一、SharedPreferences 框架体系
1、SharedPreferences 基本介绍
-
SharedPreferences 是 Android 的一个轻量级存储工具,它采用
key - value
的键值对方式进行存储 -
它允许保存和读取应用中的基本数据类型,例如,String、int、float、boolean 等
-
保存共享参数键值对信息的文件路径为:
/data/data/【应用包名】/shared_prefs/【SharedPreferences 文件名】.xml
2、SharedPreferences 使用步骤
(1)获取 SharedPreferences 实例
-
其中,fileName 是为 SharedPreferences 文件指定的名称
-
mode 是文件的操作模式,通常是
MODE_PRIVATE
(私有模式)
SharedPreferences sharedPreferences = context.getSharedPreferences(【fileName】, 【mode】);
(2)写入数据
-
使用
SharedPreferences.Editor
来编辑数据,通过 SharedPreferences 实例的 edit 方法获取 Editor 对象 -
然后使用 put 相关方法来添加或修改数据,当
key - value
不存在时为添加,当key - value
存在时为修改 -
最后调用 commit 方法来提交更改
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.putString(【key】, 【value】);
edit.commit();
(3)读取数据
-
通过 SharedPreferences 实例的 get 相关方法来读取数据
-
如果 key 不存在,则返回 defValue 默认值
sharedPreferences.getString(【key】, 【defValue】);
3、SharedPreferences 使用优化思路
-
在使用 SharedPreferences 时,我们往往只关注一存一取,即我们往往只关注【key】和【value】
-
我们往往不关注【context】、【fileName】、【mode】、【defValue】,在使用一一指定这些感觉过于繁琐
4、SharedPreferences 框架体系
- 使用 SharedPreferences 框架体系,可以优化 SharedPreferences 的使用,增强 SharedPreferences 相关业务代码的可维护性,SharedPreferences 框架体系分为以下三部分
(1)SharedPreferences 工具类
-
封装原始的 SharedPreferences 操作(存、取)
-
简化掉【mode】和【defValue】
(2)SPStore
-
定义好要使用的 【fileName】和【key】,这些不让外部随意指定
-
将每个【key】对应的存取操作其封装成 get 和 set 方法
-
简化掉【fileName】
(3)MyApplication + CommonStore
-
扩展 SPStore 中的 get 和 set 方法(减少对 SharedPreferences 文件的直接操作、更灵活的定义默认值),同时传入 context
-
简化掉【context】
二、SharedPreferences 框架体系具体实现
1、SharedPreferences 工具类
- MySPTool.java
/**
* SharedPreferences 工具类
*/
public class MySPTool {
/**
* 存 String 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
* @param value 键值
*/
public static void setString(Context context, String fileName, String key, String value) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.putString(key, value);
edit.commit();
}
/**
* 取 String 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
* @return
*/
public static String getString(Context context, String fileName, String key) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
return sharedPreferences.getString(key, "");
}
// ----------------------------------------------------------------------------------------------------
/**
* 存 int 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
* @param value 键值
*/
public static void setInt(Context context, String fileName, String key, int value) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.putInt(key, value);
edit.commit();
}
/**
* 取 int 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
* @return
*/
public static int getInt(Context context, String fileName, String key) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
return sharedPreferences.getInt(key, -1);
}
// ----------------------------------------------------------------------------------------------------
/**
* 存 float 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
* @param value 键值
*/
public static void setFloat(Context context, String fileName, String key, float value) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.putFloat(key, value);
edit.commit();
}
/**
* 取 float 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
* @return
*/
public static float getFloat(Context context, String fileName, String key) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
return sharedPreferences.getFloat(key, -1);
}
// ----------------------------------------------------------------------------------------------------
/**
* 存 boolean 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
* @param value 键值
*/
public static void setBoolean(Context context, String fileName, String key, boolean value) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.putBoolean(key, value);
edit.commit();
}
/**
* 取 boolean 类型的数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键值
* @return
*/
public static boolean getBoolean(Context context, String fileName, String key) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
return sharedPreferences.getBoolean(key, false);
}
// ----------------------------------------------------------------------------------------------------
/**
* 删除数据
*
* @param context 上下文对象
* @param fileName 文件名
* @param key 键名
*/
public static void remove(Context context, String fileName, String key) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.remove(key);
