常见的cmd命令
类 class
字面量
数据类型
输入
public static void main(String[] args) {
Scanner a=new Scanner(System.in);
int n=a.nextInt();
int m=a.nextInt();
System.out.println(m+n);
}
}
算数运算符
package wclg;
public class test {
public static void main(String[] args) {
System.out.println("123"+123);
System.out.println(1+99+"武昌理工");
}
}
符合运算符隐藏了强制类型转换
逻辑运算符
短路运算符
&& || 和& | 结果一样,只不过效率高
原反补
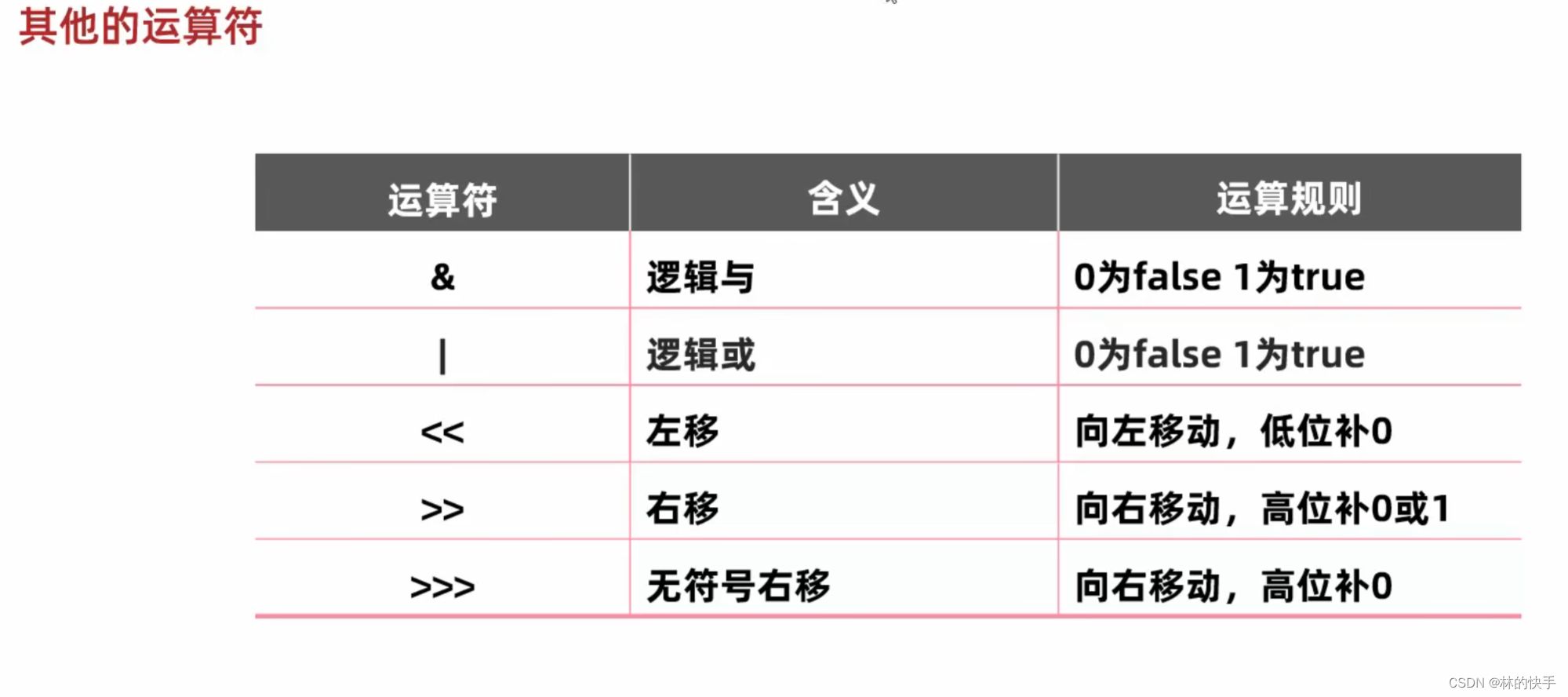
循环判断
Switch jdk12以上的特性
import java.util.Scanner;
public class day4 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
switch (n) {
case 1 -> {
System.out.println("一");
}
case 2 -> {
System.out.println("二");
}
case 3 -> {
System.out.println("三");
}
default -> {
System.out.println("没有这种选项");
}
}
}
}
获取随机数
猜数字小游戏
package tets;
import java.util.Random;
import java.util.Scanner;
public class Test7 {
public static void main(String[] args) {
Random rand = new Random();
int n = rand.nextInt(100)+1;
while(true){
Scanner sc = new Scanner(System.in);
int m=sc.nextInt();
if(n==m){
System.out.println("猜对了");
break;
}
else if(m>n){
System.out.println("猜大了");
}
else {
System.out.println("猜小了");
}
}
}
}
数组
流行的写法
数组的长度是 数组.length
数组名.fori
动态数组初始化
public class Test5 {
public static void main(String[] args) {
int [] arr =new int [5];
for(int i=0;i<arr.length;i++){
Scanner sc=new Scanner(System.in);
arr[i]=sc.nextInt();
}
for (int i = 0; i < arr.length; i++) {
System.out.printf("%d ", arr[i]);
}
}
}
整型数组未初始化默认为0
打乱数组元素,随机交换元素
package wclg;
import java.util.Random;
public class class_61 {
public static void main(String[] args) {
int []arr={1,2,3,4,5};
Random r = new Random();
for (int i = 0; i < arr.length; i++) {
int num = r.nextInt(arr.length);
int tmp = arr[num];
arr[num] = arr[i];
arr[i] = tmp;
}
