宿舍管理系统是面向学生与宿管人员的系统,目的是为了提高学校对学生宿舍的管理。本系统主要包括:学生模块、宿管模块、楼宇模块、宿舍模块、迁入迁出模块等。
前端方面利用vue.js、elementui简化组件设计,使用axios完成前后端交互,有学生、宿管、楼宇、宿舍模块,实现学生、宿管、楼宇、宿舍的增删改查询及学生入住迁出的功能。
项目主要负责的工作:完成楼宇模块、宿舍模块的设计,实现楼宇及宿舍的添加删除修改查询功能。
首页
1.项目目录
1.楼宇模块
1.1楼宇添加
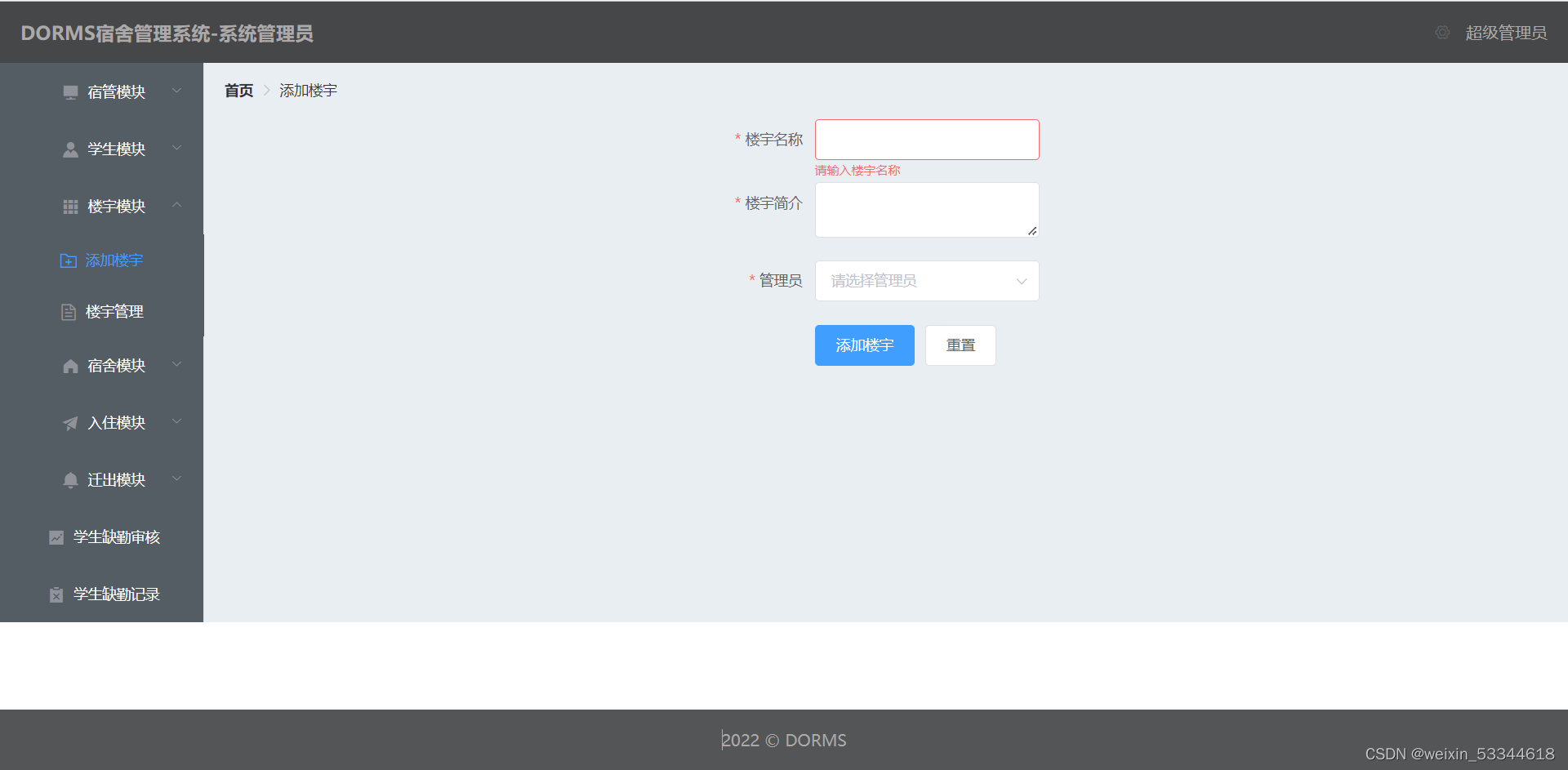
<template>
<div class="buildingAdd">
<el-form :model="buildingForm" :rules="rules" ref="buildingForm" label-width="80px" class="demo-ruleForm">
<el-form-item label="楼宇名称" prop="name">
<el-input v-model="buildingForm.name"></el-input>
</el-form-item>
<el-form-item label="楼宇简介" prop="introduction">
<el-input type="textarea" v-model="buildingForm.introduction"></el-input>
</el-form-item>
<el-form-item label="管理员" prop="adminId">
<el-select v-model="buildingForm.adminId" placeholder="请选择管理员">
<el-option v-for="item in dormitoryAdminList" :key="item.id" :label="item.name" :value="item.id"></el-option>
</el-select>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('buildingForm')">添加楼宇</el-button>
<el-button @click="resetForm('buildingForm')">重置</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
export default {
name: "BuildingAdd",
data() {
return {
buildingForm: {
name: '',
introduction: '',
adminId: '' //宿管ID
},
dormitoryAdminList: [], //所有的宿管
rules: {
name: [
{ required: true, message: '请输入楼宇名称', trigger: 'blur' }
],
introduction: [
{ required: true, message: '请输入楼宇简介', trigger: 'blur' }
],
adminId: [
{ required: true, message: '请选择宿管', trigger: 'change' }
]
}
}
},
created() {
//查询所有的楼宇
this.getDormitoryAdminList();
},
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
console.log(this.buildingForm)
//添加楼宇
const _this = this;
axios.post("http://116.62.204.4:8001/dormitory/building/add", _this.buildingForm)
.then((resp => {
console.log(resp.data);
if (resp.data.code === 0) {
//添加楼宇成功
//提示用户添加成功
_this.$message({
message: resp.data.message,
type: 'success'
});
//跳转到楼宇列表页面
_this.$router.push('/buildingList')
}
if (resp.data.code === 1) {
//添加楼宇失败
_this.$message.error(resp.data.message);
//清空文本输入框
_this.buildingForm = {};
}
if (resp.data.code === -1) {
//表示该楼宇已经存在
//提示用户不要重复添加
_this.$message({
message: resp.data.message,
type: 'warning'
});
}
}));
} else {
console.log('error submit!!');
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
},
//查询所有的宿管
getDormitoryAdminList() {
const _this = this;
axios.get("http://116.62.204.4:8001/dormitory/dormitory-admin/getDormitoryAdmin")
.then((resp) => {
console.log(resp.data.data);
_this.dormitoryAdminList = resp.data.data;
});
},
}
}
</script>
<style scoped>
.buildingAdd {
margin: 20px auto;
/*border: 1px solid #42b983;*/