edit.commit();
}
/**
* 删除所有数据
*
* @param fileName 文件名
* @param context 上下文对象
*/
public static void clear(Context context, String fileName) {
SharedPreferences sharedPreferences = context.getSharedPreferences(fileName, Context.MODE_PRIVATE);
SharedPreferences.Editor edit = sharedPreferences.edit();
edit.clear();
edit.commit();
}
}
2、SPStore
- SPStore.java
public class SPStore {
private static final String SP_NAME = "test";
// ----------------------------------------------------------------------------------------------------
private static final String NAME_KEY = "name";
private static final String AGE_KEY = "age";
// ====================================================================================================
public static String getName(Context context) {
return MySPTool.getString(context, SP_NAME, NAME_KEY);
}
public static void setName(Context context, String name) {
MySPTool.setString(context, SP_NAME, NAME_KEY, name);
}
public static int getAge(Context context) {
return MySPTool.getInt(context, SP_NAME, AGE_KEY);
}
public static void setAge(Context context, int age) {
MySPTool.setInt(context, SP_NAME, AGE_KEY, age);
}
}
3、MyApplication + CommonStore
- MyApplication.java
public class MyApplication extends Application {
public static final String TAG = MyApplication.class.getSimpleName();
private static Context context;
@Override
public void onCreate() {
super.onCreate();
context = this;
}
public static Context getContext() {
return context;
}
}
- CommonStore.java
public class CommonStore {
private static String name;
private static Integer age;
// ====================================================================================================
public static String getName() {
if (name == null) {
String spName = SPStore.getName(MyApplication.getContext());
name = spName;
}
return name;
}
public static void setName(String inputName) {
SPStore.setName(MyApplication.getContext(), inputName);
name = inputName;
}
public static Integer getAge() {
if (age == null) {
int spAge = SPStore.getAge(MyApplication.getContext());
if (spAge == -1) spAge = 0;
age = spAge;
}
return age;
}
public static void setAge(Integer inputAge) {
SPStore.setAge(MyApplication.getContext(), inputAge);
age = inputAge;
}
}
4、测试
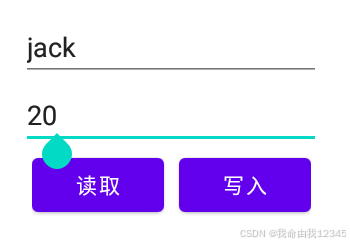
- activity_sp_test.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".SpTestActivity"
tools:ignore="MissingConstraints">
<LinearLayout
android:id="@+id/ll_content"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:orientation="vertical"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<EditText
android:id="@+id/et_name"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:inputType="text" />
<EditText
android:id="@+id/et_age"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:inputType="number" />
</LinearLayout>
<LinearLayout
android:id="@+id/ll_btns"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ll_content">
<Button
android:id="@+id/btn_read"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读取" />
<Button
android:id="@+id/btn_write"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:text="写入" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
- SpTestActivity.java
public class SpTestActivity extends AppCompatActivity {
private Button btnRead;
private Button btnWrite;
private EditText etName;
private EditText etAge;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sp_test);
btnRead = findViewById(R.id.btn_read);
btnWrite = findViewById(R.id.btn_write);
etName = findViewById(R.id.et_name);
etAge = findViewById(R.id.et_age);
btnRead.setOnClickListener(v -> {
String name = CommonStore.getName();
Integer age = CommonStore.getAge();
etName.setText(name);
etAge.setText(String.valueOf(age));
});
btnWrite.setOnClickListener(v -> {
String inputName = etName.getText().toString();
if (inputName == null || inputName.equals("")) {
Toast.makeText(this, "存入的 name 不合法", Toast.LENGTH_SHORT).show();
return;
}
String inputAgeStr = etAge.getText().toString();
int inputAge = -1;
try {
inputAge = Integer.parseInt(inputAgeStr);
} catch (NumberFormatException e) {
e.printStackTrace();
}
if (inputAge < 0) {
Toast.makeText(this, "存入的 age 不合法", Toast.LENGTH_SHORT).show();
return;
}
CommonStore.setName(inputName);
CommonStore.setAge(inputAge);
});
}
}