Spring+Spring MVC+MyBatis
环境:
- java EE Version: 2020-06 (4.16.0)
- spring-framework-5.0.1
- mybatis-3.5.6
- 8.0.21 MySQL Community Server - GPL
- navicat
- chrome
导入需要的jar包,最好都放在WEB-INF/lib下边
- spring下载: https://repo.spring.io/webapp/#/artifacts/browse/tree/General/libs-release-local/org/springframework/spring/5.0.1.RELEASE
- mybatis: https://github.com/mybatis/mybatis-3/releases
- mybatis-spring-1.3.1.jar
- mysql-connector-java-8.0.16.jar
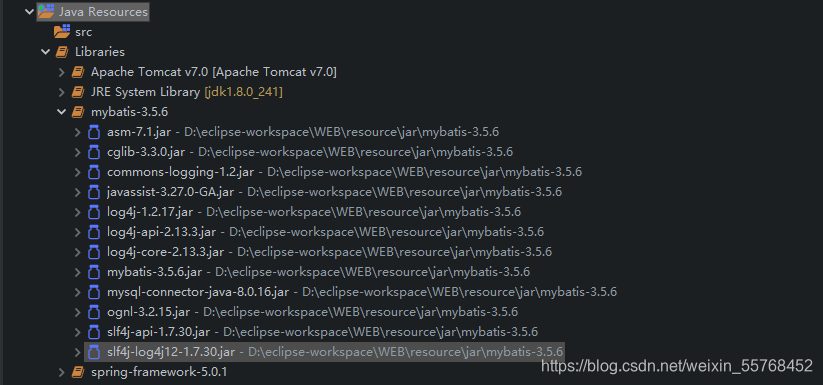
配置web.xml文件
- 注册spring配置文件的位置
- 注册ServletContext监听器
- 注册字符集过滤器
- 配置Spring MVC 核心控制器
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>ssm1</display-name>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml</param-value>
</context-param>
<listener>
<listener-class>
org.springframework.web.context.ContextLoaderListener
</listener-class>
</listener>
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
<context-param>
<param-name>spring.profiles.active</param-name>
<param-value>dev</param-value>
</context-param>
<context-param>
<param-name>spring.profiles.default</param-name>
<param-value>dev</param-value>
</context-param>
<context-param>
<param-name>spring.liveBeansView.mbeanDomain</param-name>
<param-value>dev</param-value>
</context-param>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
创建 src/applicationContext.xml 注册数据源 DataSource
- 配置数据源 c3p0 数据源
- 注册事务
-
定义事务管理器
-
编写通知
-
编写AOP 让Spring自动切入事务到目标切面
-
注册MyBatis的配置文件
-
配置SqlSessionFactory
-
-
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="dataSource"
class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass">
<value>com.mysql.cj.jdbc.Driver</value>
</property>
<property name="jdbcUrl">
<value>jdbc:mysql://127.0.0.1:3306/mybatis?serverTimezone=UTC&useUnicode=true&characterEncoding=utf8</value>
</property>
<property name="user">
<value>root</value>
</property>
<property name="password">
<value>123456</value>
</property>
<property name="acquireIncrement" value="5"></property>
<property name="initialPoolSize" value="20"></property>
<property name="maxPoolSize" value="25"></property>
