
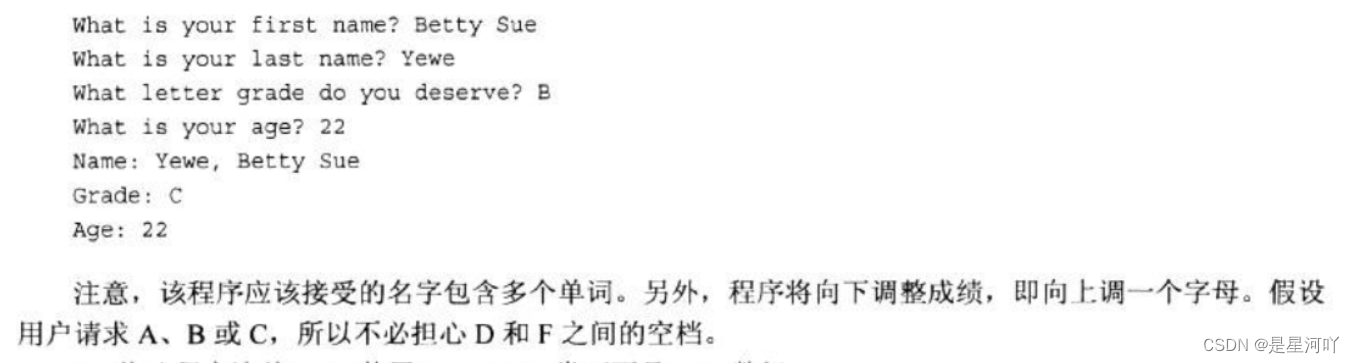
#include <iostream>
#include <string>
using namespace std;
int main() {
string first_name, last_name;
char grade;
int age;
cout << "What is your first name? ";
getline(cin, first_name);
cout << "What is your last name? ";
getline(cin, last_name);
cout << "What letter grade do you deserve? ";
cin >> grade;
cout << "What is your age? ";
cin >> age;
// 根据要求向下调整成绩
if (grade == 'A' || grade == 'a')
grade = 'B';
else if (grade == 'B' || grade == 'b')
grade = 'C';
else if (grade == 'C' || grade == 'c')
grade = 'D';
else if (grade == 'D' || grade == 'd')
grade = 'E';
else if (grade == 'F' || grade == 'f')
grade = 'G';
// 显示用户信息
cout << "Name: " << last_name << ", " << first_name << endl;
cout << "Grade: " << grade << endl;
cout << "Age: " << age << endl;
return 0;
}

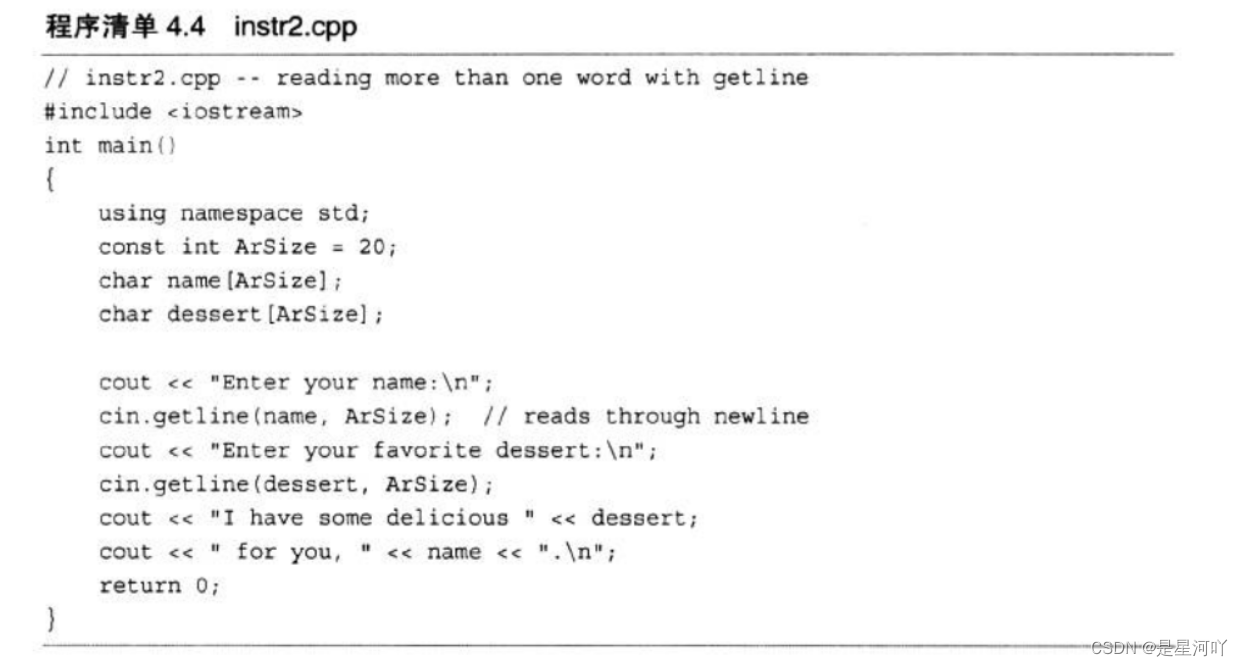
#include <iostream>
#include <string>
using namespace std;
int main() {
string name;
string dessert;
cout << "Enter your name:\n";
getline(cin, name);
cout << "Enter your favorite dessert:\n";
getline(cin, dessert);
cout << "I have some delicious " << dessert;
cout << " for you, " << name << ".\n";
return 0;
}

