
#include <iostream>
double harmonicMean(double x, double y);
int main() {
using namespace std;
double num1, num2, harmean;
//提示用户输入两个数
cout << "Please enter two numbers (type q to quit):";
while (cin >> num1 >> num2) {
//计算num1和num2的调和平均数
harmean = harmonicMean(num1,num2);
//显示结果
cout << "Harmonic Mean = " << harmean << endl;
}
cout << "Done.\n";
return 0;
}
double harmonicMean(double x, double y) {
// 如果有一个数为0,则返回0
if (x == 0 || y == 0)
return 0;
return 2.0 * x * y / (x + y);
}

#include <iostream>
#include <string>
using namespace std;
const int MAX_NUM = 10;
int input(double golfGrade[],int maxnum);
void display(const double golfGrade[], int realnum, double mean);
double mean(const double golfGrade[], int realnum);
int main() {
int realnum = 0;
double meanGrade;
double golfGrade[MAX_NUM];
realnum = input(golfGrade, MAX_NUM);
meanGrade = mean(golfGrade, realnum);
display(golfGrade, realnum, meanGrade);
return 0;
}
int input(double golfGrade[], int maxnum) {
int realnum = 0;
cout << "请输入高尔夫成绩(最多十个):\n";
while (realnum < maxnum) {
if (!(cin >> golfGrade[realnum])) {
// 非法输入,清除输入缓冲区并重置错误标志
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
break;
}
realnum++;
if (cin.peek() == '\n') {
break;
}
}
return realnum;
}
void display(const double golfGrade[], int realnum, double mean) {
cout << "所有高尔夫成绩: ";
for (int i = 0; i < realnum; i++)
cout << golfGrade[i] << " ";
cout << "\n平均成绩: " << mean << endl;
}
double mean(const double golfGrade[], int realnum) {
if (realnum == 0) {
return 0;
}
double sum = 0;
for (int i = 0; i < realnum; i++)
sum += golfGrade[i];
return sum / realnum;
}
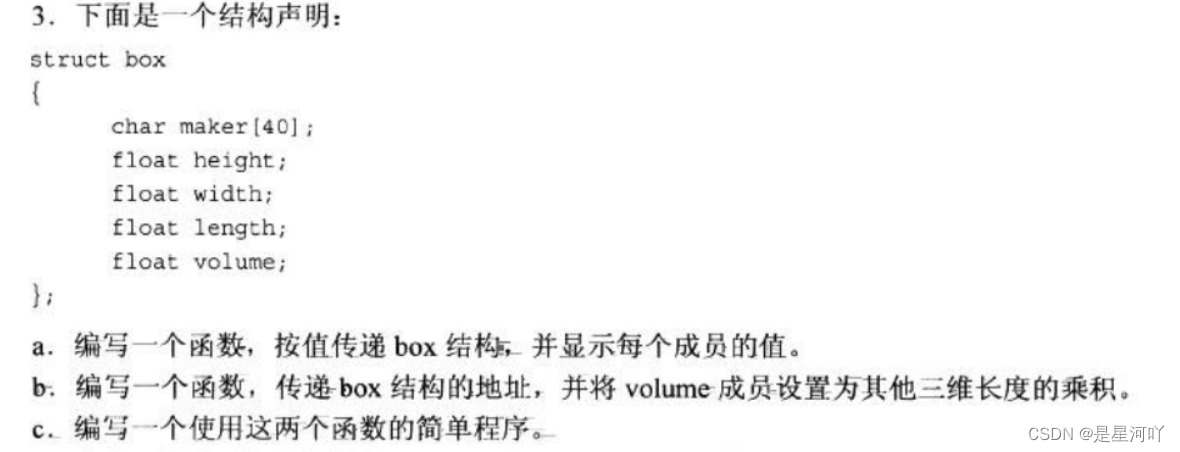
#include <iostream>
#include <string>
using namespace std;
const int MAX_MAKER_LENGTH = 40;
struct box {
char maker[MAX_MAKER_LENGTH];
float height;
float width;
float length;
float volume;
};
// 显示 box 结构的成员值
void displayBox(box b1);
// 设置 box 结构的体积成员为其他三维长度的乘积
void setVolume(box* b1);
int main() {
box myBox;
// 输入箱子信息