width: 300px;
}
</style>
1.2楼宇管理
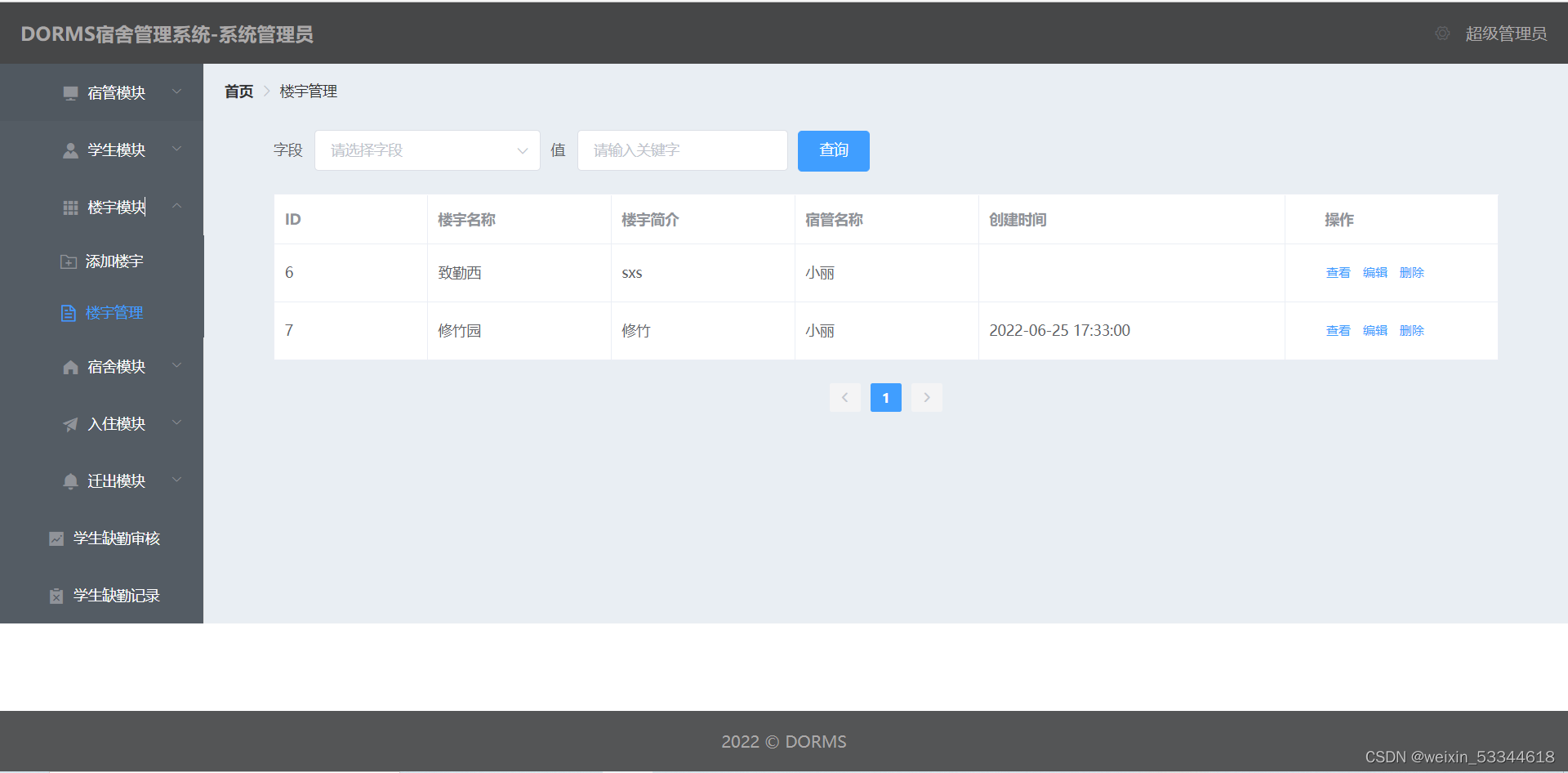
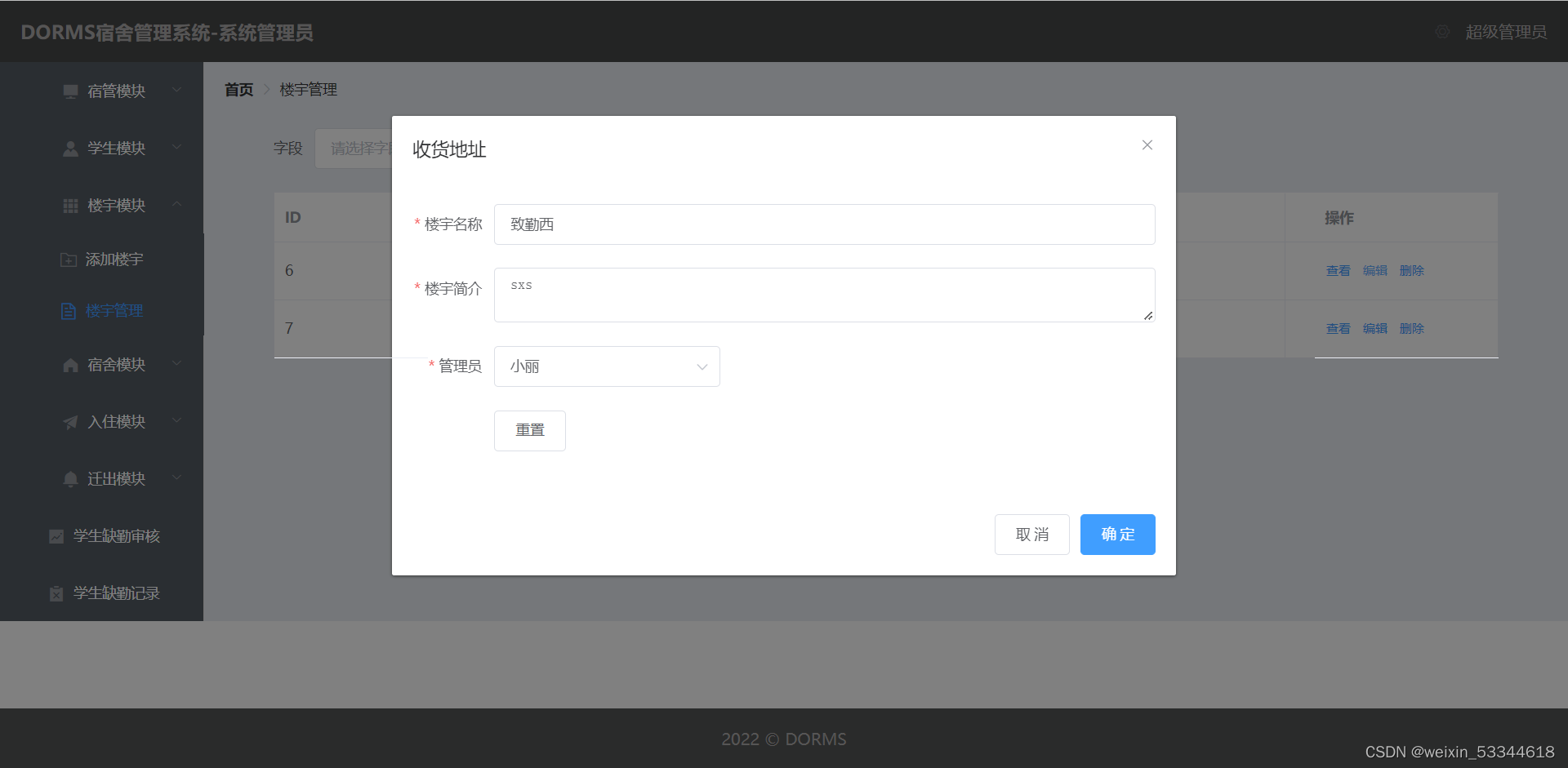
<template>
<div class="buildingList">
<div class="buildingList_header">
<el-form :inline="true" :model="formInline" :rules="rules" ref="ruleForm" class="demo-form-inline">
<el-form-item label="字段" prop="key">
<el-select v-model="formInline.key" placeholder="请选择字段">
<el-option label="楼宇名称" value="name"></el-option>
<el-option label="宿管名称" value="adminName"></el-option>
</el-select>
</el-form-item>
<el-form-item label="值">
<el-input v-model="formInline.value" placeholder="请输入关键字"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')">查询</el-button>
</el-form-item>
</el-form>
</div>
<div class="buildingList_table">
<el-table :data="tableData" border style="width: 100%">
<el-table-column fixed prop="id" label="ID" width="150">
</el-table-column>
<el-table-column prop="name" label="楼宇名称" width="180">
</el-table-column>
<el-table-column prop="introduction" label="楼宇简介" width="180">
</el-table-column>
<el-table-column prop="adminName" label="宿管名称" width="180">
</el-table-column>
<el-table-column prop="createTime" label="创建时间" width="300">
</el-table-column>
<el-table-column fixed="right" label="操作" width="180">
<template slot-scope="scope">
<el-button @click="handleClick(scope.row)" type="text" size="small">查看</el-button>
<!-- <el-button type="text" size="small">编辑</el-button> -->
<el-button type="text" size="small" @click="openUpdateD(scope.row)">编辑</el-button>
<el-dialog title="收货地址" :visible.sync="dialogFormVisible" :modal-append-to-body='false'>
<el-form :model="buildingForm" :rules="rules" ref="updateBuildingForm" label-width="80px"
class="demo-ruleForm">
<el-form-item label="楼宇名称" prop="name">
<el-input v-model="buildingForm.name"></el-input>
</el-form-item>
<el-form-item label="楼宇简介" prop="introduction">
<el-input type="textarea" v-model="buildingForm.introduction"></el-input>
</el-form-item>
<el-form-item label="管理员" prop="adminId">
<el-select v-model="buildingForm.adminId" placeholder="请选择管理员">
<el-option v-for="item in dormitoryAdminList" :key="item.id" :label="item.name" :value="item.id">
</el-option>
</el-select>
</el-form-item>
<el-form-item>
<el-button @click="resetForm('updateBuildingForm')">重置</el-button>
</el-form-item>
</el-form>
<!-- <div slot="footer" class="dialog-footer">
<el-button @click="dialogFormVisible = false">取 消</el-button>
<el-button type="primary" @click="updateSubmitForm('updateBuildingForm') > 确 定 <div >
</div> -->
<div slot="footer" class="dialog-footer">