for (int i = 0; i < arr.length; i++) {
System.out.printf("%d ", arr[i]);
}
}
}
数组的内存分配
arr2 存的是arr1的地址,通过arr1的地址内容打印出相应的值
修改arr2的值,相当于通过arr2修改了所指的地址内容值
二维数组
方法
方法是程序中最小的执行单元
也就相当于c语言的函数
重载
重载顾名思义就是方法名相同,参数不同,编译器会根据参数不同调用相应的方法
import java.util.Scanner;
public class class_64 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
int c = sc.nextInt();
int sum1=Add(a,b);
System.out.println(sum1);
int sum2=Add(a,b,c);
System.out.println(sum2);
}
public static int Add(int a,int b){
return a+b;
}
public static int Add(int a,int b,int c){
return a+b+c;
}
}
输出语句的认识
方法就是先进后出
引用
引用数据变量其实就是存的是地址值
import java.sql.SQLOutput;
import java.util.Scanner;
public class class_69 {
public static void fun1(int []arr){
System.out.print("[");
for (int i = 0; i < arr.length; i++) {
System.out.printf("%d ",arr[i]);
if(i<arr.length-1){
System.out.print(",");
}
}
System.out.println("]");
}
public static int fun2(int []arr){
int max=arr[0];
for (int i = 1; i < arr.length; i++) {
if(arr[i]>max){
max=arr[i];
}
}
return max;
}
public static int fun3(int []arr,int n){
for (int i = 0; i < arr.length; i++) {
if(arr[i]==n){
return 1;
}
}
return 0;
}
public static int[] fun4(int []arr,int from,int to){
int []temp=new int[to-from];
int j=0;
for (int i = from; i < to; i++) {
temp[j++]=arr[i];
}
return temp;
}
public static void main(String[] args) {
int []arr={11,22,33,44,55};
fun1(arr);
int max=fun2(arr);
System.out.println("max:="+max);
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
int ret=fun3(arr,n);
if(ret==1)
System.out.println("存在");
else
System.out.println("不存在");
int from=sc.nextInt();
int to=sc.nextInt();
int []copyArr= fun4(arr,from,to);
for (int i = 0; i < copyArr.length; i++) {
System.out.printf("%d ",copyArr[i]);
}
}
}
方法的值传递
传基本数据类型的方法.
