1. 递归
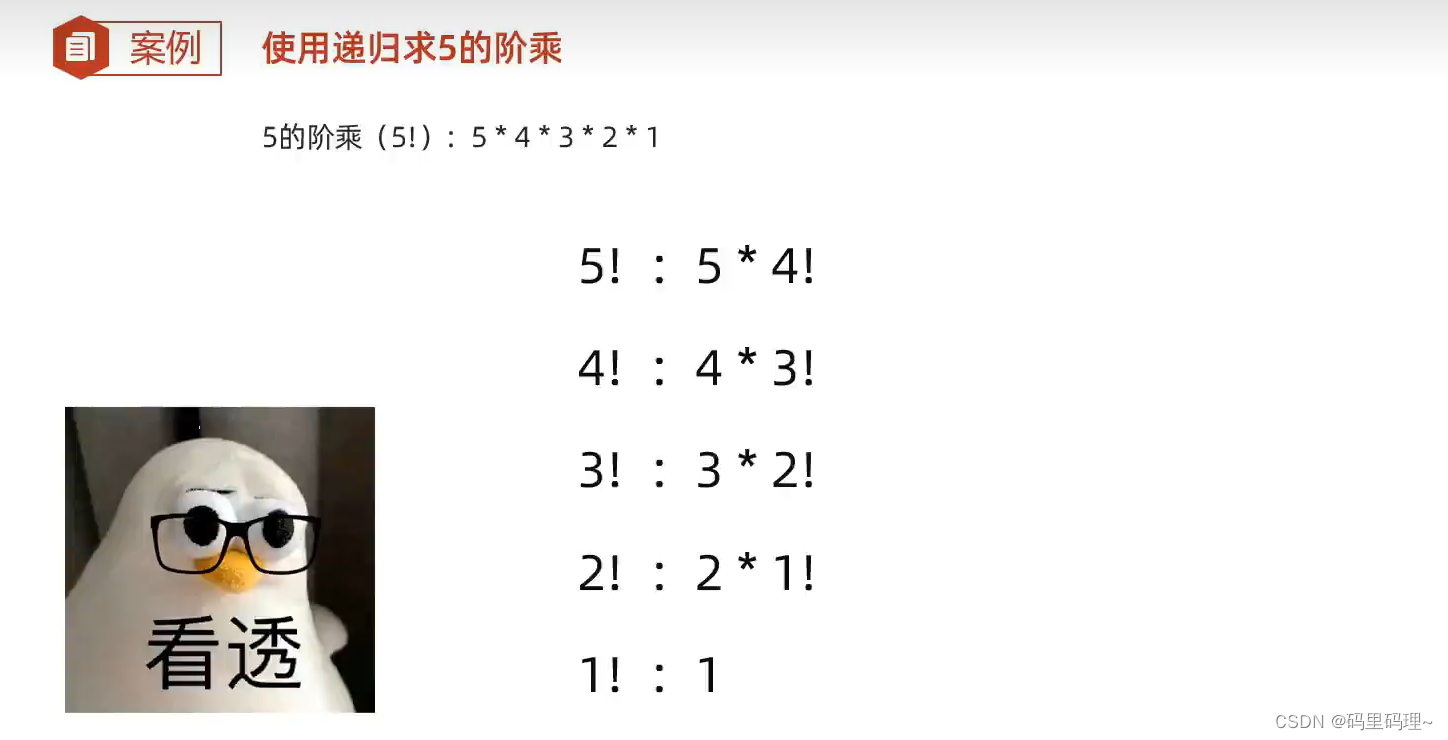
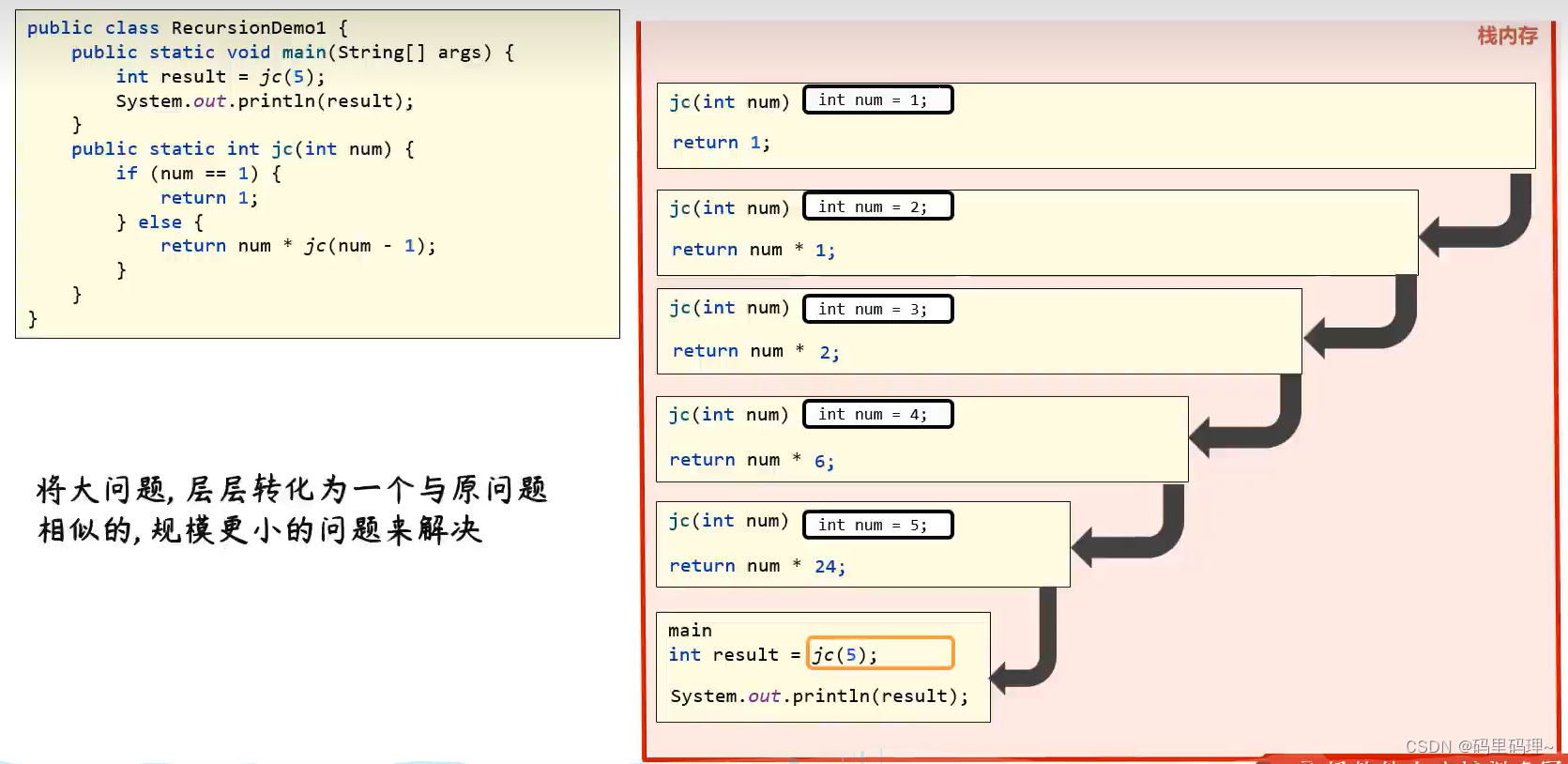
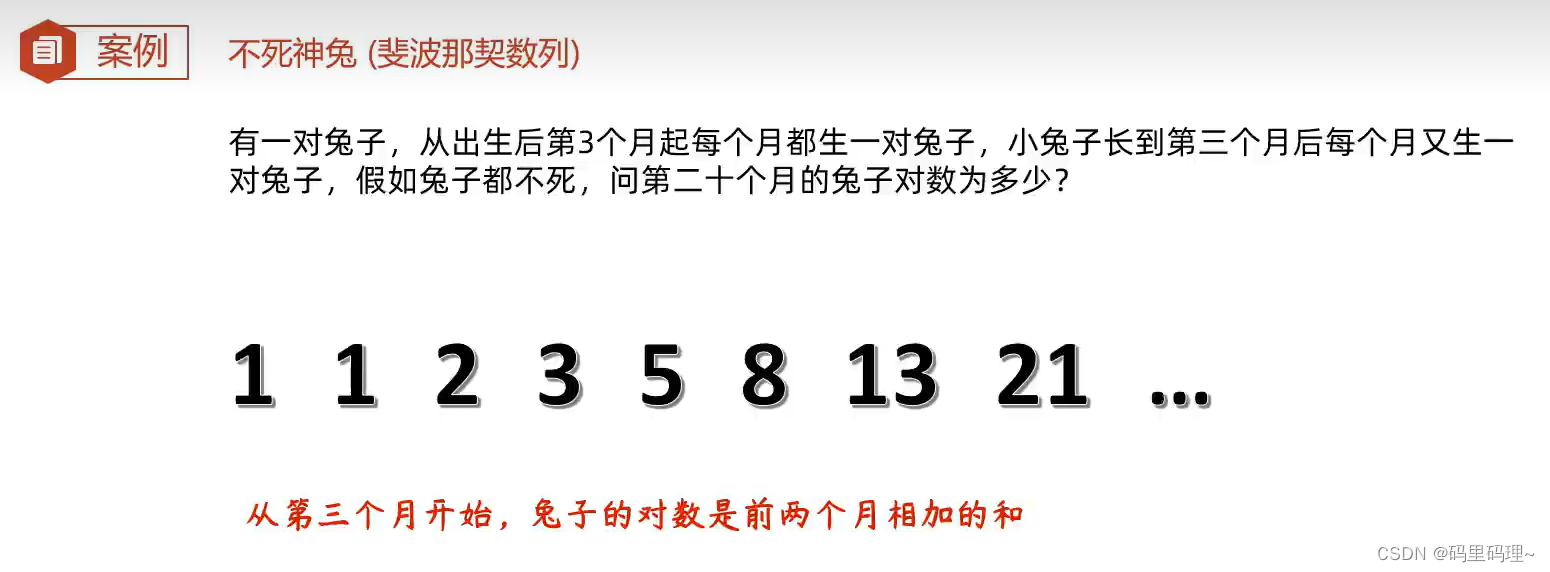
package com.itheima.recursion;
public class RecursionDemo3 {
/*
不死神兔(斐波那契额数列)
*/
public static void main(String[] args) {
int sum = getSum(20);
System.out.println(sum);
}
public static int getSum(int n) {
if (n == 1 || n == 2) {
return 1;
} else {
return getSum(n - 2) + getSum(n - 1);
}
}
}

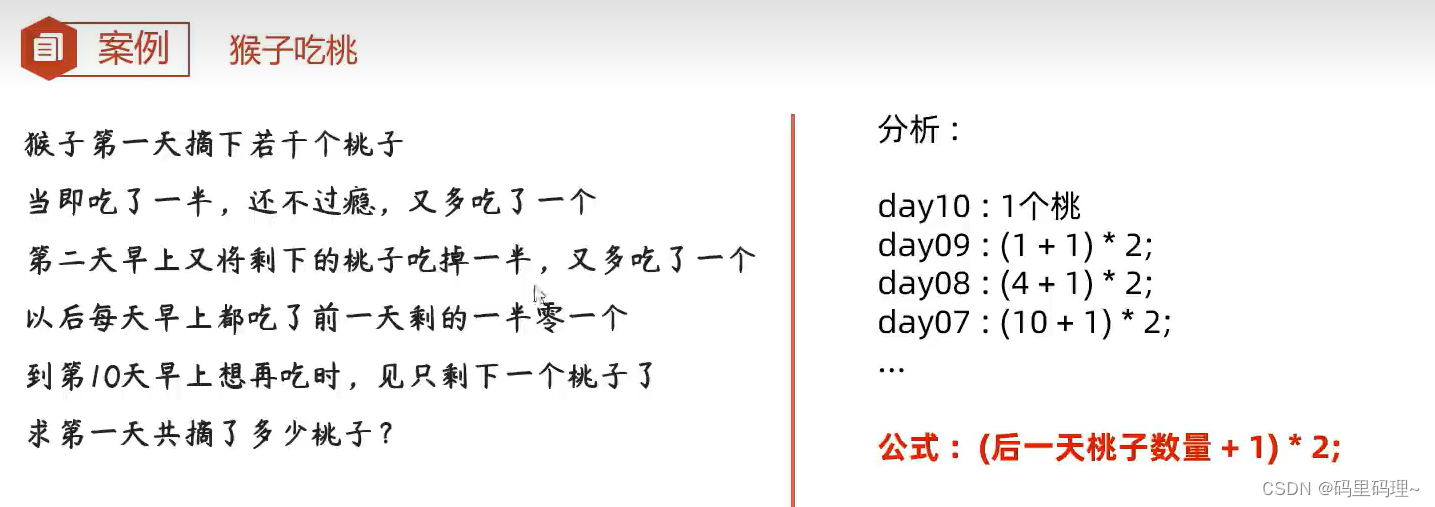
package com.itheima.recursion;
public class RecursionDemo4 {
/*
猴子吃桃
*/
public static void main(String[] args) {
int sum = monkey(1);
System.out.println(sum);
}
public static int monkey(int day) {
if (day == 10){
return 1;
}else{
return (monkey(day + 1) + 1) * 2;
}
}
}

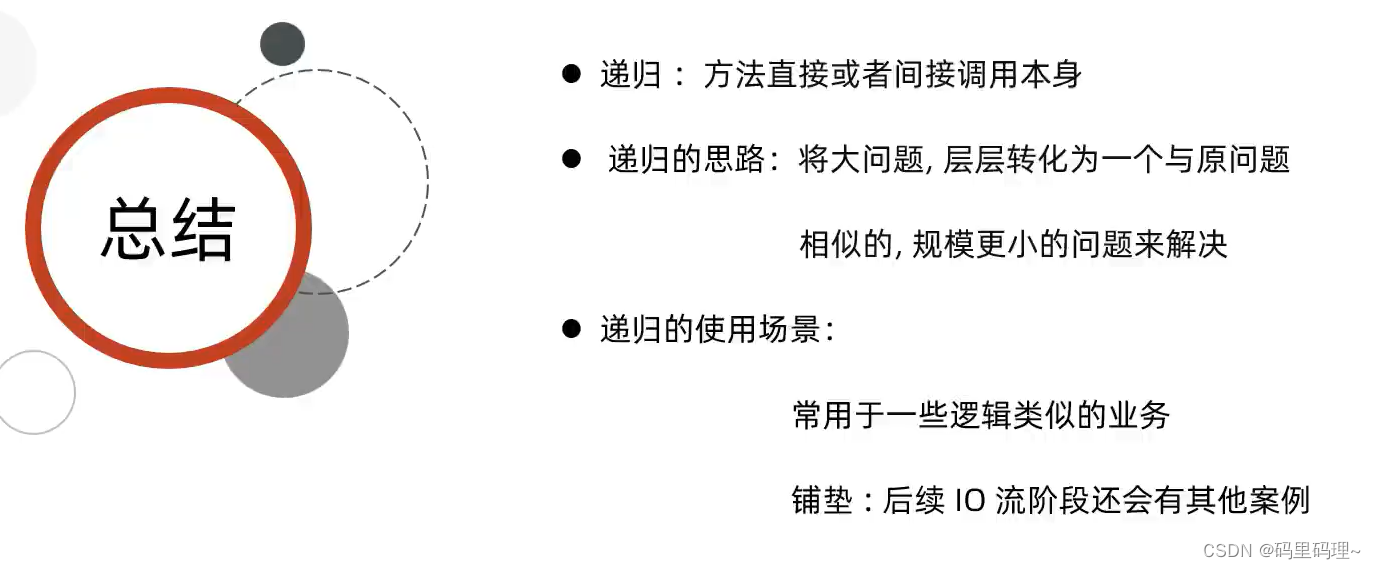
2. 异常
2.1 异常介绍
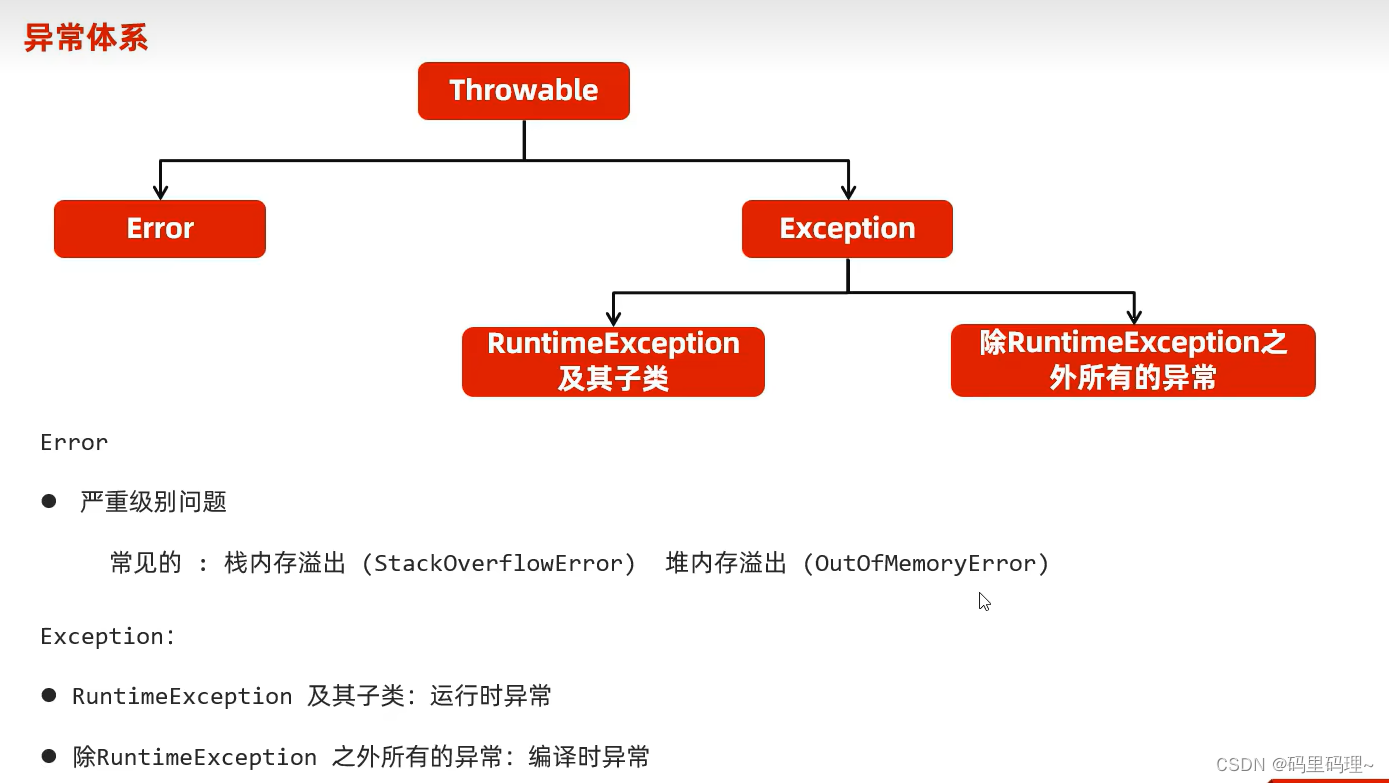
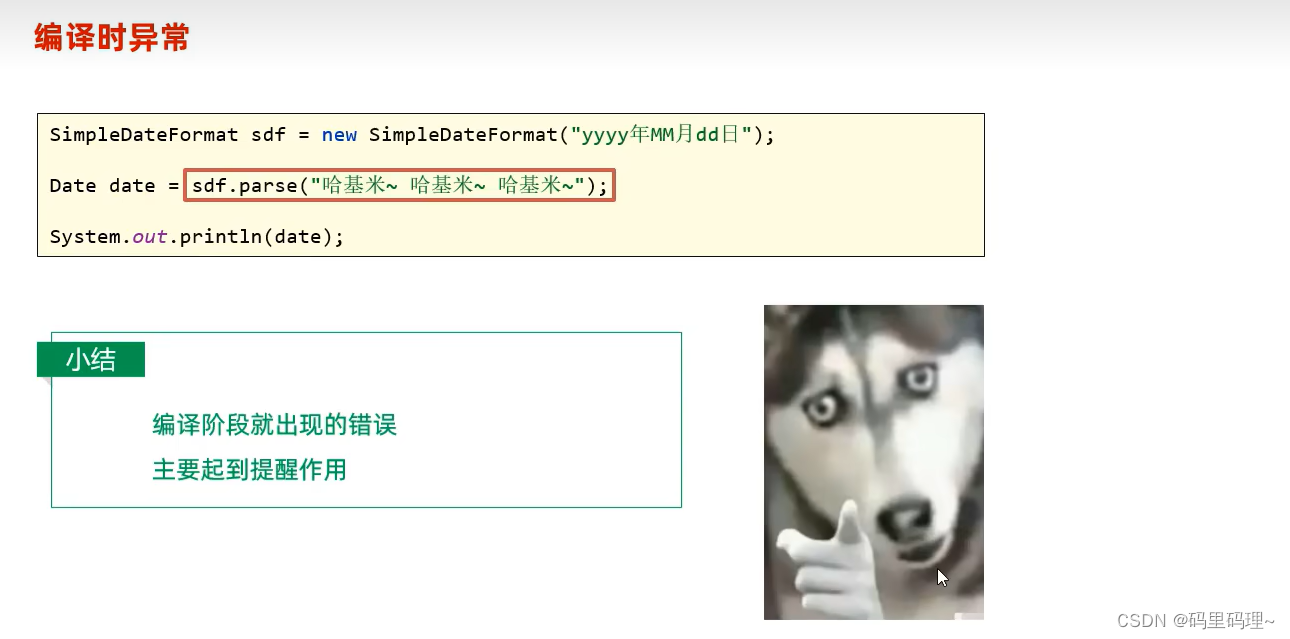
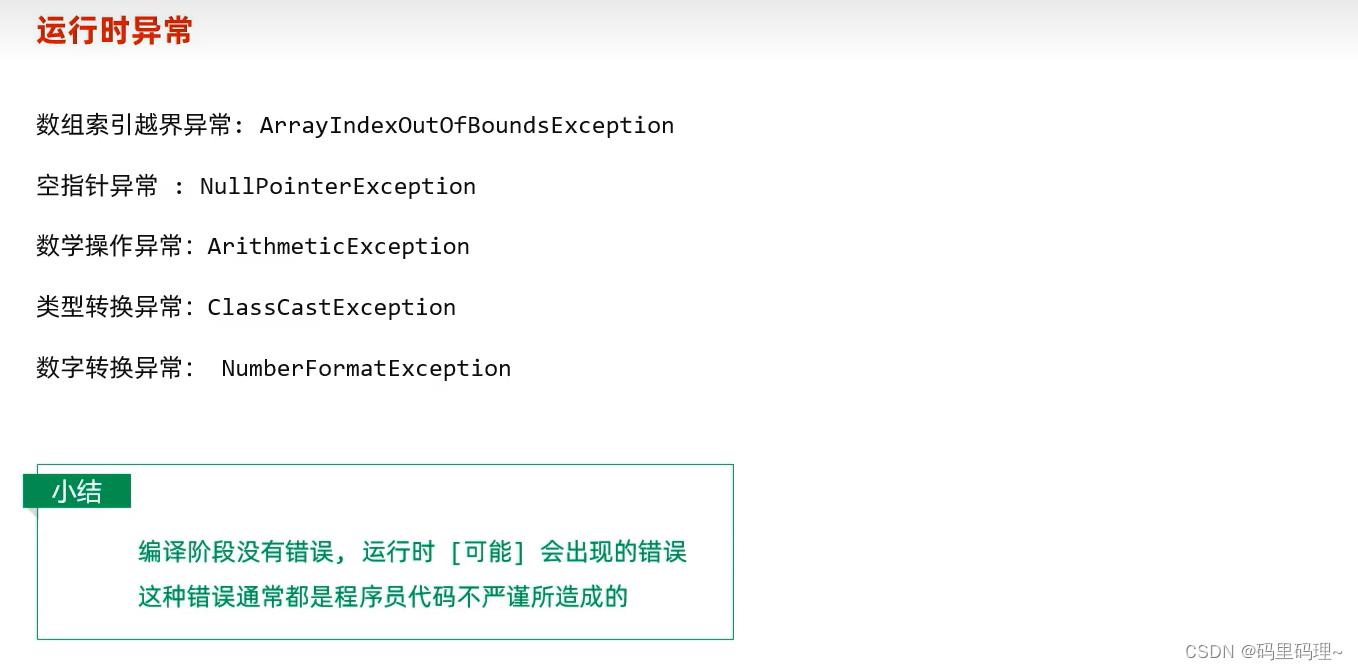
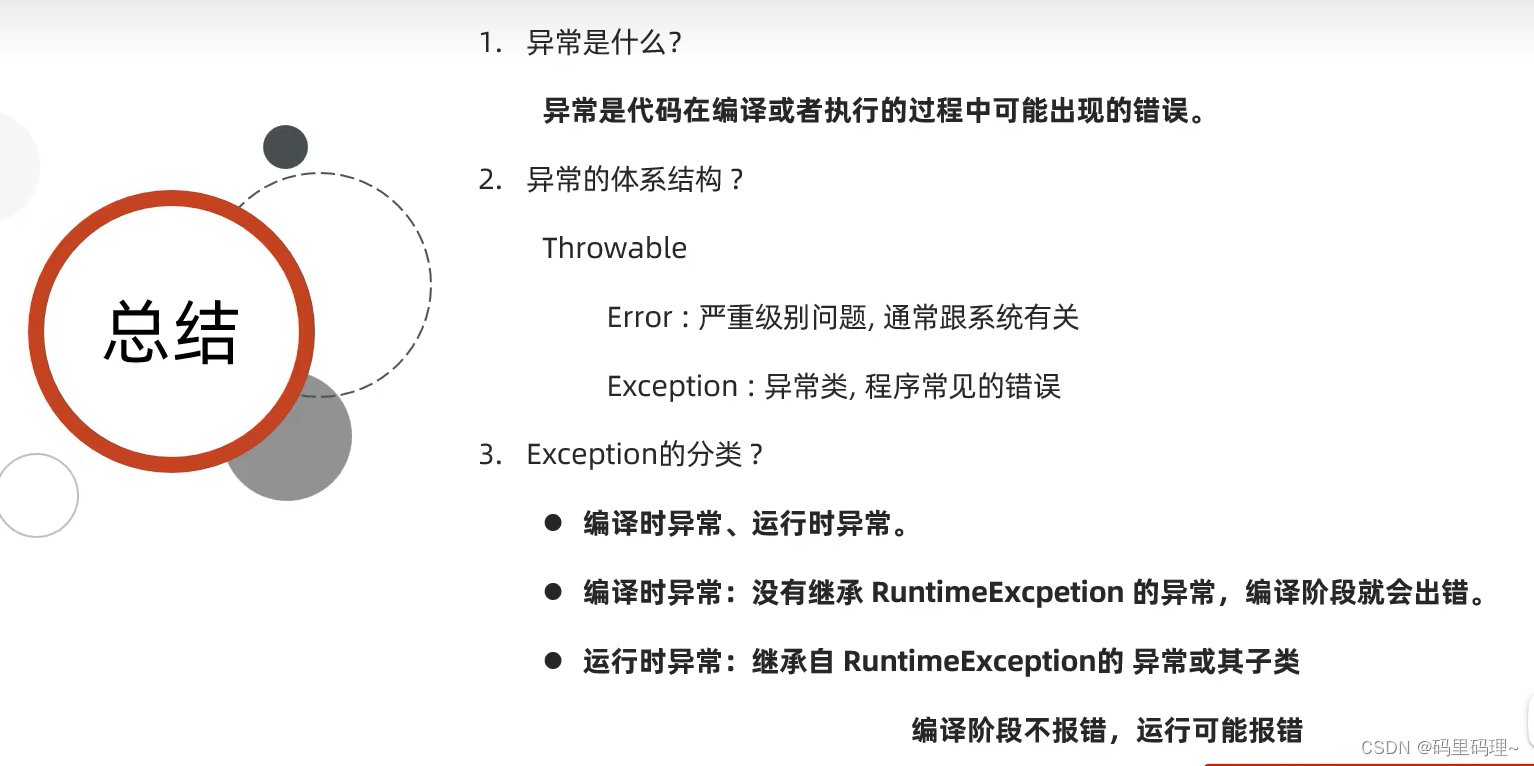
2.2 异常的处理方式
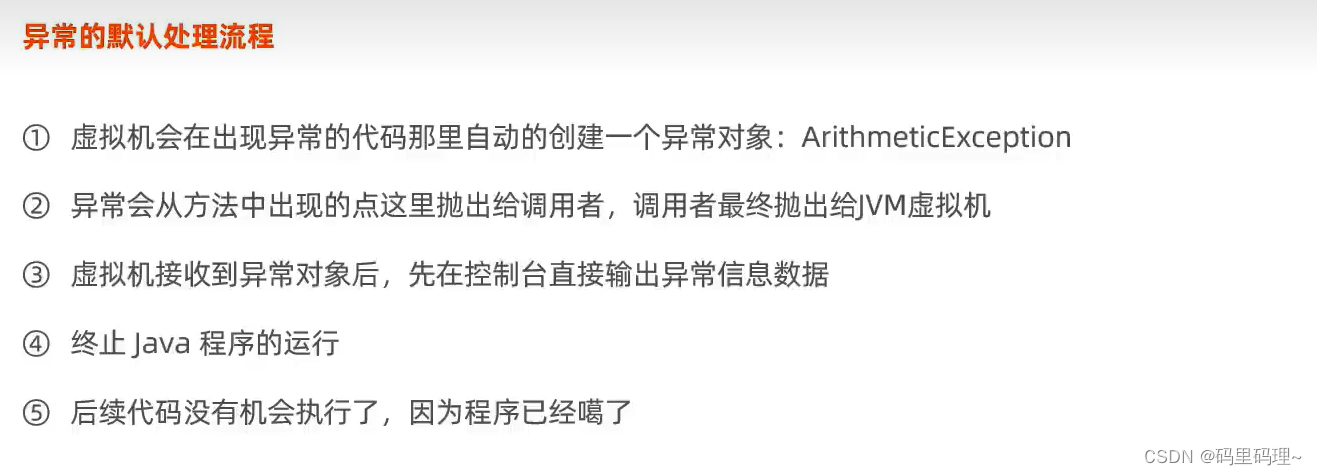
2.2.1 try...catch.. && throws
package com.itheima.exception.handle;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class HandleExceptionDemo {