<el-button @click="dialogFormVisible = false">取 消</el-button>
<el-button type="primary" @click="updateSubmitForm('updateBuildingForm')">确 定</el-button>
</div>
</el-dialog>
<el-button @click="DeleteClick(scope.row.id)" type="text" size="small" style="margin:0 0 0 10px">删除
</el-button>
</template>
</el-table-column>
</el-table>
<!-- 分页组件 -->
<div style="text-align: center;margin-top: 20px">
<el-pagination background layout="prev, pager, next" :total="total" :current-page="currentPage"
:page-sizes="selectPage" :page-size="sizePage" @current-change="currentPageChange">
</el-pagination>
</div>
</div>
</div>
</template>
<script>
export default {
name: "BuildingList",
data() {
return {
tempID: 0,
u: 'buildingList',
formInline: {
key: '',
value: '',
current: '', //当前页
size: 5, //每页显示的条数
},
tableData: [],
//当前页
currentPage: 1,
//每页显示的条数
sizePage: 5,
//总条数
total: 0,
selectPage: [5, 10, 15, 25, 30, 50, 100],
rules: {
key: [
{ required: true, message: '请选择字段', trigger: 'change' }
],
},
buildingForm: {
id: '',
name: '',
introduction: '',
adminId: '' //宿管ID
},
buildingObj: {
},
dormitoryAdminList: [], //所有的宿管
rules: {
name: [
{ required: true, message: '请输入楼宇名称', trigger: 'blur' }
],
introduction: [
{ required: true, message: '请输入楼宇简介', trigger: 'blur' }
],
adminId: [
{ required: true, message: '请选择宿管', trigger: 'change' }
]
},
dialogTableVisible: false,
dialogFormVisible: false
}
},
created() {
//初始化宿舍管理员数据
this.listPage();
this.getDormitoryAdminList();
},
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
// alert('submit!');
//每次重新搜素,让当前页重置为1
const _this = this;
_this.currentPage = 1;
_this.formInline.current = _this.currentPage;
// console.log(this.formInline)
//发起axios请求后端
axios.get("http://116.62.204.4:8001/dormitory/building/listPageLink", {
params: _this.formInline
}).then((resp) => {
console.log(resp.data);
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
} else {
console.log('error submit!!');
return false;
}
});
},
updateSubmitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
console.log(this.buildingForm)
//添加楼宇
const _this = this;
axios.post("http://116.62.204.4:8001/dormitory/building/update", _this.buildingForm)
.then((resp => {
console.log(resp.data);
if (resp.data.code === 0) {
//添加楼宇成功
//提示用户添加成功
_this.$message({
message: resp.data.message,
type: 'success'
});
//跳转到楼宇列表页面
// _this.$router.push('/buildingList')
this.$router.push(`/empty?u=${this.u}`);
}
if (resp.data.code === 1) {
//添加楼宇失败
_this.$message.error(resp.data.message);
//清空文本输入框
_this.buildingForm = {};
}
if (resp.data.code === -1) {
//表示该楼宇已经存在
//提示用户不要重复添加
_this.$message({
