- 点击添加矩形按钮可在白色区域中心添加一个矩形;
- 点击添加圆形按钮可在白色区域中心添加一个圆形;
- 鼠标点击图形选中图形;
- 鼠标按下可拖拽图形;
- 鼠标弹起结束拖拽;
- 点击删除按钮可删除当前选中图形;
- 点击变色按钮实现变色功能;
- 点击旋转按钮实现旋转功能;
- 点击缩放按钮实现对应功能;
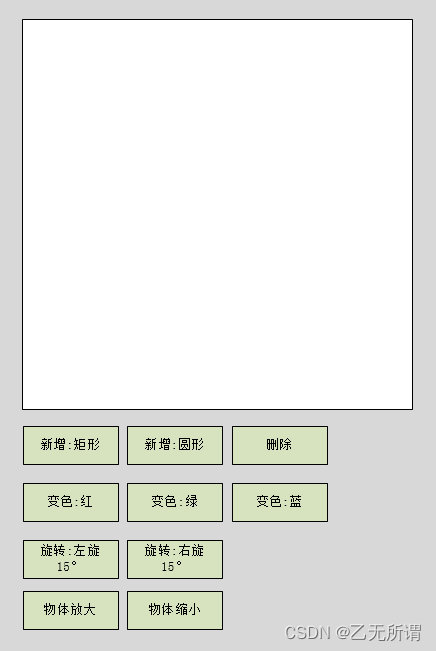
代码实现
package Daily
{
import flash.display.Sprite;
import flash.events.MouseEvent;
import flash.text.TextField;
import flash.text.TextFormat;
import flash.geom.ColorTransform;
public class ButtonClick extends Sprite
{
public var label:String;
public static const btnColor:Number = 0XB6D7A8;
public static const bgColor:Number = 0x999999;
public function ButtonClick()
{
var btnWidth:Number = stage.stageWidth/7;
var btnHeight:Number = stage.stageHeight/24;
var bx:Number = stage.stageWidth;
var by:Number = stage.stageHeight;
var shapes:Array = []; // 用于存储所有图形对象
var selectedShape:Sprite; // 用于存储当前选中的图形对象
var frequency:uint = 0;
//创建画布
var bg:Sprite = new Sprite();
bg.graphics.beginFill(bgColor);
bg.graphics.drawRect(0, 0, bx, by);
addChild(bg);
addChildAt(bg,0);
var fk:Sprite = new Sprite();
fk.graphics.beginFill(0xFFFFFF); // 白色
fk.graphics.drawRect(0.05*bx,0.05*by,0.9*bx,0.5*by); // 大小
fk.graphics.endFill();
addChildAt(fk,1);
//创建按钮
var addRectBtn:Sprite = new DrawButton("新增:矩形", btnWidth, btnHeight, 0.1*bx, 0.6*by, bg);
var addCircleBtn:Sprite = new DrawButton("新增:圆形",btnWidth, btnHeight,0.25*bx, 0.6*by, bg);
var dropBtn:Sprite = new DrawButton("清空",btnWidth, btnHeight, 0.1*bx, 0.65*by, bg);
var deleteBtn:Sprite = new DrawButton("删除选中图形",btnWidth, btnHeight, 0.25*bx, 0.65*by, bg);
var chaRedBtn:Sprite = new DrawButton("变色:红",btnWidth, btnHeight, 0.1*bx, 0.7*by, bg);
var chaGreenBtn:Sprite = new DrawButton("变色:绿",btnWidth, btnHeight, 0.25*bx,0.7*by, bg);
var chaBlueBtn:Sprite = new DrawButton("变色:蓝",btnWidth, btnHeight, 0.4*bx, 0.7*by, bg);
var levorotationBtn:Sprite = new DrawButton("旋转:左旋15°",btnWidth, btnHeight, 0.1*bx, 0.75*by, bg);
var dextrorotationBtn:Sprite = new DrawButton("旋转:右旋15°",btnWidth, btnHeight, 0.25*bx, 0.75*by, bg);
var blowUpBtn:Sprite = new DrawButton("物体放大",btnWidth, btnHeight, 0.1*bx, 0.8*by, bg);
var minificationBtn:Sprite = new DrawButton("物体缩小",btnWidth, btnHeight, 0.25*bx, 0.8*by, bg);
//注册鼠标点击事
addRectBtn.addEventListener(MouseEvent.CLICK, addRectangle);
addCircleBtn.addEventListener(MouseEvent.CLICK, addCircle);
deleteBtn.addEventListener(MouseEvent.CLICK, deleteShape);
dropBtn.addEventListener(MouseEvent.CLICK, dropShape);
chaRedBtn.addEventListener(MouseEvent.CLICK, changeRedColor);
chaGreenBtn.addEventListener(MouseEvent.CLICK, changeGreenColor);
chaBlueBtn.addEventListener(MouseEvent.CLICK, changeBlueColor);
levorotationBtn.addEventListener(MouseEvent.CLICK, levorotationShape);
dextrorotationBtn.addEventListener(MouseEvent.CLICK, dextrorotationShape);
blowUpBtn.addEventListener(MouseEvent.CLICK, blowUpShape);
minificationBtn.addEventListener(MouseEvent.CLICK, minificationShape);
/*功能(方法)区*/
//点击按钮处理函数
/*
添加按钮
*/
//创建按钮
function DrawButton(label:String,buttonWidth:Number,buttonHeight:Number,buttonX:Number,buttonY:Number,bg:Sprite):Sprite{
var btn:Sprite = new Sprite();
btn.graphics.beginFill(btnColor);
btn.graphics.drawRect(0,0,buttonWidth,buttonHeight);
btn.x = buttonX;
btn.y = buttonY;
btn.graphics.endFill();
btn.buttonMode = true;
bg.addChild(btn);
//创建按钮文本
var btnLabel:TextField = new TextField();
