
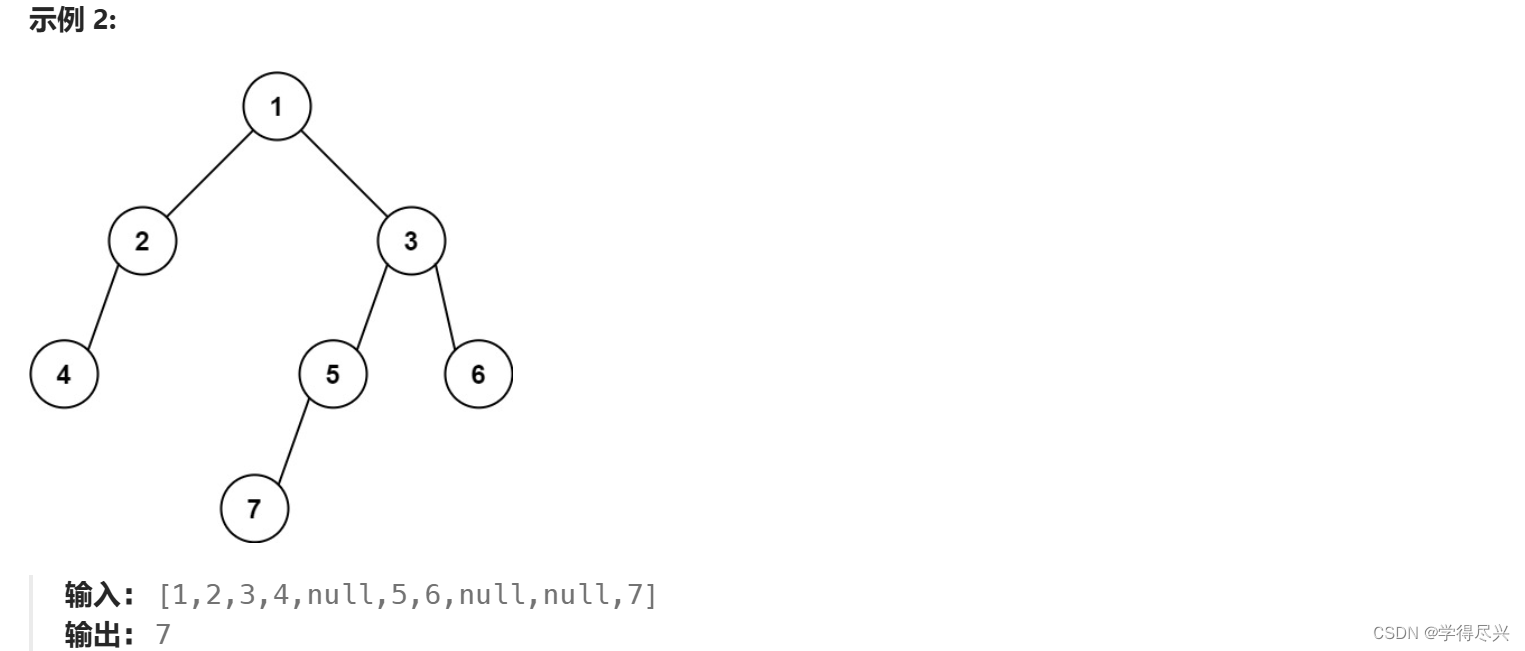
class Solution { //用层序遍历进行求解
public:
int findBottomLeftValue(TreeNode* root) {
int res; if(root == nullptr) return -1;
queue<TreeNode*> que; que.push(root);
while(!que.empty()){
int que_size = que.size();
for(int i = 0; i < que_size; i++){
TreeNode* temp = que.front(); que.pop();
if(i == 0) res = temp->val;
if(temp->left != nullptr) que.push(temp->left);
if(temp->right != nullptr) que.push(temp->right);
}
}
return res;
}
};
class Solution { //用递归法求解
public:
int findBottomLeftValue(TreeNode* root) {
if(root == nullptr) return -1;
int res, res_dep = 0;
traverse(root, res, res_dep, 1);
return res;
}
void traverse(TreeNode* cur, int& res, int& res_dep, int depth){
if(cur->left == nullptr && cur->right == nullptr){
if(depth > res_dep) {res = cur->val; res_dep = depth;}
return ;
}
if(cur->left != nullptr){
traverse(cur->left, res, res_dep, depth + 1); // 隐藏着回溯
}
if(cur->right != nullptr){
traverse(cur->right, res, res_dep, depth + 1); // 隐藏着回溯
}
}
};
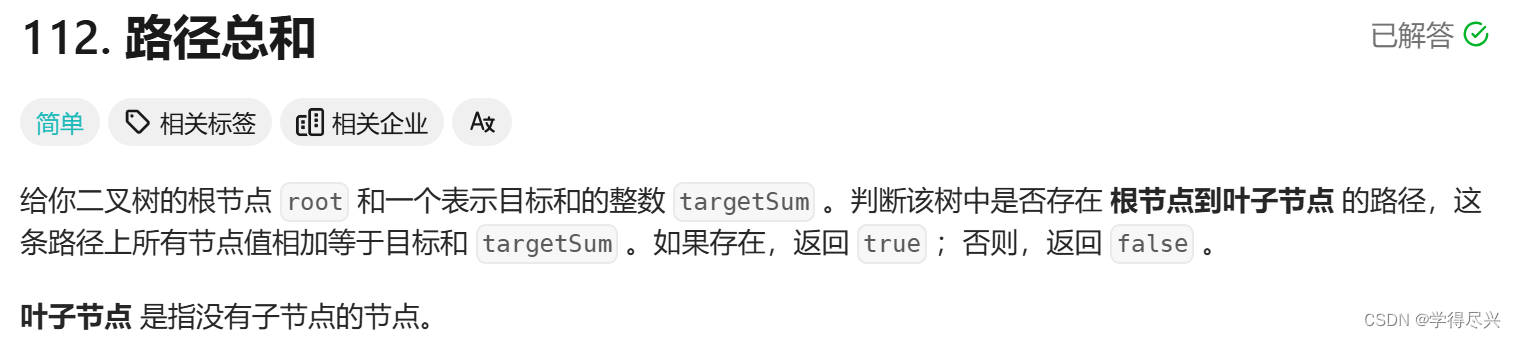
class Solution {
int sum = 0;
public:
bool hasPathSum(TreeNode* root, int targetSum) {
if(root == nullptr) return false;
if(root->left == nullptr && root->right == nullptr){
sum += root->val;
if(sum == targetSum) {sum -= root->val;return true;}
