一、定义动物抽象类;定义不同动物继承抽象类;定义一个接口,方法:返回腿的条数;实例化动物类存入数组,遍历输出。
Pet
package com.home;
public abstract class Pet {
private String name;
public Pet(String name) {
this.name = name;
}
public Pet() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public abstract String shout();
}
Inner
package com.home;
public interface Inner {
int length();
}
Cat
package com.home;
public class Cat extends Pet implements Inner{
public Cat(String name) {
super(name);
}
public Cat() {
}
@Override
public int length() {
return 4;
}
@Override
public String shout() {
return "喵呜喵呜";
}
}
Duck
package com.home;
public class Duck extends Pet implements Inner{
public Duck(String name) {
super(name);
}
public Duck() {
}
@Override
public int length() {
return 2;
}
@Override
public String shout() {
return "嘎嘎嘎";
}
}
Penguin
package com.home;
public class Penguin extends Pet implements Inner{
public Penguin(String name) {
super(name);
}
public Penguin() {
}
@Override
public int length() {
return 0;
}
@Override
public String shout() {
return "海豚音";
}
}
Test01
package com.home;
public class Test01 {
public static void main(String[] args) {
Pet [] pets=new Pet[3];
pets[0]=new Cat("猫咪");
pets[1]=new Duck("鸭子");
pets[2]=new Penguin("海豚");
System.out.println("动物名字\t腿的条数\t动物叫");
for (int i=0;i<pets.length;i++) {
if (pets[i] instanceof Cat) {
Cat c = (Cat) pets[i];
System.out.println(c.getName() + "\t\t" + c.length() + "\t\t" + c.shout());
} else if (pets[i] instanceof Duck) {
Duck d = (Duck) pets[i];
System.out.println(d.getName() + "\t\t" + d.length() + "\t\t" + d.shout());
} else if (pets[i] instanceof Penguin) {
Penguin p = (Penguin) pets[i];
System.out.println(p.getName() + "\t\t" + p.length() + "\t\t" + p.shout());
}
}
/* 使用增强for循环
for (Pet pet : pets) {
if (pet instanceof Cat) {
Cat c = (Cat) pet;
System.out.println(c.getName() + "\t\t" + c.length() + "\t\t" + c.shout());
} else if (pet instanceof Duck) {
Duck d = (Duck) pet;
System.out.println(d.getName() + "\t\t" + d.length() + "\t\t" + d.shout());
} else if (pet instanceof Penguin) {
Penguin p = (Penguin) pet;
System.out.println(p.getName() + "\t\t" + p.length() + "\t\t" + p.shout());
}
}*/
}
}
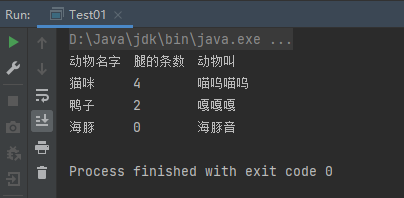
二、模拟银行存取款,基接口存取款查询;接口实现基接口并且有转账方法;创建账户类,属性:名字余额,方法:取款查询。
BankAccount
package com.home.demo02;
public interface BankAccount {
void playIn(int money);
void withdraw(int money);
void getBalance();
}
ITransferBankAccount
package com.home.demo02;
public interface ITransferBankAccount extends BankAccount {
void transferTo(CurrentAccount account1, CurrentAccount account2, int money);
}
CurrentAccount
package com.home.demo02;
public class CurrentAccount implements ITransferBankAccount {
private String name;
private double balance;
public CurrentAccount() {
}
public CurrentAccount(String name, double balance) {
this.name = name;
this.balance = balance;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void setBalance(double balance) {
this.balance = balance;
}
@Override
public void playIn(int money) {
this.balance += money;
}
@Override
public void withdraw(int money) {
this.balance -= money;
}
@Override
public void getBalance() {
System.out.println(balance);
}
@Override
public void transferTo(CurrentAccount account1, CurrentAccount account2, int money) {
account1.setBalance(account1.balance + money);
account2.setBalance(account2.balance - money);
}
}
Test02
package com.home.demo02;
public class Test02 {
public static void main(String[] args) {
CurrentAccount c1 = new CurrentAccount("公主", 10000);
CurrentAccount c2 = new CurrentAccount("护卫", 3000);
c1.transferTo(c2, c1, 5000);
c1.getBalance();
c2.getBalance();
c1.playIn(150000);
c2.withdraw(6000);
c1.getBalance();
c2.getBalance();
}
}
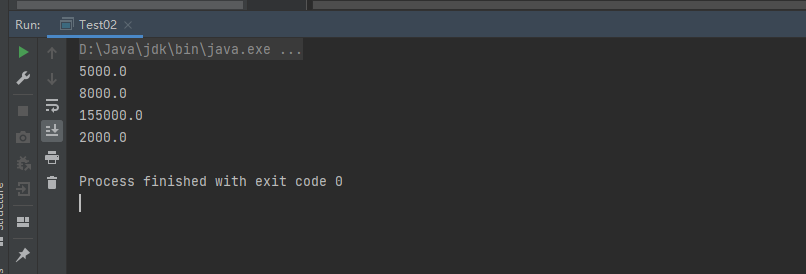
三、定义两个接口,一个实现输出大写英文字母表的功能,一个实现输出小写英文字母表的功能;定义一个实现类去实现两个接口的方法。
InterfaceA
package com.home.demo03;
public interface InterfaceA {
void printA();
}
InterfaceB
package com.home.demo03;
public interface InterfaceB {
void printB();
}
Impl
package com.home.demo03;
public class Impl implements InterfaceA,InterfaceB{
@Override
public void printA() {
for (int i=65;i<=90;i++){
System.out.print((char) i);
}
}
@Override
public void printB() {
for (int i=97;i<=122;i++){
System.out.print((char) i);
}
}
}
Test03
package com.home.demo03;
public class Test03 {
public static void main(String[] args) {
Impl impl=new Impl();
impl.printA();
impl.printB();
}
}
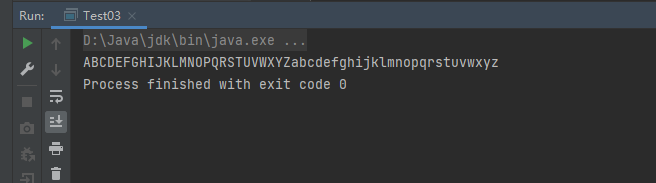