cout << "请输入箱子的制造商: ";
cin.getline(myBox.maker, MAX_MAKER_LENGTH);
cout << "请输入箱子的高度: ";
cin >> myBox.height;
cout << "请输入箱子的宽度: ";
cin >> myBox.width;
cout << "请输入箱子的长度: ";
cin >> myBox.length;
// 计算并设置箱子的体积
setVolume(&myBox);
// 显示箱子的信息
cout << "\n箱子的信息如下:" << endl;
displayBox(myBox);
return 0;
}
void displayBox(box b1) {
cout << "制造商: " << b1.maker << endl;
cout << "高度: " << b1.height << endl;
cout << "宽度: " << b1.width << endl;
cout << "长度: " << b1.length << endl;
cout << "体积: " << b1.volume << endl;
}
void setVolume(box* b1) {
b1->volume = b1->height * b1->length * b1->width;
}

#include <iostream>
using namespace std;
int factorial(int n);
int main() {
int n,n_factorial;
cout << "Please enter a integer(type -1 to quit): ";
while (std::cin >> n && n >= 0) {
n_factorial = factorial(n);
cout << n << " ! = " << n_factorial << endl;
}
return 0;
}
int factorial(int n) {
if (n == 0)
return 1;
n = n * factorial(n - 1);
return n;
}
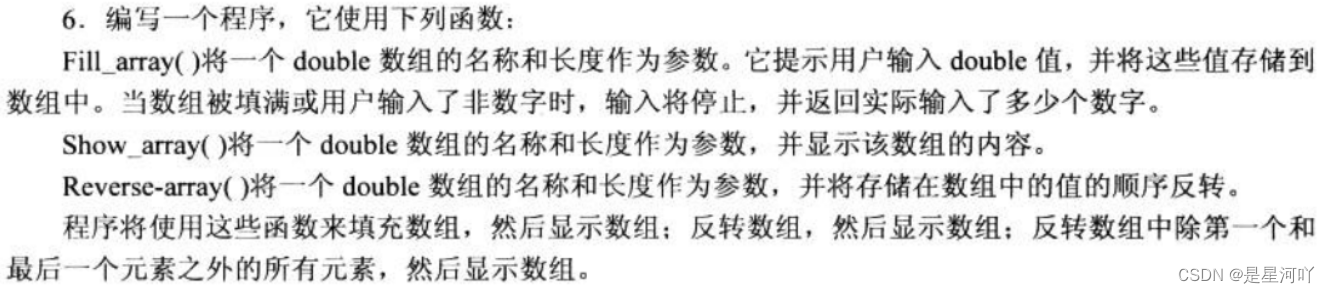
#include <iostream>
// Function to fill the array with double values
int fillArray(double arr[], int length) {
int count = 0;
for (int i = 0; i < length; ++i) {
std::cout << "Enter value for index " << i << ": ";
if (!(std::cin >> arr[i])) {
// If input is not a double value, stop input
break;
}
count++;
}
return count;
}
// Function to display the contents of the array
void showArray(double arr[], int length) {
std::cout << "Array contents:";
for (int i = 0; i < length; ++i) {
std::cout << " " << arr[i];
}
std::cout << std::endl;
}
// Function to reverse the array
void reverseArray(double arr[], int length) {
int start = 0;
int end = length - 1;
while (start < end) {
// Swap elements at start and end positions
double temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
// Function to display array excluding first and last elements
void showArrayExcludingFirstLast(double arr[], int length) {
std::cout << "Array contents excluding first and last elements:";