message: resp.data.message,
type: 'warning'
});
}
}));
} else {
console.log('error submit!!');
return false;
}
});
},
handleClick(row) {
console.log(row);
},
DeleteClick(id) {
// console.log(row);
const _this = this;
axios.get(`http://116.62.204.4:8001/dormitory/building/delete/${id}`, {
params: _this.formInline
}).then((resp) => {
console.log(resp.data);
// this.reload();
// _this.tableData = resp.data.data.pages;
// _this.total = resp.data.data.total;
console.log('传参前的u:' + this.u);
this.$router.push(`/empty?u=${this.u}`);
// _this.reload();
// this.$router.replace('/buildingList');
});
},
openUpdateD(row) {
this.dialogFormVisible = true;
this.buildingForm.name = row.name;
this.buildingForm.introduction = row.introduction;
this.buildingForm.adminId = row.adminId;
this.buildingForm.id = row.id;
},
//分页查询宿舍管理数据
listPage() {
//发起axios请求
const _this = this;
axios.get(`http://116.62.204.4:8001/dormitory/building/listPage/1/${_this.sizePage}`)
.then((resp) => {
console.log(resp.data)
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
},
//查询所有的宿管
getDormitoryAdminList() {
const _this = this;
axios.get("http://116.62.204.4:8001/dormitory/dormitory-admin/getDormitoryAdmin")
.then((resp) => {
console.log(resp.data.data);
_this.dormitoryAdminList = resp.data.data;
});
},
//点击页码切换每一页
currentPageChange(page) {
// console.log(page)
const _this = this;
//首先,先判断是模糊分页查询,还是普通的分页查询
if (_this.formInline.value == '') {
//如果模糊查询的值为空的话那就进行普通的分页查询
//将点击的每一页赋值给当前页
_this.currentPage = page;
//发起axios请求
axios.get(`http://116.62.204.4:8001/dormitory/building/listPage/${_this.currentPage}/${_this.sizePage}`)
.then((resp) => {
console.log(resp.data)
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
} else {
//模糊分页查询
//将点击的每一页页码赋值给当前页
_this.formInline.current = page;
//发起axios请求
axios.get("http://116.62.204.4:8001/dormitory/building/listPageLink", {
params: _this.formInline
}).then((resp) => {
console.log(resp.data);
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
}
}
}
}
</script>
<style scoped>
.buildingList {
margin: 30px auto 15px;
width: 1200px;
/*border: 1px solid #42b983;*/
}
</style>
2.宿舍模块
2.1宿舍添加
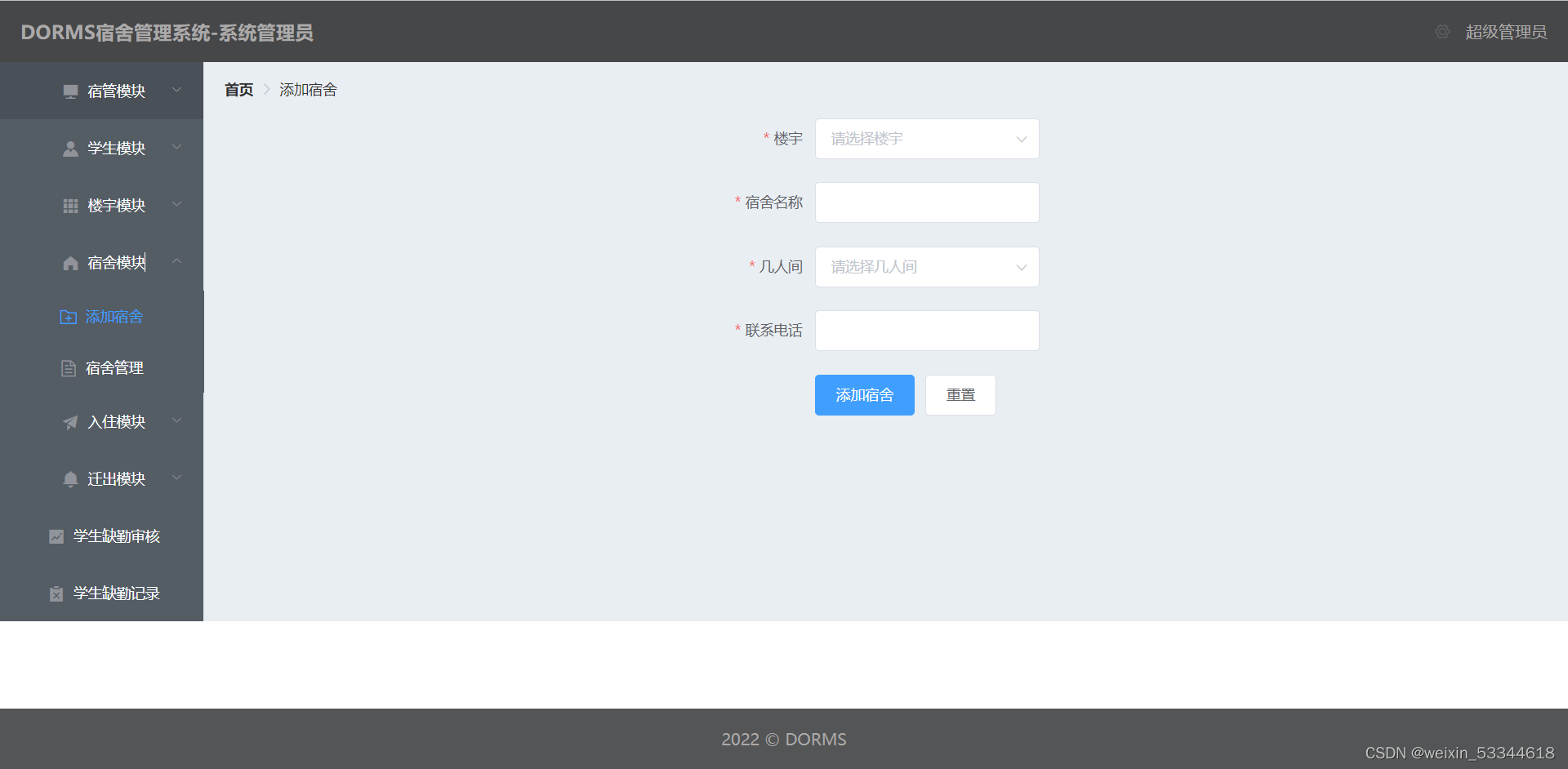
<template>
<div class="dormitoryAdd">
<el-form :model="dormitoryAdminForm" :rules="rules" ref="dormitoryAdminForm" label-width="80px" class="demo-ruleForm">
<el-form-item label="楼宇" prop="buildingId">
<el-select v-model="dormitoryAdminForm.buildingId" placeholder="请选择楼宇">
<el-option v-for="item in buildingList" :key="item.id" :label="item.name" :value="item.id"></el-option>
</el-select>
