Eleven puzzle
Time Limit: 20000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Submission(s): 442 Accepted Submission(s): 107
Problem Description
Partychen invents a new game named “Eleven Puzzle” .Just like the classic game “Eight Puzzle”,but there some difference between them:The shape of the board is different and there are two empty tiles.
The tile in black means it’s empty
Each step you can move only one tile.
Here comes the problem.How many steps at least it required to done the game.
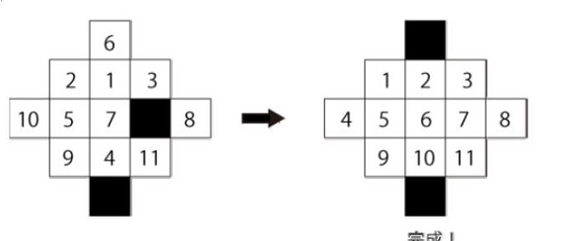
The tile in black means it’s empty
Each step you can move only one tile.
Here comes the problem.How many steps at least it required to done the game.
Input
The first line of input contains one integer specifying the number of test cases to follow.
Every case contains five lines to describe the initial status of the board. 0 means empty.
It’s confirmed that the board is legal.
Every case contains five lines to describe the initial status of the board. 0 means empty.
It’s confirmed that the board is legal.
Output
Output one line for each testcase.Contain an integer denotes the minimum step(s) it need to complete the game.Or “No solution!” if it’s impossible to complete the game within 20 steps.
Sample Input
3 2 1 0 3 4 5 6 7 8 9 0 11 10 0 1 2 3 4 5 6 7 8 9 10 11 0 0 11 10 9 8 7 6 5 4 3 2 1 0
Sample Output
2 0 No solution!
思路:双向搜索(百度文库),和八数码思路一样。
package hdu;
import java.util.ArrayDeque;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.Queue;
import java.util.Scanner;
public class Hdu20130707_3095_4 {
public static final int N = 7;
public static int[][] map = new int[N][N];
public static int x1,x2,y1,y2;
public static int[][] endMap = {
{-1, -1, -1, -1, -1, -1, -1},
{-1, -1, -1, 0, -1, -1, -1},
{-1, -1, 1, 2, 3, -1, -1},
{-1, 4, 5, 6, 7, 8, -1},
{-1, -1, 9, 10, 11, -1, -1},
{-1, -1, -1, 0, -1, -1, -1},
{-1, -1, -1, -1, -1, -1, -1}
};
public static int[][] dis = {
{-1, 0, 1, 0},
{0, 1, 0, -1}
};
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int cases = sc.nextInt();
init(map);
while(cases-- > 0) {
map[1][3] = sc.nextInt();
for(int i=2; i<5; i++) {
map[2][i] = sc.nextInt();
}
for(int i=1; i<6; i++) {
map[3][i] = sc.nextInt();
}
for(int i=2; i<5; i++) {
map[4][i] = sc.nextInt();
}
map[5][3] = sc.nextInt();
if(judge(map)) {
System.out.println(0);
} else {
blank();
System.out.println(bfs());
}
}
}
private static String bfs() {
Map<Integer, Integer> hashmap1 = new HashMap<Integer, Integer>();
Map<Integer, Integer> hashmap2 = new HashMap<Integer, Integer>();
//正向广搜
Queue<Node3095_4> queue = new ArrayDeque<Node3095_4>();
queue.add(new Node3095_4(0, copyMap(map), x1, y1, x2, y2));
hashmap1.put(new Node3095_4(0, copyMap(map), x1, y1, x2, y2).hashCode(), 0);
//反向广搜
Queue<Node3095_4> queueEnd = new ArrayDeque<Node3095_4>();
queueEnd.add(new Node3095_4(0, copyMap(endMap), 1, 3, 5, 3));
hashmap2.put(new Node3095_4(0, copyMap(endMap), 1, 3, 5, 3).hashCode(), 0);
int x1, y1, x2, y2, count, k;
int[][] map, map1;
Node3095_4 temp, node;
while(!queue.isEmpty() || !queueEnd.isEmpty()) {
for(int index=0; index<2; index++) {