for (int i = 1; i < length - 1; ++i) {
std::cout << " " << arr[i];
}
std::cout << std::endl;
}
int main() {
const int SIZE = 10; // Maximum size of the array
double arr[SIZE];
int count = fillArray(arr, SIZE);
std::cout << "Filled " << count << " elements into the array." << std::endl;
showArray(arr, count);
reverseArray(arr, count);
std::cout << "Reversed array:";
showArray(arr, count);
reverseArray(arr, count); // Reversing again to bring back to original order
showArrayExcludingFirstLast(arr, count);
return 0;
}
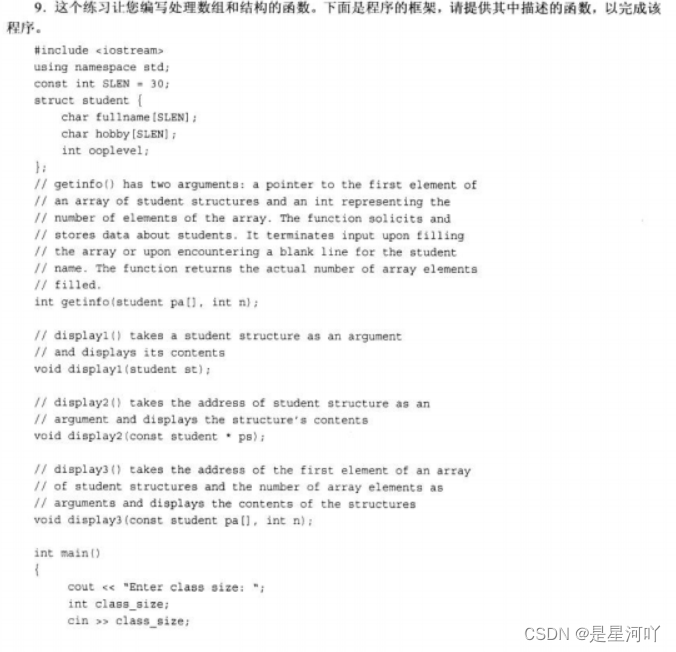
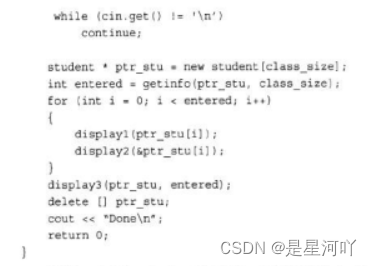
#include <iostream>
#include <cstring>
using namespace std;
const int SLEN = 30;
struct Student {
char fullname[SLEN];
char hobby[SLEN];
int ooplevel;
};
int getinfo(Student pa[], int m);
void display1(Student st);
void display2(const Student* ps);
void display3(const Student pa[], int n);
int main() {
cout << "Enter class size: ";
int class_size;
cin >> class_size;
while (cin.get() != '\n')
continue;
Student* ptr_stu = new Student[class_size];
int entered = getinfo(ptr_stu, class_size);
for (int i = 0; i < entered; i++) {
cout << "\nStudent #" << (i + 1) << ":\n";
display1(ptr_stu[i]);
display2(&ptr_stu[i]);
}
display3(ptr_stu, entered);
delete[] ptr_stu;
cout << "Done\n";
return 0;
}
// getinfo() 函数
int getinfo(Student pa[], int m) {
int count = 0;
cout << "Enter information for students (or blank line to stop):\n";
while (count < m) {
cout << "Student #" << (count + 1) << ":\n";
cout << "Enter full name: ";
cin.getline(pa[count].fullname, SLEN);
if (strlen(pa[count].fullname) == 0) {
break; // Stop input if blank line is encountered
}
cout << "Enter hobby: ";
cin.getline(pa[count].hobby, SLEN);
cout << "Enter oop level: ";
cin >> pa[count].ooplevel;
cin.get(); // Clear input buffer
count++;
}
return count;
}
// display1() 函数
void display1(Student st) {
cout << "Full Name: " << st.fullname << endl;
cout << "Hobby: " << st.hobby << endl;
