文章目录
study_day02-Python程序设计基础
1.1 基本数据类型——元组(tuple)
首先定义与列表相似,只不过将 [] 改为 ()
- 元组的元素是不可以被修改的
tuples = ('Alex','Alpha','Beta')
lists = ['Alex', 'Alpha', 'Beta']
print(tuples.index('Alex')) # 查找指定元素索引
print(tuples.count('Alpha')) # 查找指定元素出现的次数
# tuple ——> list
print(list(tuples))
# list ——> tuple
print(tuple(lists))
0
1
['Alex', 'Alpha', 'Beta']
('Alex', 'Alpha', 'Beta')
1.2 基本数据类型 —— 集合
主要用于保存不重复元素,集合中所有元素都要放在一对{},两个相邻的元素使用逗号隔开。
集合最重要的一个应用就是去重,因为集合中的每个元素都是唯一的
- 集合本身是可变的,但是集合中的元素是不可变的,比如字符串,整型数字,浮点型数字,元组
- 集合是无序的,集合中的元素没有先后之分
- 集合的元素是互异的,不重复的
1.2.1 集合元素的增加
sets = {1,1,2,5,6,8,9,4,7,5,6,22,1,3,5}
print(sets)
sets.add(520) # 单个元素的增加
print(sets)
sets.add(1) # 添加一个已经存在的元素,没有效果
print(sets)
sets1 = {"Python","Java","C++"}
sets2 = {"Markdown","Numpy","Pandas"}
sets.update(sets1,sets2) # 集合元素的添加,update可以支持传入多个参数
print(sets)
{1, 2, 3, 4, 5, 6, 7, 8, 9, 22}
{1, 2, 3, 4, 5, 6, 7, 8, 9, 520, 22}
{1, 2, 3, 4, 5, 6, 7, 8, 9, 520, 22}
{1, 2, 3, 4, 5, 6, 7, 8, 9, 520, 'Numpy', 'Markdown', 'Pandas', 22, 'C++', 'Python', 'Java'}
sets = {1,1,2,5,6,8,9,4,7,5,6,22,1,3,5}
sets.discard(1) # 可以删除集合中指定元素,删除元素不存在时,也不会报错
sets.remove(2) # 可以删除指定元素,删除元素不存在时,则会报错
print(sets.pop()) # 删除随机元素,并返回该元素,当集合为空时,删除则会引发KeyError
sets.clear() # 清空集合所有元素
print(sets)
3
set()
1.2.2 集合的运算
set1 = {1,2,3,4,5,6}
set2 = {5,2,0,1,3,1,4}
print(set1.union(set2)) # 取两个集合的并集
print(set1.difference(set2)) # 取两个集合的差集
set1.difference_update(set2)
print(set1) # 计算集合的差集之后,对原集合进行更新
{0, 1, 2, 3, 4, 5, 6}
{6}
{6}
set1 = {1,2,3,4,5,6}
set2 = {5,2,0,1,3,1,4}
print(set1.intersection(set2)) # 取交集
set1.intersection_update(set2) # 取交集之后对原集合进行更新
print(set1)
{1, 2, 3, 4, 5}
{1, 2, 3, 4, 5}
set1 = {1,2,3,4,5,6}
set2 = {5,2,0,1,3,1,4}
print(set1.symmetric_difference(set2)) # 对称差集, 并集减去交集, 就是去除两个集合中重复的元素
set1.symmetric_difference_update(set2) # 取对称差集之后对原集合进行更新
print(set1)
{0, 6}
{0, 6}
set1 = {1,2,3,4,5,6}
set2 = {5,2,0,1,3,1,4}
set1 - set2 # 差集
set1 & set2 # 交集
set1 | set2 # 并集
set1 ^ set2 # 对称差集
set1 = {1,2,3,4,5,6}
set2 = {5,2,0,1,3,1,4}
print(set1.issubset(set2)) # 判断一个集合的元素是否在另一个集合中
print(set1.issuperset(set2)) # 判断一个集合的元素是否包含另一个集合的所有元素
