http://user.qzone.qq.com/892054726/blog/1439803064
http://dev.mysql.com/doc/refman/5.6/en/optimize-overview.html
8.1 Optimization Overview
Optimizing at the Database Level
The most important factor in making a database application fast is its basic design:
-
Are the tables structured properly? In particular, do the columns have the right data types, and does each table have the appropriate columns for the type of work? For example, applications that perform frequent updates often have many tables with few columns, while applications that analyze large amounts of data often have few tables with many columns.
-
Are the right indexes in place to make queries efficient?
-
Are you using the appropriate storage engine for each table, and taking advantage of the strengths and features of each storage engine you use? In particular, the choice of a transactional storage engine such as
InnoDB
or a nontransactional one such asMyISAM
can be very important for performance and scalability.NoteIn MySQL 5.5 and higher,
InnoDB
is the default storage engine for new tables. In practice, the advancedInnoDB
performance features mean thatInnoDB
tables often outperform the simplerMyISAM
tables, especially for a busy database. -
Does each table use an appropriate row format? This choice also depends on the storage engine used for the table. In particular, compressed tables use less disk space and so require less disk I/O to read and write the data. Compression is available for all kinds of workloads with
InnoDB
tables, and for read-onlyMyISAM
tables. -
Does the application use an appropriate locking strategy? For example, by allowing shared access when possible so that database operations can run concurrently, and requesting exclusive access when appropriate so that critical operations get top priority. Again, the choice of storage engine is significant. The
InnoDB
storage engine handles most locking issues without involvement from you, allowing for better concurrency in the database and reducing the amount of experimentation and tuning for your code. -
Are all memory areas used for caching sized correctly? That is, large enough to hold frequently accessed data, but not so large that they overload physical memory and cause paging. The main memory areas to configure are the
InnoDB
buffer pool, theMyISAM
key cache, and the MySQL query cache.
Optimizing at the Hardware Level
The way to optimize seek time is to distribute the data onto more than one disk.
-
Disk reading and writing. When the disk is at the correct position, we need to read or write the data. With modern disks, one disk delivers at least 10–20MB/s throughput. This is easier to optimize than seeks because you can read in parallel from multiple disks.
-
When the data is in main memory, we must process it to get our result. Having large tables compared to the amount of memory is the most common limiting factor. But with small tables, speed is usually not the problem.
Balancing Portability and Performance
To use performance-oriented SQL extensions in a portable MySQL program, you can wrap MySQL-specific keywords in a statement within /*! */
comment delimiters. Other SQL servers ignore the commented keywords. For information about writing comments, see Section 9.6, “Comment Syntax”.
8.2 Optimizing SQL Statements
Disk reading and writing. When the disk is at the correct position, we need to read or write the data. With modern disks, one disk delivers at least 10–20MB/s throughput. This is easier to optimize than seeks because you can read in parallel from multiple disks.
When the data is in main memory, we must process it to get our result. Having large tables compared to the amount of memory is the most common limiting factor. But with small tables, speed is usually not the problem.
8.2.1.1 Speed of SELECT Statements
To avoid wasted disk space, construct a small set of indexes that speed up many related queries used in your application.
Indexes are especially important for queries that reference different tables, using features such as joins and foreign keys. You can use the EXPLAIN
statement to determine which indexes are used for a SELECT
Learn the tuning techniques, indexing techniques, and configuration parameters that are specific to the storage engine for each table. Both InnoDB and MyISAM have sets of guidelines for enabling and sustaining high performance in queries. For details, see Section 8.5.6, “Optimizing InnoDB Queries” and Section 8.6.1, “Optimizing MyISAM Queries”.
8.2.1.2 How MySQL Optimizes WHERE Clauses
-
In some cases, MySQL can read rows from the index without even consulting the data file. If all columns used from the index are numeric, only the index tree is used to resolve the query.
-
8.2.2 Optimizing DML Statements
To optimize insert speed, combine many small operations into a single large operation. Ideally, you make a single connection, send the data for many new rows at once, and delay all index updates and consistency checking until the very end.
In some cases, MySQL can read rows from the index without even consulting the data file. If all columns used from the index are numeric, only the index tree is used to resolve the query.