/*
异常的处理方式 :
1. try...catch捕获异常
好处: 异常对象可以被捕获, 后续的代码可以继续执行
格式:
try {
可能会出现异常的代码
} catch (异常名称 对象名) {
异常的处理方案
}
执行流程:
1. 执行 try {} 中的代码, 看是否有异常对象产生
2. 没有 : catch就不会捕获, 后续代码继续执行
3. 有 : catch捕获异常对象, 执行catch {} 中的处理方案, 后续代码继续执行
2. throws 抛出异常
----------------------------------------------------
问题: 正在面临的异常, 是否需要暴露出来
- 不需要暴露 : try...catch捕获
- 需要暴露 : 抛出异常
*/
public static void main(String[] args) throws Exception {
tryCatchDemo();
}
public static void method() throws ParseException, FileNotFoundException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日");
Date date = sdf.parse("abc");
System.out.println(date);
FileReader fr = new FileReader("D:\\A.txt");
}
private static void tryCatchDemo() {
System.out.println("开始");
try {
int[] arr = null;
System.out.println(arr[10]);
System.out.println(10 / 0);
} catch (ArithmeticException e) { // ArithmeticException e = new ArithmeticException();
System.out.println("捕获了运算异常");
} catch (NullPointerException e) { // NullPointerException e = new NullPointerException();
System.out.println("捕获了空指针异常");
