#include <stdio.h>
#include <string.h>
int main()
{
FILE *fp;
char *str = "zhouzhixin shuai";
char readBuf[128] = {0};
// FILE *fopen(const char *path, const char *mode);
fp = fopen("./zhou.txt", "w+");//以W+的方式打开,没有此文件则创建
// size_t fwrite(const void *ptr, size_t size, size_t nmemb,FILE *stream);
fwrite(str, sizeof(char), strlen(str), fp);//一次性写sizeof(char)个写strlen(str)次
//fwrite(str, sizeof(char)*strlen(str), 1, fp);//一次性写sizeof(char)*strlen(str)写1次
fseek(fp, 0, SEEK_SET);//fseek光标
// size_t fread(void *ptr, size_t size, size_t nmemb, FILE *stream);
fread(readBuf, sizeof(char), strlen(str), fp);
// int fseek(FILE *stream, long offset, int whence);
printf("read:%s \n",str);
fclose(fp);
return 0;
}

fopen原型:FILE *fopen(const char *path, const char *mode);
fopen参数:打开哪个文件,以怎样的方式打开
r+ Open for reading and writing. The stream is positioned at the beginning of
the file.
w Truncate file to zero length or create text file for writing. The stream
is positioned at the beginning of the file.
w+ Open for reading and writing. The file is created if it does not exist,
otherwise it is truncated. The stream is positioned at the beginning of
the file.
a Open for appending (writing at end of file). The file is created if it
does not exist. The stream is positioned at the end of the file.
a+ Open for reading and appending (writing at end of file). The file is cre‐
ated if it does not exist. The initial file position for reading is at the
beginning of the file, but output is always appended to the end of the
file.
fwrite原型: size_t fwrite(const void *ptr, size_t size, size_t nmemb,FILE *stream);
ptr:缓存区buf
size:sizeof char 写入一个字符的大小
nmemb:个数
which file:哪个文件
fread同上。
#include <stdio.h>
#include <string.h>
int main()
{
FILE *fp;
char *str = "zhouzhixin shuai";
char readBuf[128] = {0};
// FILE *fopen(const char *path, const char *mode);
fp = fopen("./zhou.txt", "w+");
// size_t fwrite(const void *ptr, size_t size, size_t nmemb,FILE *stream);
int n_write = fwrite(str, sizeof(char), strlen(str), fp);
//fwrite(str, sizeof(char)*strlen(str), 1, fp);
// size_t fread(void *ptr, size_t size, size_t nmemb, FILE *stream);
fseek(fp, 0, SEEK_SET);
int n_read = fread(readBuf, sizeof(char), strlen(str), fp);
// int n_read = fread(readBuf, sizeof(char)*strlen(str), 1, fp);
//int fseek(FILE *stream, long offset, int whence);
printf("read data:%s\n",readBuf);
printf("n_write=%d,n_read=%d\n",n_write,n_read);
fclose(fp);
return 0;
}

注意fread与fwrite的返回值
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
struct Test
{
int a;
char c;
};
int main()
{
FILE *fp;
struct Test data[2] ={{100,'a'},{101,'c'}};
struct Test data2[2];
fp = fopen("./file1", "w+");
// size_t fread(void *ptr, size_t size, size_t nmemb, FILE *stream);
// size_t fwrite(const void *ptr, size_t size, size_t nmemb,FILE *stream);
int n_write = fwrite(&data, sizeof(struct Test), 1, fp);
fseek(fp, 0, SEEK_SET);
int n_read = fread(&data2, sizeof(struct Test), 1, fp);
printf("read %d,%c\n",data[0].a,data[0].c);
printf("read %d,%c\n",data[1].a,data[1].c);
fclose(fp);
return 0;
}
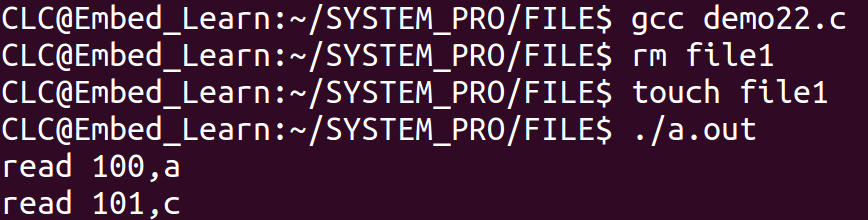
fgetc 原型:int fgetc(FILE *stream)
从指定的流 stream 获取下一个字符(一个无符号字符),并把位置标识符往前移动。
#include <stdio.h>
int main()
{
FILE *fp;
char c;
fp = fopen("./test.txt","r");
while(!feof(fp))
{
c = fgetc(fp);
printf("%c",c);
}
fclose(fp);
return 0;
}
fputc原型:int fputc(int char, FILE *stream)
把参数 char 指定的字符(一个无符号字符)写入到指定的流 stream 中,并把位置标识符往前移动。
#include <stdio.h>
#include <string.h>
int main()
{
FILE *fp;
int i;
char *str = "ni hao shuai !";
int len = strlen(str);
fp = fopen("./test.txt", "w+");
//int fputc(int c, FILE *stream);
for(i=0;i<len;i++)//此处的len需要在strlen(str)之前变化前接收到,错误示范i<strlen(str)
{
fputc(*str,fp);
str++;
}
fclose(fp);
return 0;
}
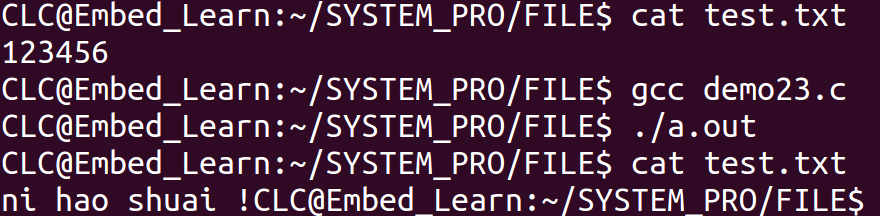
feof原型:int feof(FILE *stream)
测试给定流 stream 的文件结束标识符。
#include <stdio.h>
int main()
{
FILE *fp;
char c;
fp = fopen("./test.txt","r");
while(!feof(fp))
{
c = fgetc(fp);
printf("%c",c);
}
fclose(fp);
return 0;
}
//返回值
//当设置了与流关联的文件结束标识符时,该函数返回一个非零值,否则返回零