</el-form-item>
<el-form-item label="宿舍名称" prop="name">
<el-input v-model="dormitoryAdminForm.name"></el-input>
</el-form-item>
<el-form-item label="几人间" prop="type">
<el-select v-model="dormitoryAdminForm.type" placeholder="请选择几人间">
<el-option label="2人间" value="2"></el-option>
<el-option label="4人间" value="4"></el-option>
<el-option label="6人间" value="6"></el-option>
<el-option label="8人间" value="8"></el-option>
</el-select>
</el-form-item>
<el-form-item label="联系电话" prop="telephone">
<el-input v-model="dormitoryAdminForm.telephone"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('dormitoryAdminForm')">添加宿舍</el-button>
<el-button @click="resetForm('dormitoryAdminForm')">重置</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
export default {
name: "DormitoryAdd",
data() {
return {
dormitoryAdminForm: {
buildingId: '',
name: '',
telephone : '',
type : ''
},
buildingList : [], //所有的楼宇
rules: {
name: [
{required: true, message: '请输入宿舍名称', trigger: 'blur'}
],
type: [
{required: true, message: '请选择几人间', trigger: 'change'}
],
buildingId: [
{required: true, message: '请选择楼宇', trigger: 'change'}
],
telephone: [
{required: true, message: '请输入手机号', trigger: 'blur'}
]
}
}
},
created() {
//查询所有的楼宇
this.getBuildingList();
},
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
console.log(this.dormitoryAdminForm)
//添加宿舍
const _this = this;
axios.post("http://116.62.204.4:8001/dormitory/dormitory/add", _this.dormitoryAdminForm)
.then((resp => {
console.log(resp.data);
if (resp.data.code === 0) {
//添加宿舍成功
//提示用户添加成功
_this.$message({
message: resp.data.message,
type: 'success'
});
//跳转到宿舍列表页面
_this.$router.push('/dormitoryList')
}
if (resp.data.code === 1) {
//添加宿舍失败
_this.$message.error(resp.data.message);
//清空文本输入框
_this.dormitoryAdminForm = {};
}
if (resp.data.code === -1) {
//表示该宿舍已经存在
//提示用户不要重复添加
_this.$message({
message: resp.data.message,
type: 'warning'
});
}
}));
} else {
console.log('error submit!!');
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
},
//查询所有的楼宇
getBuildingList(){
const _this = this;
axios.get("http://116.62.204.4:8001/dormitory/building/getBuilding")
.then((resp) => {
console.log(resp.data.data);
_this.buildingList = resp.data.data;
});
}
}
}
</script>
<style scoped>
.dormitoryAdd{
margin: 20px auto;
/*border: 1px solid #42b983;*/
width: 300px;
}
</style>
2.2宿舍管理
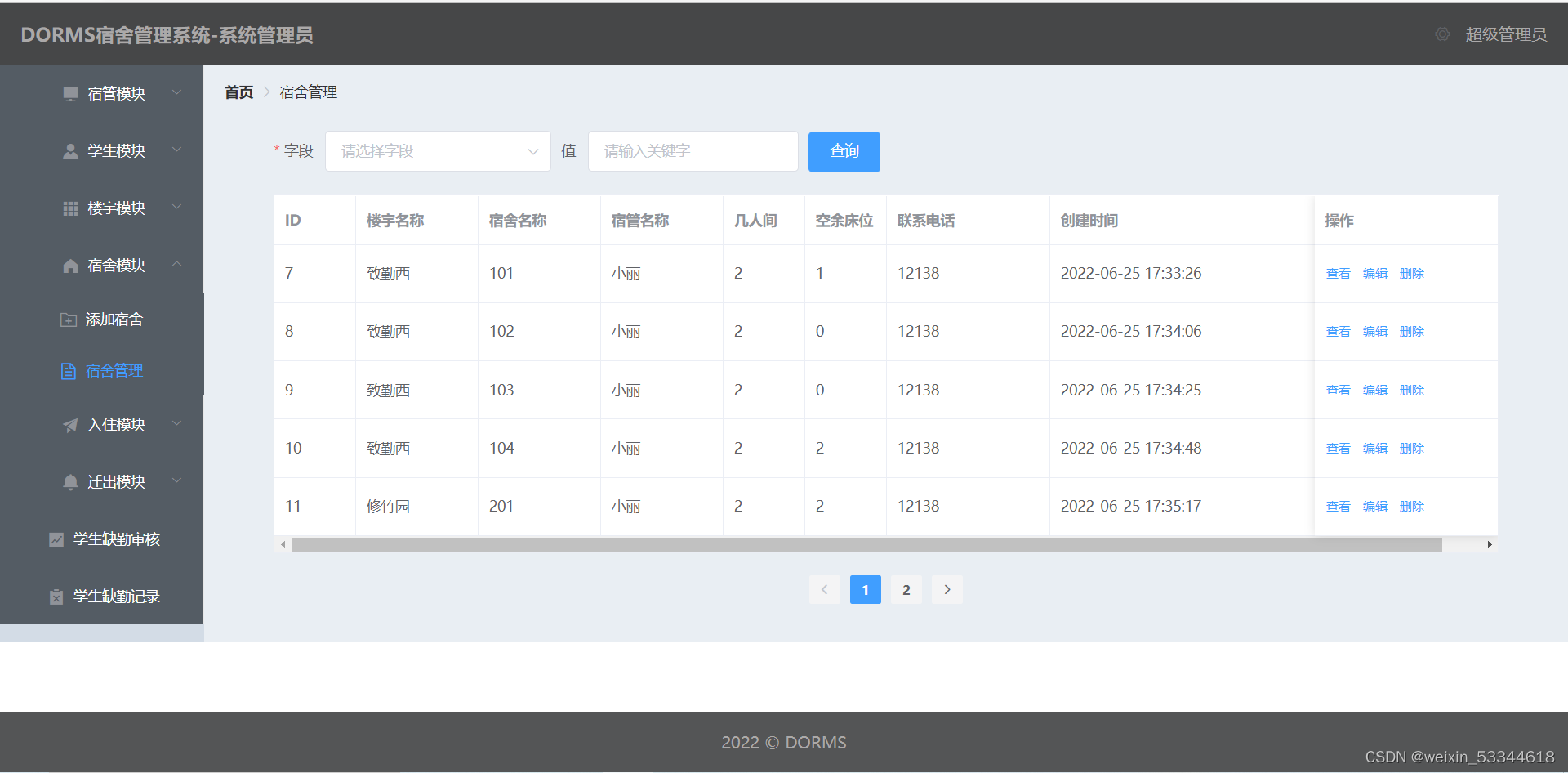
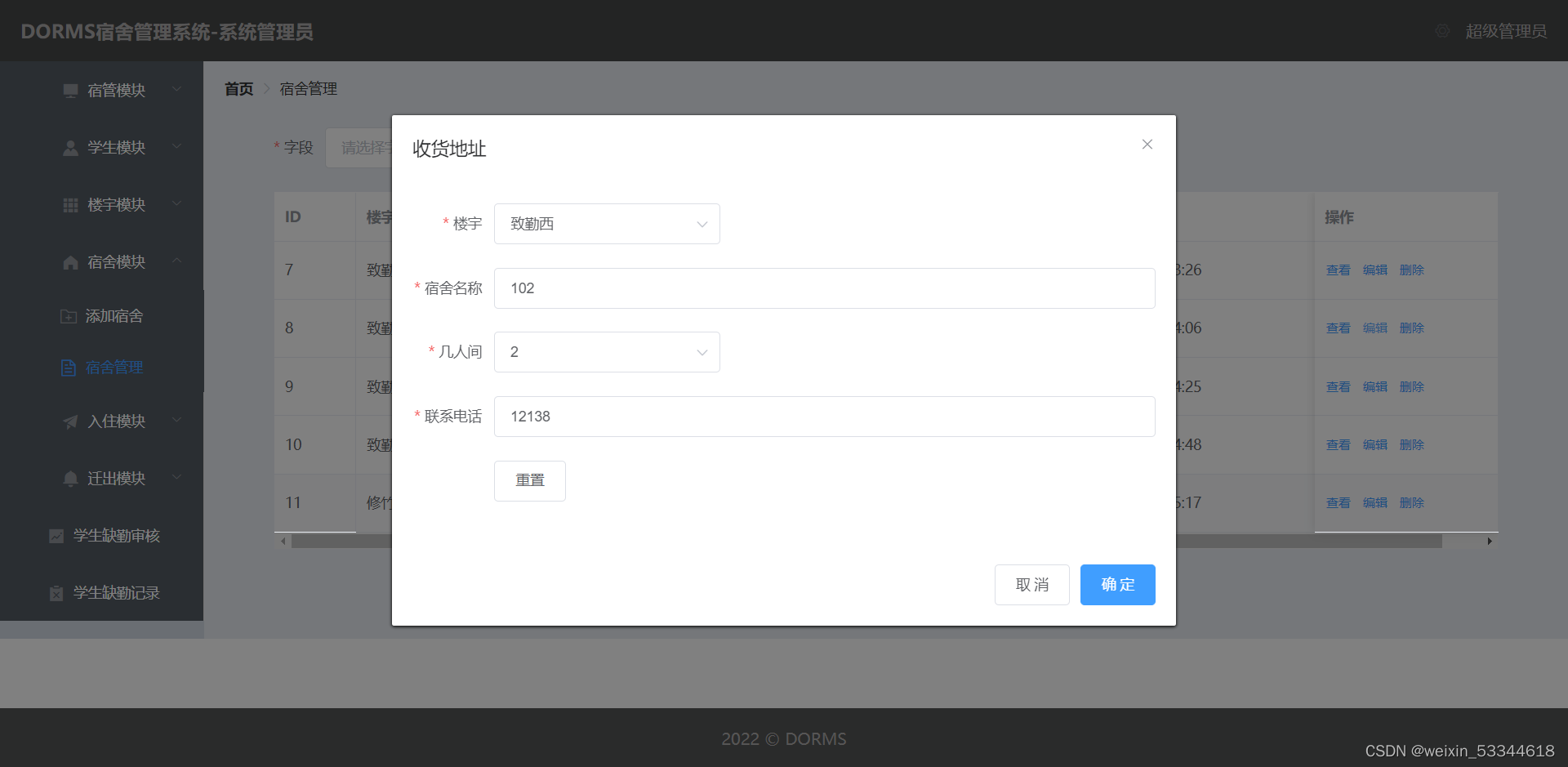
<template>
<div class="dormitoryList">
<div class="dormitoryList_header">
<el-form :inline="true" :model="formInline" :rules="rules" ref="ruleForm" class="demo-form-inline">
