watch监听基本使用
- watch是一个函数,需要引入使用,监听多个数据只要多次调用watch函数即可
- watch第一个参数是监听的数据
- watch第二个参数是监听后的回调函数,参数为newVal和oldVal
- watch第三个参数是配置对象,包含immediate和deep
watch监听ref基本类型数据
<template>
<div>watch监听</div><br/>
<div @click="count++" style="cursor: pointer;">点击增加: {{count}}</div>
</template>
<script lang="ts">
import {defineComponent} from "vue";
export default defineComponent({
name: "WatchPra"
})
</script>
<script setup lang="ts">
import {ref,watch} from 'vue';
let count = ref<number>(0);
watch(count,(newVal,oldVal)=>{
console.log('监听到数据:',newVal,oldVal)
},{immediate:true})
</script>
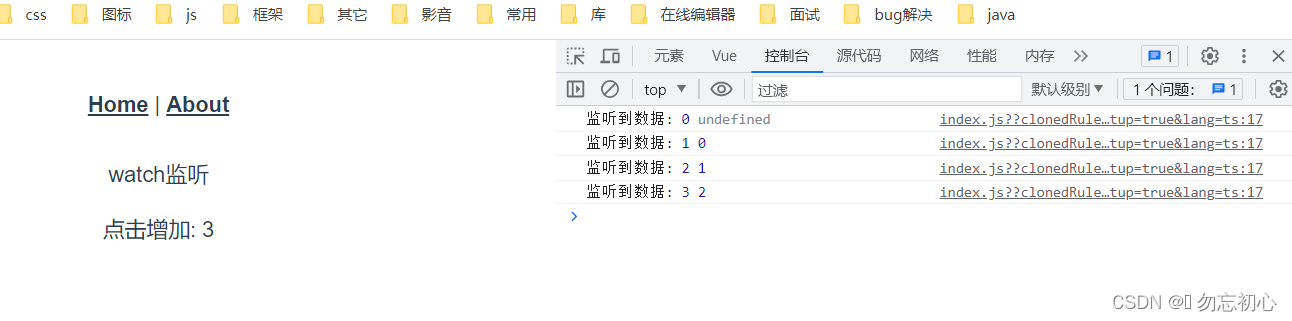
watch监听ref复杂类型数据
- 直接监听ref对象,只能监听到当前ref对象的值被替换
- 如果想监听ref代理对象的改变,则需要设置deep进行深度监听
- 直接监听ref对象的value值,会默认开启深度监听
- 监听ref代理对象内部的某个属性值,值是复杂数据类型时,默认开启深度监听,如果参数一使用函数式写法,不会默认开启深度监听
- 监听ref对象内部某个属性值,值是基本数据类型时,写法参数一使用函数式写法,默认开启深度监听
<template>
<div>watch监听</div><br/>
<!-- <div @click="count++" style="cursor: pointer;">点击增加: {{count}}</div>-->
<div @click="cat.age++" style="cursor: pointer;">点击增加: {{cat}}</div>
</template>
<script lang="ts">
import {defineComponent} from "vue";
export default defineComponent({
name: "WatchPra"
})
</script>
<script setup lang="ts">
import {ref,watch} from 'vue';
let cat = ref<object>({name:'小五',age:3,color:'orange'})
watch(cat,(newVal,oldVal)=>{
console.log('监听到变化: ', {cat})
})
</script>
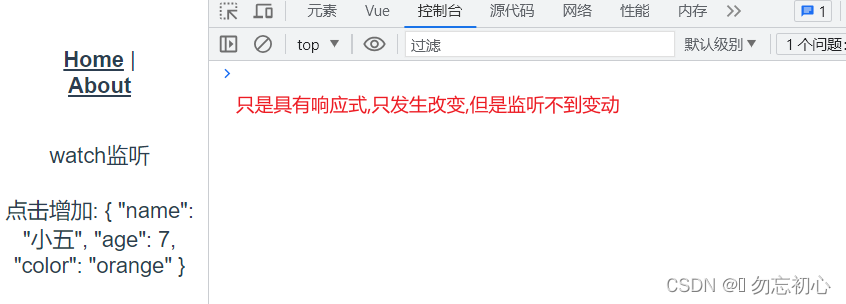
<template>
<div>watch监听</div><br/>
<!-- <div @click="count++" style="cursor: pointer;">点击增加: {{count}}</div>-->
<div @click="cat.age++" style="cursor: pointer;">点击增加: {{cat}}</div>
<div @click="cat={name:'小五',age:3,color:'white'}" style="cursor: pointer;">点击替换: {{cat}}</div>
</template>
<script lang="ts">
import {defineComponent} from "vue";
export default defineComponent({
name: "WatchPra"
})
</script>
<script setup lang="ts">
import {ref,watch} from 'vue';