<property name="minPoolSize" value="5"></property>
</bean>
<bean id="txManager"
class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource" />
</bean>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="*" propagation="REQUIRED"
isolation="DEFAULT" read-only="false" />
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut id="txPointcut"
expression="execution(* com.gen.service.*.*(..))" />
<aop:advisor advice-ref="txAdvice"
pointcut-ref="txPointcut" />
</aop:config>
<bean id="sqlSessionFactory"
class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="configLocation"
value="classpath:mybatis-config.xml"></property>
</bean>
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="sqlSessionFactory" ref="sqlSessionFactory" />
<property name="basePackage" value="com.gen.dao" />
</bean>
<context:component-scan
base-package="com.gen.service" />
</beans>
创建 src/mybatis-config.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<typeAlias alias="Student" type="com.gen.entity.Student"/>
</typeAliases>
<mappers>
<mapper resource="com/gen/dao/StudentMapper.xml"/>
</mappers>
</configuration>
创建 src/springmvc.xml
- 配置包扫描器 扫描@Controller
- 加载注解驱动
- 配置视图解释器 逻辑视图前缀+逻辑视图后缀
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<context:component-scan base-package="com.gen.controller"/>
<mvc:annotation-driven/>
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
</beans>
创建数据表
CREATE TABLE `users` (
`id` int NOT NULL AUTO_INCREMENT,
`username` varchar(32) NOT NULL COMMENT '用户名称',
`sex` char(1) DEFAULT NULL COMMENT '性别',
`address` varchar(256) DEFAULT NULL COMMENT '地址',
`idnumber` varchar(20) DEFAULT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `users_id_index` (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=20201014 DEFAULT CHARSET=utf8
后续开发
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-uUpqRS86-1616756545636)(C:\Users\ShiGen\Desktop\DestopImg\MAC14.jpg)]
创建包 com.gen.entity
和实体类 Student
省略setter getter constructor方法
package com.gen.entity;
public class Student {
private Integer id;
private String username;
private Character sex;
private String address;
