制作页面跳转
一、页面构成
每个页面都由三个文件构成,分别是css,hml,js。三者之间关系如下图1所示:
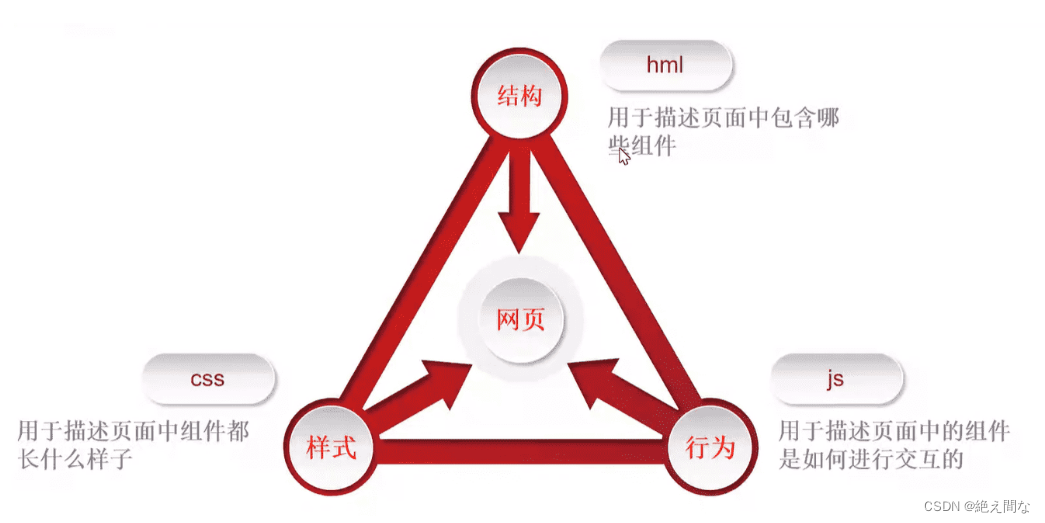
- hml:定义页面中存在的组件
- css:定义组件的形状,大小等格式参数
- js :定义组件间的交互
二、预备语法
1.button组件
由于在页面切换时会使用到button组件,在华为官网(如下)查看基本语法。
hml文件中定义:
<!-- xxx.hml --> <div class="container"> <button class="circle" type="circle" >+</button> <button class="text" type="text"> button</button> </div>
css文件中设定格式:
/* xxx.css */ .container { width: 100%; height: 100%; background-color: #F1F3F5; flex-direction: column; align-items: center; justify-content: center; } .circle { font-size: 120px; background-color: blue; radius: 72px; } .text { margin-top: 30px; text-color: white; font-size: 30px; font-style: normal; background-color: blue; width: 50%; height: 100px; }
button开发指导-基础组件-常见组件开发指导-基于JS扩展的类Web开发范式-UI开发-基于JS开发-开发-HarmonyOS应用开发
2.事件绑定
事件通过'on'或者'@'绑定在组件上,当组件触发事件时会执行JS文件中对应的事件处理函数。
通过事件绑定按钮,从而按下按钮时页面跳转。在华为官网(如下)查看基本语法。
<!-- xxx.hml --> <div class="container"> <text class="title">{{count}}</text> <div class="box"> <input type="button" class="btn" value="increase" onclick="increase" /> <input type="button" class="btn" value="decrease" @click="decrease" /> <!-- 传递额外参数 --> <input type="button" class="btn" value="double" @click="multiply(2)" /> <input type="button" class="btn" value="decuple" @click="multiply(10)" /> <input type="button" class="btn" value="square" @click="multiply(count)" /> </div> </div>
// xxx.js export default { data: { count: 0 }, increase() { this.count++; }, decrease() { this.count--; }, multiply(multiplier) { this.count = multiplier * this.count; } };
HML语法参考-语法-框架说明-基于JS扩展的类Web开发范式-UI开发-基于JS开发-开发-HarmonyOS应用开发
3.页面跳转
使用router.push进行页面跳转
三、实践
做完以上的准备工作后,可以开始制作本次的作业。
在此仅书写步骤:
- 新建项目;
- 在/entry/src/main/js/MainAbility/pages中建立两个页面文件夹first和second,并新建三个文件;
- 在hml中定义部件;
- 在css中定义属性;
- 在js中写入跳转程序。
注:在运行前须在entry/src/main/config.json中进行配置,将自行创建的页面加入,否则会产生如下图3报错