8.2.2 Optimizing DML Statements
The time required for inserting a row is determined by the following factors, where the numbers indicate approximate proportions:
-
Connecting: (3)
-
Sending query to server: (2)
-
Parsing query: (2)
-
Inserting row: (1 × size of row)
-
Inserting indexes: (1 × number of indexes)
-
Closing: (1)
This does not take into consideration the initial overhead to open tables, which is done once for each concurrently running query.
The size of the table slows down the insertion of indexes by log N
, assuming B-tree indexes.
You can use the following methods to speed up inserts:
-
If you are inserting many rows from the same client at the same time, use
INSERT
statements with multipleVALUES
lists to insert several rows at a time. This is considerably faster (many times faster in some cases) than using separate single-rowINSERT
statements. If you are adding data to a nonempty table, you can tune thebulk_insert_buffer_size
variable to make data insertion even faster. See Section 5.1.4, “Server System Variables”. -
When loading a table from a text file, use
LOAD DATA INFILE
. This is usually 20 times faster than usingINSERT
statements. See Section 13.2.6, “LOAD DATA INFILE Syntax”. -
Take advantage of the fact that columns have default values. Insert values explicitly only when the value to be inserted differs from the default. This reduces the parsing that MySQL must do and improves the insert speed.
-
See Section 8.5.5, “Bulk Data Loading for InnoDB Tables” for tips specific to
InnoDB
tables. -
See Section 8.6.2, “Bulk Data Loading for MyISAM Tables” for tips specific to
MyISAM
tables. -
-
MySQLDump 导出的数据备份文件中,插入语句如下:
LOCK TABLES `cargo_type` WRITE;
/*!40000 ALTER TABLE `cargo_type` DISABLE KEYS */;
INSERT INTO `cargo_type` VALUES (1,'a','a'),(2,'b','b'),(3,'c','c'),(4,'d','d');
/*!40000 ALTER TABLE `cargo_type` ENABLE KEYS */;
UNLOCK TABLES;
8.2.2.2 Speed of UPDATE Statements
Another way to get fast updates is to delay updates and then do many updates in a row later. Performing multiple updates together is much quicker than doing one at a time if you lock the table.
8.2.3 Optimizing Database Privileges
The more complex your privilege setup, the more overhead applies to all SQL statements. Simplifying the privileges established by GRANT statements enables MySQL to reduce permission-checking overhead when clients execute statements. For example, if you do not grant any table-level or column-level privileges, the server need not ever check the contents of the tables_priv and columns_priv tables. Similarly, if you place no resource limits on any accounts, the server does not have to perform resource counting. If you have a very high statement-processing load, consider using a simplified grant structure to reduce permission-checking overhead.
8.2.5 Other Optimization Tips
If your application makes several database requests to perform related updates, combining the statements into a stored routine can help performance. Similarly, if your application computes a single result based on several column values or large volumes of data, combining the computation into a UDF (user-defined function) can help performance.
If possible, classify reports as “live” or as “statistical”, where data needed for statistical reports is created only from summary tables that are generated periodically from the live data.
If you have data that does not conform well to a rows-and-columns table structure, you can pack and store data into a BLOB column. In this case, you must provide code in your application to pack and unpack information, but this might save I/O operations to read and write the sets of related values.
With Web servers, store images and other binary assets as files, with the path name stored in the database rather than the file itself. Most Web servers are better at caching files than database contents, so using files is generally faster. (Although you must handle backups and storage issues yourself in this case.)
If you need really high speed, look at the low-level MySQL interfaces. For example, by accessing the MySQL InnoDB or MyISAM storage engine directly, you could get a substantial speed increase compared to using the SQL interface.
Replication can provide a performance benefit for some operations. You can distribute client retrievals among replication servers to split up the load. To avoid slowing down the master while making backups, you can make backups using a slave server. See Chapter 17, Replication.
