) {
// 获取名称判断是否为time
String name = c.getName();
if ("lasttime".equals(name)) {
flag = true;
Date date = new Date();
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String lasttime = simpleDateFormat.format(date);
lasttime = URLEncoder.encode(lasttime,"utf-8");
c.setValue(lasttime);
// 添加进入
response.addCookie(c);
c.setMaxAge(60*60*24);
String value = c.getValue();
response.getWriter().write("上次访问时间为"+value);
}
}
}
if (cos==null || flag == false || cos.length == 0) {
Date date = new Date();
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy--MM--dd HH:mm:ss");
String lasttime = simpleDateFormat.format(date);
lasttime = URLEncoder.encode(lasttime,"utf-8");
Cookie c = new Cookie("lasttime",lasttime);
response.addCookie(c);
c.setMaxAge(60*60*24);
response.getWriter().write("欢迎首次访问");
}
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doPost(request, response);
}
}
Session
(重点)
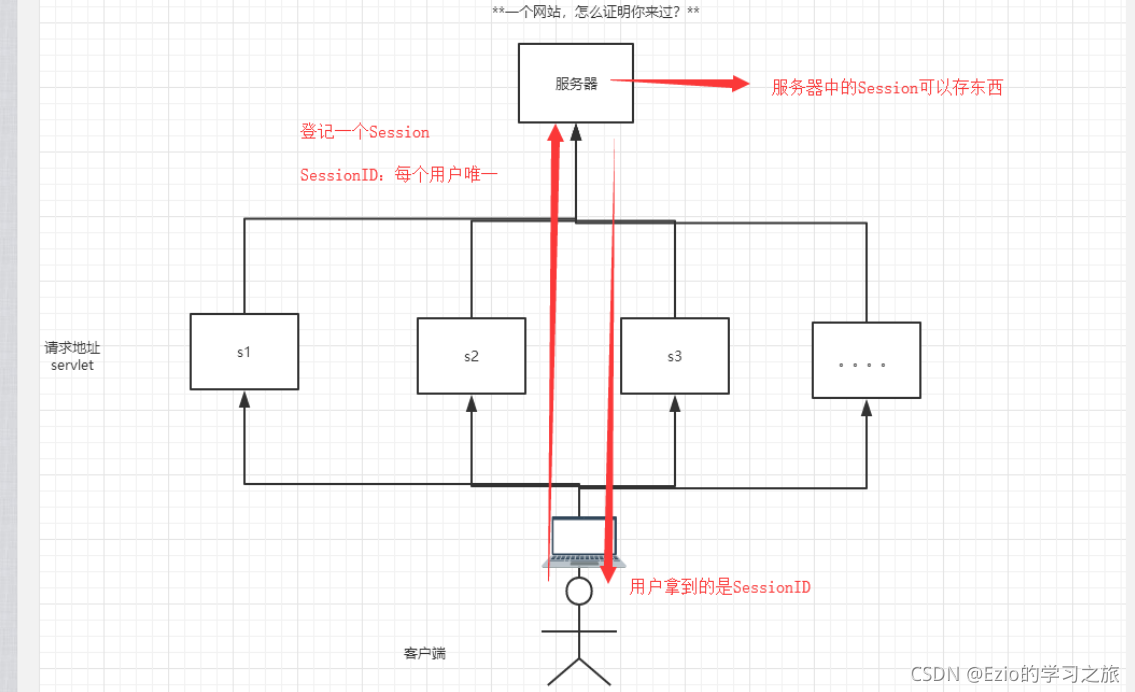
什么是
Session
:
服务器会给每一个用户(浏览器)创建一个
Seesion
对象;
一个
Seesion
独占一个浏览器,只要浏览器没有关闭,这个
Session
就存在;
用户登录之后,整个网站它都可以访问!
-->
保存用户的信息;保存购物车的信息
…..
Session
和
cookie
的区别:
Cookie
是把用户的数据写给用户的浏览器,浏览器保存 (可以保存多个)
Session
把用户的数据写到用户独占
Session
中,服务器端保存
(保存重要的信息,减少服务器资
源的浪费)
Session
对象由服务创建;
Session源码
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by Fernflower decompiler)
//
package javax.servlet.http;
import java.util.Enumeration;
import javax.servlet.ServletContext;
public interface HttpSession {
long getCreationTime();
String getId();
long getLastAccessedTime();
ServletContext getServletContext();
void setMaxInactiveInterval(int var1);
int getMaxInactiveInterval();
/** @deprecated */
HttpSessionContext getSessionContext();
Object getAttribute(String var1);
/** @deprecated */
Object getValue(String var1);
Enumeration<String> getAttributeNames();
/** @deprecated */
String[] getValueNames();
void setAttribute(String var1, Object var2);
/** @deprecated */
void putValue(String var1, Object var2);
void removeAttribute(String var1);
/** @deprecated */
void removeValue(String var1);
void invalidate();
boolean isNew();
}