private String idnumber;
创建包 com.gen.dao
创建接口 IStudentDao
和对应的 StudentMapper.xml
package com.gen.dao;
import java.util.List;
import com.gen.entity.Student;
public interface IStudentDao {
public int add(Student student);
public int delete(Integer id);
public int update(Student student);
public Student selectOne(Integer id);
public List<Student> selectAll();
}
<?xml version="1.0" encoding="utf-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.gen.dao.IStudentDao">
<resultMap type="com.gen.entity.Student"
id="studentResultMap">
<id column="id" property="id" />
<result column="username" property="username" />
<result column="sex" property="sex" />
<result column="address" property="address" />
<result column="idnumber" property="idnumber" />
</resultMap>
<select id="selectAll" resultMap="studentResultMap"
resultType="com.gen.entity.Student">
SELECT * FROM users
</select>
<select id="selectOne" resultMap="studentResultMap"
parameterType="int">
SELECT id,username,sex,address,idnumber
FROM users
where
id=#{id}
</select>
<insert id="add" parameterType="Student">INSERT INTO
users(id, username,
sex, address, idnumber)
VALUES(#{id}, #{username}, #{sex},
#{address},#{idnumber})
</insert>
<delete id="delete" parameterType="Integer">
delete from users where
id=#{id}
</delete>
<update id="update" parameterType="Student">
UPDATE users SET
username=#{username},sex=#{sex},address=#{address},idnumber=#{idnumber}
WHERE id= #{id}
</update>
</mapper>
创建包 com.gen.service
创建接口 IStudentService
以及实现类 StudentServiceImpl
package com.gen.service;
import java.util.List;
import com.gen.entity.Student;
public interface IStudentService {
public int add(Student student);
public int delete(Integer id);
public int update(Student student);
public Student selectOne(Integer id);
public List<Student> selectAll();
public void testTransaction();
}
package com.gen.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.gen.dao.IStudentDao;
import com.gen.entity.Student;
@Service
public class StudentServiceImpl implements IStudentService{
@Autowired
private IStudentDao studentDao;
public IStudentDao getStudentDao() {