if((!queue.isEmpty()&&index==0) || queueEnd.isEmpty()) {
temp = queue.poll();k=1;
} else {
temp = queueEnd.poll();k=2;
}
x1 = temp.x1;
x2 = temp.x2;
y1 = temp.y1;
y2 = temp.y2;
count = temp.count+1;
if(count > 10) break;
map = temp.map;
int x, y;
//第一个空格
for(int i=0; i<4; i++) {
x = x1+dis[0][i];
y = y1+dis[1][i];
if(map[x][y]!=-1 && map[x][y]!=0) {//可以交换
map1 = copyMap(map);
map1[x1][y1] = map[x][y];
map1[x][y] = 0;
node = new Node3095_4(count, map1, x, y, x2, y2);
int key = node.hashCode();
if(k==1) {
if(hashmap2.containsKey(key)) {
return (hashmap2.get(key)+count)+"";
} else {
if(!hashmap1.containsKey(key)) {
queue.add(node);
hashmap1.put(key, count);
}
}
} else if(k==2){
if(hashmap1.containsKey(key)) {
return (hashmap1.get(key)+count)+"";
} else {
if(!hashmap2.containsKey(key)) {
queueEnd.add(node);
hashmap2.put(key, count);
}
}
}
}
}
//第二个空格
for(int i=0; i<4; i++) {
x = x2+dis[0][i];
y = y2+dis[1][i];
if(map[x][y]!=-1 && map[x][y]!=0) {//可以交换
map1 = copyMap(map);
map1[x2][y2] = map[x][y];
map1[x][y] = 0;
node = new Node3095_4(count, map1, x1, y1, x, y);
int key = node.hashCode();
if(k==1) {
if(hashmap2.containsKey(key)) {
return (hashmap2.get(key)+count)+"";
} else {
if(!queue.contains(node)) {
queue.add(node);
hashmap1.put(key, count);
}
}
} else if(k==2){
if(hashmap1.containsKey(key)) {
return (hashmap1.get(key)+count)+"";
} else {
if(!hashmap2.containsKey(key)) {
queueEnd.add(node);
hashmap2.put(key , count);
}
}
}
}
}
}
}
return "No solution!";
}
//判断是否是最终结果
private static boolean judge(int[][] map) {
if(map[1][3] != 0) return false;
for(int i=2; i<5; i++) {
if(map[2][i] != i-1) return false;
}
for(int i=1; i<6; i++) {
if(map[3][i] != i+3) return false;
}
for(int i=2; i<5; i++) {
if(map[4][i] != i+7) return false;
}
if(map[5][3] != 0) return false;
return true;
}
private static void init(int[][] map2) {
for(int i=0; i<N; i++) {
Arrays.fill(map[i], -1);
}
}
private static int[][] copyMap(int[][] map) {
int[][] map1 = new int[N][N];
for(int i=0; i<N; i++) {
System.arraycopy(map[i], 0, map1[i], 0, N);
}
return map1;
}
private static void blank() {
boolean ok = false;
for(int i=1; i<N; i++) {
for(int j=1; j<N; j++) {
if(map[i][j] == 0) {
if(ok == false) {
x1 = i;
y1 = j;
ok = true;
} else {
x2 = i;
y2 = j;
return ;
}
}
}
}
}
}
class Node3095_4 {
int count;
int[][] map = new int[7][7];
int x1, y1;
int x2, y2;
public Node3095_4() {
}
public Node3095_4(int count, int[][] map, int x1, int y1, int x2, int y2) {
this.count = count;
this.map = map;
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
}
@Override
public boolean equals(Object obj) {
int[][] map1 = ((Node3095_4)obj).map;
for(int i=1; i<7; i++ ) {
for(int j=1; j<7; j++) {
if(map[i][j]!=map1[i][j]){
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
StringBuffer sb = new StringBuffer();
sb.append(map[1][3]);
for(int i=2; i<5; i++) {
sb.append(map[2][i]);
}
for(int i=1; i<6; i++) {
sb.append(map[3][i]);
}
for(int i=2; i<5; i++) {
sb.append(map[4][i]);
}
sb.append(map[5][3]);
return sb.toString().hashCode();
}
}
/**
3
2
1 0 3
4 5 6 7 8
9 0 11
10
0
1 2 3
4 5 6 7 8
9 10 11
0
0
11 10 9
8 7 6 5 4
3 2 1
0
*/