public class Test1 {
public static void main(String[] args) {
int number=100;
int ret=fun(number);
System.out.println(ret);
}
public static int fun(int number) {
number=200;
return number;
}
}
传引用数据类型
public class Test2 {
public static void main(String[] args) {
int []arr={1,2,3,4,5};
fun(arr);
System.out.println(arr[0]);
}
public static void fun(int []arr){
arr[0]=100;
}
}
练习
import javax.swing.*;
import java.util.Scanner;
public class class_74 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double score = sc.nextDouble();
int month = sc.nextInt();
String ret = sc.next();
if (month >= 5 && month <= 10) {
double m = fun(score, ret,0.9,0.85);
System.out.println(m);
} else if (month >= 11 && month <= 12 || month >= 1 && month <= 4) {
double m = fun(score, ret,0.7,0.65);
System.out.println(m);
}
}
public static double fun ( double score, String ret,double a, double b) {
if (ret.equals("头等舱"))
score *= a;
else
score *= b;
return score;
}
}
ctrl+p看函数参数
ctrl+alt+m自动抽取方法
public class Test2 {
public static int is_prime(int i){
for (int j = 2; j <=Math.sqrt(i) ; j++) {
if(i % j == 0){
return 0;
}
}
return 1;
}
public static void main(String[] args) {
int count = 0;
for (int i = 101; i <=200; i++) {
if(is_prime(i)==1)
{
count++;
System.out.printf("%d ", i);
}
}
System.out.println();
System.out.println("total:="+count);
}
}
import javax.swing.*;
import java.util.Random;
public class Test3 {
public static void main(String[] args) {
//1.大写字母和小写字母放入数组当中
char []arr=new char[52];
for (int i = 0; i < arr.length; i++) {
if(i<=25) {
//添加小写字母
arr[i] = (char) (97 + i);
}else{
//-26之前i的值
arr[i] = (char) (65 + i-26);
}
}
//2.随机4次
//随机抽取数组的索引
//利用随机索引,获取对应的元素
//然后4个字母拼接一起
Random r=new Random();
int j=0;
String result="";
for (int i = 0; i < 4; i++) {
int index=r.nextInt(arr.length);
result+=arr[index];
}
//3.随机抽取一个数字0-9
Random c=new Random();
int a=c.nextInt(10);
//拼接数字
result+=a;
System.out.println(result);
}
}
public class Test4 {
public static void main(String[] args) {
int []arr = {1,2,3,4,5,6,7,8,9};
int []tmp = new int[arr.length];
for (int i = 0; i < arr.length; i++) {
tmp[i] = arr[i];
}
for (int i = 0; i < tmp.length; i++) {
System.out.printf("%d ", tmp[i]);
}
}
}
shift+F6一键替换
import java.util.Scanner;
public class Test5 {
public static void main(String[] args) {
int []newArr=getScore();
int max=getMax(newArr);
int min=getMin(newArr);
int sum=getSum(newArr);
int df= (sum-max-min)/4;
System.out.println(df);
}
public static int[] getScore() {
int []arr=new int[6];
Scanner sc=new Scanner(System.in);
for(int i=0;i<6;) {
int score=sc.nextInt();
if(score>=0 && score<=100) {
arr[i]=score;
i++;
}
else{
System.out.println("输入成绩错误");
}
}
return arr;
}
public static int getMax(int[] arr) {
int max=arr[0];
for (int i = 0; i < arr.length; i++) {
if(max<arr[i]) {
max=arr[i];
}
}
return max;
}
public static int getMin(int[] arr) {