} catch (Exception e) {
System.out.println("捕获了异常");
}
System.out.println("结束");
}
}
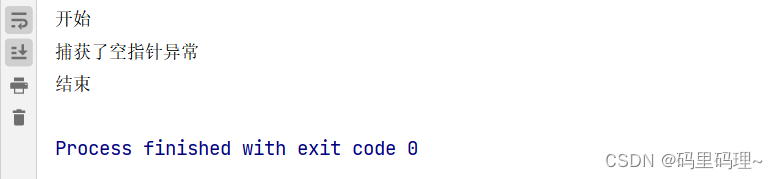
2.2.2 案例
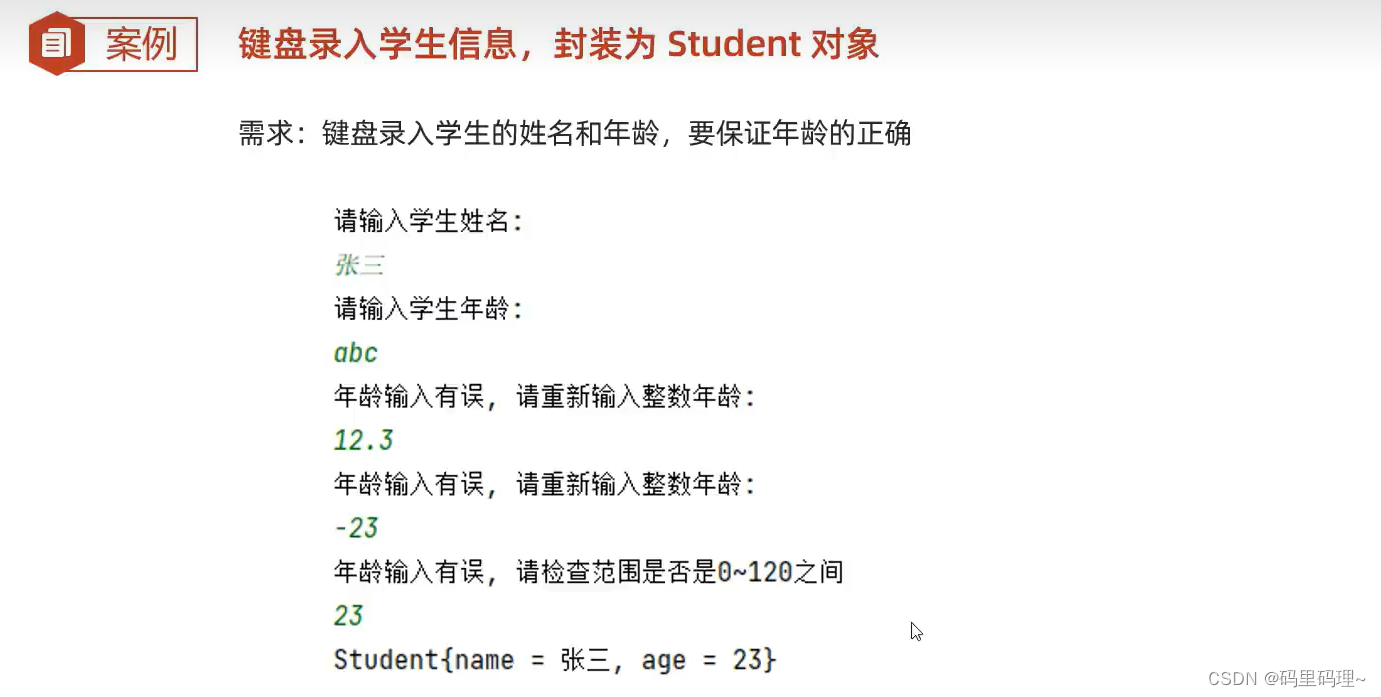
package com.itheima.exception;
public class StudentAgeException extends RuntimeException {
public StudentAgeException() {
}
public StudentAgeException(String message) {
super(message);
}
}
package com.itheima.domain;
import com.itheima.exception.StudentAgeException;
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
setAge(age);
}
/**
* 获取
*
* @return name
*/
public String getName() {
return name;
}
/**
* 设置
*
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* 获取
*
* @return age
*/
public int getAge() {
return age;
}
/**
* 设置
*
* @param age
*/
public void setAge(int age) {
if(age >= 0 && age <= 120){
this.age = age;
} else {
// 错误的年龄
throw new StudentAgeException("年龄范围有误, 需要0~120之间的年龄");
}
}
public String toString() {
return "Student{name = " + name + ", age = " + age + "}";
}
}
package com.itheima.test;
import com.itheima.domain.Student;
import com.itheima.exception.StudentAgeException;
