1、效果图
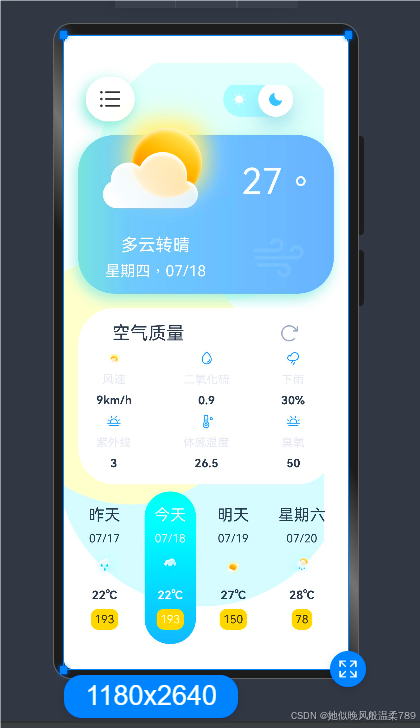
2、代码
2.1 DataModel.ets
@Observed
export class Weather {
icons: Resource;
name: string;
num: string;
constructor(icons: Resource, name: string, num: string) {
this.icons = icons;
this.name = name;
this.num = num;
}
}
@Observed
export class BottomWeather {
day: string;
name: string;
icons: Resource;
templ: string;
num: string;
isThisDay: boolean;
colors: string;
constructor(day: string, name: string, icons: Resource, templ: string, num: string, isThisDay: boolean, colors: string) {
this.name = name;
this.templ = templ;
this.day = day;
this.icons = icons;
this.num = num;
this.isThisDay = isThisDay;
this.colors = colors;
}
}
interface WeatherInterFace {
data: WeatherInterFaceData
}
interface WeatherInterFaceData {
list: Data[]
}
export interface Data {
day: string;
name: string;
icons: Resource;
templ: string;
num: string;
isThisDay: boolean;
colors: string;
}
export const res: WeatherInterFace = {
data: {
list: [{
day: "昨天",
name: "07/17",
icons: $r("app.media.ButtomRing"),
templ: "22℃",
num: "193",
isThisDay: false,
colors: "#ffd400"
}, {
day: "今天",
name: "07/18",
icons: $r("app.media.ButtomWindowRing"),
templ: "22℃",
num: "193",
isThisDay: true,
colors: "#ffd400"
}, {
day: "明天",
name: "07/19",
icons: $r("app.media.ButtomSun"),
templ: "27℃",
num: "150",
isThisDay: false,
colors: "#00ffaa"
}, {
day: "星期六",
name: "07/20",
icons: $r("app.media.ButtomSunRing"),
templ: "28℃",
num: "78",
isThisDay: false,
colors: "#ff6264"
}
],
}
}
2.2 IndexPage.ets
import AirShow from './views/AirShow'
import Top from './views/Top'
import WeatherShow from './views/WeatherShow'
import BottomShow from './views/BottomShow'
@Entry
@Component
struct IndexPage {
@State message: string = 'Hello World'
build() {
Scroll() {
Column() {
Top()
WeatherShow()
AirShow()
BottomShow()
}.margin(0)
.padding(0)
.width('100%')
.backgroundImage($r("app.media.backImageMask1"))
}
}
}
2.3 Top.ets
@Component
export default struct Top {
@State message: string = 'Hello World'
build() {
Row({space : 120}) {
Button() {
Image($r("app.media.Vector")).height(22)
}.padding(20)
.backgroundColor(Color.White)
.shadow({radius : 50, color:"#93DDD3", offsetX:-10, offsetY: 20})
.margin({left : 30})
Image($r("app.media.menuRight"))
.width(150)
.margin({top : 30})
}
.height(100)
.width('100%')
}
}
2.4 WeatherShow.ets
@Component
export default struct WeatherShow {
build() {
Column() {
Row() {
Image($r("app.media.sun")).height(200).margin({ top: -70 })
Text("27。").fontSize(50).fontColor(Color.White)
}
Row({ space: 60 }) {
Column({ space: 10 }) {
Text("多云转晴").fontColor(Color.White).fontSize(24)
Text("星期四,07/18").fontColor(Color.White).fontSize(21)
}
Image($r("app.media.Wind")).width(80)
}.margin({ bottom: 10 })