btnLabel.text = label;
btn.addChild(btnLabel);
var textFormat:TextFormat = new TextFormat();
textFormat.size = 10;
btnLabel.defaultTextFormat = textFormat;
btnLabel.setTextFormat(textFormat);
return btn;
}
/*
按钮添加图形
*/
//按钮添加矩形
function addRectangle(event:MouseEvent):void {
var rectangle:Sprite = new Sprite();
rectangle.graphics.beginFill(0x444444);
rectangle.graphics.drawRect(0, 0, 0.08*bx, 0.04*by); // 矩形大小
rectangle.graphics.endFill();
rectangle.x = Math.random() * 0.8*bx; // 随机位置
rectangle.y = Math.random() * 0.55*by;
addChild(rectangle);
shapes.push(rectangle);
rectangle.addEventListener(MouseEvent.MOUSE_DOWN, selectShape);
}
//按钮添加圆形
function addCircle(event:MouseEvent):void {
var rectangle:Sprite = new Sprite();
rectangle.graphics.beginFill(0x444444);
rectangle.graphics.drawCircle(0,0,0.04*by); // 圆形大小
rectangle.graphics.endFill();
rectangle.x = Math.random() * 0.7*bx; // 随机位置
rectangle.y = Math.random() * 0.55*by;
addChild(rectangle);
shapes.push(rectangle);
rectangle.addEventListener(MouseEvent.MOUSE_DOWN, selectShape);
}
/*
按钮删除图形
*/
//按钮删除图形
function deleteShape(event:MouseEvent):void {
if (selectedShape && contains(selectedShape)) {
removeChild(selectedShape);
shapes.splice(shapes.indexOf(selectedShape), 1);
selectedShape = null;
}
}
//按钮清空图形
function dropShape(event:MouseEvent):void {
for each (var shape:Sprite in shapes) {
if (contains(shape)) {
removeChild(shape);
}
}
shapes = [];
selectedShape = null;
}
/*
按钮改变颜色(红绿蓝)
*/
function changeRedColor(event:MouseEvent):void {
if(selectedShape){
// 创建一个 ColorTransform 对象
var colorTransform:ColorTransform = new ColorTransform();
// 设置颜色变换
colorTransform.color = 0xFF0000; // 红色
// 将颜色变换应用到显示对象
selectedShape.transform.colorTransform = colorTransform;
}else
return;
}
function changeGreenColor(event:MouseEvent):void {
if(selectedShape){
// 创建一个 ColorTransform 对象
var colorTransform:ColorTransform = new ColorTransform();
// 设置颜色变换
colorTransform.color = 0x00FF00; // 绿色
// 将颜色变换应用到显示对象
selectedShape.transform.colorTransform = colorTransform;
}else
return;
}
function changeBlueColor(event:MouseEvent):void {
if(selectedShape){
// 创建一个 ColorTransform 对象
var colorTransform:ColorTransform = new ColorTransform();
// 设置颜色变换
colorTransform.color = 0x00FFFF; // 蓝色
// 将颜色变换应用到显示对象
selectedShape.transform.colorTransform = colorTransform;
}else
return;
}
/*
按钮旋转
*/
// 左旋转15度
function levorotationShape(event:MouseEvent):void {
if (selectedShape) {
selectedShape.rotation -= 15;
}
}
// 右旋转15度
function dextrorotationShape(event:MouseEvent):void {
if (selectedShape) {
selectedShape.rotation += 15;
}
}
/*
按钮缩放
*/
//放大
function blowUpShape(event:MouseEvent):void {
if (selectedShape) {
selectedShape.scaleX *= 1.2; // 水平方向缩放
selectedShape.scaleY *= 1.2; // 垂直方向缩放
}
}
//缩小
function minificationShape(event:MouseEvent):void {
if (selectedShape) {
selectedShape.scaleX *= 0.8; // 水平方向缩放
selectedShape.scaleY *= 0.8; // 垂直方向缩放
}
//缩小
}
/*
鼠标拖拽
*/
//点击鼠标,开启拖拽
function selectShape(event:MouseEvent):void {
selectedShape = Sprite(event.currentTarget);
setChildIndex(selectedShape, numChildren - 1); // 将选中的图形置于顶层
selectedShape.startDrag(); //开启拖拽
selectedShape.addEventListener(MouseEvent.MOUSE_UP, releaseShape);
}
//释放鼠标按钮,关闭拖拽
function releaseShape(event:MouseEvent):void {
if (selectedShape) {
selectedShape.stopDrag();
}
}
}
}
}