import java.util.Scanner;
public class TryCatchTest {
/*
需求: 键盘录入学生的姓名和年龄, 封装为Student学生对象
*/
public static void main(String[] args) {
Student stu = new Student();
Scanner sc = new Scanner(System.in);
System.out.println("请输入学生姓名: ");
String name = sc.nextLine();
stu.setName(name);
System.out.println("请输入学生年龄: ");
int age = 0;
while (true) {
try {
age = Integer.parseInt(sc.nextLine());
stu.setAge(age);
break;
} catch (NumberFormatException e) {
System.out.println("年龄输入有误, 请重新输入整数年龄: ");
} catch (StudentAgeException e) { // StudentAgeException e = new StudentAgeException("年龄范围有误, 需要0~120之间的年龄");
String message = e.getMessage();
System.out.println(message);
}
}
System.out.println(stu);
}
}
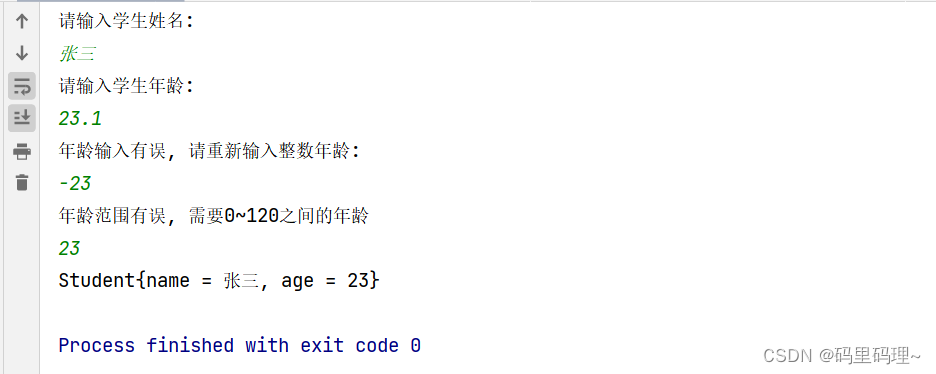
package com.itheima.exception;
public class ThrowableMethodDemo {
/*
Throwable常用方法 :
public String getMessage() 获取异常的错误原因
public void printStackTrace() 展示完整的异常错误信息
*/
public static void main(String[] args) {
System.out.println("开始");
try {
System.out.println(10 / 0);
} catch (ArithmeticException e) {
String message = e.getMessage();
System.out.println(message);
}