print(set1.isdisjoint(set2)) # 判断两个集合是否包含相同的元素,包含返回False 不包含返回True
False
False
False
1.3基本数据类型——字典
定义:{key1:value1,key2:value2}
-
键与值之间用冒号隔开
-
项与项之间用逗号隔开
-
键是唯一的,但值可以不唯一
-
字典是Python中唯一的映射类型,key-value结构
-
字典的键是不可变的,只能是字符串,数字,元组,必须唯一
-
字典可以存放多个值,可以修改
infos = {
'张三':[1210,'一班','河南师范大学'],
'李四':[1211,'二班','河南师范大学']
}
print(infos['张三'])
[1210, '一班', '河南师范大学']
1.3.1 字典中常用方法
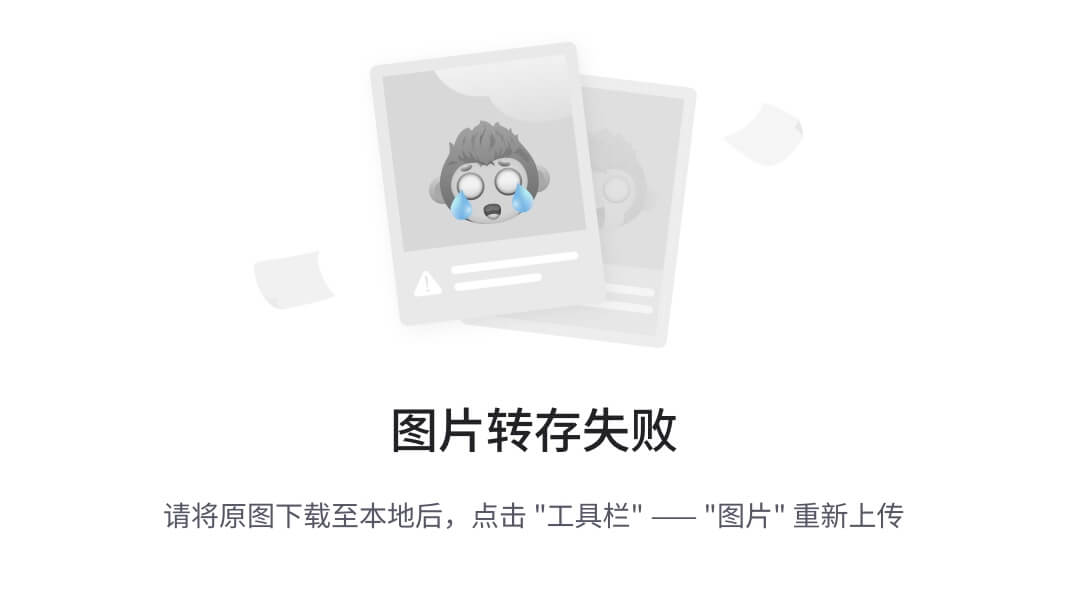
infos = {
'张三':[1210,'一班','河南师范大学'],
'李四':[1211,'二班','河南师范大学']
}
print(infos.keys()) # 查看所有的键
print(infos.values()) # 查看所有的值
print(infos.items()) # 查看所有的键值对
print(infos.get('Loong','你查询的信息不存在')) # 如果查询的键存在,则返回该键的值,不存在则返回设置的信息
print(infos.get('张三','你查询的信息不存在'))
print(infos.get('Loong')) # 如果不设置,则没有任何返回结果
dict_keys(['张三', '李四'])
dict_values([[1210, '一班', '河南师范大学'], [1211, '二班', '河南师范大学']])
dict_items([('张三', [1210, '一班', '河南师范大学']), ('李四', [1211, '二班', '河南师范大学'])])
你查询的信息不存在
[1210, '一班', '河南师范大学']
None
infos = {
'张三':[1210,'一班','河南师范大学'],
'李四':[1211,'二班','河南师范大学']
}
infos['Loong'] = [520,'一班','河南师范大学'] # 添加新的键值对
infos['李四'] = [1210,'一班','河南师范大学'] # 添加新的键值对,如果添加的键已经存在,则会覆盖原先的
print(infos)
infos.setdefault('王五',[123,'二班','河南师范大学']) # 添加新的键值对,如果键不存在,则添加对应信息
print(infos)
print(infos.setdefault('Loong',[123,'二班','河南师范大学'])) # 添加新的键值对,如果键存在,则返回原先的该键对应的信息
{'张三': [1210, '一班', '河南师范大学'], '李四': [1210, '一班', '河南师范大学'], 'Loong': [520, '一班', '河南师范大学']}
{'张三': [1210, '一班', '河南师范大学'], '李四': [1210, '一班', '河南师范大学'], 'Loong': [520, '一班', '河南师范大学'], '王五': [123, '二班', '河南师范大学']}
[520, '一班', '河南师范大学']
info1 = {
'张三':[1210,'一班','河南师范大学'],
'李四':[1211,'二班','河南师范大学']
}
info2 = {
'Loong':[520,'一班','河南师范大学']
}
info1.update(info2) # 合并字典
print(info1)
{'张三': [1210, '一班', '河南师范大学'], '李四': [1211, '二班', '河南师范大学'], 'Loong': [520, '一班', '河南师范大学']}
infos = {
'张三':[1210,'一班','河南师范大学'],
'李四':[1211,'二班','河南师范大学']
}
print(infos.popitem()) # 随机删除字典中的元素,并返回删除后的值,原始数据会被修改,如果是空字典则会引发Key error 错误
print(infos)
print(infos.pop('张三')) # 删除指定的键,返回该键的值,如果该键不存在,则引发Key error 错误
print(infos)
('李四', [1211, '二班', '河南师范大学'])
{'张三': [1210, '一班', '河南师范大学']}
[1210, '一班', '河南师范大学']
{}
1.4 数据类型综合练习