int min=arr[0];
for (int i = 0; i < arr.length; i++) {
if(min>arr[i]) {
min=arr[i];
}
}
return min;
}
public static int getSum(int[] arr) {
int sum=0;
for (int i = 0; i < arr.length; i++) {
sum+=arr[i];
}
return sum;
}
}
import javax.swing.*;
import java.util.Scanner;
public class Test6 {
public static void main(String[] args) {
int n = 0;
Scanner sc = new Scanner(System.in);
n = sc.nextInt();
int count = 0;
int tmp=n;
while (tmp != 0) {
count++;
tmp/=10;
}
int ret = fun(n,count);
System.out.println(ret);
}
public static int fun(int n, int count){
int []arr = new int[count];
int i=arr.length-1;
while(n!=0){
arr[i--]=n%10;
n/=10;
}
for ( int j = 0;j <count; j++) {
arr[j]+=5;
arr[j]%=10;
}
int left=0;
int right=count-1;
while(left<right){
int tmp=arr[left];
arr[left]=arr[right];
arr[right]=tmp;
left++;
right--;
}
int number=0;
for (int k = 0; k < count; k++) {
number=number*10+arr[k];
}
return number;
}
}
import java.util.Random;
public class Tets8 {
public static void main(String[] args) {
int[]arr={2,588,888,1000,10000};
int[] newAarr=new int[arr.length];
Random random=new Random();
for(int i=0;i<5;){
int index=random.nextInt(arr.length);
int ret=arr[index];
if((fun(newAarr,ret))==0){
newAarr[i++]=ret;
}
}
for(int i=0;i<arr.length;i++){
System.out.print(newAarr[i]+" ");
}
}
public static int fun(int[] arr,int ret){
for(int i=0;i<arr.length;i++){
if(arr[i]==ret)
return 1;
}
return 0;
}
}
方法2
import java.util.Random;
public class Test9 {
public static void main(String[] args) {
int []arr= {2, 588, 888, 1000, 10000};
int []newArr=new int[arr.length];
Random myRandom=new Random();
for(int i=0;i<arr.length;i++){
int index=myRandom.nextInt(arr.length);
int temp=arr[index];
arr[index]=arr[i];
arr[i]=temp;
}
for(int i=0;i<arr.length;i++){
System.out.print(arr[i]+" ");
}
}
}
import java.util.Random;
import java.util.Scanner;
public class Test1 {
public static void main(String[] args) {
//1.生成中奖号码
int[] arr = fun1();//123456 7
//2.用户输入号码(红球+蓝球)
int[] newarr = fun2();//654321 7 也算,只要存在即可
//3.判断用户中奖情况
int readcount=0;
int bluecount=0;
//判断红球
for (int i = 0; i < newarr.length-1; i++) {
int readnum= newarr[i];
for (int j = 0; j < arr.length-1; j++) {
if(readnum==arr[j]){
readcount++;
break;
}
}
}
//判断蓝球
int bluednum= newarr[newarr.length-1];
if(bluednum==arr[arr.length-1]){
bluecount++;
}
}
public static int[] fun2() {
//用于用户购买的彩票号码
int[] arr = new int[7];
Scanner sc = new Scanner(System.in);
for (int i = 0; i < 6; ) {
System.out.println("请输入第" + (i + 1) + "个红球号码");
int readnum = sc.nextInt();
//要求红球在1-33之间,并且不重复才添加数组里面
if (readnum >= 1 && readnum <= 33) {
int flag = contains(arr, readnum);
if (flag == 0) {
arr[i] = readnum;
i++;
} else {
System.out.println("重复,请重新输入 ");
}
} else {
System.out.println("当前红球超出范围");
}
}
System.out.println("输入蓝球号码");
while (true) {
int bluenum = sc.nextInt();