8.3.1 How MySQL Uses Indexes
In some cases, a query can be optimized to retrieve values without consulting the data rows. (An index that provides all the necessary results for a query is called a covering index.) If a query uses from a table only columns that are included in some index, the selected values can be retrieved from the index tree for greater speed:
SELECT key_part3
FROM tbl_name
WHERE key_part1
=1
When a query needs to access most of the rows, reading sequentially is faster than working through an index. Sequential reads minimize disk seeks, even if not all the rows are needed for the query.
8.3.2 Using Primary Keys
The primary key for a table represents the column or set of columns that you use in your most vital queries. Query performance benefits from the NOT NULL
optimization, because it cannot include any NULL
values. With the InnoDB
storage engine, the table data is physically organized to do ultra-fast lookups and sorts based on the primary key column or columns.
If your table is big and important, but does not have an obvious column or set of columns to use as a primary key, you might create a separate column with auto-increment values to use as the primary key. These unique IDs can serve as pointers to corresponding rows in other tables when you join tables using foreign keys.
8.3.3 Using Foreign Keys
If a table has many columns, and you query many different combinations of columns, it might be efficient to split the less-frequently used data into separate tables with a few columns each, and relate them back to the main table by duplicating the numeric ID column from the main table. That way, each small table can have a primary key for fast lookups of its data, and you can query just the set of columns that you need using a join operation. Depending on how the data is distributed, the queries might perform less I/O and take up less cache memory because the relevant columns are packed together on disk. (To maximize performance, queries try to read as few data blocks as possible from disk; tables with only a few columns can fit more rows in each data block.)
8.4 Optimizing Database Structure
8.4.1 Optimizing Data Size
Design your tables to minimize their space on the disk.
Table Columns
-
Use the most efficient (smallest) data types possible. MySQL has many specialized types that save disk space and memory. For example, use the smaller integer types if possible to get smaller tables. MEDIUMINT
is often a better choice than INT
because a MEDIUMINT
column uses 25% less space.
-
Declare columns to be NOT NULL
if possible. It makes SQL operations faster, by enabling better use of indexes and eliminating overhead for testing whether each value is NULL
. You also save some storage space, one bit per column. If you really need NULL
values in your tables, use them. Just avoid the default setting that allows NULL
values in every column.
-
Use the most efficient (smallest) data types possible. MySQL has many specialized types that save disk space and memory. For example, use the smaller integer types if possible to get smaller tables.
MEDIUMINT
is often a better choice thanINT
because aMEDIUMINT
column uses 25% less space. -
Declare columns to be
NOT NULL
if possible. It makes SQL operations faster, by enabling better use of indexes and eliminating overhead for testing whether each value isNULL
. You also save some storage space, one bit per column. If you really needNULL
values in your tables, use them. Just avoid the default setting that allowsNULL
values in every column.
Indexes
-
The primary index of a table should be as short as possible. This makes identification of each row easy and efficient. For InnoDB
tables, the primary key columns are duplicated in each secondary index entry, so a short primary key saves considerable space if you have many secondary indexes.
The primary index of a table should be as short as possible. This makes identification of each row easy and efficient. For InnoDB
tables, the primary key columns are duplicated in each secondary index entry, so a short primary key saves considerable space if you have many secondary indexes.
Joins
-
-
In some circumstances, it can be beneficial to split into two a table that is scanned very often. This is especially true if it is a dynamic-format table and it is possible to use a smaller static format table that can be used to find the relevant rows when scanning the table.
Normalization
-
If speed is more important than disk space and the maintenance costs of keeping multiple copies of data, for example in a business intelligence scenario where you analyze all the data from large tables, you can relax the normalization rules, duplicating information or creating summary tables to gain more speed.
-
In some circumstances, it can be beneficial to split into two a table that is scanned very often. This is especially true if it is a dynamic-format table and it is possible to use a smaller static format table that can be used to find the relevant rows when scanning the table.
If speed is more important than disk space and the maintenance costs of keeping multiple copies of data, for example in a business intelligence scenario where you analyze all the data from large tables, you can relax the normalization rules, duplicating information or creating summary tables to gain more speed.
8.4.3 Optimizing for Many Tables
Some techniques for keeping individual queries fast involve splitting data across many tables. When the number of tables runs into the thousands or even millions, the overhead of dealing with all these tables becomes a new performance consideration.