}
.linearGradient({
direction: GradientDirection.Right,
colors: [['#91D9D5', 0], ['#85C2E4', 0.2], ['#7AAEF3', 1]]
})
.width("90%")
.borderRadius(50)
.shadow({ radius: 50, color: "#fface5dd", offsetX: -10, offsetY: 20 })
}
}
2.5 AirShow.ets
import { Weather } from './DataModel';
@Component
export default struct AirShow {
@State weatherData: Weather[] = [
new Weather($r('app.media.sun'), "风速", "9km/h"),
new Weather($r('app.media.DropIcon'), "二氧化硫", "0.9"),
new Weather($r('app.media.CloudRainIcon'), "下雨", "30%"),
new Weather($r('app.media.SunHorizonIcon'), "紫外线", "3"),
new Weather($r('app.media.ThermometerIcon'), "体感温度", "26.5"),
new Weather($r('app.media.SunHorizonIcon'), "臭氧", "50"),
]
build() {
Column() {
Row({space : 130}) {
Text('空气质量').fontSize(25)
Image($r('app.media.ArrowClockwise')).width(30)
}.margin({top: 20})
Column() {
Flex({ justifyContent: FlexAlign.SpaceBetween, alignItems: ItemAlign.Center, wrap: FlexWrap.Wrap }) {
ForEach(this.weatherData, (weather: Weather) => {
WeatherIocn({
weather: weather
})
})
}.margin({left:25, bottom: 20})
}
}.margin({ top: 20 })
.width("90%")
.borderRadius(50)
.backgroundColor(Color.White)
}
}
@Component
struct WeatherIocn {
@ObjectLink weather: Weather;
build() {
Column({space : 10}) {
Image(this.weather.icons).width(20)
Text(this.weather.name).fontColor("#e6e9f2")
Text(this.weather.num).fontWeight(FontWeight.Bold)
}.margin({right : 40, top : 10})
}
}
2.6 BottomShow.ets
import { BottomWeather } from './DataModel'
import { res, Data } from './DataModel'
@Component
export default struct BottomShow {
@State bottomWeather: BottomWeather[] = [
new BottomWeather("昨天", "07/17", $r("app.media.ButtomRing"), "22℃", "193", false, "#ffd400"),
new BottomWeather("今天", "07/18", $r("app.media.ButtomWindowRing"), "22℃", "193", true, "#ffd400"),
new BottomWeather("明天", "07/19", $r("app.media.ButtomSun"), "27℃", "150", false, "#00ffaa"),
new BottomWeather("星期六", "07/20", $r("app.media.ButtomSunRing"), "28℃", "78", false, "#ff6264"),
]
build() {
Row({ space: 20 }) {
ForEach(res.data.list, (item: Data) => {
Column({ space: 10 }) {
Text(item.day).fontSize(22).fontColor(item.isThisDay ? Color.White : '')
Text(item.name).fontColor(item.isThisDay ? Color.White : '')
Image(item.icons).height(40)
Text(item.templ).fontWeight(FontWeight.Bold).fontColor(item.isThisDay ? Color.White : '')
Text(item.num)
.backgroundColor("#ffd400")
.padding(5)
.border({ radius: 10 })
.fontColor(item.isThisDay ? Color.White : '')
}.padding({ top: 20, bottom: 20, left: 10, right: 10 })
.linearGradient({
colors: item.isThisDay ? [["#3cf4dd", 0], ["#35dff2", 0.3], ["#3babff", 1]] : []
}).borderRadius(35)
.margin({ top: -20 })
})
}
.margin({ top: 30, bottom: 30 })
.alignItems(VerticalAlign.Top)
}
}