else {sum -= root->val; return false;}
}
bool left = false, right = false;
if(root->left != nullptr) {
sum += root->val;
left = hasPathSum(root->left, targetSum);
sum -= root->val;
}
if(root->right != nullptr) {
sum += root->val;
right = hasPathSum(root->right, targetSum);
sum -= root->val;
}
return left || right;
}
};

class Solution {
public:
TreeNode* buildTree(vector<int>& inorder, vector<int>& postorder) {
if(inorder.empty()) return nullptr;
int tree_size = inorder.size();
TreeNode* root = new TreeNode(postorder[tree_size - 1]);
if(tree_size == 1) return root; //减枝操作,去掉这句也行
int cut_index;
for(cut_index = 0; cut_index < tree_size; cut_index++)
if(inorder[cut_index] == root->val) break;
// 切割中序数组
// 左闭右开区间:[0, cut_index)
vector<int> inorder_left(inorder.begin(), inorder.begin() + cut_index);
// 左闭右开区间:[cut_index + 1, end)
vector<int> inorder_right(inorder.begin() + cut_index + 1, inorder.end());
// 切割后序数组
// 左闭右开区间:[0, cut_index)
vector<int> postorder_left(postorder.begin(), postorder.begin() + cut_index);
// 左闭右开区间:[cut_index, end - 1)!!!!!
vector<int> postorder_right(postorder.begin() + cut_index, postorder.end() - 1);
root->left = buildTree(inorder_left, postorder_left);
root->right = buildTree(inorder_right, postorder_right);
return root;
}
};