return studentDao;
}
public void setStudentDao(IStudentDao studentDao) {
this.studentDao = studentDao;
}
@Override
public int add(Student student) {
return studentDao.add(student);
}
@Override
public int delete(Integer id) {
return studentDao.delete(id);
}
@Override
public int update(Student student) {
return studentDao.update(student);
}
@Override
public Student selectOne(Integer id) {
return studentDao.selectOne(id);
}
@Override
public List<Student> selectAll() {
return studentDao.selectAll();
}
@Override
public void testTransaction() {
delete(4);
Integer.parseInt("a");
delete(3);
}
}
创建包 com.gen.controller
创建控制器类
package com.gen.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import com.gen.entity.Student;
import com.gen.service.IStudentService;
@Controller
public class StudentController {
@Autowired
IStudentService studentService;
@RequestMapping("/addPage.do")
public String addPage() {
return "add";
}
@RequestMapping("/add.do")
public ModelAndView add(Student student) {
ModelAndView modelAndView = new ModelAndView();
studentService.add(student);
modelAndView.setViewName("redirect:selectAll.do");
return modelAndView;
}
@RequestMapping("/delete.do")
public ModelAndView delete(Integer id) {
ModelAndView modelAndView = new ModelAndView();
studentService.delete(id);
modelAndView.setViewName("redirect:selectAll.do");
return modelAndView;
}
@RequestMapping("/update.do")
public ModelAndView update(Student student) {
ModelAndView modelAndView = new ModelAndView();
studentService.update(student);
modelAndView.setViewName("redirect:selectAll.do");
return modelAndView;
}
@RequestMapping("/selectAll.do")
public ModelAndView selectAll() {
ModelAndView modelAndView = new ModelAndView();
List<Student> all = studentService.selectAll();
modelAndView.addObject("all", all);
modelAndView.setViewName("list");
return modelAndView;
}
@RequestMapping("/selectOne.do")
public ModelAndView selectOne(Integer id) {
ModelAndView modelAndView = new ModelAndView();
Student student = studentService.selectOne(id);
modelAndView.addObject("student", student);