cout << "OOP Level: " << st.ooplevel << endl;
}
// display2() 函数
void display2(const Student* ps) {
cout << "Full Name: " << ps->fullname << endl;
cout << "Hobby: " << ps->hobby << endl;
cout << "OOP Level: " << ps->ooplevel << endl;
}
// display3() 函数
void display3(const Student pa[], int n) {
for (int i = 0; i < n; ++i) {
cout << "Student #" << (i + 1) << ":\n";
cout << "Full Name: " << pa[i].fullname << endl;
cout << "Hobby: " << pa[i].hobby << endl;
cout << "OOP Level: " << pa[i].ooplevel << endl;
}
}
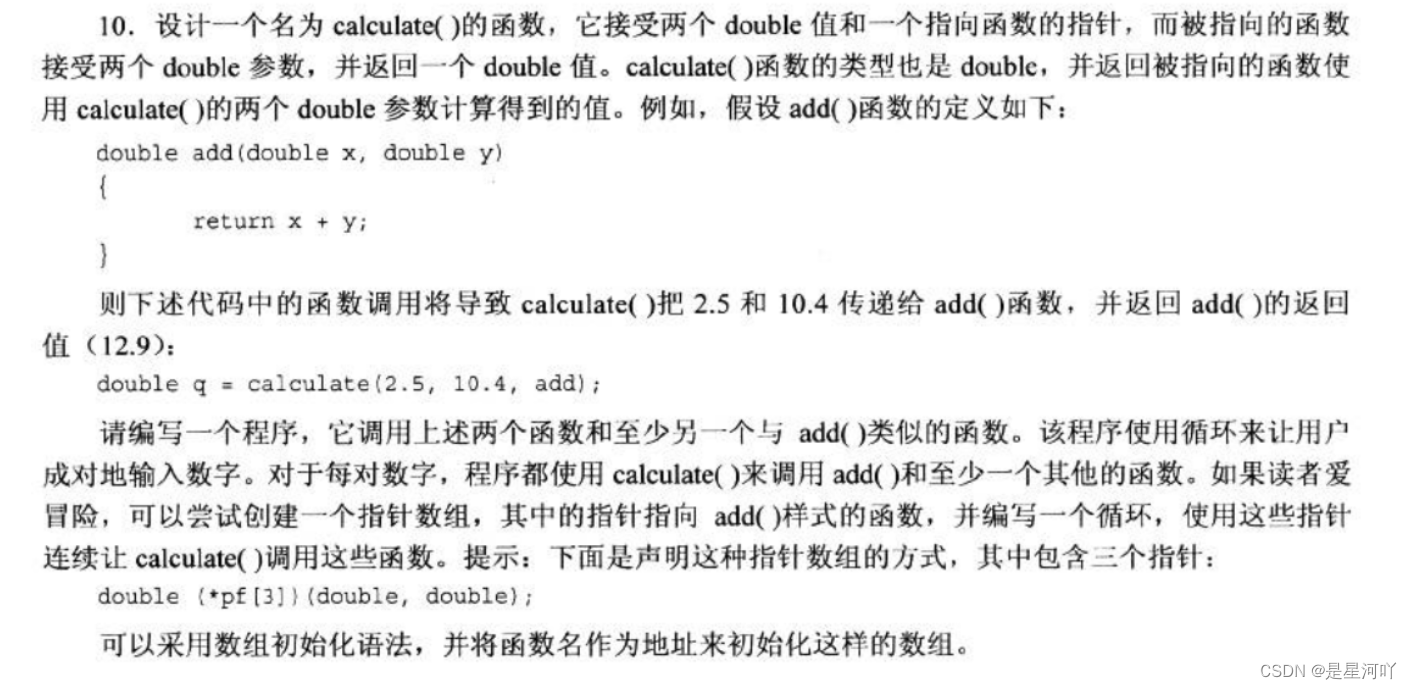
#include <iostream>
// Function prototype for calculate()
double calculate(double x, double y, double (*pf)(double, double));
// Function prototypes for functions similar to add()
double add(double x, double y);
double subtract(double x, double y);
double multiply(double x, double y);
int main() {
// Array of function pointers
double (*pf[3])(double, double) = { add, subtract, multiply };
double x, y;
char choice;
std::cout << "Enter two numbers separated by space (q to quit): ";
while (std::cin >> x >> y) {
std::cout << "Enter function to use (a for add, s for subtract, m for multiply): ";
std::cin >> choice;
double result;
switch (choice) {
case 'a':
result = calculate(x, y, pf[0]);
break;
case 's':
result = calculate(x, y, pf[1]);
break;
case 'm':
result = calculate(x, y, pf[2]);
break;
default:
std::cout << "Invalid choice! Try again.\n";
continue;
}
std::cout << "Result: " << result << std::endl;
std::cout << "Enter two numbers separated by space (q to quit): ";
}
std::cout << "Bye!\n";
return 0;
}
// Function definition for calculate()
double calculate(double x, double y, double (*pf)(double, double)) {
return pf(x, y);
}
// Function definition for add()
double add(double x, double y) {
return x + y;
}
// Function definition for subtract()
double subtract(double x, double y) {
return x - y;
}
// Function definition for multiply()
double multiply(double x, double y) {
return x * y;
}