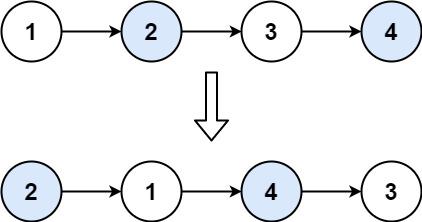
刚学到这里时,看似很简单,其实很容易混乱;
步骤大约为:
先创造一个虚拟的头节点然后透过空的头节点的next指针将交换的节点固定;
不一定非要用节点是否指向空来作为循环条件(我经常在创造列表时候,将列表长度储存并以此来判定是否循环);
分别用两个指针指向空的头节点和他连接的下一个节点(类似头插法的用法)
在循环中开辟新空间储存节点位置(如图1的“3”的位置)然后先用空的头节点链接2,2再反指向1,最后再用1指向储存的3;(叙事有点混乱)(永远都是0,1位置的指针来交换1,2位置的节点)
#include <iostream>
#include <cstdio>
#include <cstdlib>
#include <algorithm>
#include <cmath>
#include <cstring>
#include <vector>
using namespace std;
int len;
struct ListNode
{
int val;
ListNode *next;
ListNode() : val(0), next(NULL) {}
ListNode(int x) : val(x), next(NULL) {}
ListNode(int x, ListNode *next) : val(x), next(next) {}
};
ListNode *createByTail()
{
ListNode *head;
ListNode *p1,*p2;
int n=0;
int num;
cin>>len;
head=NULL;
while(n<len && cin>>num)
{
p1=new ListNode(num);
n=n+1;
if(n==1)
head=p1;
else
p2->next=p1;
p2=p1;
}
return head;
}
ListNode *creatcircle(int n)
{
ListNode *head;
ListNode *p1,*p2;
for(int i=1;i<=n;i++)
{
p1=new ListNode(i);
if(i==1) head=p1;
else p2->next=p1;
p2=p1;
}
p2->next=head;
return head;
}
void gyf(int len,int n,ListNode *head)
{
ListNode *p;p=head;
if(n==1)
{
cout<<p->val;p=p->next;
for(int i=1;i<len;i++)
{
cout<<' '<<p->val;p=p->next;
}
cout<<endl;
}
else
{
for(int j=0;j<len-1;j++)
{
for(int i=1;i<=n-2;i++)
{
p=p->next;
}
cout<<p->next->val<<' ';
p->next=p->next->next;p=p->next;
}
cout<<p->val<<endl;
}
}
void display(ListNode *head)
{
ListNode *p;p=head;
cout<<"head";
for(int i=0;i<len;i++)
{
cout<<"-->"<<p->val;
p=p->next;
}
cout<<"-->tail";
}
class Solution
{
public:
ListNode *swappairs(ListNode *head)
{
ListNode *_head=new ListNode(0);
_head->next=head;
ListNode *p1,*p2;
p1=_head;
p2=head;
for(int i=0;i<len/2;i++)
{
ListNode *temp;
temp=p2->next->next;
p1->next=p2->next;
p2->next->next=p2;
p2->next=temp;
p1=p2;
p2=p1->next;
}
return _head->next;
}
};
int main()
{
ListNode *head=createByTail();
ListNode *head1=Solution().swappairs(head);
display(head1);
return 0;
}