8.4.3.1 How MySQL Opens and Closes Tables
To minimize the problem with multiple client sessions having different states on the same table, the table is opened independently by each concurrent session. This uses additional memory but normally increases performance.
The table_open_cache
and max_connections
system variables affect the maximum number of files the server keeps open. If you increase one or both of these values, you may run up against a limit imposed by your operating system on the per-process number of open file descriptors.
table_open_cache
is related to max_connections
. For example, for 200 concurrent running connections, specify a table cache size of at least 200 * N
, where N
is the maximum number of tables per join in any of the queries which you execute. You must also reserve some extra file descriptors for temporary tables and files.
Make sure that your operating system can handle the number of open file descriptors implied by thetable_open_cache
setting. If table_open_cache
is set too high, MySQL may run out of file descriptors and refuse connections, fail to perform queries, and be very unreliable.
N
8.4.3.2 Disadvantages of Creating Many Tables in the Same Database
If you have many MyISAM
tables in the same database directory, open, close, and create operations are slow. If you execute SELECT
statements on many different tables, there is a little overhead when the table cache is full, because for every table that has to be opened, another must be closed. You can reduce this overhead by increasing the number of entries permitted in the table cache.
8.5 Optimizing for InnoDB Tables
8.5.1 Optimizing Storage Layout for InnoDB Tables
Once your data reaches a stable size, or a growing table has increased by tens or some hundreds of megabytes, consider using the OPTIMIZE TABLE
statement to reorganize the table and compact any wasted space. OPTIMIZE TABLE
copies the data part of the table and rebuilds the indexes.
In InnoDB
, having a long PRIMARY KEY
(either a single column with a lengthy value, or several columns that form a long composite value) wastes a lot of disk space. The primary key value for a row is duplicated in all the secondary index records that point to the same row.
For tables that are big, or contain lots of repetitive text or numeric data, consider using COMPRESSED
row format.
8.5.2 Optimizing InnoDB Transaction Management
Application might encounter performance issues if it commits thousands of times per second, and different performance issues if it commits only every 2-3 hours.
Avoid performing rollbacks after inserting, updating, or deleting huge numbers of rows. If a big transaction is slowing down server performance, rolling it back can make the problem worse, potentially taking several times as long to perform as the original DML operations. Killing the database process does not help, because the rollback starts again on server startup.
To minimize the chance of this issue occurring:
-
Increase the size of the buffer pool so that all the DML changes can be cached rather than immediately written to disk.
-
Set
innodb_change_buffering=all
so that update and delete operations are buffered in addition to inserts. -
Consider issuing
COMMIT
statements periodically during the big DML operation, possibly breaking a single delete or update into multiple statements that operate on smaller numbers of rows.
innodb_force_recovery=3
, as explained in Section 14.15.1, “The InnoDB Recovery Process”. If you can afford the loss of some of the latest committed transactions if a crash occurs, you can set the
innodb_flush_log_at_trx_commit
parameter to 0. InnoDB
tries to flush the log once per second anyway, although the flush is not guaranteed. Also, set the value of innodb_support_xa
to 0, which will reduce the number of disk flushes due to synchronizing on disk data and the binary log.-
When rows are modified or deleted, the rows and associated undo logs are not physically removed immediately, or even immediately after the transaction commits. The old data is preserved until transactions that started earlier or concurrently are finished, so that those transactions can access the previous state of modified or deleted rows. Thus, a long-running transaction can prevent
InnoDB
from purging data that was changed by a different transaction. -
As of MySQL 5.6.4,
InnoDB
can avoid the overhead associated with setting up the transaction ID (TRX_ID
field) for transactions that are known to be read-only. A transaction ID is only needed for a transaction that might perform write operations or locking reads such as SELECT ... FOR UPDATE
. Eliminating unnecessary transaction IDs reduces the size of internal data structures that are consulted each time a query or DML statement constructs aread view. 8.5.4 Optimizing InnoDB Redo Logging
Make your redo log files big, even as big as the buffer pool. When InnoDB has written the redo log files full, it must write the modified contents of the buffer pool to disk in a checkpoint. Small redo log files cause many unnecessary disk writes. Although historically big redo log files caused lengthy recovery times, recovery is now much faster and you can confidently use large redo log files.
