/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/** AbsoluteLayout is a LayoutManager that works as a replacement for "null" layout to
* allow placement of components in absolute positions.
*
* @see AbsoluteConstraints
* @version 1.01, Aug 19, 1998
*/
public class AbsoluteLayout implements LayoutManager2, java.io.Serializable {
/** generated Serialized Version UID */
static final long serialVersionUID = -1919857869177070440L;
/** Adds the specified component with the specified name to
* the layout.
* @param name the component name
* @param comp the component to be added
*/
public void addLayoutComponent(String name, Component comp) {
throw new IllegalArgumentException();
}
/** Removes the specified component from the layout.
* @param comp the component to be removed
*/
public void removeLayoutComponent(Component comp) {
constraints.remove(comp);
}
/** Calculates the preferred dimension for the specified
* panel given the components in the specified parent container.
* @param parent the component to be laid out
*
* @see #minimumLayoutSize
*/
public Dimension preferredLayoutSize(Container parent) {
int maxWidth = 0;
int maxHeight = 0;
for (java.util.Enumeration e = constraints.keys(); e.hasMoreElements();) {
Component comp = (Component)e.nextElement();
AbsoluteConstraints ac = (AbsoluteConstraints)constraints.get(comp);
Dimension size = comp.getPreferredSize();
int width = ac.getWidth ();
if (width == -1) width = size.width;
int height = ac.getHeight ();
if (height == -1) height = size.height;
if (ac.x + width > maxWidth)
maxWidth = ac.x + width;
if (ac.y + height > maxHeight)
maxHeight = ac.y + height;
}
return new Dimension (maxWidth, maxHeight);
}
/** Calculates the minimum dimension for the specified
* panel given the components in the specified parent container.
* @param parent the component to be laid out
* @see #preferredLayoutSize
*/
public Dimension minimumLayoutSize(Container parent) {
int maxWidth = 0;
int maxHeight = 0;
for (java.util.Enumeration e = constraints.keys(); e.hasMoreElements();) {
Component comp = (Component)e.nextElement();
AbsoluteConstraints ac = (AbsoluteConstraints)constraints.get(comp);
Dimension size = comp.getMinimumSize();
int width = ac.getWidth ();
if (width == -1) width = size.width;
int height = ac.getHeight ();
if (height == -1) height = size.height;
if (ac.x + width > maxWidth)
maxWidth = ac.x + width;
if (ac.y + height > maxHeight)
maxHeight = ac.y + height;
}
return new Dimension (maxWidth, maxHeight);
}
/** Lays out the container in the specified panel.
* @param parent the component which needs to be laid out
*/
public void layoutContainer(Container parent) {
for (java.util.Enumeration e = constraints.keys(); e.hasMoreElements();) {
Component comp = (Component)e.nextElement();
AbsoluteConstraints ac = (AbsoluteConstraints)constraints.get(comp);
Dimension size = comp.getPreferredSize();
int width = ac.getWidth ();
if (width == -1) width = size.width;
int height = ac.getHeight ();
if (height == -1) height = size.height;
comp.setBounds(ac.x, ac.y, width, height);
}
}
/** Adds the specified component to the layout, using the specified
* constraint object.
* @param comp the component to be added
* @param constr where/how the component is added to the layout.
*/
public void addLayoutComponent(Component comp, Object constr) {
if (!(constr instanceof AbsoluteConstraints))
throw new IllegalArgumentException();
constraints.put(comp, constr);
}
/** Returns the maximum size of this component.
* @see Component#getMinimumSize()
* @see Component#getPreferredSize()
* @see LayoutManager
*/
public Dimension maximumLayoutSize(Container target) {
return new Dimension(Integer.MAX_VALUE, Integer.MAX_VALUE);
}
/** Returns the alignment along the x axis. This specifies how
* the component would like to be aligned relative to other
* components. The value should be a number between 0 and 1
* where 0 represents alignment along the origin, 1 is aligned
* the furthest away from the origin, 0.5 is centered, etc.
*/
public float getLayoutAlignmentX(Container target) {
return 0;
}
/** Returns the alignment along the y axis. This specifies how
* the component would like to be aligned relative to other
* components. The value should be a number between 0 and 1
* where 0 represents alignment along the origin, 1 is aligned
* the furthest away from the origin, 0.5 is centered, etc.
*/
public float getLayoutAlignmentY(Container target) {
return 0;
}
/** Invalidates the layout, indicating that if the layout manager
* has cached information it should be discarded.
*/
public void invalidateLayout(Container target) {
}
/** A mapping <Component, AbsoluteConstraints> */
protected java.util.Hashtable constraints = new java.util.Hashtable();
}
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/** An object that encapsulates position and (optionally) size for
* Absolute positioning of components.
*
* @see
* @version 1.01, Aug 19, 1998
*/
public class AbsoluteConstraints implements java.io.Serializable {
/** generated Serialized Version UID */
static final long serialVersionUID = 5261460716622152494L;
/** The X position of the component */
public int x;
/** The Y position of the component */
public int y;
/** The width of the component or -1 if the component's preferred width should be used */
public int width = -1;
/** The height of the component or -1 if the component's preferred height should be used */
public int height = -1;
/** Creates a new AbsoluteConstraints for specified position.
* @param pos The position to be represented by this AbsoluteConstraints
*/
public AbsoluteConstraints(Point pos) {
this (pos.x, pos.y);
}
/** Creates a new AbsoluteConstraints for specified position.
* @param x The X position to be represented by this AbsoluteConstraints
* @param y The Y position to be represented by this AbsoluteConstraints
*/
public AbsoluteConstraints(int x, int y) {
this.x = x;
this.y = y;
}
/** Creates a new AbsoluteConstraints for specified position and size.
* @param pos The position to be represented by this AbsoluteConstraints
* @param size The size to be represented by this AbsoluteConstraints or null
* if the component's preferred size should be used
*/
public AbsoluteConstraints(Point pos, Dimension size) {
this.x = pos.x;
this.y = pos.y;
if (size != null) {
this.width = size.width;
this.height = size.height;
}
}
/** Creates a new AbsoluteConstraints for specified position and size.
* @param x The X position to be represented by this AbsoluteConstraints
* @param y The Y position to be represented by this AbsoluteConstraints
* @param width The width to be represented by this AbsoluteConstraints or -1 if the
* component's preferred width should be used
* @param height The height to be represented by this AbsoluteConstraints or -1 if the
* component's preferred height should be used
*/
public AbsoluteConstraints(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
/** @return The X position represented by this AbsoluteConstraints */
public int getX () {
return x;
}
/** @return The Y position represented by this AbsoluteConstraints */
public int getY () {
return y;
}
/** @return The width represented by this AbsoluteConstraints or -1 if the
* component's preferred width should be used
*/
public int getWidth () {
return width;
}
/** @return The height represented by this AbsoluteConstraints or -1 if the
* component's preferred height should be used
*/
public int getHeight () {
return height;
}
public String toString () {
return super.toString () +" [x="+x+", y="+y+", width="+width+", height="+height+"]";
}
}
Apache NetBeans绝对布局
最新推荐文章于 2024-08-07 10:14:41 发布
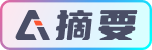