Cactus is a connected undirected graph in which every edge lies on at most one simple cycle. Intuitively cactus is a generalization of a tree where some cycles are allowed. Your task first is to verify if the given graph is a cactus or not. Important difference between a cactus and a tree is that a cactus can have a number of spanning subgraphs that are also cactuses. The number of such subgraphs (including the graph itself ) determines cactusness of a graph (this number is one for a cactus that is just a tree). The cactusness of a graph that is not a cactus is considered to be zero.
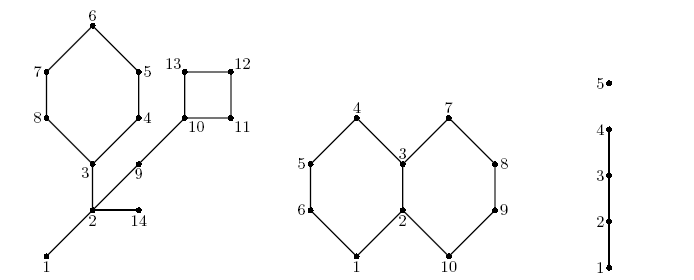
The first graph on the picture is a cactus with cactusness 35. The second graph is not a cactus because edge (2, 3) lies on two cycles. The third graph is not a cactus because it is not connected.
Input
The input will contain several test cases, each of them as described below. Consecutive test cases are separated by a single blank line.
The first line of the input contains two integer numbers n and m (1n
20000, 0
m
1000) . Here n is the number of vertices in the graph. Vertices are numbered from 1 to n . Edges of the graph are represented by a set of edge-distinct paths, where m is the number of such paths.
Each of the following m lines contains a path in the graph. A path starts with an integer number ki (2ki
1000) followed by ki integers from 1 to n . These ki integers represent vertices of a path. Path can go to the same vertex multiple times, but every edge is traversed exactly once in the whole input file. There are no multiedges in the graph (there is at most one edge between any two vertices).
Output
For each test case, the output must follow the description below. The outputs of two consecutive cases will be separated by a blank line.
Write to the output file a single integer number - the cactusness of the given graph. Note that cactusness can be quite a large number.
Sample Input
14 3 9 1 2 3 4 5 6 7 8 3 7 2 9 10 11 12 13 10 2 2 14 10 2 7 1 2 3 4 5 6 1 6 3 7 8 9 10 2 5 1 4 1 2 3 4
Sample Output
35 0 0
#include<iostream> #include<vector> #include<stack> #include<cstring> #include<string.h> #include<cstdio> #include<set> using namespace std; #define mod 10000 const int maxn = 20000+5; vector<int> G[maxn]; bool vis[maxn]; int step[maxn]; int pos[maxn]; //bool g[maxn][maxn]; int S[maxn] , c; int n , m , k , u , v; struct Edge { int u , v; Edge(int uu = -1 ,int vv = -1) : u(uu) , v(vv) { } }tmp; inline bool operator < (const Edge & e1 , const Edge & e2) { if (e1.u==e2.u) return e1.v < e2.v; return e1.u < e2.u; } inline bool operator == (const Edge & e1 , const Edge & e2) { return e1.u==e2.u && e1.v==e2.v; } class Bignum { private: int val[5000]; int sz; public: Bignum() { memset(val,0,sizeof(val)); sz = 0; } Bignum(int x) { memset(val,0,sizeof(val)); sz = 0; while (x) { val[sz++] = x % mod ; x /= mod; } } Bignum(const Bignum & bn) { memset(val,0,sizeof(val)); sz = bn.sz; for (int i = 0 ; i < sz ; ++i) val[i] = bn.val[i]; } Bignum & operator = (const Bignum & bn) { memset(val,0,sizeof(val)); sz = bn.sz; for (int i = 0 ; i < sz ; ++i) val[i] = bn.val[i]; return *this; } Bignum operator * (int x) { Bignum ret; Bignum tmp(x); for (int i = 0 ; i < sz ; ++i) { for (int j = 0 ; j < tmp.sz ; ++j) { ret.val[i+j] += tmp.val[j]*val[i]; ret.val[i+j+1] += ret.val[i+j] / mod; ret.val[i+j] %= mod; } } ret.sz = tmp.sz + sz; while (ret.val[ret.sz]) { ret.val[ret.sz+1] += ret.val[ret.sz] / mod; ret.val[ret.sz] %= mod; ++ret.sz; } while (ret.sz > 0 && ret.val[ret.sz-1]==0) --ret.sz; return ret; } void print() { printf("%d",val[--sz]); --sz; while (sz >= 0) { if (val[sz] < 10) printf("000%d",val[sz]); else if (val[sz] < 100) printf("00%d",val[sz]); else if (val[sz] < 1000) printf("0%d",val[sz]); else printf("%d",val[sz]); --sz; } cout << endl; } }ans; set<Edge> edge; stack<Edge> DFS; void init() { c = 0; ans = 1; while (DFS.size()) DFS.pop(); for (int i = 1 ; i <= n ; ++i) { vis[i] = false; G[i].clear(); } edge.clear(); } void input() { while (m--) { scanf("%d%d",&k,&u); while (--k) { scanf("%d",&v); G[u].push_back(v); G[v].push_back(u); pos[u] = pos[v] = step[u] = step[v] = -1; //g[u][v] = g[v][u] = false; u = v; } } } bool dfs(int rt) { DFS.push(Edge(-1,rt)); step[rt] = 0; int edge_cnt = 0; while (DFS.size()) { tmp = DFS.top(); u = tmp.u , v = tmp.v; if (u==-1 && vis[v]) break; if (!vis[v]) { if (u > 0) step[v] = step[u]+1; vis[v] = true; S[c++] = v; bool isleaf = true; for (int i = 0 ; i < G[v].size() ; ++i) { int y = G[v][i]; if (step[y]==-1 || abs(step[y]-step[v])!=1) { isleaf = false; if (pos[v]==-1) pos[v] = DFS.size(); DFS.push(Edge(v,y)); } } if (isleaf) { --c; DFS.pop(); } } else { if (step[u] <= step[v]) { DFS.pop(); while (c > 0 && pos[S[c-1]] > DFS.size()) --c; continue; } ans = ans*(step[u]-step[v]+2); int x = v , y; for (int i = c-1 ; i >= 0 ; --i) { y = S[i]; // if (g[x][y]) return false; //g[x][y] = g[y][x] = true; edge.insert(Edge(min(x,y),max(x,y))); ++edge_cnt; if (edge.size()!=edge_cnt) return false; x = y; if (S[i]==v) break; } DFS.pop(); while (c > 0 && pos[S[c-1]] > DFS.size()) --c; } } for (int i = 1; i <= n ; ++i) if (!vis[i]) return false; return true; } void solve() { if (!dfs(1)) cout << 0 << endl; else ans.print(); } int main() { //freopen("input.in","r",stdin); while (scanf("%d%d",&n,&m)==2) { init(); input(); solve(); } }