#include <iostream>
struct Node {
int value;
Node *next;
Node(int data) : value(data), next(nullptr) {};
};
void print(Node * head);
Node * delKValueNode(Node * head, int k);
int main()
{
Node n1(1);
Node n2(2);
Node n3(3);
Node n4(1);
Node n5(9);
Node n6(1);
Node n7(2);
Node n8(7);
n1.next = &n2;
n2.next = &n3;
n3.next = &n4;
n4.next = &n5;
n5.next = &n6;
n6.next = &n7;
n7.next = &n8;
print(delKValueNode(&n1, 7));
system("pause");
return 0;
}
void print(Node *head) //打印函数
{
Node *cur = head;
while (cur != nullptr)
{
std::cout << cur->value << std::endl;
cur = cur->next;
}
}
Node * delKValueNode(Node * head, int k) //删除值为k的结点
{
if (head == nullptr)
{
return head;
}
Node *cur = head, *newHead = nullptr;
while (cur != nullptr) //寻找新的头结点
{
if (cur->value != k)
{
C++删除单链表值为K的结点空间复杂度O(1)
最新推荐文章于 2024-05-18 17:15:41 发布
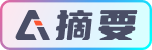