<el-form-item label="字段" prop="key">
<el-select v-model="formInline.key" placeholder="请选择字段">
<el-option label="楼宇名称" value="buildingName"></el-option>
<el-option label="宿舍名称" value="name"></el-option>
<el-option label="宿管名称" value="adminName"></el-option>
</el-select>
</el-form-item>
<el-form-item label="值">
<el-input v-model="formInline.value" placeholder="请输入关键字"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')">查询</el-button>
</el-form-item>
</el-form>
</div>
<div class="dormitoryList_table">
<el-table :data="tableData" border style="width: 100%">
<el-table-column fixed prop="id" label="ID" width="80">
</el-table-column>
<el-table-column prop="buildingName" label="楼宇名称" width="120">
</el-table-column>
<el-table-column prop="name" label="宿舍名称" width="120">
</el-table-column>
<el-table-column prop="adminName" label="宿管名称" width="120">
</el-table-column>
<el-table-column prop="type" label="几人间" width="80">
</el-table-column>
<el-table-column prop="spareBeds" label="空余床位" width="80">
</el-table-column>
<el-table-column prop="telephone" label="联系电话" width="160">
</el-table-column>
<el-table-column prop="createTime" label="创建时间" width="300">
</el-table-column>
<el-table-column fixed="right" label="操作" width="180">
<template slot-scope="scope">
<el-button @click="handleClick(scope.row)" type="text" size="small">查看</el-button>
<!-- <el-button type="text" size="small">编辑</el-button> -->
<el-button type="text" size="small" @click="openUpdateD(scope.row)">编辑</el-button>
<el-dialog title="收货地址" :visible.sync="dialogFormVisible" :modal-append-to-body='false'>
<el-form :model="dormitoryAdminForm" :rules="rulesUpdate" ref="updateDormitoryForm" label-width="80px"
class="demo-ruleForm">
<el-form-item label="楼宇" prop="buildingId">
<el-select v-model="dormitoryAdminForm.buildingId" placeholder="请选择楼宇">
<el-option v-for="item in buildingList" :key="item.id" :label="item.name" :value="item.id">
</el-option>
</el-select>
</el-form-item>
<el-form-item label="宿舍名称" prop="name">
<el-input v-model="dormitoryAdminForm.name"></el-input>
</el-form-item>
<el-form-item label="几人间" prop="type">
<el-select v-model="dormitoryAdminForm.type" placeholder="请选择几人间">
<el-option label="2人间" value="2"></el-option>
<el-option label="4人间" value="4"></el-option>
<el-option label="6人间" value="6"></el-option>
<el-option label="8人间" value="8"></el-option>
</el-select>
</el-form-item>
<el-form-item label="联系电话" prop="telephone">
<el-input v-model="dormitoryAdminForm.telephone"></el-input>
</el-form-item>
<el-form-item>