let cat = ref<object>({name:'小五',age:3,color:'orange'})
watch(cat,(newVal,oldVal)=>{
console.log('监听到变化: ', {cat})
})
</script>
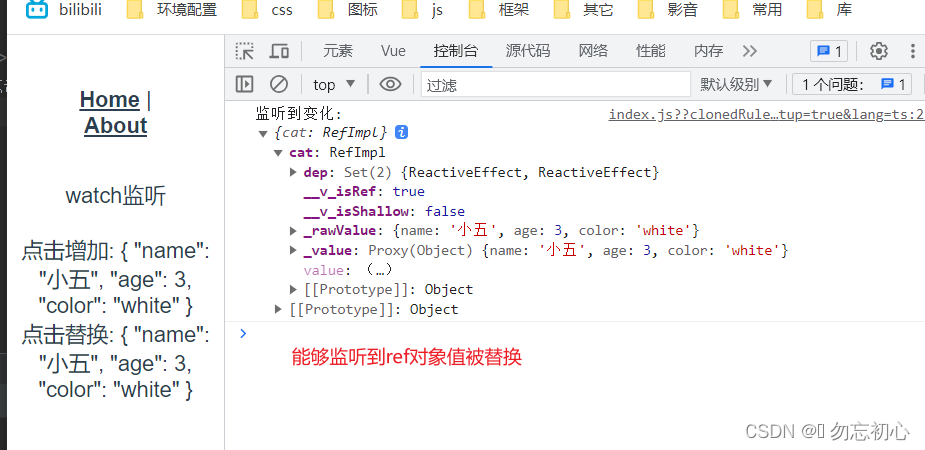
<template>
<div>watch监听</div><br/>
<!-- <div @click="count++" style="cursor: pointer;">点击增加: {{count}}</div>-->
<!-- <div @click="cat.age++" style="cursor: pointer;">点击增加: {{cat}}</div>-->
<!-- <div @click="cat={name:'小五',age:3,color:'white'}" style="cursor: pointer;">点击替换: {{cat}}</div>-->
<div @click="cat.active.push('抓老鼠')" style="cursor: pointer;">点击添加数据: {{cat}}</div>
</template>
<script lang="ts">
import {defineComponent} from "vue";
export default defineComponent({
name: "WatchPra"
})
</script>
<script setup lang="ts">
import {ref,watch} from 'vue';
let cat = ref<object>({name:'小五',age:3,color:'orange',active:['睡','吃']})
watch(cat.value,(newVal,oldVal)=>{
console.log('监听到变化: ', {cat})
})
</script>
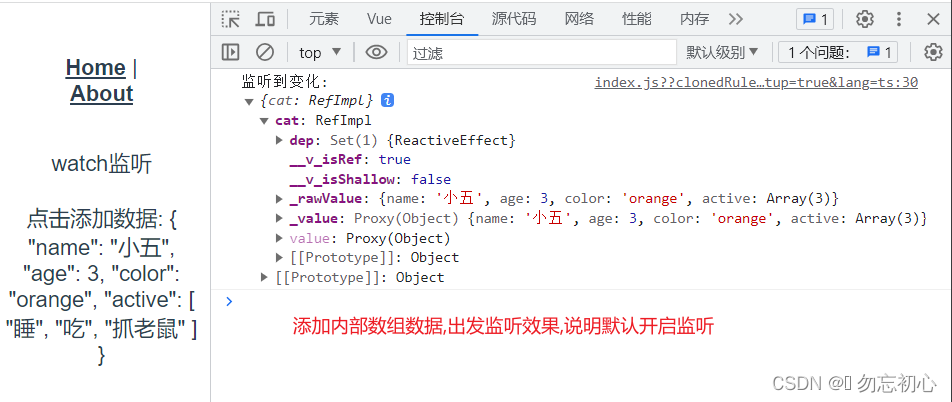
<template>
<div>watch监听</div><br/>
<!-- <div @click="count++" style="cursor: pointer;">点击增加: {{count}}</div>-->
<!-- <div @click="cat.age++" style="cursor: pointer;">点击增加: {{cat}}</div>-->
<!-- <div @click="cat={name:'小五',age:3,color:'white'}" style="cursor: pointer;">点击替换: {{cat}}</div>-->
<!-- <div @click="cat.active.push('抓老鼠')" style="cursor: pointer;">点击添加数据: {{cat}}</div>-->
<div @click="person.reactive.son = '小小姜'" style="cursor: pointer;">点击改变数据: {{person}}</div>
</template>
<script lang="ts">
import {defineComponent} from "vue";
export default defineComponent({
name: "WatchPra"
})
</script>
<script setup lang="ts">
import {ref,watch} from 'vue';
let person = ref({name:'老姜',age:18,sex:'男',reactive:{ son:'小姜', sister:'小姜妹' }});
watch(()=>person.value.reactive.son,(newVal,oldVal)=>{
console.log('监听到: ',person.value.reactive)
})
</script>
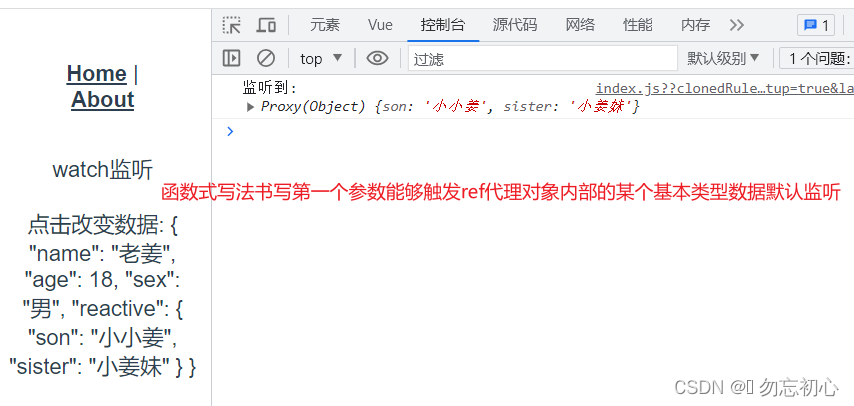
监听reactive定义数据
- 监听reactive定义的代理数据,写法和逻辑和监听ref定义复杂数据的代理数据一样
watch监听高级用法