工具类:EditEntityTool.cs
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.Geometry;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using AcDotNetTool;
namespace _03编辑图形
{
public static partial class EditEntityTool
{
public static void ChangeEntityColor(this ObjectId c1Id, short colorIndex)
{
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForRead);
Entity ent1 = (Entity)c1Id.GetObject(OpenMode.ForWrite);
ent1.ColorIndex = colorIndex;
trans.Commit();
}
}
public static void ChangeEntityColor(this Entity ent, short colorIndex)
{
if (ent.IsNewObject)
{
ent.ColorIndex = colorIndex;
}
else
{
ent.ObjectId.ChangeEntityColor(colorIndex);
}
}
public static void MoveEntity(this ObjectId entId, Point3d sourcePoint, Point3d targetPoint)
{
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForRead);
Entity ent = (Entity)entId.GetObject(OpenMode.ForWrite);
Vector3d vector = sourcePoint.GetVectorTo(targetPoint);
Matrix3d mt = Matrix3d.Displacement(vector);
ent.TransformBy(mt);
trans.Commit();
}
}
public static void MoveEntity(this Entity ent, Point3d sourcePoint, Point3d targetPoint)
{
if (ent.IsNewObject)
{
Vector3d vector = sourcePoint.GetVectorTo(targetPoint);
Matrix3d mt = Matrix3d.Displacement(vector);
ent.TransformBy(mt);
}
else
{
ent.ObjectId.MoveEntity(sourcePoint, targetPoint);
}
}
public static Entity CopyEntity(this ObjectId entId, Point3d sourcePoint, Point3d targetPoint)
{
Entity entR;
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForRead);
Entity ent = (Entity)entId.GetObject(OpenMode.ForWrite);
Vector3d vector = sourcePoint.GetVectorTo(targetPoint);
Matrix3d mt = Matrix3d.Displacement(vector);
entR = ent.GetTransformedCopy(mt);
trans.Commit();
}
return entR;
}
public static Entity CopyEntity(this Entity ent, Point3d sourcePoint, Point3d targetPoint)
{
Entity entR;
if (ent.IsNewObject)
{
Vector3d vector = sourcePoint.GetVectorTo(targetPoint);
Matrix3d mt = Matrix3d.Displacement(vector);
entR = ent.GetTransformedCopy(mt);
}
else
{
entR = ent.ObjectId.CopyEntity(sourcePoint, targetPoint);
}
return entR;
}
public static void RotateEntity(this ObjectId entId, Point3d center,double degree)
{
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForRead);
Entity ent = (Entity)entId.GetObject(OpenMode.ForWrite);
Matrix3d mt = Matrix3d.Rotation(degree.DegreeToAngle(), Vector3d.ZAxis, center);
ent.TransformBy(mt);
trans.Commit();
}
}
public static void RotateEntity(this Entity ent, Point3d center, double degree)
{
if (ent.IsNewObject)
{
Matrix3d mt = Matrix3d.Rotation(degree.DegreeToAngle(), Vector3d.ZAxis, center);
ent.TransformBy(mt);
}
else
{
ent.ObjectId.RotateEntity(center, degree);
}
}
public static Entity MirrorEntity(this ObjectId entId, Point3d point1, Point3d point2, bool isEraseSoruce)
{
Entity entR;
Matrix3d mt = Matrix3d.Mirroring(new Line3d(point1, point2));
using (Transaction trans = entId.Database.TransactionManager.StartTransaction())
{
Entity ent = (Entity)trans.GetObject(entId, OpenMode.ForWrite);
entR = ent.GetTransformedCopy(mt);
if (isEraseSoruce)
{
ent.Erase();
}
trans.Commit();
}
return entR;
}
public static Entity MirrorEntity(this Entity ent, Point3d point1, Point3d point2, bool isEraseSoruce)
{
Entity entR;
if (ent.IsNewObject == true)
{
Matrix3d mt = Matrix3d.Mirroring(new Line3d(point1, point2));
entR = ent.GetTransformedCopy(mt);
}
else
{
entR = ent.ObjectId.MirrorEntity(point1, point2, isEraseSoruce);
}
return entR;
}
public static void ScaleEntity(this ObjectId entId, Point3d basePoint, double facter)
{
Matrix3d mt = Matrix3d.Scaling(facter, basePoint);
using (Transaction trans = entId.Database.TransactionManager.StartTransaction())
