2.队列
2.1队列的概念及结构
队列:只允许在一端进行插入数据操作,在另一端进行删除数据操作的特殊线性表,队列具有先进先出FIFO(First In First Out)
入队列:进行插入操作的一端称为队尾
出队列:进行删除操作的一端称为队头
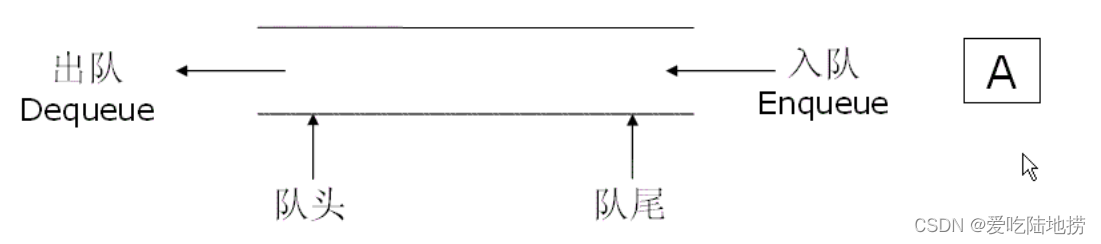
2.2链队列的实现
队列也可以数组和链表的结构实现,使用链表的结构实现更优一些,因为如果使用数组的结构,出队列在数组头上出数据,效率会比较低
#include<stdio.h>
#include<assert.h>
#include<stdlib.h>
#include<stdbool.h>
typedef int QDataType;
typedef struct QueueNode
{
struct QueueNode* next;
QDataType data;
}QueueNode;
typedef struct Queue
{
QueueNode* head;
QueueNode* tail;
}Queue;
1)初始化队列
void QueueInit(Queue* q)//初始化队列
{
assert(q!=NULL);
q->head = q->tail = NULL;
}
2)销毁队列
void QueueDestroy(Queue* q)//销毁队列
{
assert(q != NULL);
QueueNode* p = q->head;
while (p != NULL)
{
QueueNode* s = p->next;
free(p);
p = s;
}
}
3)队尾入队列
void QueuePush(Queue* q, QDataType x)//队尾入队列
{
assert(q!=NULL);
QueueNode* newnode = (QueueNode*)malloc(sizeof(QueueNode));
if (newnode == NULL)exit(1);
newnode->data = x;
newnode->next = NULL;
if (q->head == NULL)
{
q->head=q->tail= newnode;
}
else
{
q->tail->next = newnode;
q->tail = q->tail->next;
}
}
4)队头出队列
void QueuePop(Queue* q)//队头出队列
{
assert(q != NULL);
assert(!QueueEmpty(q));
if (q->head->next == NULL)
{
free(q->head);
q->head = q->tail = NULL;
}
else
{
QueueNode* s = q->head;
q->head = q->head->next;
free(s);
}
}
5)获取队列头部元素
QDataType QueueFront(Queue* q)//获取队列头部元素
{
assert(q != NULL);
assert(!QueueEmpty(q));
return q->head->data;
}
6)获取队列队尾元素
QDataType QueueBack(Queue* q)//获取队列队尾元素
{
assert(q != NULL);
assert(!QueueEmpty(q));
return q->tail->data;
}
7)检测队列是否为空
bool QueueEmpty(Queue* q)//检测队列是否为空
{
assert(q != NULL);
return q->head == NULL;
}
8)获取队列中有效元素个数
int QueueSize(Queue* q)//获取队列中有效元素个数
{
assert(q != NULL);
int i = 0;
QueueNode* s = q->head;
while (s != NULL)
{
i++;
s = s->next;
}
return i;
}
队列的应用场景
1.排队,保持绝对公平性
2.广度优先遍历