if (bluenum >= 1 && bluenum <= 16) {
arr[arr.length - 1] = bluenum;
break;
} else {
System.out.println("蓝球号码超出范围请重新输入");
}
}
return arr;
}
public static int[] fun1() {
// 1.6个红球,一个蓝球
//红球不能重复,蓝球可以重复
int[] arr = new int[7];
Random r = new Random();
for (int i = 0; i < 6; ) {
//获取红球号码
int readNum = r.nextInt(33) + 1;
int flag = contains(arr, readNum);//保证红球不重复
if (flag == 0) {
arr[i] = readNum;
i++;
}
}
//获取蓝球号码
//最后一个蓝球号码
int bluenum = r.nextInt(16) + 1;
arr[arr.length - 1] = bluenum;
return arr;
}
public static int contains(int[] arr, int Num) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == Num) {
return 1;
}
}
return 0;
}
}
面向对象
封装,继承,多态,面向对象的三大特性
面向对象就是找到对应的方法解决对应的问题
类和对象
不写main方法的类
默认初始化值
'
封装
比如人画圆, 画圆的方法属于圆的方法
对象代表什么,就得封装对应的数据,并提供数据对应的行为
private关键字
set修改成员变量的值
get是获取成员变量的值
import java.util.Set;
public class GirlFriend {
private String name;
private int age;
//针对于每一个私有化成员变量,都要有Get和set方法
//set类方法:给成员变量赋值
//get类方法:对外提供成员变量的值
//作用:给成员变量name进行赋值
public void Set_name (String n){
name = n;
}
//对外提供name属性的
public String Get_name (){
return name;
}
//作用:给成员变量age进行赋值
public void Set_age (int a){
if(a>=18 && a<=25){
age=a;
}else {
System.out.println("非法参数");
}
}
//对外提供age属性的
public int Get_age (){
return age;
}
public void Sleep() {
System.out.println("她在睡觉");
}
public void eat() {
System.out.println("她在吃饭");
}
}
public class GirlFriendTest { public static void main(String[] args) { GirlFriend g = new GirlFriend(); g.Set_name("小囧囧"); g.Set_age(19); System.out.println(g.Get_name()); System.out.println(g.Get_age()); g.Sleep(); g.eat(); } }
this 关键字
成员和局部变量
加this就使用成员变量的age 也就是 private 的age
public class Test2 {
private int age;
public void method(){
int age=10;
System.out.println(this.age);
}
}
public class Tets1 {
public static void main(String[] args) {
Test2 t2=new Test2();
t2.method();
}
}
this的内存布局
this的本质是: 所在方法调用者的地址值
public class GirlFriendTest {
public static void main(String[] args) {
GirlFriend g = new GirlFriend();
g.Set_name("小囧囧");
g.Set_age(19);
System.out.println(g.Get_name());
System.out.println(g.Get_age());
g.Sleep();
g.eat();
}
}
import java.util.Set;
public class GirlFriend {
private String name;
private int age;
//针对于每一个私有化成员变量,都要有Get和set方法
//set类方法:给成员变量赋值
//get类方法:对外提供成员变量的值
//作用:给成员变量name进行赋值
public void Set_name (String name){
this.name = name;
}
//对外提供name属性的
public String Get_name (){
return name;
}
//作用:给成员变量age进行赋值
public void Set_age (int age){
if(age>=18 && age<=25){
this.age = age;
}else {
System.out.println("非法参数");
}
}
//对外提供age属性的
public int Get_age (){
return age;
}
public void Sleep() {
System.out.println("她在睡觉");
}
public void eat() {
System.out.println("她在吃饭");
}
}
构造
创造对象的时候 ,虚拟机会自动调用构造方法,作用是给成员变量进行初始化
有参构造和空参构造
构造就是public加类名
public class Student {
//如果我们自己没有任何的构造方法
//那么虚拟机给我们加一个空参构造方法
private String name;
private int age;
public Student(){