modelAndView.setViewName("detail");
return modelAndView;
}
@RequestMapping("/toUpdate.do")
public ModelAndView toUpdate(Integer id) {
ModelAndView modelAndView = new ModelAndView();
Student student = studentService.selectOne(id);
modelAndView.addObject("student", student);
modelAndView.setViewName("update");
return modelAndView;
}
}
在WebContent/WEB-INF 下创建文件夹jsp 创建相应的页面
- add.jsp
- detail.jsp
- lit.jsp
- update.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>add.jsp</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
<style type="text/css">
input {
width: 100%;
}
</style>
</head>
<body>
<h2>add.jsp</h2>
<form action="add.do" method="post">
<table style="width: 80%;">
<tr>
<td>id:</td>
<td><input type="text" name="id" id="id"></td>
<td></td>
</tr>
<tr>
<td>username:</td>
<td><input type="text" name="username" id="username">
<td></td>
</tr>
<tr>
<td>sex:</td>
<td><input type="text" name="sex" id="sex"></td>
<td></td>
</tr>
<tr>
<td>address:</td>
<td><input type="text" name="address" id="address"></td>
<td></td>
</tr>
<tr>
<td>idnumber:</td>
<td><input type="text" name="idnumber" id="idnumber"></td>
<td></td>
<tr>
<tr>
<td colspan="3">
<input type="submit" value="提交" style="width: 100px;background-color: #3EDE83;">
<input type="reset" value="重置" style="width: 100px;background-color: #F0283D;">
</td>
</tr>
</table>
</form>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>detail.jsp</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
</head>
<body>
<h2>detail.jsp</h2>
<table>
<tr>
<td>id:</td>
<td>${student.id }</td>
</tr>
<tr>
<td>username:</td>
<td>${student.username }</td>
</tr>
<tr>
<td>sex:</td>
<td>${student.sex }</td>
</tr>
<tr>
<td>address:</td>
<td>${student.address }</td>
</tr>
<tr>
<td>idnumber:</td>
<td>${student.idnumber }</td>
</tr>
<tr>
<td></td>
<td><a href="selectAll.do">返回</a></td>
</tr>
</table>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>list.jsp</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
</head>
<body>
<h2>list.jsp</h2>
<table>
<tr>
<th>id:</th>
<th>username:</th>
<th>sex:</th>
<th>address:</th>