{
Entity ent = (Entity)entId.GetObject(OpenMode.ForWrite);
ent.TransformBy(mt);
trans.Commit();
}
}
public static void ScaleEntity(this Entity ent, Point3d basePoint, double facter)
{
if (ent.IsNewObject == true)
{
Matrix3d mt = Matrix3d.Scaling(facter, basePoint);
ent.TransformBy(mt);
}
else
{
ent.ObjectId.ScaleEntity(basePoint, facter);
}
}
public static void EraseEntity(this ObjectId entId)
{
using (Transaction trans = entId.Database.TransactionManager.StartTransaction())
{
Entity ent = (Entity)entId.GetObject(OpenMode.ForWrite);
ent.Erase();
trans.Commit();
}
}
public static List<Entity> ArrayRectEntity(this ObjectId entId,int rowNum,int columnNum,double disRow,double disColumn)
{
List<Entity> entList = new List<Entity>();
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
Entity ent = (Entity)entId.GetObject(OpenMode.ForWrite);
for (int i = 0; i < rowNum; i++)
{
for (int j = 0; j < columnNum; j++)
{
Matrix3d mt = Matrix3d.Displacement(new Vector3d(j * disColumn, i * disRow, 0));
Entity entA = ent.GetTransformedCopy(mt);
btr.AppendEntity(entA);
trans.AddNewlyCreatedDBObject(entA,true);
entList.Add(entA);
}
}
ent.Erase();
trans.Commit();
}
return entList;
}
public static List<Entity> ArrayRectEntity(this Entity entS, int rowNum, int columnNum, double disRow, double disColumn)
{
if (entS.IsNewObject == true)
{
List<Entity> entList = new List<Entity>();
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
for (int i = 0; i < rowNum; i++)
{
for (int j = 0; j < columnNum; j++)
{
Matrix3d mt = Matrix3d.Displacement(new Vector3d(j * disColumn, i * disRow, 0));
Entity entA = entS.GetTransformedCopy(mt);
btr.AppendEntity(entA);
trans.AddNewlyCreatedDBObject(entA, true);
entList.Add(entA);
}
}
trans.Commit();
}
return entList;
}
else
{
return entS.ArrayRectEntity(rowNum, columnNum, disRow, disColumn);
}
}
public static List<Entity> ArrayPolarEntity(this ObjectId entId, int num, double degree,Point3d center)
{
List<Entity> entList = new List<Entity>();
using (Transaction trans = entId.Database.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(entId.Database.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
Entity ent = (Entity)entId.GetObject(OpenMode.ForWrite);
degree = degree > 360 ? 360 : degree;
degree = degree < -360 ? -360 : degree;
int divAngNum = num-1;
if (degree == 360 || degree == -360)
{
divAngNum = num;
}
for (int i = 0; i < num; i++)
{
Matrix3d mt = Matrix3d.Rotation((i * degree / divAngNum).DegreeToAngle(), Vector3d.ZAxis, center);
Entity entA = ent.GetTransformedCopy(mt);
btr.AppendEntity(entA);
trans.AddNewlyCreatedDBObject(entA, true);
entList.Add(entA);
}
ent.Erase();
trans.Commit();
}
return entList;
}
public static List<Entity> ArrayPolarEntity(this Entity ent, int num, double degree, Point3d center)
{
if (ent.IsNewObject == true)
{
List<Entity> entList = new List<Entity>();
Database db = HostApplicationServices.WorkingDatabase;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
degree = degree > 360 ? 360 : degree;
degree = degree < -360 ? -360 : degree;
int divAngNum = num - 1;
if (degree == 360 || degree == -360)
{
divAngNum = num;
}
for (int i = 0; i < num; i++)
{
Matrix3d mt = Matrix3d.Rotation((i * degree / divAngNum).DegreeToAngle(), Vector3d.ZAxis, center);
Entity entA = ent.GetTransformedCopy(mt);
btr.AppendEntity(entA);
trans.AddNewlyCreatedDBObject(entA, true);
entList.Add(entA);
}
trans.Commit();
}
return entList;
}
else
{
return ent.ObjectId.ArrayPolarEntity(num, degree, center);
}
}
}
}
改变图形颜色
[CommandMethod("EditDemo")]
public void EditDemo()
{
Database db = HostApplicationServices.WorkingDatabase;