System.out.println("看看我执行没有");
}
public Student(String name, int age){
this.name = name;
this.age = age;
}
public void setName(String name){
this.name = name;
}
public String getName(){
return name;
}
public void setAge(int age){
this.age = age;
}
public int getAge(){
return age;
}
}
public class StudentTest {
public static void main(String[] args) {
//创建对象
//调用空参构造
Student s = new Student("zhangsan",18);
/* s.setAge(18);
s.setName("小囧囧");*/
System.out.println(s.getAge());
System.out.println(s.getName());
}
}
如果我们需要键盘录入数据则使用空参构造最好
如果我们给数据使用有参构造最好
不同情况使用不同构造方法
标准javabean类型
下载插件ptg一键生成Javabean类型
这个也可以 点构造函数即可
package Test3;
public class User {
private String username;
private String password;
private String email;
private String gender;
private int age;
public User() {
}
public User(String username, String password, String email, String gender, int age) {
this.username = username;
this.password = password;
this.email = email;
this.gender = gender;
this.age = age;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String toString() {
return "User{username = " + username + ", password = " + password + ", email = " + email + ", gender = " + gender + ", age = " + age + "}";
}
}
package Test3;
public class UserTest {
public static void main(String[] args) {
User u=new User("张三","123","666@qq.com","男",18);
System.out.println(u);
}
}
二个引用指向同一个对象
基本数据类型和引用数据类型
基本数据类型存的是真实值
引用数据类型存的是地址值
成员变量和局部变量区别
面向对象练习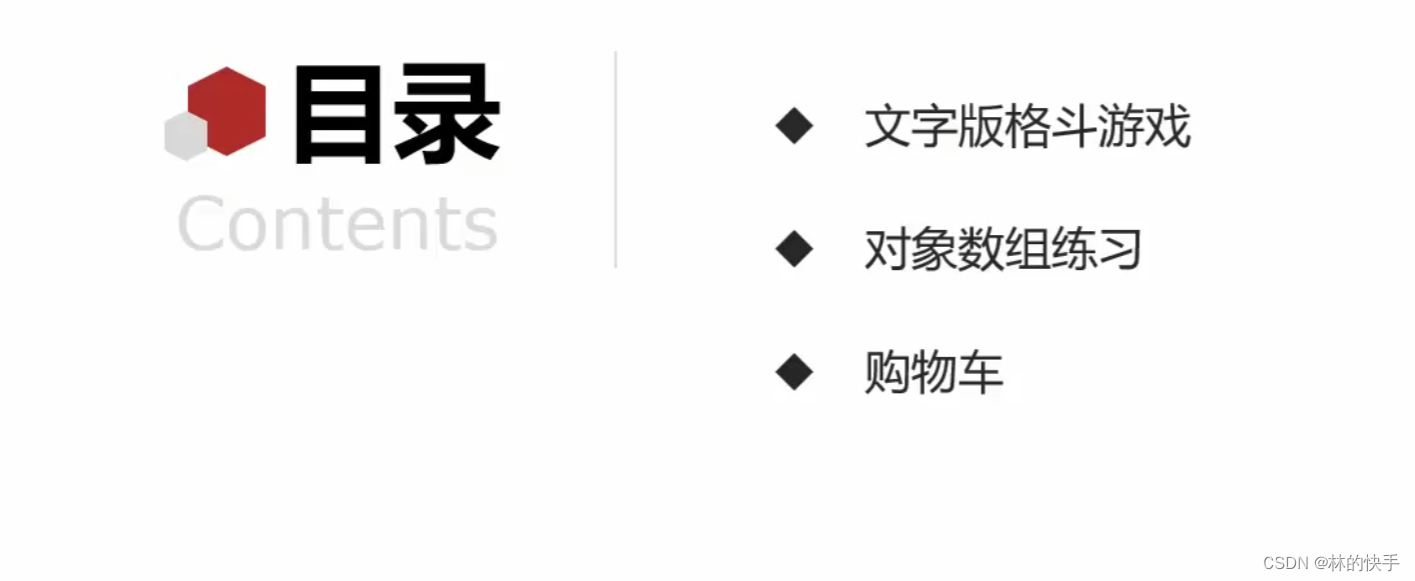
练习1
第一版程序
在这段代码中,this.getName()和 role.getName()都是用来访问对象的方法。
-
this.getName()表示调用attack方法的对象(即攻击者)的名字。在Java中,关键字this代表当前对象的引用,所以this.getName()表示调用当前对象的getName()方法,获取当前对象的名字。
-
role.getName()表示被攻击的对象(即参数中的role)的名字。在Java中,role是attack方法的参数,它是一个Role类型的对象,在这个对象上调用getName()方法,获取被攻击对象的名字。
在这段代码中,Role不是数据类型,而是一个类名。在面向对象的编程中,类是用来描述具有相似属性和行为的对象的模板。在这里,Role类描述了角色的属性(如名字和血量)以及行为(如攻击)。在attack方法中,Role role表示传入的参数是一个Role类的实例,即被攻击的角色是一个Role类型的对象。
import java.util.Random;
public class Role {
private String name;
private int blood;
public Role() {
}
public Role(String name, int blood) {
this.name = name;
this.blood = blood;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getBlood() {
return blood;
}
public void setBlood(int blood) {
this.blood = blood;
}
/*定义一个方法用于攻击别人
谁攻击谁
Role r1=new Role();
Role r2=new Role();
r1.攻击(r2)
方法的调用者攻击参数
*/
public void attack(Role role) {
//计算造成的伤害1~20
Random num = new Random();
int hurt=num.nextInt(20)+1;
//修改一下挨揍人的血量
//剩余血量
int remainboold=role.getBlood()-hurt;
remainboold=remainboold<0?0:remainboold;
//修改一下挨揍人的血量
role.setBlood(remainboold);
System.out.println(this.getName() +
"举起拳头打了"+ role.getName()+"一下,造成了"+hurt+"点伤害"+
"还剩下"+remainboold+"血量");
}
}
public class GameTest {
public static void main(String[] args) {
// 1.创建第一个角色
Role r1=new Role("乔峰",100);
//2.创建第二个角色
Role r2= new Role("鸠摩智",100);
//3.开始格斗,回合制游戏
while (true){
//r1开始攻击r2
r1.attack(r2);