<th>idnumber:</th>
<th colspan="3">poeration:</th>
</tr>
<c:forEach items="${all}" var="stu">
<tr>
<td>${stu.id}</td>
<td>${stu.username}</td>
<td>${stu.sex}</td>
<td>${stu.address}</td>
<td>${stu.idnumber }</td>
<td><a href="selectOne.do?id=${stu.id}">详细信息 </a></td>
<td><a href="toUpdate.do?id=${stu.id}">修改 </a></td>
<td><a class="delete" href="delete.do?id=${stu.id}"
οnclick="return confirm('你确认要删除该学生信息吗?')">删除 </a></td>
</tr>
</c:forEach>
</table>
<a href="addPage.do">添加用户</a>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>update.jsp</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
<style type="text/css">
input{width: 100%}
</style>
</head>
<body>
<h2>update.jsp</h2>
<form action="update.do" method="post">
<table style="width: 80%;">
<tr>
<td>id:</td>
<td><input type="text" name="id" value="${student.id}"
readonly="readonly" /></td>
<td></td>
</tr>
<tr>
<td>username:</td>
<td><input type="text" name="username"
value="${student.username}" /></td>
<td></td>
</tr>
<tr>
<td>sex:</td>
<td><input type="text" name="sex" value="${student.sex}" /></td>
<td></td>
</tr>
<tr>
<td>address:</td>
<td><input type="text" name="address"
value="${student.address}" /></td>
<td></td>
</tr>
<tr>
<td>idnumber:</td>
<td><input type="text" name="idnumber"
value="${student.idnumber }"></td>
<td></td>
</tr>
<tr>
<td><input type="submit" value="修改" style="background-color: #3EDE83;"/></td>
<td></td>
<td></td>
</tr>
</table>
</form>
</body>
</html>
其它的样式表和生成日志的配置文件
- /SSM/WebContent/css/style.css
- /SSM/src/jdbc.properties
table{width: 100%;margin: 0 auto; border-collapse: collapse;border: 2px solid black;}
tr,th,td{border: 1px solid orange;}
a{display: inline-block;background-color: #3EDE83;border-radius: 5%;padding: 4px;text-decoration: none;}
a.delete{background-color:#F0283D;}
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://127.0.0.1:3306/mybatis?serverTimezone=UTC&useUnicode=true&characterEncoding=utf8
jdbc.username=root
jdbc.password=123456
项目架构
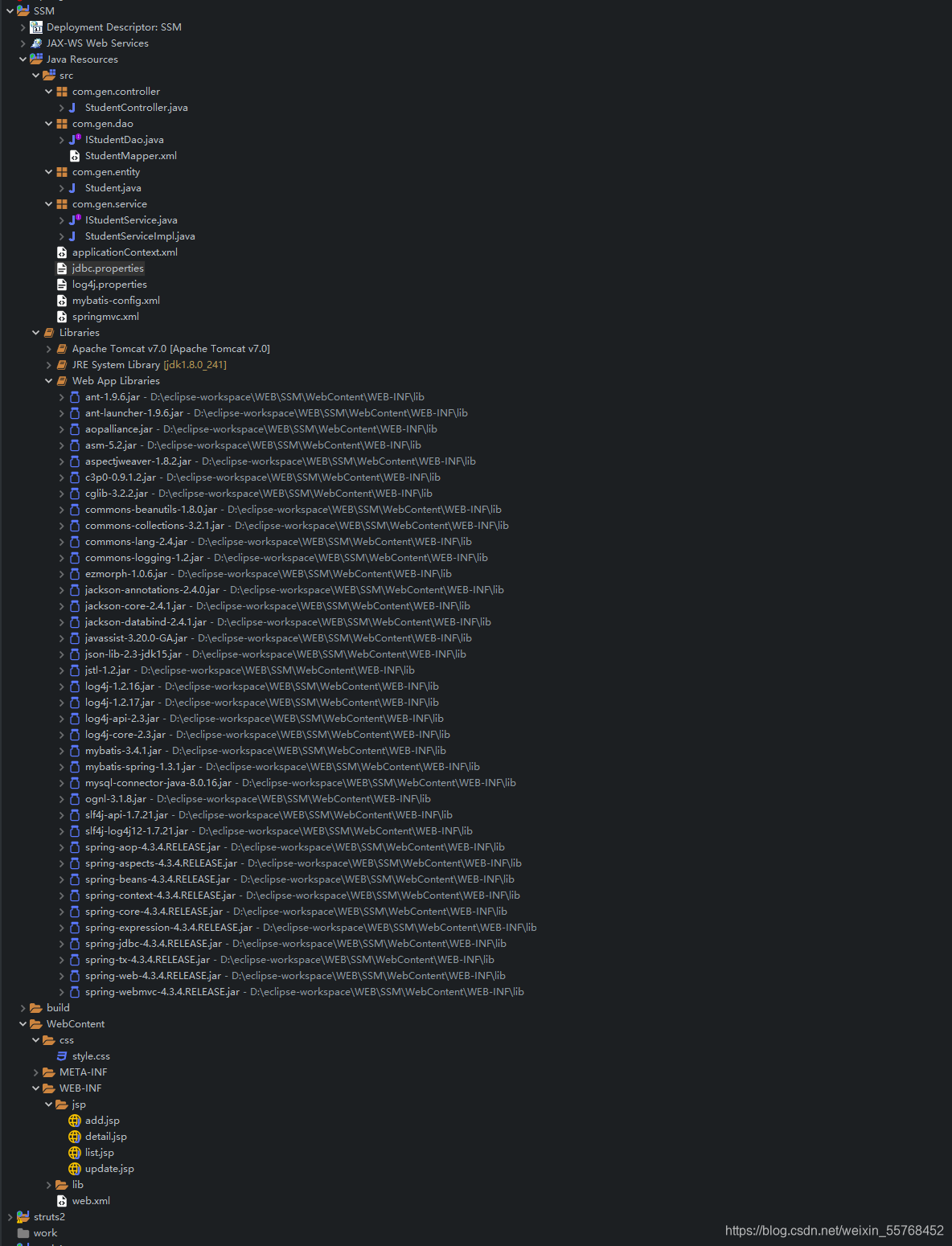
最终的效果
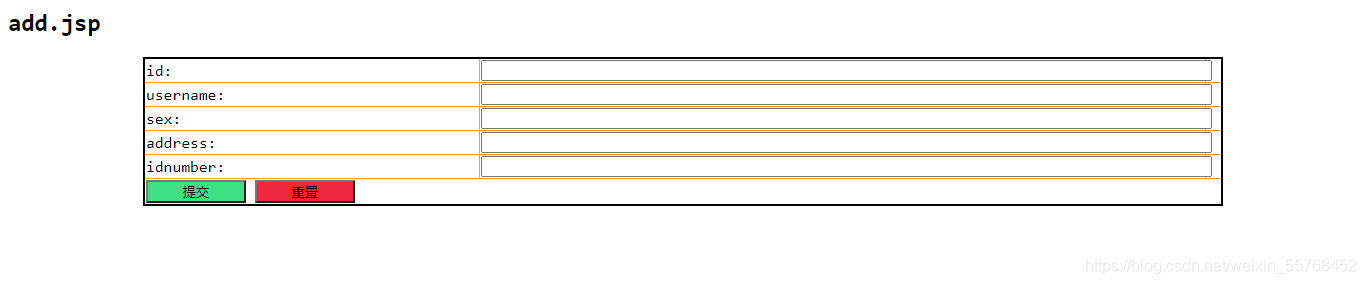
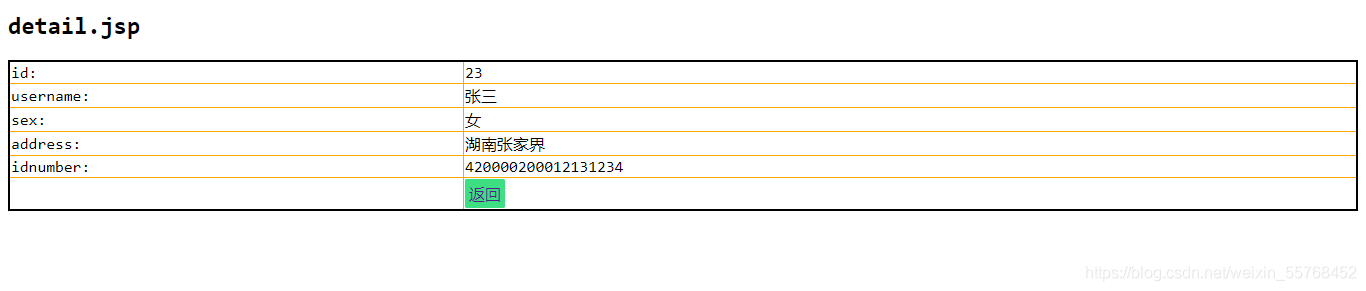