The size and number of redo log files are configured using the innodb_log_file_size and innodb_log_files_in_group configuration options. For information about modifying an existing redo log file configuration, see Section 14.4.2, “Changing the Number or Size of InnoDB Redo Log Files”.
Consider increasing the size of the log_buffer. A large log buffer enables large transactions to run without a need to write the log to disk before the transactions commit. Thus, if you have transactions that update, insert, or delete many rows, making the log buffer larger saves disk I/O. Log buffer size is configured using the innodb_log_buffer_size configuration option.
8.5.5 Bulk Data Loading for InnoDB Tables
When importing data into InnoDB, turn off autocommit mode , because it performs a log flush to disk for every insert.The mysqldump option --opt creates dump files that are fast to import into an InnoDB table, even without wrapping them with the SET autocommit and COMMIT statements.
If you have UNIQUE constraints on secondary keys, you can speed up table imports by temporarily turning off the uniqueness checks during the import session:
If you have FOREIGN KEY constraints in your tables, you can speed up table imports by turning off the foreign key checks for the duration of the import session:
When doing bulk inserts into tables with auto-increment columns, set innodb_autoinc_lock_mode to 2 instead of the default value 1.
8.5.6 Optimizing InnoDB Queries
Specify a set of primary key columns for each table, columns that are used in the most important and time-critical queries.
Do not specify too many or too long columns in the primary key, because these column values are duplicated in each secondary index. When an index contains unnecessary data, the I/O to read this data and memory to cache it reduce the performance and scalability of the server
Do not create a separate secondary index for each column, because each query can only make use of one index. Indexes on rarely tested columns or columns with only a few different values might not be helpful for any queries. If you have many queries for the same table, testing different combinations of columns, try to create a small number of concatenated indexes rather than a large number of single-column indexes. If an index contains all the columns needed for the result set (known as a covering index), the query might be able to avoid reading the table data at all.
-
If you often have recurring queries for tables that are not updated frequently, enable the query cache:
[mysqld] - query_cache_type = 1
- query_cache_size = 10M
If you often have recurring queries for tables that are not updated frequently, enable the query cache:
[mysqld]8.6 Optimizing for MyISAM Tables
The MyISAM
storage engine performs best with read-mostly data or with low-concurrency operations, because table locks limit the ability to perform simultaneous updates.
8.6.1 Optimizing MyISAM Queries
To help MySQL better optimize queries, use ANALYZE TABLE or run myisamchk --analyze on a table after it has been loaded with data. This updates a value for each index part that indicates the average number of rows that have the same value.
MyISAM supports concurrent inserts: If a table has no free blocks in the middle of the data file, you can INSERT new rows into it at the same time that other threads are reading from the table. Another possibility is to run OPTIMIZE TABLE to defragment the table after you have deleted a lot of rows from it.
It is normally not useful to split a table into different tables just because the rows become large. In accessing a row, the biggest performance hit is the disk seek needed to find the first byte of the row.
Use ALTER TABLE ... ORDER BY expr1, expr2, ... if you usually retrieve rows in expr1, expr2, ... order.
If you often need to calculate results such as counts based on information from a lot of rows, it may be preferable to introduce a new table and update the counter in real time.
Use OPTIMIZE TABLE periodically to avoid fragmentation with dynamic-format MyISAM tables.
Declaring a MyISAM table with the DELAY_KEY_WRITE=1 table option makes index updates faster because they are not flushed to disk until the table is closed.
You can increase performance by caching queries or answers in your application and then executing many inserts or updates together. Locking the table during this operation ensures that the index cache is only flushed once after all updates. You can also take advantage of MySQL's query cache to achieve similar results;
8.6.2 Bulk Data Loading for MyISAM Tables
To speed up INSERT operations that are performed with multiple statements for nontransactional tables, lock your tables:
LOCK TABLES a WRITE;
INSERT INTO a VALUES (1,23),(2,34),(4,33);
INSERT INTO a VALUES (8,26),(6,29);
...