//判断r2的剩余血量
if(r2.getBlood()==0){
System.out.println(r1.getName()+"KO了"+r2.getName());
break;
}
//r2攻击r1
r1.attack(r2);
if(r1.getBlood()==0){
System.out.println(r2.getName()+"KO了"+r1.getName());
break;
}
}
}
}
优化版
public class GameTest {
public static void main(String[] args) {
// 1.创建第一个角色
Role r1 = new Role("乔峰", 100, '男');
//2.创建第二个角色
Role r2 = new Role("鸠摩智", 100, '男');
//展示一下角色信息
r1.showRoleInfo();
r2.showRoleInfo();
//3.开始格斗,回合制游戏
while (true){
//r1开始攻击r2
r1.attack(r2);
//判断r2的剩余血量
if(r2.getBlood()==0){
System.out.println(r1.getName()+"KO了"+r2.getName());
break;
}
//r2攻击r1
r1.attack(r2);
if(r1.getBlood()==0){
System.out.println(r2.getName()+"KO了"+r1.getName());
break;
}
}
}
}
import java.util.Random;
public class Role {
private String name;
private int blood;
private char gender;
private String face;
String[] boyfaces = {"风流俊雅", "气宇轩昂", "相貌英俊", "五官端正", "相貌平平", "一塌糊涂", "面目狰狞"};
String[] girlfaces = {"美奂绝伦", "沉鱼落雁", "婷婷玉立", "身材娇好", "相貌平平", "相貌简陋", "惨不忍睹"};
// attack 攻击描述:
String[] attacks_desc={
"%s使出了一招【背心钉】,转到对方的身后,一掌向%s背心的灵台穴拍去。",
"%s使出了一招【游空探爪】,飞起身形自半空中变掌为抓锁向%s。",
"%s大喝一声,身形下伏,一招【劈雷坠地】,捶向%s双腿。",
"%s运气于掌,一瞬间掌心变得血红,一式【掌心雷】,推向%s。",
"%s阴手翻起阳手跟进,一招【没遮拦】,结结实实的捶向%s。",
"%s上步抢身,招中套招,一招【劈挂连环】,连环攻向%s。"
};
// injured 受伤描述:
String[] injureds_desc={
"结果%s退了半步,毫发无损",
"结果给%s造成一处瘀伤",
"结果一击命中,%s痛得弯下腰",
"结果%s痛苦地闷哼了一声,显然受了点内伤",
"结果%s摇摇晃晃,一跤摔倒在地",
"结果%s脸色一下变得惨白,连退了好几步",
"结果『轰』的一声,%s口中鲜血狂喷而出",
"结果%s一声惨叫,像滩软泥般塌了下去"
};
public Role() {
}
public Role(String name, int blood, char gender) {
this.name = name;
this.blood = blood;
this.gender = gender;
//随机长相
setFace(gender);
}
public Role(String name, int blood, char gender, String face, String[] boyfaces, String[] girlfaces) {
this.name = name;
this.blood = blood;
this.gender = gender;
this.face = face;
this.boyfaces = boyfaces;
this.girlfaces = girlfaces;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getBlood() {
return blood;
}
public void setBlood(int blood) {
this.blood = blood;
}
/*定义一个方法用于攻击别人
谁攻击谁
Role r1=new Role();
Role r2=new Role();
r1.攻击(r2)
方法的调用者攻击参数
*/
public void attack(Role role) {
Random r = new Random();
int index = r.nextInt(attacks_desc.length);
String KungFu=attacks_desc[index];
//输出一个攻击效果
System.out.printf(KungFu,this.getName(),role.getName());
//计算造成的伤害1~20
int hurt = r.nextInt(20) + 1;
//修改一下挨揍人的血量
//剩余血量
int remainboold = role.getBlood() - hurt;
remainboold = remainboold < 0 ? 0 : remainboold;
//修改一下挨揍人的血量
role.setBlood(remainboold);
/*
受伤的描述
血量>90 0索引的描述
80~90 1索引的描述
70~80 2索引的描述
60~70 3索引的描述
40~60 4索引的描述
20~50 5索引的描述
10~20 6索引的描述
小于10的 7索引的描述
*/
if(remainboold >90){
System.out.printf(injureds_desc[0],role.getName());
}else if(remainboold >80 && remainboold <=90){
System.out.printf(injureds_desc[1],role.getName());
}else if(remainboold >70 && remainboold <=80){
System.out.printf(injureds_desc[2],role.getName());