<!-- <el-button type="primary" @click="updateSubmitForm('updateBuildingForm')">修改</el-button> -->
<!-- <el-button type="primary" @click="updateSubmitForm('updateDormitoryForm')">修改</el-button> -->
<el-button @click="resetForm('dormitoryAdminForm')">重置</el-button>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="dialogFormVisible = false">取 消</el-button>
<el-button type="primary" @click="updateSubmitForm('updateDormitoryForm')">确 定</el-button>
</div>
</el-dialog>
<el-button @click="DeleteClick(scope.row.id)" type="text" size="small" style="margin:0 0 0 10px">删除
</el-button>
</template>
</el-table-column>
</el-table>
<!-- 分页组件 -->
<div style="text-align: center;margin-top: 20px">
<el-pagination background layout="prev, pager, next" :total="total" :current-page="currentPage"
:page-sizes="selectPage" :page-size="sizePage" @current-change="currentPageChange">
</el-pagination>
</div>
</div>
</div>
</template>
<script>
export default {
name: "DormitoryList",
data() {
return {
u: 'dormitoryList',
formInline: {
key: '',
value: '',
current: '', //当前页
size: 5, //每页显示的条数
},
dormitoryAdminForm: {
id: 0,
buildingId: '',
name: '',
telephone: '',
type: ''
},
buildingList: [], //所有的楼宇
rulesUpdate: {
name: [
{ required: true, message: '请输入宿舍名称', trigger: 'blur' }
],
type: [
{ required: true, message: '请选择几人间', trigger: 'change' }
],
buildingId: [
{ required: true, message: '请选择楼宇', trigger: 'change' }
],
telephone: [
{ required: true, message: '请输入手机号', trigger: 'blur' }
]
},
tableData: [],
//当前页
currentPage: 1,
//每页显示的条数
sizePage: 5,
//总条数
total: 0,
selectPage: [5, 10, 15, 25, 30, 50, 100],
rules: {
key: [
{ required: true, message: '请选择字段', trigger: 'change' }
],
},
dialogTableVisible: false,
dialogFormVisible: false
}
},
created() {
//初始化宿舍管理员数据
this.listPage();
this.getBuildingList();
},
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
// alert('submit!');
//每次重新搜素,让当前页重置为1
const _this = this;
_this.currentPage = 1;
_this.formInline.current = _this.currentPage;
// console.log(this.formInline)
//发起axios请求后端
axios.get("http://116.62.204.4:8001/dormitory/dormitory/listPageLink", {
params: _this.formInline
}).then((resp) => {
console.log(resp.data);
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
} else {
console.log('error submit!!');
return false;
}
});
},
DeleteClick(id) {
// console.log(row);
const _this = this;
axios.get(`http://116.62.204.4:8001/dormitory/dormitory/delete/${id}`, {
params: _this.formInline
}).then((resp) => {
console.log(resp.data);
// this.reload();
// _this.tableData = resp.data.data.pages;
// _this.total = resp.data.data.total;