UNLOCK TABLES;
This benefits performance because the index buffer is flushed to disk only once, after all INSERT statements have completed. Normally, there would be as many index buffer flushes as there are INSERT statements. Explicit locking statements are not needed if you can insert all rows with a single INSERT.
INSERT, UPDATE, and DELETE operations are very fast in MySQL, but you can obtain better overall performance by adding locks around everything that does more than about five successive inserts or updates. If you do very many successive inserts, you could do a LOCK TABLES followed by an UNLOCK TABLES once in a while (each 1,000 rows or so) to permit other threads to access table. This would still result in a nice performance gain.
INSERT is still much slower for loading data than LOAD DATA INFILE, even when using the strategies just outlined.
To increase performance for MyISAM tables, for both LOAD DATA INFILE and INSERT, enlarge the key cache by increasing the key_buffer_size system variable.
8.7 Optimizing for MEMORY Tables
Consider using MEMORY tables for noncritical data that is accessed often, and is read-only or rarely updated. Benchmark your application against equivalent InnoDB or MyISAM tables under a realistic workload, to confirm that any additional performance is worth the risk of losing data, or the overhead of copying data from a disk-based table at application start.
For best performance with MEMORY tables, examine the kinds of queries against each table, and specify the type to use for each associated index, either a B-tree index or a hash index. On the CREATE INDEX statement, use the clause USING BTREE or USING HASH. B-tree indexes are fast for queries that do greater-than or less-than comparisons through operators such as > or BETWEEN. Hash indexes are only fast for queries that look up single values through the = operator, or a restricted set of values through the IN operator.
8.8.1 Optimizing Queries with EXPLAIN
EXPLAIN EXTENDED can be used to obtain additional execution plan information. See Section 8.8.3, “EXPLAIN EXTENDED Output Format”.
EXPLAIN PARTITIONS is useful for examining queries involving partitioned tables. See Section 19.3.5, “Obtaining Information About Partitions”.
8.8.2 EXPLAIN Output Format
Each output row from EXPLAIN provides information about one table.
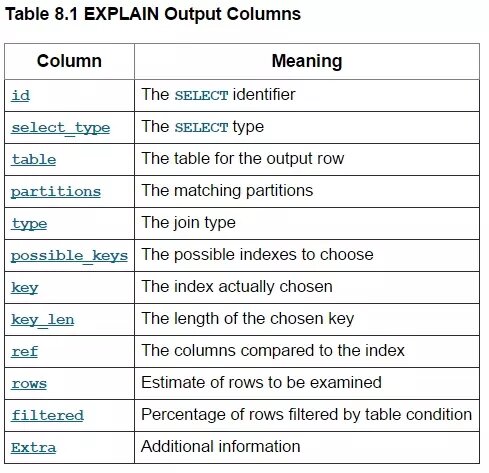
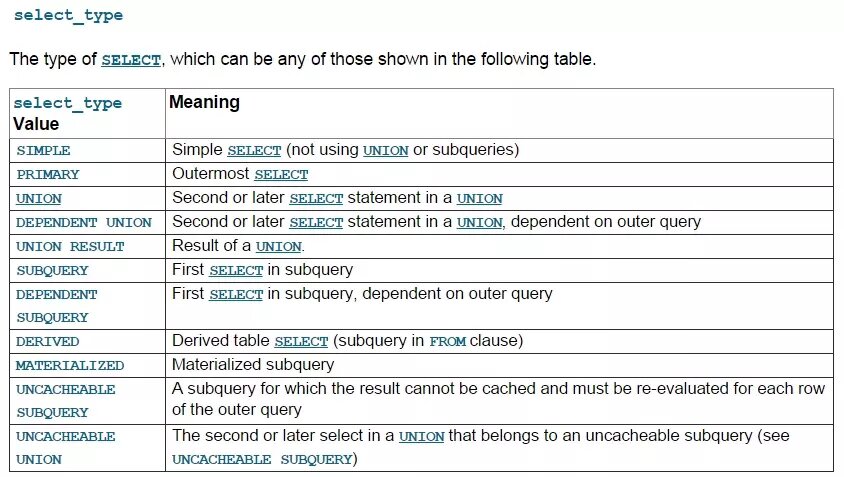
To force MySQL to use or ignore an index listed in the possible_keys column, use FORCE INDEX, USE INDEX, or IGNORE INDEX in your query.