"js": [ { "pages": [ "pages/index/index", "pages/second/second" ], "name": ".MainAbility", "window": { "designWidth": 720, "autoDesignWidth": false } } ]
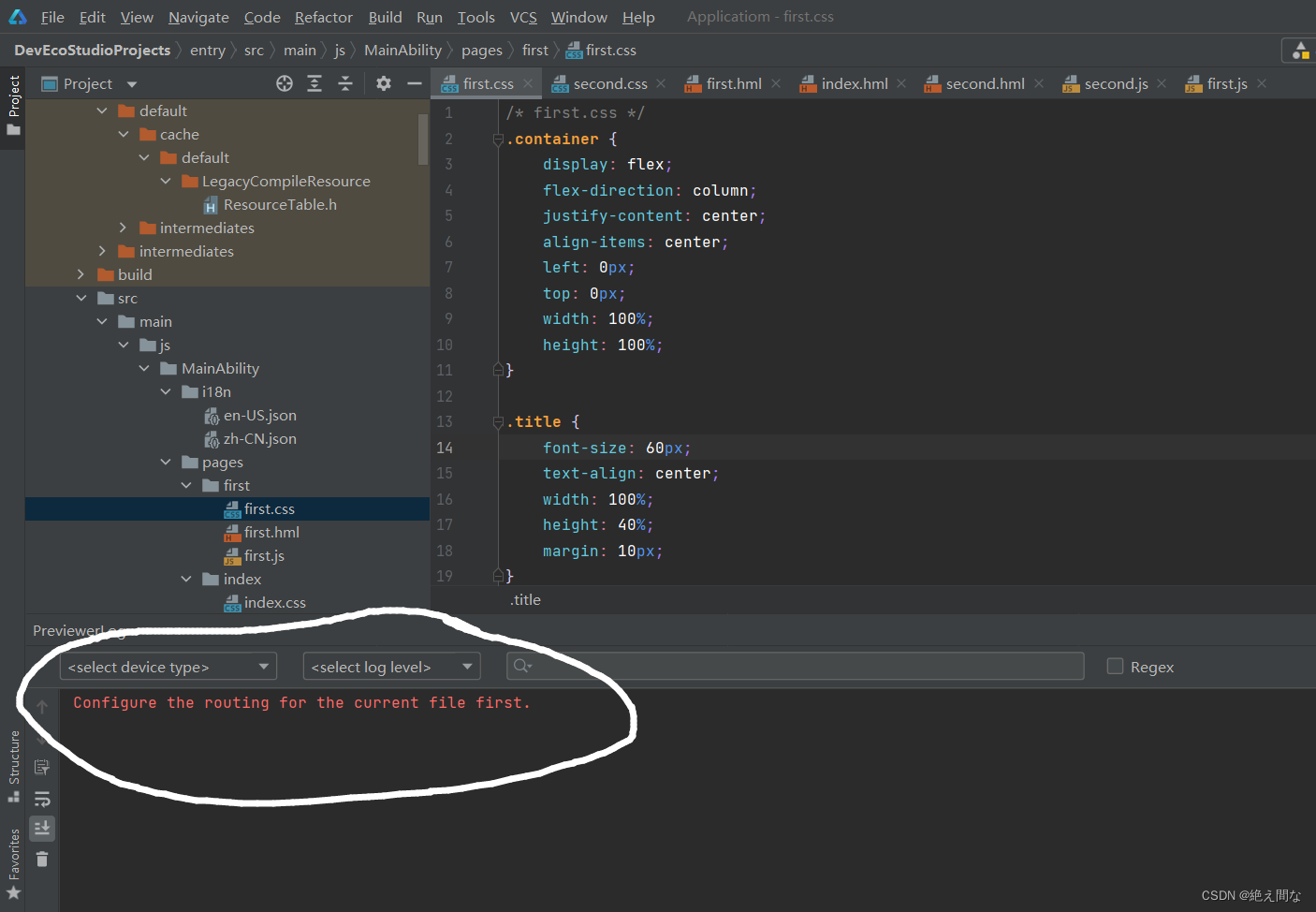
四、结果及代码
在此附上结果和各文件代码
结果图:
gif粘不上,算了
代码:
<!-- first.hml --> <div class="container"> <text class="title"> 魔幻旅程 </text> <input type="button" class="btn" value="启程" onclick="To_second" /> </div>
/* first.css */ .container { display: flex; flex-direction: column; justify-content: center; align-items: center; left: 0px; top: 0px; width: 100%; height: 100%; } .title { font-size: 60px; text-align: center; width: 100%; height: 40%; margin: 10px; } .btn { font-size: 40px; text-align: center; width: 30%; height: 7%; margin: 10px; } @media screen and (orientation: landscape) { .title { font-size: 60px; } } @media screen and (device-type: tablet) and (orientation: landscape) { .title { font-size: 100px; } }
//first.js import router from '@system.router'; export default{ To_second(){ router.push({ uri:"pages/second/second" }) } }
<!-- second.hml --> <div class="container"> <text class="title"> 发生错误 </text> <input type="button" class="btn" value="重试" onclick="To_first" /> </div>
/* second.css */ .container { display: flex; flex-direction: column; justify-content: center; align-items: center; left: 0px; top: 0px; width: 100%; height: 100%; } .title { font-size: 60px; text-align: center; width: 100%; height: 40%; margin: 10px; } .btn { font-size: 40px; text-align: center; width: 30%; height: 7%; margin: 10px; } @media screen and (orientation: landscape) { .title { font-size: 60px; } } @media screen and (device-type: tablet) and (orientation: landscape) { .title { font-size: 100px; } }
//second.js import router from '@system.router'; export default{ To_first(){ router.push({ uri:"pages/first/first" }) } }