System.out.println("结束");
}
}
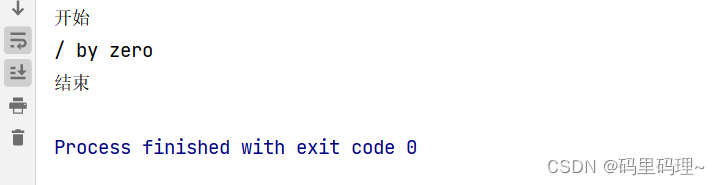
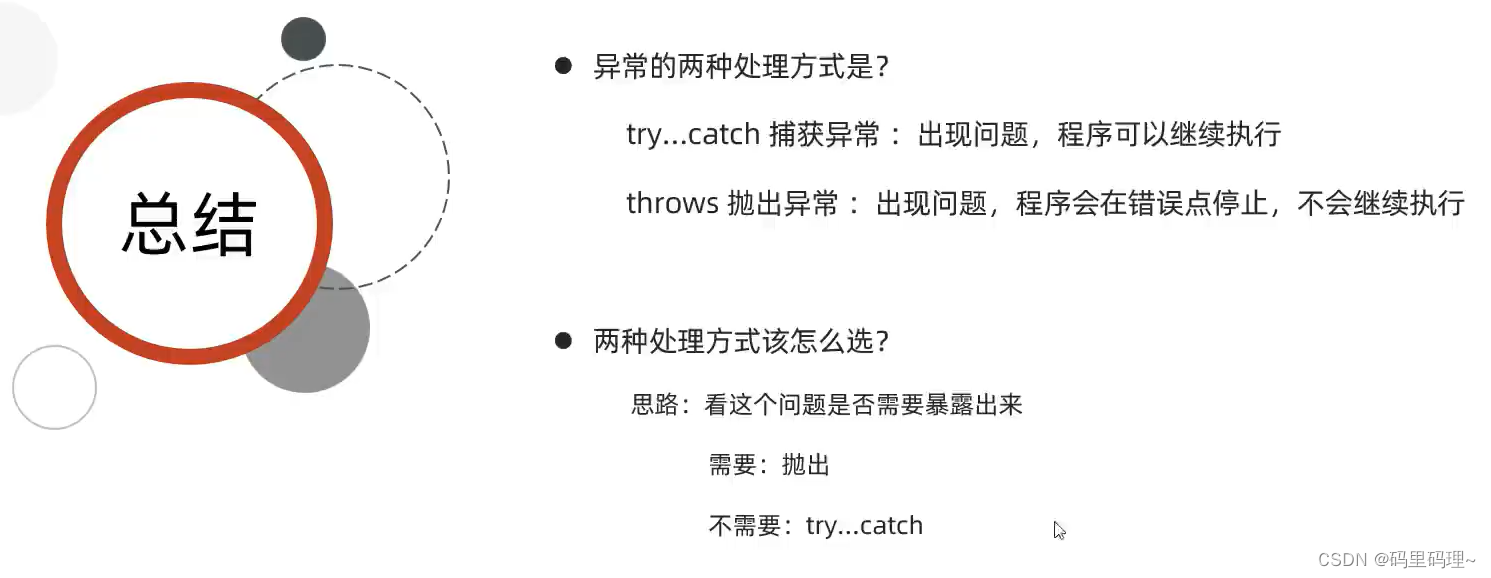
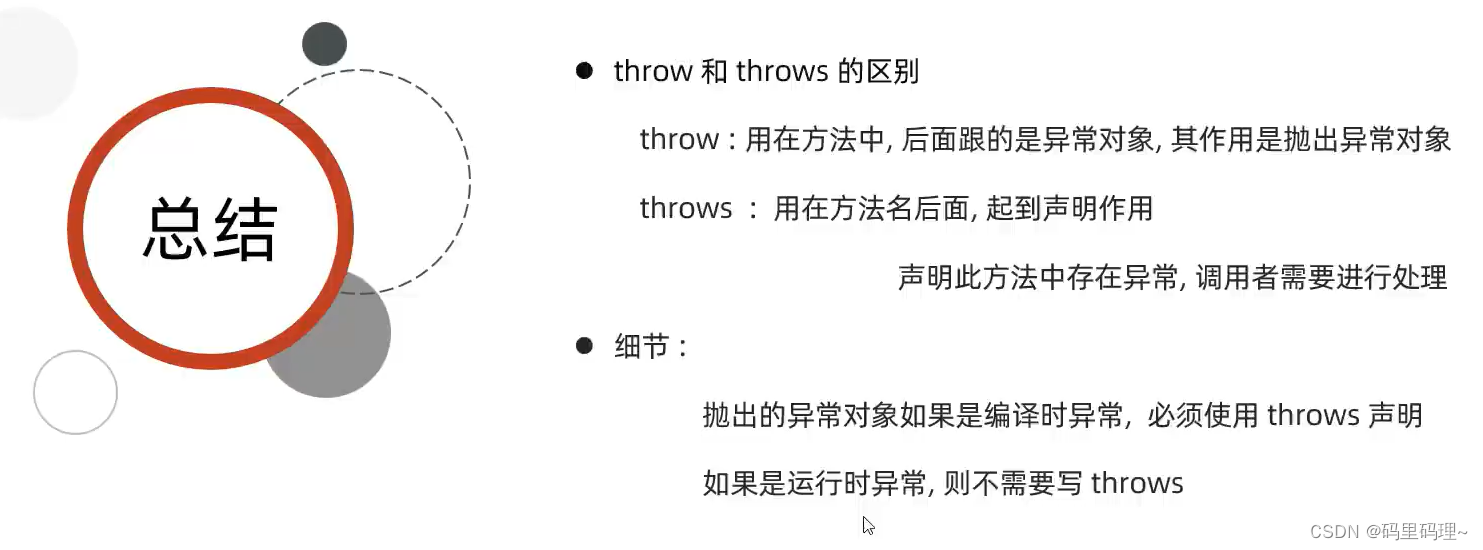
2.3 自定义异常
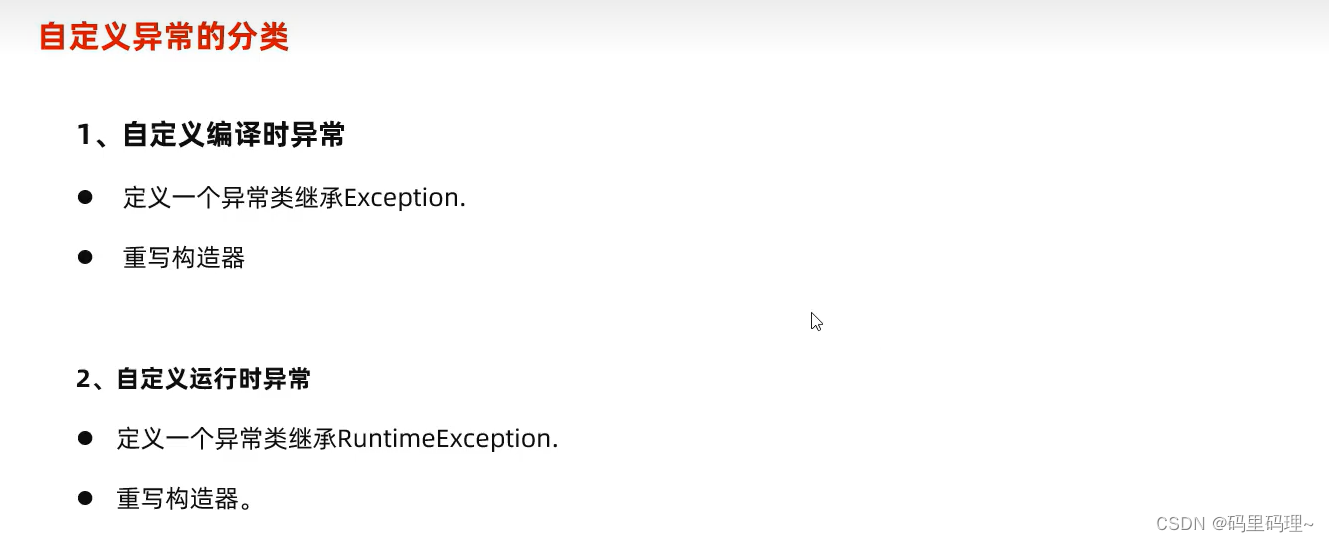
package com.itheima.exception;
public class StudentAgeException extends RuntimeException {
public StudentAgeException() {
}
public StudentAgeException(String message) {
super(message);
}
}
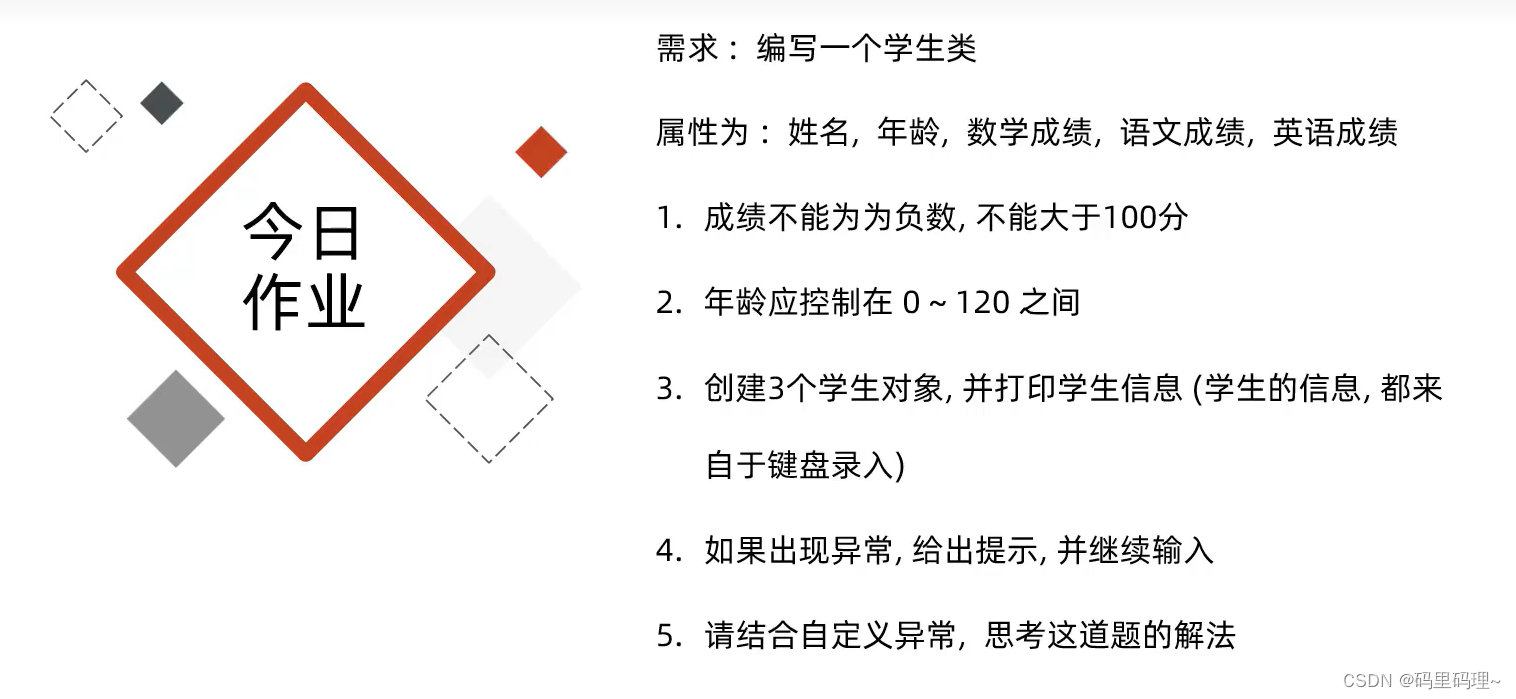
package com.itheima.homework;
public class StudentAgeException extends RuntimeException{
public StudentAgeException() {
}
public StudentAgeException(String message) {
super(message);
}
}
package com.itheima.homework;
public class StudentScoreException extends RuntimeException{
public StudentScoreException() {
}
public StudentScoreException(String message) {
super(message);
}
}
package com.itheima.homework;
public class Student {
private String name;
private int age;
private int mathScore;
private int chineseScore;
private int englishScore;
public Student() {
}
public Student(String name, int age, int mathScore, int chineseScore, int englishScore) {
this.name = name;