import requests
url = "https://api-takumi-static.mihoyo.com/content_v2_user/app/b9d5f96cd69047eb/getContentList?iPageSize=7&iPage=2&sLangKey=zh-cn&iChanId=699&isPreview=0"
headers = {"user-agent":"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/128.0.0.0 Safari/537.36"}
dicts = requests.get(url,headers=headers).json()
info = eval(dicts['data']['list'][1]['sExt'])['699_0'][0]
for i in dicts['data']['list']:
url = eval(i['sExt'])['699_0'][0]['url']
with open(f'./image/{dicts["data"]["list"].index(i)}_{info["name"]}','wb') as f:
f.write(requests.get(url,headers=headers).content)
2.1 流程控制语句——循环
-
for 循环:计次循环,指重复一定次数的循环
-
while 循环:一直重复,直到条件不满足时才结束
for 迭代变量 in 迭代对象:
&emp;循环体
nums = [1,2,3,4,5]
for i in nums:
print(i**2)
1
4
9
16
25
2.1.1 range的使用
range(起始,结束,步长)
# 打印 1-10 以内的奇数
for i in range(1,11,2):
print(i)
1
3
5
7
9
2.1.2 enumerate的使用
lists = ['A','B','C','D','E','F','G']
for i in lists:
print(i,lists.index(i))
for index,i in enumerate(lists):
print(index,i)
A 0
B 1
C 2
D 3
E 4
F 5
G 6
0 A
1 B
2 C
3 D
4 E
5 F
6 G
2.1.3 zip函数
可以将多个可迭代对象进行组合,然后进行遍历
list1 = ['a','b','c','d']
list2 = ['A','B','C','D']
for x,y in zip(list1,list2):
print(x,y)
a A
b B
c C
d D
2.1.4 推导式
# 构建一个列表,用来存储1-100之间所有元素的平方和立方值
nums = []
for i in range(1,101):
nums.append((i**2,i**3))
nums = [(i**2,i**3) for i in range(1,101)] # 列表推导式
num = {i:(i**2,i**3) for i in range(1,101)} # 字典推导式
{1: (1, 1), 2: (4, 8), 3: (9, 27), 4: (16, 64), 5: (25, 125), 6: (36, 216), 7: (49, 343), 8: (64, 512), 9: (81, 729), 10: (100, 1000), 11: (121, 1331), 12: (144, 1728), 13: (169, 2197), 14: (196, 2744), 15: (225, 3375), 16: (256, 4096), 17: (289, 4913), 18: (324, 5832), 19: (361, 6859), 20: (400, 8000), 21: (441, 9261), 22: (484, 10648), 23: (529, 12167), 24: (576, 13824), 25: (625, 15625), 26: (676, 17576), 27: (729, 19683), 28: (784, 21952), 29: (841, 24389), 30: (900, 27000), 31: (961, 29791), 32: (1024, 32768), 33: (1089, 35937), 34: (1156, 39304), 35: (1225, 42875), 36: (1296, 46656), 37: (1369, 50653), 38: (1444, 54872), 39: (1521, 59319), 40: (1600, 64000), 41: (1681, 68921), 42: (1764, 74088), 