Circle c1 = new Circle(new Point3d(100, 100, 0), new Vector3d(0, 0, 1), 50);
Circle c2 = new Circle(new Point3d(200, 100, 0), new Vector3d(0, 0, 1), 50);
db.AddEntityToModelSpace(c1);
c1.ChangeEntityColor(1);
c2.ChangeEntityColor(6);
db.AddEntityToModelSpace(c2);
}
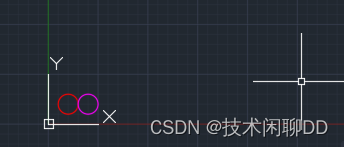
移动图形
[CommandMethod("MoveDemo")]
public void MoveDemo()
{
Database db = HostApplicationServices.WorkingDatabase;
Circle c1 = new Circle(new Point3d(100, 100, 0), new Vector3d(0, 0, 1), 50);
Circle c2 = new Circle(new Point3d(100, 100, 0), new Vector3d(0, 0, 1), 50);
Circle c3 = new Circle(new Point3d(100, 100, 0), new Vector3d(0, 0, 1), 50);
Point3d p1 = new Point3d(100, 100, 0);
Point3d p2 = new Point3d(200, 300, 0);
db.AddEnityToModelSpace(c1,c2);
c2.MoveEntity(p1, p2);
c3.MoveEntity(p2, p1);
db.AddEnityToModelSpace(c3);
}
## 复制图形
```csharp
[CommandMethod("CopyDemo")]
public void CopyDemo()
{
Database db = HostApplicationServices.WorkingDatabase;
Circle c1 = new Circle(new Point3d(100, 100, 0), Vector3d.ZAxis, 100);
Circle c2 = (Circle)c1.CopyEntity(new Point3d(100, 100, 0), new Point3d(100, 200, 0));
db.AddEnityToModelSpace(c1,c2);
Circle c3 = (Circle)c1.CopyEntity(new Point3d(0, 0, 0), new Point3d(-100, 0, 0));
db.AddEnityToModelSpace(c3);
}
旋转图形
[CommandMethod("RotateDemo")]
public void RotateDemo()
{
Database db = HostApplicationServices.WorkingDatabase;
Line l1 = new Line(new Point3d(100, 100, 0), new Point3d(300, 100, 0));
Line l2 = new Line(new Point3d(100, 100, 0), new Point3d(300, 100, 0));
Line l3 = new Line(new Point3d(100, 100, 0), new Point3d(300, 100, 0));
l1.RotateEntity(new Point3d(100, 100, 0), 30);
db.AddEnityToModelSpace(l1,l2,l3);
l2.RotateEntity(new Point3d(0, 0, 0), 60);
l3.RotateEntity(new Point3d(200, 500, 0), 90);
}
镜像图形
[CommandMethod("MirrorDemo1")]
public void MirrorDemo1()
{
Database db = HostApplicationServices.WorkingDatabase;
Circle c1 = new Circle(new Point3d(100, 100, 0), Vector3d.ZAxis, 50);
ObjectId cId = db.AddEnityToModelSpace(c1);
Entity Ent = cId.MirrorEntity(new Point3d(200, 100, 0), new Point3d(200, 300, 0), true);
db.AddEnityToModelSpace(Ent);
Circle c2 = new Circle(new Point3d(100, 200, 0), Vector3d.ZAxis, 50);
Entity ent2 = c2.MirrorEntity(new Point3d(200, 100, 0), new Point3d(200, 300, 0), true);
db.AddEnityToModelSpace(c2,ent2);
}
缩放图形
[CommandMethod("ScaleDemo")]
public void ScaleDemo()
{
Database db = HostApplicationServices.WorkingDatabase;
Circle c1 = new Circle(new Point3d(100, 100, 0), Vector3d.ZAxis, 50);
db.AddEnityToModelSpace(c1);
Circle c2 = new Circle(new Point3d(200, 100, 0), Vector3d.ZAxis, 50);
c2.ScaleEntity(new Point3d(200, 100, 0), 0.5);
db.AddEnityToModelSpace(c2);
Circle c3 = new Circle(new Point3d(300, 100, 0), Vector3d.ZAxis, 50);
db.AddEnityToModelSpace(c3);
c3.ScaleEntity(new Point3d(0, 0, 0), 2);
}
删除图形对象
[CommandMethod("EraseDemo")]
public void EraseDemo()
{
Database db = HostApplicationServices.WorkingDatabase;
Circle c1 = new Circle(new Point3d(100, 100, 0), Vector3d.ZAxis, 50);
Circle c2 = new Circle(new Point3d(200, 100, 0), Vector3d.ZAxis, 50);
db.AddEnityToModelSpace(c1, c2);
c2.ObjectId.EraseEntity();
}
矩形阵列图形
[CommandMethod("ArrayRectDemo1")]
public void ArrayRectDemo1()
{
Database db = HostApplicationServices.WorkingDatabase;
Circle c = new Circle(new Point3d(100, 100, 0), Vector3d.ZAxis, 10);
c.ArrayRectEntity(5, 6, -50, 50);
}
环形阵列图形
[CommandMethod("ArrayPolarDemo")]
public void ArrayPolarDemo()
{
Database db = HostApplicationServices.WorkingDatabase;
Line l = new Line(new Point3d(100, 100, 0), new Point3d(120, 120, 0));
l.ArrayPolarEntity(7, -270, new Point3d(110, 300, 0));
}