this.age = age;
this.mathScore = mathScore;
this.chineseScore = chineseScore;
this.englishScore = englishScore;
}
/**
* 获取
*
* @return name
*/
public String getName() {
return name;
}
/**
* 设置
*
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* 获取
*
* @return age
*/
public int getAge() {
return age;
}
/**
* 设置
*
* @param age
*/
public void setAge(int age) {
if (age >= 0 && age <= 120) {
this.age = age;
} else {
throw new StudentAgeException("您输入的年龄有误, 请输入0~120之间的年龄");
}
}
/**
* 获取
*
* @return mathScore
*/
public int getMathScore() {
return mathScore;
}
/**
* 设置
*
* @param mathScore
*/
public void setMathScore(int mathScore) {
this.mathScore = setScore(mathScore);
}
/**
* 获取
*
* @return chineseScore
* @param i
*/
public int getChineseScore(int i) {
return chineseScore;
}
/**
* 设置
*
* @param chineseScore
*/
public void setChineseScore(int chineseScore) {
this.chineseScore = setScore(chineseScore);
}
/**
* 获取
*
* @return englishScore
*/
public int getEnglishScore() {
return englishScore;
}
/**
* 设置
*
* @param englishScore
*/
public void setEnglishScore(int englishScore) {
this.englishScore = setScore(englishScore);
}
public int setScore(int score) {
if (score >= 0 && score <= 100) {
return score;
} else {
throw new StudentScoreException("您输入的成绩有误, 请输入0~100之间的分数");
}
}
public String toString() {
return "Student{name = " + name + ", age = " + age + ", mathScore = " + mathScore + ", chineseScore = " + chineseScore + ", englishScore = " + englishScore + "}";
}
}
package com.itheima.homework;
import java.util.ArrayList;
import java.util.Scanner;
public class TestException {
public static void main(String[] args) {
ArrayList<Student> list = new ArrayList<>();
for (int i = 0; i < 3; i++) {
Student stu = new Student();
list.add(stu);
}
Scanner sc = new Scanner(System.in);
for (int i = 0; i < 3; i++) {
Student stu = list.get(i);
System.out.println("请输入第" + (i + 1) + "名学生的姓名:");
stu.setName(sc.nextLine());
// 捕捉年龄输入的异常
while (true) {
try {
System.out.println("请输入第" + (i + 1) + "名学生的年龄:");
stu.setAge(Integer.parseInt(sc.nextLine()));
break;
} catch (StudentAgeException e) {
System.out.println(e.getMessage());
} catch (NumberFormatException e) {