43: (1849, 79507), 44: (1936, 85184), 45: (2025, 91125), 46: (2116, 97336), 47: (2209, 103823), 48: (2304, 110592), 49: (2401, 117649), 50: (2500, 125000), 51: (2601, 132651), 52: (2704, 140608), 53: (2809, 148877), 54: (2916, 157464), 55: (3025, 166375), 56: (3136, 175616), 57: (3249, 185193), 58: (3364, 195112), 59: (3481, 205379), 60: (3600, 216000), 61: (3721, 226981), 62: (3844, 238328), 63: (3969, 250047), 64: (4096, 262144), 65: (4225, 274625), 66: (4356, 287496), 67: (4489, 300763), 68: (4624, 314432), 69: (4761, 328509), 70: (4900, 343000), 71: (5041, 357911), 72: (5184, 373248), 73: (5329, 389017), 74: (5476, 405224), 75: (5625, 421875), 76: (5776, 438976), 77: (5929, 456533), 78: (6084, 474552), 79: (6241, 493039), 80: (6400, 512000), 81: (6561, 531441), 82: (6724, 551368), 83: (6889, 571787), 84: (7056, 592704), 85: (7225, 614125), 86: (7396, 636056), 87: (7569, 658503), 88: (7744, 681472), 89: (7921, 704969), 90: (8100, 729000), 91: (8281, 753571), 92: (8464, 778688), 93: (8649, 804357), 94: (8836, 830584), 95: (9025, 857375), 96: (9216, 884736), 97: (9409, 912673), 98: (9604, 941192), 99: (9801, 970299), 100: (10000, 1000000)}
# 任务一:创建一个列表,里面存放1-10所有偶数的平方和立方
lists = [(i**2,i**3) for i in range(2,11,2)]
# 任务二:创建一个字典,里面存放1-10所有奇数的平方根和立方根,其中字典的键是对应的数值,字典的值是平方根和立方根列表
sets = {i:(i**(1/2),i**(1/3)) for i in range(1,10,2)}
print(lists)
print(sets)
[(4, 8), (16, 64), (36, 216), (64, 512), (100, 1000)]
{1: (1.0, 1.0), 3: (1.7320508075688772, 1.4422495703074083), 5: (2.23606797749979, 1.7099759466766968), 7: (2.6457513110645907, 1.912931182772389), 9: (3.0, 2.080083823051904)}
2.2 输出语句——print
help(print) # 可以使用help函数打印函数的帮助信息
Help on built-in function print in module builtins:
print(*args, sep=' ', end='\n', file=None, flush=False)
Prints the values to a stream, or to sys.stdout by default.
sep
string inserted between values, default a space.
end
string appended after the last value, default a newline.
file
a file-like object (stream); defaults to the current sys.stdout.
flush
whether to forcibly flush the stream.
print('你','好','吗',sep='',end='?')
