将
MyBatis
与
Spring
进行整合,主要解决的问题就是将
SqlSessionFactory
对象交由
Spring
来管理。所以,该整合,只需要将
SqlSessionFactory
的对象生成器
SqlSessionFactoryBean
注
册 在
Spring
容器中,再将其注入给
Dao
的实现类即可完成整合。
实现
Spring
与
MyBatis
的整合常用的方式:扫描的
Mapper
动态代理。Spring 像插线板一样,
mybatis
框架是插头,可以容易的组合到一起。插线板
spring
插
上
mybatis
,两个框架就是一个整体
项目结构
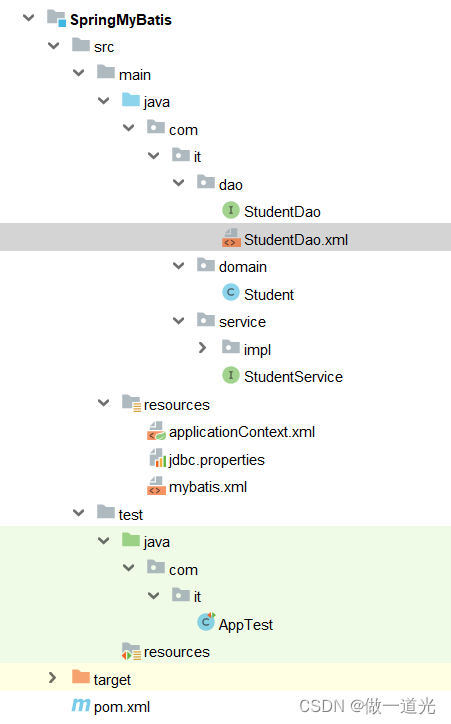
4.1.1MySQL
创建数据库
springdb,
新建表
Student
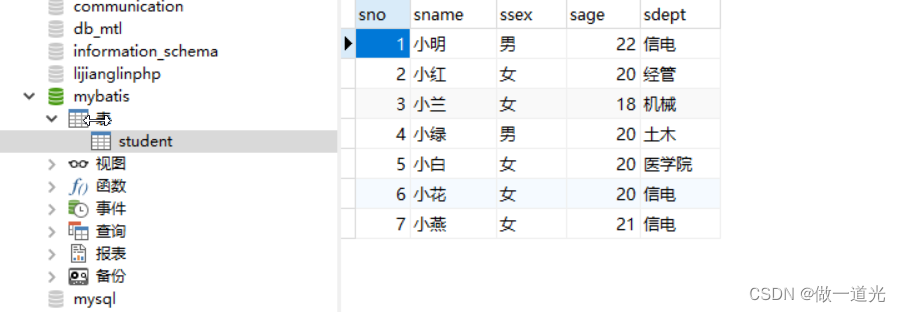
4.1.2maven
依赖
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>spring3</artifactId>
<groupId>com.it</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>SpringMyBatis</artifactId>
<name>SpringMyBatis</name>
<!-- FIXME change it to the project's website -->
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<dependencies>
<!-- 单元测试-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<!-- spring核心ioc-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!--做spring事务用到的-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!-- mybatis依赖-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.1</version>
</dependency>
<!-- mybatis和spring集成的依赖-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.1</version>
</dependency>
<!--mysql驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.9</version>
</dependency>
<!-- 阿里公司的数据库连接池-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.12</version>
</dependency>
</dependencies>
<build>
<!-- 设置编译compile生成traget文件时可以把后缀为xml的文件一起加入进去-->
<!-- 现在是把src/main/java目录中的xml文件包含到输出结果中,输出到target/classes目录中-->
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
<!-- 指定jdk的版本 -->
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
<pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) -->
<plugins>
<!-- clean lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#clean_Lifecycle -->
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.1.0</version>
</plugin>
<!-- default lifecycle, jar packaging: see https://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_jar_packaging -->
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.1</version>
</plugin>
<plugin>
<artifactId>maven-jar-plugin</artifactId>
<version>3.0.2</version>
</plugin>
<plugin>
<artifactId>maven-install-plugin</artifactId>
<version>2.5.2</version>
</plugin>
<plugin>
<artifactId>maven-deploy-plugin</artifactId>
<version>2.8.2</version>
</plugin>
<!-- site lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#site_Lifecycle -->
<plugin>
<artifactId>maven-site-plugin</artifactId>
<version>3.7.1</version>
</plugin>
<plugin>
<artifactId>maven-project-info-reports-plugin</artifactId>
<version>3.0.0</version>
</plugin>
</plugins>
</pluginManagement>
</build>
</project>
4.1.3定义实体类
Student
package com.it.domain;
public class Student {
private Integer sno;
private String sname;
private String ssex;
private Integer sage;
private String sdept;
public Student() {
}
public Student(Integer sno, String sname, String ssex, Integer sage, String sdept) {
this.sno = sno;
this.sname = sname;
this.ssex = ssex;
this.sage = sage;
this.sdept = sdept;
}
public Integer getSno() {
return sno;
}
public void setSno(Integer sno) {
this.sno = sno;
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public String getSsex() {
return ssex;
}
public void setSsex(String ssex) {
this.ssex = ssex;
}
public Integer getSage() {
return sage;
}
public void setSage(Integer sage) {
this.sage = sage;
}
public String getSdept() {
return sdept;
}
public void setSdept(String sdept) {
this.sdept = sdept;
}
@Override
public String toString() {
return "Student{" +
"sno=" + sno +
", sname='" + sname + '\'' +
", ssex='" + ssex + '\'' +
", sage=" + sage +
", sdept='" + sdept + '\'' +
'}';
}
}
4.1.4定义
StudentDao
接口
package com.it.dao;
import com.it.domain.Student;
import java.util.List;
public interface StudentDao {