System.out.println("您输入的年龄有误, 请重新输入整数的年龄");
}
}
// 捕捉输入数学成绩的异常
while (true) {
try {
System.out.println("请输入第" + (i + 1) + "名学生的数学成绩:");
stu.setMathScore(Integer.parseInt(sc.nextLine()));
break;
} catch (StudentScoreException e) {
System.out.println(e.getMessage());
} catch (NumberFormatException e) {
System.out.println("您输入的成绩有误, 请重新输入整数成绩");
}
}
// 捕捉输入语文成绩的异常
while (true) {
try {
System.out.println("请输入第" + (i + 1) + "名学生语文成绩:");
stu.setChineseScore(Integer.parseInt(sc.nextLine()));
break;
} catch (StudentScoreException e) {
System.out.println(e.getMessage());
} catch (NumberFormatException e) {
System.out.println("您输入的成绩有误, 请重新输入整数成绩");
}
}
// 捕捉输入英语成绩的异常
while (true) {
try {
System.out.println("请输入第" + (i + 1) + "名学生的英语成绩:");
stu.setEnglishScore(Integer.parseInt(sc.nextLine()));
break;
} catch (StudentScoreException e) {
System.out.println(e.getMessage());
} catch (NumberFormatException e) {
System.out.println("您输入的成绩有误, 请重新输入整数成绩");
}
}
}
for (int i = 0; i < 3; i++) {
Student stu = list.get(i);
System.out.println("第" + (i + 1) + "个学生的信息为:" + stu);
}
}
}
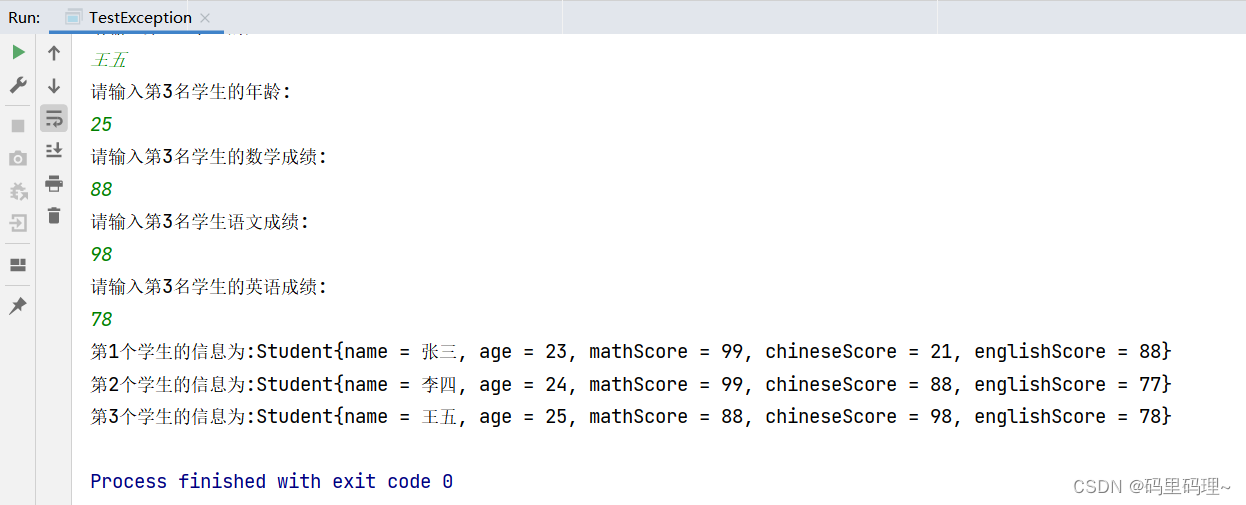