题1
head.h
#ifndef __HEAD_H__
#define __HEAD_H__
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef unsigned int sizeof_t;
char *Create();
void Input(char *p);
sizeof_t my_strlen(const char *p);
char *my_strcat(char *dest,const char *src);
void Bubble(char *s);
char *free_fun(char *p);
#endif
tese.c
#include "head11.h"
//堆区申请空间
char *Create()
{
char *p=(char *)malloc(sizeof(char)*20);
if(p == NULL)
return NULL;
else
return p;
}
//输入
void Input(char *p)
{
printf("请输入一个字符串:");
scanf("%s",p);
}
//计算字符串长度(非函数)
sizeof_t my_strlen(const char *p)
{
sizeof_t i=0;
while(*(p+i) != '\0')
i++;
return i;
}
//字符串连接
char *my_strcat(char *dest,const char *src)
{
int i;
for(i=0; *(dest+i)!='\0'; i++);
for(int j=0; *(src+j)!='\0'; j++)
{
*(dest+i) = *(src+j);
i++;
}
return dest;
}
//bubble
void Bubble(char *s)
{
int len=0;
while(*(s+len) != '\0')
len++;
for(int i=0; i<len-1; i++)
{
int count=0;
for(int j=0; j<len-i-1; j++)
{
if(*(s+j) > *(s+j+1))
{
*(s+j) = *(s+j) ^ *(s+j+1);
*(s+j+1) = *(s+j) ^ *(s+j+1);
*(s+j) = *(s+j) ^ *(s+j+1);
count++;
}
}
if(count == 0)
break;
}
}
//释放空间
char *free_fun(char *p)
{
if(p != NULL)
{
free(p);
p=NULL;
}
return p;
}
main.c
#include "head11.h"
int main(int argc, const char *argv[])
{
char *s1 = Create();
char *s2 = Create();
Input(s1);
Input(s2);
sizeof_t len1 = my_strlen(s1);
sizeof_t len2 = my_strlen(s2);
printf("strlen(s1) = %d\n",len1);
printf("strlen(s2) = %d\n",len2);
char *s = my_strcat(s1,s2);
printf("s = %s\n",s);
printf("-------------------\n");
Bubble(s);
printf("s = %s\n",s);
s1 = free_fun(s1);
s2 = free_fun(s2);
return 0;
}
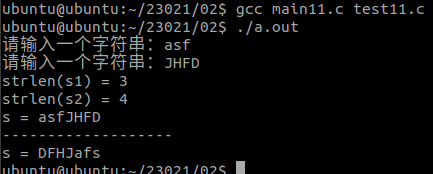
题2
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 64
struct car
{
char name[N];
char colour[N];
float price;
}c[5];
void Input(struct car *p)
{
for(int i=0; i<5; i++)
{
printf("品牌:");
scanf("%s",(p+i)->name);
printf("颜色:");
scanf("%s",(p+i)->colour);
printf("价格:");
scanf("%f",&(p+i)->price);
}
}
void Output(struct car *p)
{
printf("品牌\t颜色\t价格\n");
for(int i=0; i<5; i++)
{
printf("%s\t%s\t%.2fW\n",(p+i)->name,(p+i)->colour,(p+i)->price);
}
}
int Max(struct car *p)
{
int maxi;
for(int i=0; i<5; i++)
{
if(i == 0)
maxi = i;
if((p+maxi)->price < (p+i)->price)
maxi = i;
}
return maxi;
}
int main(int argc, const char *argv[])
{
//定义5辆车的信息,结构体成员包含【品牌、颜色、价格】
Input(c);
Output(c);
int maxi = Max(c);
printf("最贵的车辆信息为:\n%s\t%s\t%.2fW\n",c[maxi].name,c[maxi].colour,c[maxi].price);
return 0;
}
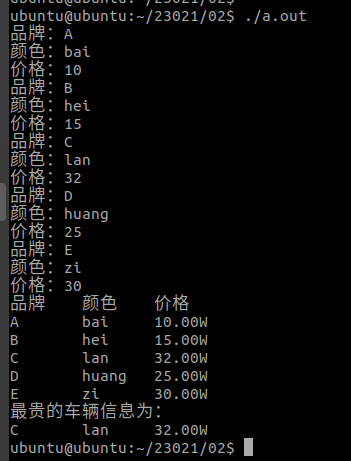