你好吗?
print语句的格式化输出
f-strings 方法
name = "Loong"
age = 20
x = 18
pi = 3.14159265358
print(f"My name is {name} and I am {age} years old")
print(f"x + 1 = {x+1}")
print(f"Pi is {pi:.2f}") # :.<precision>f :浮点数格式化
print("My name is {} and I am {} years old".format(name,age))
My name is Loong and I am 20 years old
x + 1 = 19
Pi is 3.14
My name is Loong and I am 20 years old
# 美化输出
from rich import print as pprint
name = "Loong"
age = 20
x = 18
pi = 3.14159265358
pprint(f"My name is {name} and I am {age} years old")
pprint(f"x + 1 = {x+1}")
pprint(f"Pi is {pi:.2f}") # :.<precision>f :浮点数格式化
pprint("My name is {} and I am {} years old".format(name,age))
My name is Loong and I am 20 years old
x + 1 = 19
Pi is 3.14
My name is Loong and I am 20 years old
for i in range(1,10):
for j in range(1,i+1):
print(f"{j}*{i} = {i*j}",end=" ")
print()
1*1 = 1
1*2 = 2 2*2 = 4
1*3 = 3 2*3 = 6 3*3 = 9
1*4 = 4 2*4 = 8 3*4 = 12 4*4 = 16
1*5 = 5 2*5 = 10 3*5 = 15 4*5 = 20 5*5 = 25
1*6 = 6 2*6 = 12 3*6 = 18 4*6 = 24 5*6 = 30 6*6 = 36
1*7 = 7 2*7 = 14 3*7 = 21 4*7 = 28 5*7 = 35 6*7 = 42 7*7 = 49
1*8 = 8 2*8 = 16 3*8 = 24 4*8 = 32 5*8 = 40 6*8 = 48 7*8 = 56 8*8 = 64
1*9 = 9 2*9 = 18 3*9 = 27 4*9 = 36 5*9 = 45 6*9 = 54 7*9 = 63 8*9 = 72 9*9 = 81
print('\t'.join(['1*2 = 2','2*2 = 4'])) # 使用制表符将列表连起来
1*2 = 2 2*2 = 4
print('\n'.join(['\t'.join([f"{a}* {b} = {a*b}" for b in range(1,a+1)]) for a in range(1,10)]))
1* 1 = 1
2* 1 = 2 2* 2 = 4
3* 1 = 3 3* 2 = 6 3* 3 = 9
4* 1 = 4 4* 2 = 8 4* 3 = 12 4* 4 = 16
5* 1 = 5 5* 2 = 10 5* 3 = 15 5* 4 = 20 5* 5 = 25
6* 1 = 6 6* 2 = 12 6* 3 = 18 6* 4 = 24 6* 5 = 30 6* 6 = 36
7* 1 = 7 7* 2 = 14 7* 3 = 21 7* 4 = 28 7* 5 = 35 7* 6 = 42 7* 7 = 49
8* 1 = 8 8* 2 = 16 8* 3 = 24 8* 4 = 32 8* 5 = 40 8* 6 = 48 8* 7 = 56 8* 8 = 64
9* 1 = 9 9* 2 = 18 9* 3 = 27 9* 4 = 36 9* 5 = 45 9* 6 = 54 9* 7 = 63 9* 8 = 72 9* 9 = 81
2.3 流程控制语句——while
while 条件表达式:
循环体
count = 0
while count < 10:
if count == 2:
count += 1
continue
if count == 8:
break
print(f"第{count+1}次循环")
count = count + 1
第1次循环
第2次循环
第4次循环
第5次循环
第6次循环
第7次循环
第8次循环
break: 终止循环
continue: 跳过当前循环
Cell In[45], line 1
break: 终止循环
^
SyntaxError: invalid syntax
2.4 流程控制语句——输入语句
a = input("请输入")
print(a)
请输入520
520
2.5 流程控制语句——条件判断语句
-
单分支
if 条件1:
执行满足条件一的代码 -
双分支
if 条件1:
执行满足条件一的代码
else:
执行其他条件的代码 -
多分支
if 条件1:
执行满足条件一的代码
elif 条件2:
执行满足条件二的代码
else:
执行其他条件的代码
import random
num = random.randint(0,101)
guess_number = input("请输入你要猜的数字:")
while(guess_number!="esc"):
if guess_number.isdigit():
guess_number = int(guess_number)
if num > guess_number:
print("你猜的小了")
elif num < guess_number:
print("你猜的大了")
else:
print("恭喜你猜对了!")