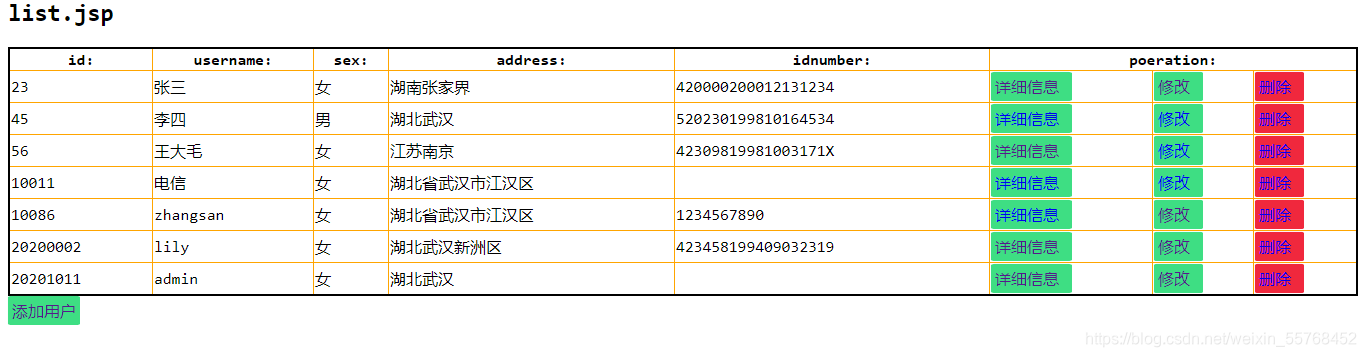
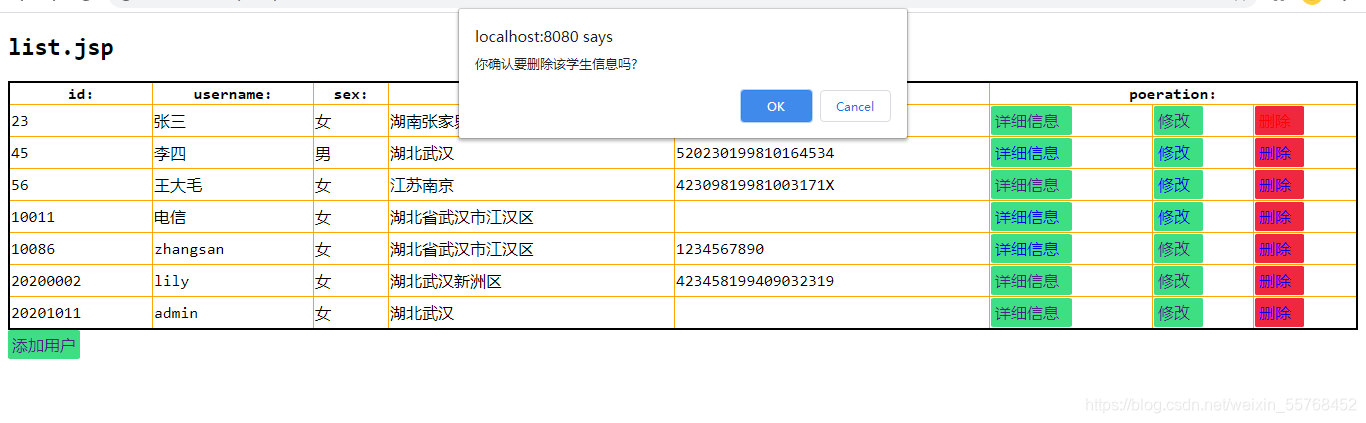

总结
- spring的反转和切片操作略显生疏
- 配置文件
/SSM/src/jdbc.properties
的读取存在问题
- UI设计
- 前端和后端数据的验证
- 增加业务
- 实现拦截器来控制权限
Plus版实现完整的功能
需要的技术:JavaScript jQuery以及各种插件以及以上SSM技术,剩下的后期再加上
实现的完整效果:完整的可实现的教务系统
数据库:mybatis
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 主键 |
username | varchar(32) | 否 | 用户名,支持英文的 |
sex | char(1) | 否 | 一个字符 男/女 |
address | varchar(256) | | 住址 |
idnumber | varchar(18) | 否 | 身份证号(中国) |
字段 | 类型 | 是否为空 | 备注 |
---|
courseid | varchar(30) | 否 | 课程号,主键 |
coursename | varchar(60) | 否 | 课程名 |
credit | float | 否 | 学分 |
departid | int | 否 | 院系号 |
teacher | int | 否 | 教师号 |
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 序列号自增 |
uid | int | 否 | 用户ID |
courseid | varchar(30) | 否 | 课程ID |
字段 | 类型 | 是否为空 | 备注 |
---|
cid | varchar(20) | 否 | 班级号 |
cname | varchar(40) | 否 | 班级名 |
departid | int | 否 | 院系号 |
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 序列号 自增 |
uid | int | 否 | 用户ID |
cid | varchar(20) | 否 | 班级号 |
字段 | 类型 | 是否为空 | 备注 |
---|
rid | int | 否 | 角色ID |
describ | varchar(20) | 否 | 描述(中文) |
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 用户ID |
rid | int | 否 | 角色ID |
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 用户ID,主键 |
password | varchar(60) | 否 | 加盐之后的密码 |
salt | varchar(60) | 否 | 盐 |
字段 | 类型 | 是否为空 | 备注 |
---|
departid | int | 否 | 院系ID |
name | varchar(32) | 否 | 院系名字 |
deanid | int | | 院长ID |
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 用户ID 主键 |
operator | int | | 注册的操作员 |
registrationtime | varchar(20) | | 注册时间 |
logintime | varchar(20) | | 登录时间 |
ip | varchar(39) | | 登录地址 |
需要实现的业务逻辑
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 用户ID,主键 |
password | varchar(60) | 否 | 加盐之后的密码 |
salt | varchar(60) | 否 | 盐 |
字段 | 类型 | 是否为空 | 备注 |
---|
departid | int | 否 | 院系ID |
name | varchar(32) | 否 | 院系名字 |
deanid | int | | 院长ID |
字段 | 类型 | 是否为空 | 备注 |
---|
id | int | 否 | 用户ID 主键 |
operator | int | | 注册的操作员 |
registrationtime | varchar(20) | | 注册时间 |
logintime | varchar(20) | | 登录时间 |
ip | varchar(39) | | 登录地址 |
##后期待开发