#include <iostream>
#include <cstring>
using namespace std;
int main() {
const int max_length = 100; // 定义最大长度
char first_name[max_length]; // 存储名字的数组
char last_name[max_length]; // 存储姓氏的数组
// 请求用户输入名字
cout << "Enter your first name:";
cin.getline(first_name, max_length);
// 请求用户输入姓氏
cout << "Enter your last name:";
cin.getline(last_name, max_length);
// 组合名字和姓氏,并在中间加上逗号和空格
char full_name[2 * max_length + 3]; // 考虑到逗号和空格,所以数组大小是两个名字长度加上逗号和空格,再加1表示字符串结尾的空字符
strcpy_s(full_name, last_name); // 复制姓氏到 full_name
strcat_s(full_name, ", "); // 添加逗号和空格
strcat_s(full_name, first_name); // 添加名字
// 显示组合结果
cout << "Here's the information in a single string:" << full_name << endl;
return 0;
}

#include <iostream>
#include <string>
using namespace std;
struct CandyBar {
string brand;
double weight;
int calorie;
};
int main() {
CandyBar snack =
{
"Mocha Munch",
2.3,
350
};
cout << "糖果品牌: " << snack.brand << endl;
cout << "糖果重量: " << snack.weight << endl;
cout << "糖果卡路里含量: " << snack.calorie << endl;
return 0;
}

#include <iostream>
#include <string>
using namespace std;
// 定义 CandyBar 结构
struct CandyBar {
string brand;
double weight;
int calories;
};
int main() {
// 创建 CandyBar 数组并初始化
CandyBar snacks[3] = {
{"Mocha Munch", 2.3, 350},
{"KitKat", 1.5, 210},
{"Snickers", 2.0, 280}
};
// 显示每个结构的内容
for (int i = 0; i < 3; ++i) {
cout << "第 " << i + 1 << " 个糖块:" << endl;
cout << "品牌:" << snacks[i].brand << endl;
cout << "重量:" << snacks[i].weight << " 盎司" << endl;
cout << "卡路里含量:" << snacks[i].calories << " 卡路里" << endl << endl;
}
return 0;
}

#include <iostream>
#include <string>
using namespace std;
//定义披萨饼信息的结构
struct Pizza {
string company_name;
double diameter;
double weight;
};
int main() {
//创建一个Pizza结构变量
Pizza pizza;
//请求用户输入披萨饼信息
cout << "请输入披萨饼公司的名称:";
getline(cin, pizza.company_name);
cout << "请输入披萨饼的直径:";
cin >> pizza.diameter;
cout << "请输入披萨饼的重量:";
cin >> pizza.weight;
// 显示比萨饼信息
cout << "\n比萨饼信息如下:" << endl;
cout << "公司名称:" << pizza.company_name << endl;
cout << "直径:" << pizza.diameter << " 英寸" << endl;
cout << "重量:" << pizza.weight << " 磅" << endl;
return 0;
}

#include <iostream>
#include <string>
using namespace std;
//定义披萨饼信息的结构
struct Pizza {
string company_name;
double diameter;
double weight;
};
int main() {
//创建一个Pizza结构变量
Pizza* pizza_ptr = new Pizza;
// 请求用户输入比萨饼直径
cout << "请输入比萨饼的直径(英寸):";
cin >> pizza_ptr->diameter;
cin.ignore(); // 忽略输入缓冲区中的换行符
// 请求用户输入比萨饼公司名称
cout << "请输入比萨饼公司的名称:";
getline(cin, pizza_ptr->company_name);
// 请求用户输入比萨饼重量
cout << "请输入比萨饼的重量(磅):";
cin >> pizza_ptr->weight;
// 显示比萨饼信息
cout << "\n比萨饼信息如下:" << endl;
cout << "公司名称:" << pizza_ptr->company_name << endl;
cout << "直径:" << pizza_ptr->diameter << " 英寸" << endl;
cout << "重量:" << pizza_ptr->weight << " 磅" << endl;
// 释放动态分配的内存
delete pizza_ptr;
return 0;
}

#include <iostream>
#include <string>
using namespace std;
// 定义 CandyBar 结构
struct CandyBar {
string brand;
double weight;
int calories;
};
int main() {
// 动态分配包含3个 CandyBar 元素的数组
CandyBar* snacks = new CandyBar[3];
// 初始化数组中的每个元素
snacks[0] = {"Mocha Munch", 2.3, 350};
snacks[1] = {"KitKat", 1.5, 210};
snacks[2] = {"Snickers", 2.0, 280};
// 显示每个结构的内容
for (int i = 0; i < 3; ++i) {
cout << "第 " << i+1 << " 个糖块:" << endl;
cout << "品牌:" << snacks[i].brand << endl;
cout << "重量:" << snacks[i].weight << " 盎司" << endl;
cout << "卡路里含量:" << snacks[i].calories << " 卡路里" << endl << endl;
}
// 释放动态分配的内存
delete[] snacks;
return 0;
}

#include <iostream>
#include <array>
using namespace std;
int main() {
const int num_attempts = 3; // 成绩次数
array<double, num_attempts> scores; // 存储成绩的 array 对象
// 请求用户输入成绩
cout << "请输入三次40码跑的成绩:" << endl;
for (int i = 0; i < num_attempts; ++i) {
cout << "第 " << i+1 << " 次成绩:";
cin >> scores[i];
}
// 计算平均成绩
double total_score = 0;
for (int i = 0; i < num_attempts; ++i) {
total_score += scores[i];
}
double average_score = total_score / num_attempts;
// 显示成绩次数和平均成绩
cout << "成绩次数:" << num_attempts << endl;
cout << "平均成绩:" << average_score << endl;
return 0;
}