break
else:
print("请不要输入非法字符")
guess_number = input("请输入你要猜的数字:")
请输入你要猜的数字:2311
你猜的大了
请输入你要猜的数字:5465
你猜的大了
请输入你要猜的数字:esc
# 统计每个单词出现的频率,找出TOP10高频词汇,要求单词长度大于3
strs = """
Certainly! Here's a short English passage for you:
In the tranquility of the early morning, the sun gently caressed the dew-kissed petals of the roses in the garden. A soft breeze whispered through the trees, carrying the sweet scent of blooming jasmine. The world was awakening to a symphony of birdsong, each note a testament to the beauty of a new day. The vibrant hues of the sky painted a picture of hope and promise, as the first light of dawn broke through the darkness, heralding the arrival of a day filled with endless possibilities.
"""
for i in strs:
if not(i.isalpha()) and i!=" ":
strs = strs.replace(i,"")
nstrs = strs.split(" ")
# print(nstrs)
nums = {}
lists = []
for i in nstrs:
if len(i) >= 3:
nums[i]=strs.count(i)
for i in nums.items():
lists.append(i)
# print(lists)
lists.sort()
print(lists)
[('Certainly', 1), ('English', 1), ('Heres', 1), ('The', 2), ('and', 1), ('arrival', 1), ('awakening', 1), ('beauty', 1), ('birdsong', 1), ('blooming', 1), ('breeze', 1), ('broke', 1), ('caressed', 1), ('carrying', 1), ('darkness', 1), ('dawn', 1), ('day', 2), ('dewkissed', 1), ('each', 1), ('early', 1), ('endless', 1), ('filled', 1), ('first', 1), ('for', 1), ('garden', 1), ('gently', 1), ('heralding', 1), ('hope', 1), ('hues', 1), ('jasmine', 1), ('light', 1), ('morning', 1), ('new', 1), ('note', 1), ('painted', 1), ('passage', 1), ('petals', 1), ('picture', 1), ('possibilities', 1), ('promise', 1), ('roses', 1), ('scent', 1), ('short', 1), ('sky', 1), ('soft', 1), ('sun', 1), ('sweet', 1), ('symphony', 1), ('testament', 1), ('the', 13), ('through', 2), ('tranquility', 1), ('trees', 1), ('vibrant', 1), ('was', 1), ('whispered', 1), ('with', 1), ('world', 1), ('youIn', 1)]
3.1 运算符
- 算数运算符
- 赋值运算符
- 成员运算符
- in:判断
- 身份运算符
is:判断内存中地址是否相同
is not:判断内存中地址是否不相同
lists = []
n = int(input())
count = 8
for i in range(0,n):
if i==0:
lists.append(count)
else:
count = count*10+8
lists.append(count)
num = 0
for i in lists:
num += i
print(num)
5
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[130], line 10
8 count = count*10+8
9 lists.append(count)
---> 10 sum(lists)
TypeError: 'int' object is not callable
lists = []
for i in range(1,101):
if str(i).count('5'):
lists.append(i)
print(i,end=" ")
print()
print(lists)
[print(i,end=' ') for i in range(1,101) if '5' in str(i)]
5 15 25 35 45 50 51 52 53 54 55 56 57 58 59 65 75 85 95
[5, 15, 25, 35, 45, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 65, 75, 85, 95]
5 15 25 35 45 50 51 52 53 54 55 56 57 58 59 65 75 85 95