题目
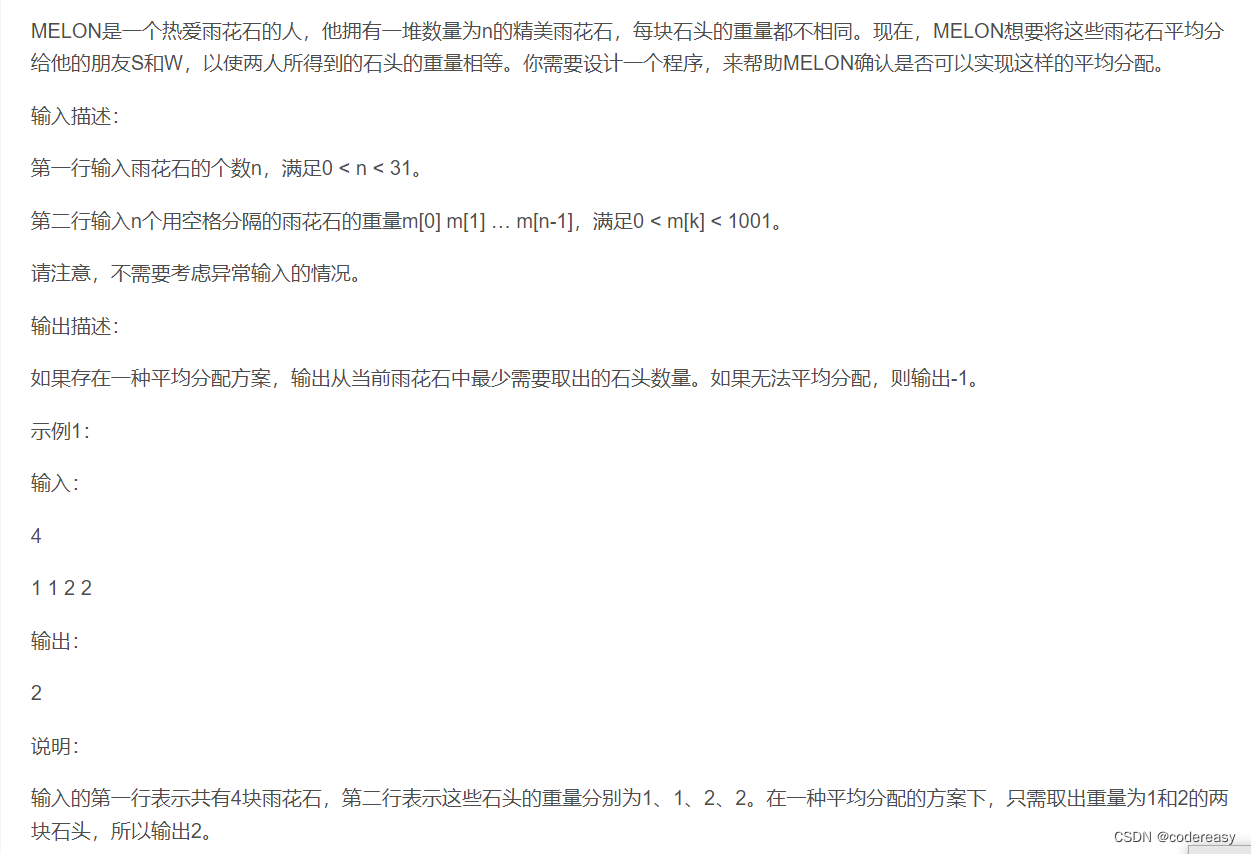
代码
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int checkSplit(int stoneCount, vector<int>& stoneWeights) {
int totalWeight = 0;
for (int weight : stoneWeights) {
totalWeight += weight;
}
if (totalWeight % 2 != 0) {
return -1;
}
int halfWeight = totalWeight / 2;
vector<vector<int>> dpTable(stoneCount + 1, vector<int>(halfWeight + 1, stoneCount));
dpTable[0][0] = 0;
for (int i = 1; i <= stoneCount; i++) {
int currentWeight = stoneWeights[i - 1];
for (int j = 0; j <= halfWeight; j++) {
if (j < currentWeight) {
dpTable[i][j] = dpTable[i - 1][j];
} else {
int option1 = dpTable[i - 1][j];
int option2 = dpTable[i - 1][j - currentWeight] + 1;
dpTable[i][j] = min(option1, option2);
}
}
}
if (dpTable[stoneCount][halfWeight] == stoneCount) {
return -1;
}
return min(stoneCount - dpTable[stoneCount][halfWeight], dpTable[stoneCount][halfWeight]);
}
int main() {
int stoneCount;
cin >> stoneCount;
vector<int> stoneWeights(stoneCount);
for (int i = 0; i < stoneCount; i++) {
cin >> stoneWeights[i];
}
int result = checkSplit(stoneCount, stoneWeights);
cout << result << endl;
return 0;
}