ts 之 定义类 Class
定义一个简单的 类
class City {
cName:string;
cLevel:number;
constructor(name:string,lever:number){
this.cName = name;
this.cLevel = lever
}
about(){
console.log("我是介绍之-"+this.cName+"等级为-"+this.cLevel);
}
}
let res = new City("小渣亮",10)
console.log("res",res);
res.about()
定义一个数据类的封装
ts / DateHelper.ts
class DateHelper{
dataKey:string;
privateKey:string;
constructor(dataKey:string,privateKey:string){
this.dataKey =dataKey;
this.privateKey = privateKey;
}
readData():any{
let strData:string |null = localStorage.getItem(this.dataKey);
let arrData:any = [];
if ( strData != null ) {
arrData = JSON.parse(strData)
}
return arrData;
}
savaData(arrData:Array<Object>):void{
let str:string = JSON.stringify(arrData);
localStorage.setItem(this.dataKey,str)
}
addData(conStr:string):number{
let arr:any = this.readData();
interface locObject {
[key: string]: any
}
let obj:locObject = {
content:conStr,
}
let newId:any = arr.length > 0 ? arr[arr.length - 1][this.privateKey] + 1: 1;
obj[this.privateKey] = newId;
arr.push(obj)
this.savaData(arr)
return newId;
}
removeDataById(id:string | number):boolean{
let arr = this.readData()
interface locObject {
[key: string]: any
}
let index = arr.findIndex( (ele:locObject) => {
return ele[this.privateKey] == id;
})
if ( index > -1) {
arr.splice(index,1);
this.savaData(arr)
return true;
}
return false;
}
}
自动转化 ts 为js设置
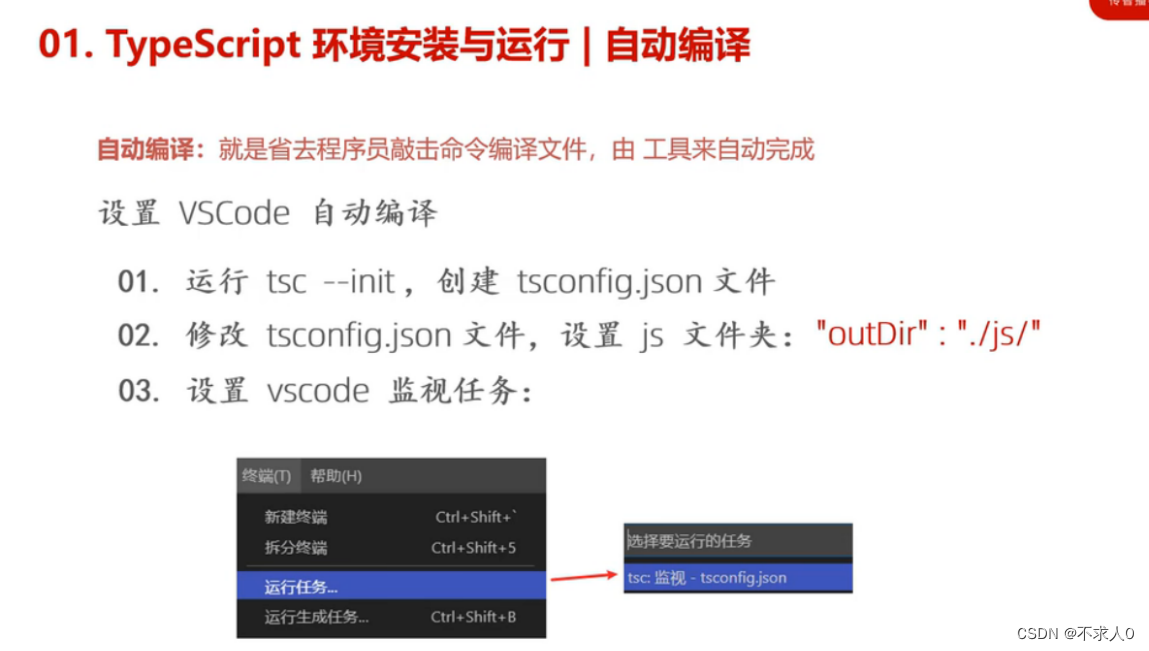
01.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script src="./js/DateHelper.js"></script>
<div>
我是
<div id="apple"></div>
<div>
<input type="text" id="txt">
</div>
<button id="btnOk">发布</button>
</div>
<script>
let db = new DateHelper('plData', 'id');
window.onload = function () {
let arr = db.readData();
console.log("arr", arr);
for (let ele of arr) {
makeDiv(ele.id, ele.content)
}
}
document.querySelector("#btnOk").onclick = () => {
let strCon = document.querySelector("#txt").value
let id = db.addData(strCon);
makeDiv(id, strCon)
document.querySelector("#txt").value = "";
}
function makeDiv(pId, strContent) {
let divRoot = document.querySelector("#apple");
let div = document.createElement("div");
div.innerHTML = pId + '-' + strContent;
divRoot.appendChild(div);
let spanObj = document.createElement("span");
spanObj.innerHTML = '<a href="###">删除<a/>';
spanObj.setAttribute('pId', pId)
spanObj.onclick = remove;
div.appendChild(spanObj)
}
function remove(e) {
let btnDel = e.srcElement;
let conDiv = btnDel.parentNode;
conDiv.parentNode.parentNode.removeChild(conDiv.parentNode)
let id = conDiv.getAttribute('pid');
db.removeDataById(id)
}
</script>
</body>
</html>
效果
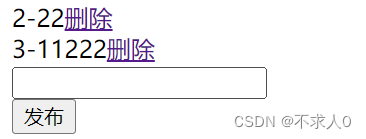