console.log('传参前的u:' + this.u);
this.$router.push(`/empty?u=${this.u}`);
// _this.reload();
// this.$router.replace('/buildingList');
});
},
handleClick(row) {
console.log(row);
},
openUpdateD(row) {
this.dialogFormVisible = true;
this.dormitoryAdminForm.buildingId = row.buildingId;
this.dormitoryAdminForm.name = row.name;
this.dormitoryAdminForm.telephone = row.telephone;
this.dormitoryAdminForm.type = row.type;
this.dormitoryAdminForm.id = row.id;
},
updateSubmitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
console.log(this.dormitoryAdminForm)
//添加宿舍
const _this = this;
axios.post("http://116.62.204.4:8001/dormitory/dormitory/update", _this.dormitoryAdminForm)
.then((resp => {
console.log(resp.data);
if (resp.data.code === 0) {
//添加宿舍成功
//提示用户添加成功
_this.$message({
message: resp.data.message,
type: 'success'
});
//跳转到宿舍列表页面
// _this.$router.push('/dormitoryList')
this.$router.push(`/empty?u=${this.u}`);
}
if (resp.data.code === 1) {
//添加宿舍失败
_this.$message.error(resp.data.message);
//清空文本输入框
_this.dormitoryAdminForm = {};
}
if (resp.data.code === -1) {
//表示该宿舍已经存在
//提示用户不要重复添加
_this.$message({
message: resp.data.message,
type: 'warning'
});
}
}));
} else {
console.log('error submit!!');
return false;
}
});
},
getBuildingList() {
const _this = this;
axios.get("http://116.62.204.4:8001/dormitory/building/getBuilding")
.then((resp) => {
console.log(resp.data.data);
_this.buildingList = resp.data.data;
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
},
//分页查询宿舍管理数据
listPage() {
//发起axios请求
const _this = this;
axios.get(`http://116.62.204.4:8001/dormitory/dormitory/listPage/1/${_this.sizePage}`)
.then((resp) => {
console.log(resp.data)
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
},
//点击页码切换每一页
currentPageChange(page) {
// console.log(page)
const _this = this;
//首先,先判断是模糊分页查询,还是普通的分页查询
if (_this.formInline.value == '') {
//如果模糊查询的值为空的话那就进行普通的分页查询
//将点击的每一页赋值给当前页
_this.currentPage = page;
//发起axios请求
axios.get(`http://116.62.204.4:8001/dormitory/dormitory/listPage/${_this.currentPage}/${_this.sizePage}`)
.then((resp) => {
console.log(resp.data)
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
} else {
//模糊分页查询
//将点击的每一页页码赋值给当前页
_this.formInline.current = page;
//发起axios请求
axios.get("http://116.62.204.4:8001/dormitory/dormitory/listPageLink", {
params: _this.formInline
}).then((resp) => {
console.log(resp.data);
_this.tableData = resp.data.data.pages;
_this.total = resp.data.data.total;
});
}
}
}
}
</script>
<style scoped>
.dormitoryList {
margin: 30px auto 15px;
width: 1200px;
/*border: 1px solid #42b983;*/
}
</style>