The ref
column shows which columns or constants are compared to the index named in the key
column to select rows from the table.
If the value is func
, the value used is the result of some function. To see which function, use EXPLAIN EXTENDED
followed by SHOW WARNINGS
. The function might actually be an operator such as an arithmetic operator.
-
rows
The
rows
column indicates the number of rows MySQL believes it must examine to execute the query.For
InnoDB
tables, this number is an estimate, and may not always be exact.
8.10.1 The InnoDB Buffer Pool
Ideally, you set the size of the buffer pool to as large a value as practical, leaving enough memory for other processes on the server to run without excessive paging. The larger the buffer pool, the more InnoDB acts like an in-memory database, reading data from disk once and then accessing the data from memory during subsequent reads. The buffer pool even caches data changed by insert and update operations, so that disk writes can be grouped together for better performance.
Configuration Options
Several InnoDB
system variables control the size of the buffer pool and let you tune the LRU algorithm:
-
Specifies the size of the buffer pool. If your buffer pool is small and you have sufficient memory, making the pool larger can improve performance by reducing the amount of disk I/O needed as queries access
InnoDB
tables. -
Divides the buffer pool into a user-specified number of separate regions, each with its own LRU list and related data structures, to reduce contention during concurrent memory read and write operations. This option takes effect only when you set the
innodb_buffer_pool_size
to a size of 1 gigabyte or more. The total size you specify is divided among all the buffer pools. For best efficiency, specify a combination ofinnodb_buffer_pool_instances
andinnodb_buffer_pool_size
so that each buffer pool instance is at least 1 gigabyte. -
-
To avoid deadlocks when performing multiple concurrent write operations on a single InnoDB table, acquire necessary locks at the start of the transaction by issuing a SELECT ... FOR UPDATE statement for each group of rows expected to be modified, even if the DML statements come later in the transaction. If transactions modify or lock more than one table, issue the applicable statements in the same order within each transaction. Deadlocks affect performance rather than representing a serious error, because InnoDB automatically detects deadlock conditions and rolls back one of the affected transactions.
8.12.4.1 Using Symbolic Links for Databases on Unix
On Unix, the way to symlink a database is first to create a directory on some disk where you have free space and then to create a soft link to it from the MySQL data directory.shell>
mkdir /dr1/databases/test
shell>
ln -s /dr1/databases/test /path/to/datadir
MySQL does not support linking one directory to multiple databases. Replacing a database directory with a symbolic link works as long as you do not make a symbolic link between databases.
8.12.4.3 Using Symbolic Links for Databases on Windows
On Windows, symbolic links can be used for database directories. This enables you to put a database directory at a different location (for example, on a different disk) by setting up a symbolic link to it. Use of database symlinks on Windows is similar to their use on Unix, although the procedure for setting up the link differs. 详见http://dev.mysql.com/doc/refman/5.6/en/windows-symbolic-links.html
8.12.7.3 Thread Pool Tuning
thread_pool_size is the most important parameter controlling thread pool performance. It can be set only at server startup. Our experience in testing the thread pool indicates the following:
If the primary storage engine is InnoDB, the optimal thread_pool_size setting is likely to be between 16 and 36, with the most common optimal values tending to be from 24 to 36. We have not seen any situation where the setting has been optimal beyond 36. There may be special cases where a value smaller than 16 is optimal.
If the primary storage engine is MyISAM, the thread_pool_size setting should be fairly low. We tend to get optimal performance for values from 4 to 8. Higher values tend to have a slightly negative but not dramatic impact on performance.

The number of thread groups in the thread pool. This is the most important parameter controlling thread pool performance. It affects how many statements can execute simultaneously. The default value is 16, with a range from 1 to 64 of permissible values. If a value outside this range is specified, the thread pool plugin does not load and the server writes a message to the error log.
This variable was added in MySQL 5.6.10. It is available only if the thread pool plugin is enabled.