}else if(remainboold >60 && remainboold <=70){
System.out.printf(injureds_desc[3],role.getName());
}else if(remainboold >40 && remainboold <=60){
System.out.printf(injureds_desc[4],role.getName());
}else if(remainboold >20 && remainboold <=50){
System.out.printf(injureds_desc[5],role.getName());
}else if(remainboold >10 && remainboold <=20){
System.out.printf(injureds_desc[6],role.getName());
}
else
{
System.out.printf(injureds_desc[7],role.getName());
}
System.out.println();
}
public char getGender() {
return gender;
}
public void setGender(char gender) {
this.gender = gender;
}
public String getFace() {
return face;
}
public void setFace(char gender) {
Random r = new Random();
//长相是随机的
if (gender == '男') {
//从boyfaces里面随机
int index = r.nextInt(boyfaces.length);
this.face = boyfaces[index];
} else if (gender == '女') {
//从girlfaces里面随机
int index = r.nextInt(girlfaces.length);
this.face = girlfaces[index];
} else {
this.face = "面目狰狞";
}
}
public String[] getBoyfaces() {
return boyfaces;
}
public void setBoyfaces(String[] boyfaces) {
this.boyfaces = boyfaces;
}
public String[] getGirlfaces() {
return girlfaces;
}
public void setGirlfaces(String[] girlfaces) {
this.girlfaces = girlfaces;
}
public void showRoleInfo() {
System.out.println("姓名为" + getName());
System.out.println("姓别为" + getGender());
System.out.println("血量为" + getBlood());
System.out.println("颜值为" + getFace());
}
}
练习2
ctr+p查参数
package Tets;
public class Goods {
private String id;
private String name;
private double price;
private int count;
public Goods() {
}
public Goods(String id, String name, double price, int count) {
this.id = id;
this.name = name;
this.price = price;
this.count = count;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
}
package Tets;
public class GoodTest {
public static void main(String[] args) {
//1.创建一个数组
Goods[]arr=new Goods[3];
//2.创建3个商品对象
Goods g1=new Goods("001","p40",5999.0,100);
Goods g2=new Goods("002","p50",6999.0,200);
Goods g3=new Goods("003","p60",7999.0,500);
//3. 把商品添加到数组中
arr[0]=g1;
arr[1]=g2;
arr[2]=g3;
for (int i = 0; i < arr.length; i++) {
Goods g=arr[i];
System.out.println(g.getId()+","+g.getName()+","+g.getPrice()+","+g.getCount());
}
}
}
键盘录入
nextLine遇到空格不停止
练习3
string
package com.ithema;
import java.util.Random;
public class StringDemo01 {
public static void main(String[] args) {
//1.使用直接赋值的方式获取一个字符串对象
String s1="abc";
System.out.println(s1);
//2.使用new的方式来获取一个字符串对象
//空参构造:可以获取一个空白字符串对象
String s2=new String();
System.out.println('*'+s2+'!');
//传递一个字符串,根据传递的字符串内容再创建一个新的字符串对象
String s3=new String("abc");
System.out.println(s3);
//传递一个字符数组,根据字符数组的内容再创建一个新的字符串对象
//需求:我们修改字符串的内容 abc Qbc
char []arr={'a','b','c'};
String s4=new String(arr);
System.out.println(s4);
//传递一个字节数组,根据字节数组的内容再创建一个新的字符串对象
byte []bytes={97,98,99};
String s5=new String(bytes);
System.out.println(s5);
}
}
引用数据类型 s1!=s2 因为new开辟不同的空间