反转链表
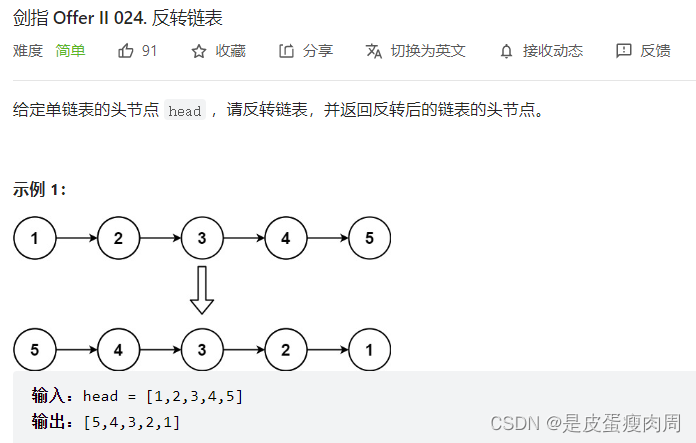
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode* pre=NULL;
ListNode* next=NULL;
ListNode* cur=head;
while(cur!=NULL){
next=cur->next;
cur->next=pre;
pre=cur;
cur=next;
}
return pre;
}
};
删除链表的结点
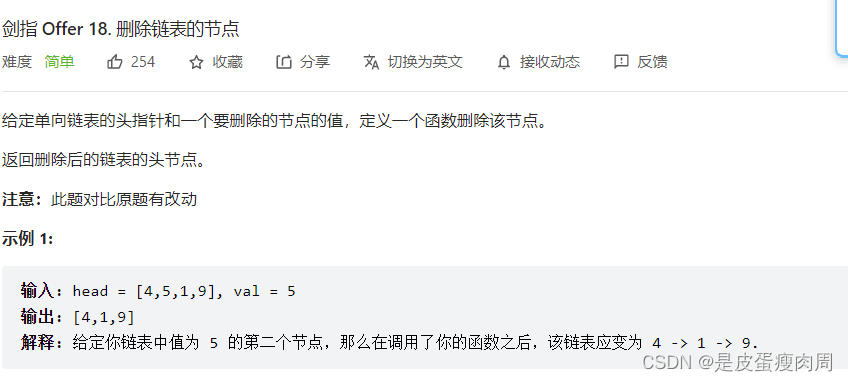
class Solution {
public:
ListNode* deleteNode(ListNode* head, int val) {
if(head->val==val) return head->next;
ListNode* p=head;
while(p!=NULL){
if((p->next)->val==val){
break;
}
p=p->next;
}
if(p->next!=NULL){
p->next=(p->next)->next;
}
return head;
}
};
返回倒数第k个节点
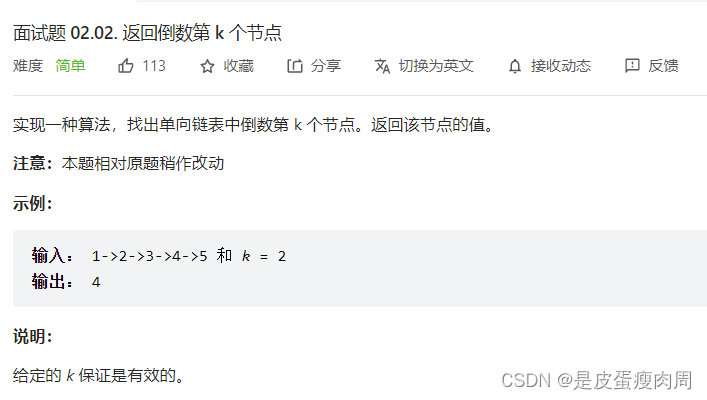
class Solution {
public:
int kthToLast(ListNode* head, int k) {
ListNode* pre=NULL;
ListNode* next=NULL;
ListNode* cur=head;
while(cur!=NULL){
next=cur->next;
cur->next=pre;
pre=cur;
cur=next;
}
ListNode* p=pre;
int i=1;
while(p!=NULL){
if(i==k){
break;
}
p=p->next;
i++;
}
return p->val;
}
};
删除链表中的重复项
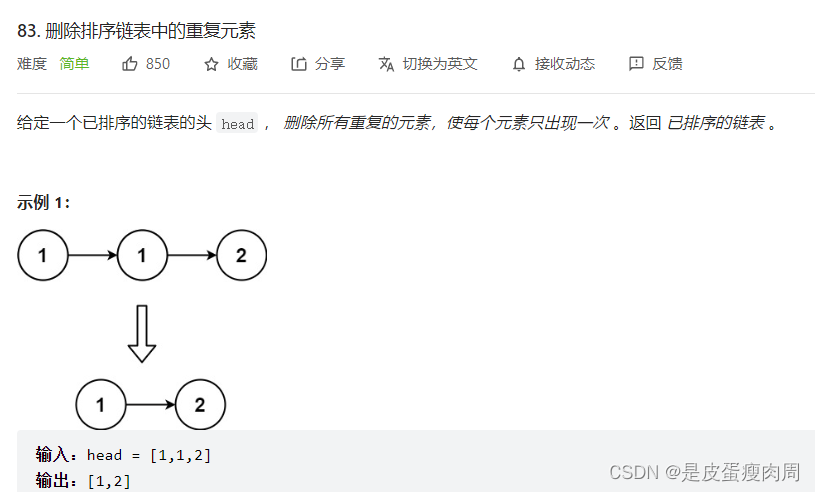
class Solution {
public:
ListNode* deleteDuplicates(ListNode* head) {
ListNode* p=head;
while(p!=NULL&&p->next!=NULL){
if((p->val)==(p->next)->val){
p->next=(p->next)->next;
p=p;
}else p=p->next;
}
return head;
}
};