List<Student> selectStudents();
int insertStudent(Student student);
}
4.1.5定义映射文件
mapper
在Dao
接口的包中创建
MyBatis
的映射文件
mapper,命名与接口名相同,本例StudentDao.xml
。
mapper
中的
namespace
取值也为
Dao
接口的全限定性名。
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.it.dao.StudentDao">
<select id="selectStudents" resultType="com.it.domain.Student">
select * from student order by sno desc
</select>
<insert id="insertStudent">
insert into student values (#{sno},#{sname},#{ssex},#{sage},#{sdept})
</insert>
</mapper>
4.1.6
定义
Service
接口和实现类
接口
package com.it.service;
import com.it.domain.Student;
import java.util.List;
public interface StudentService {
List<Student> queryStudent();
int addStudent(Student student);
}
实现类
package com.it.service.impl;
import com.it.dao.StudentDao;
import com.it.domain.Student;
import com.it.service.StudentService;
import java.util.List;
public class StudentServiceImpl implements StudentService {
// 引用类型
private StudentDao studentDao;
//使用set注入的方式
public void setStudentDao(StudentDao studentDao) {
this.studentDao = studentDao;
}
@Override
public List<Student> queryStudent() {
List<Student> students = studentDao.selectStudents();
return students;
}
@Override
public int addStudent(Student student) {
int i = studentDao.insertStudent(student);
return i;
}
}
4.1.7
定义
MyBatis
主配置文件
在
src
下定义
MyBatis
的主配置文件,命名为
mybatis.xml
。
这里有两点需要注意:
(
1
)主配置文件中不再需要数据源的配置了。因为数据源要交给
Spring
容器来管理了。
(
2
)这里对
mapper
映射文件的注册,使用
<package/>
标签,即只需给出
mapper
映射文件所在的包即可。因为 mapper
的名称与
Dao
接口名相同,可以使用这种简单注册方式。这种方式的好处是,若有多个映射文件,这里的配置也是不用改变的。当然,也可使用原来的<resource/>标签方式。
c
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- 设置日志-->
<settings>
<setting name="logImpl" value="STDOUT_LOGGING"/>
</settings>
<!-- 设置别名-->
<typeAliases>
<!-- name:实体类所在的包名,表示这个包下的所有类都叫做这个别名-->
<package name="com.it.domain"/>
</typeAliases>
<mappers>
<!-- name:是包名,这个包中的所有的mapper.xml一次都能加载!-->
<package name="com.it.dao"/>
</mappers>
</configuration>
创建测试类
package com.it;
import static org.junit.Assert.assertTrue;
import com.it.dao.StudentDao;
import com.it.domain.Student;
import com.it.service.StudentService;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.util.List;
/**
* Unit test for simple App.
*/
public class AppTest
{
@Test
public void test1(){
String config="applicationContext.xml";
ApplicationContext ac=new ClassPathXmlApplicationContext(config);
String[] names = ac.getBeanDefinitionNames();
for (String name:names){
System.out.println("容器中对象的名字:"+name+"|"+ac.getBean(name));
}
}
@Test
public void test2(){
String config="applicationContext.xml";
ApplicationContext ac=new ClassPathXmlApplicationContext(config);
StudentDao studentDao = (StudentDao) ac.getBean("studentDao");
Student student=new Student();
student.setSno(10);
student.setSname("迪迦奥特曼");
student.setSsex("男");
student.setSage(30);
student.setSdept("软件学院");
int i = studentDao.insertStudent(student);
System.out.println("i="+i);
}
@Test
public void test3(){
String config="applicationContext.xml";
ApplicationContext ac=new ClassPathXmlApplicationContext(config);
StudentService service = (StudentService) ac.getBean("studentService");
Student student=new Student();
student.setSno(11);
student.setSname("赛文奥特曼");
student.setSsex("男");
student.setSage(35);
student.setSdept("软件学院");
int i = service.addStudent(student);
// spring和mybatis整合在一起使用,事务是自动提交的。无需执行SqlSession.commit();
System.out.println("i="+i);
}
@Test
public void test4(){
String config="applicationContext.xml";
ApplicationContext ac=new ClassPathXmlApplicationContext(config);
StudentService service = (StudentService) ac.getBean("studentService");
List<Student> studentList = service.queryStudent();
for (Student student:studentList){
System.out.println(student);
}
// spring和mybatis整合在一起使用,事务是自动提交的。无需执行SqlSession.commit()
}
}
测试结果
1、
2、
3、
4、