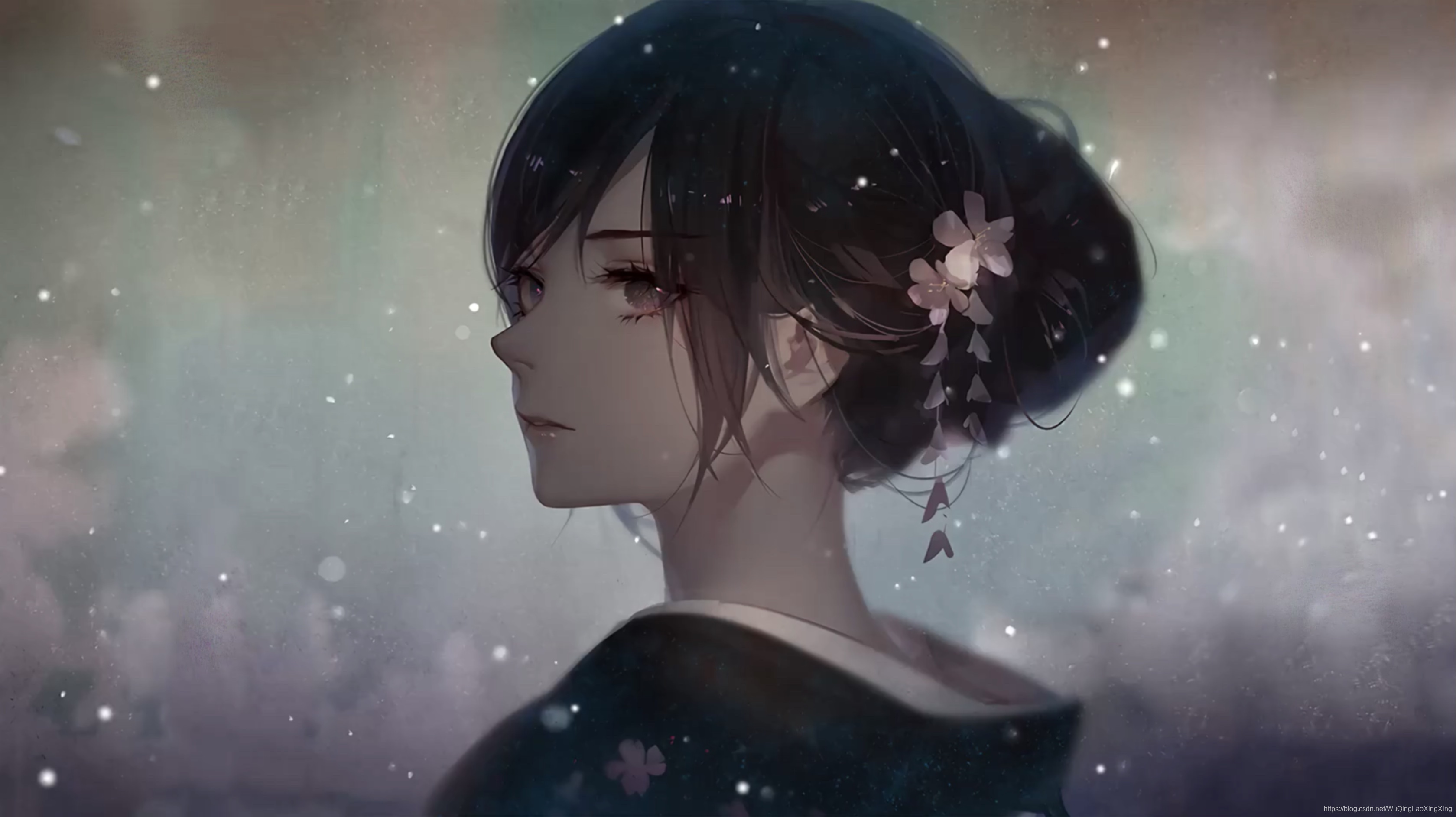
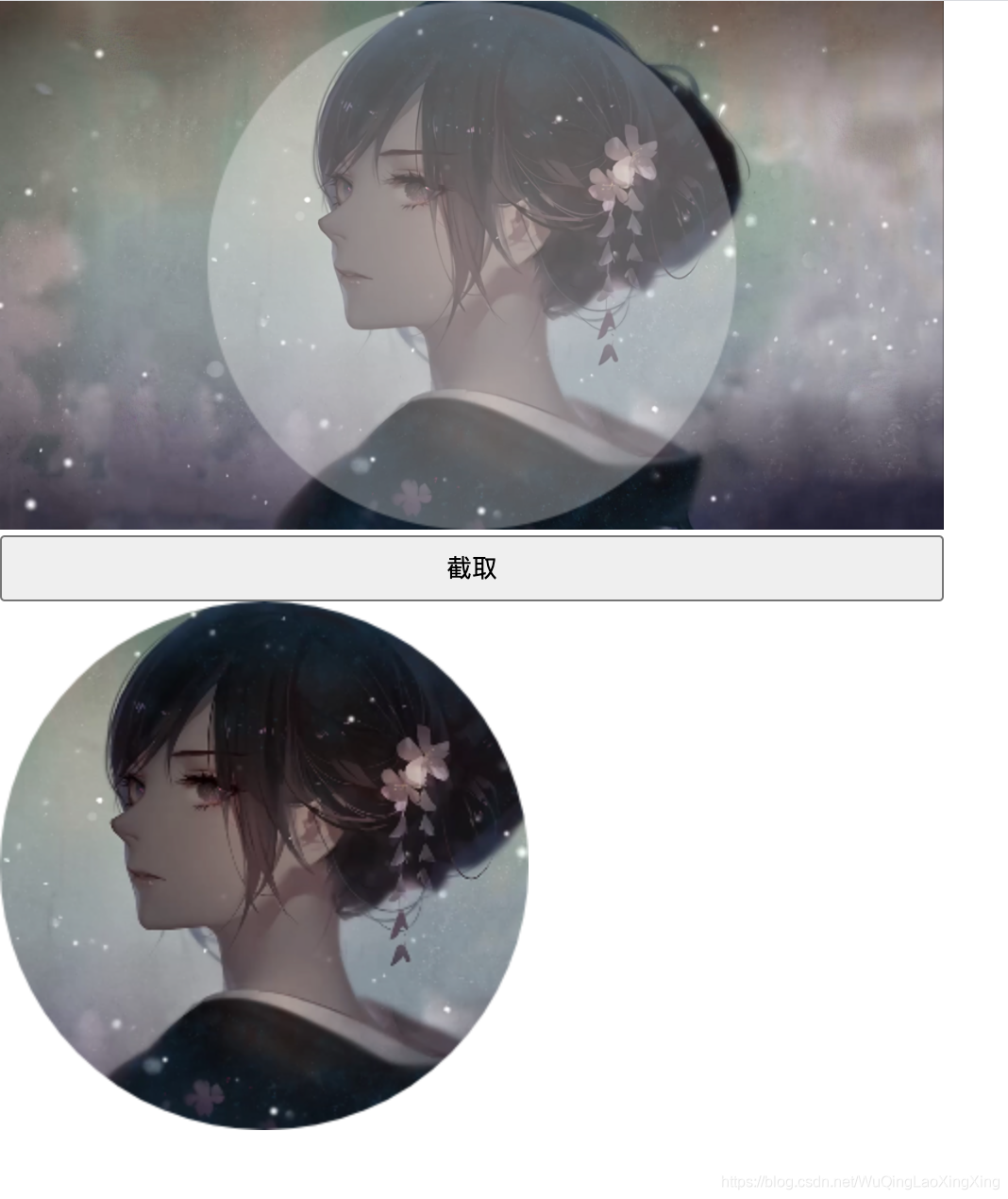
- 定义结构
<div class="img-intercep-wrap">
<div class="pic_wrapper">
<img src="./1.jpg">
<canvas class="pic_show"></canvas>
</div>
<button class="translate">截取</button>
<canvas class="pic_draw"></canvas>
</div>
- 样式部分
* {
margin: 0;
padding: 0;
}
.img-intercep-wrap{
font-size: 0;
}
.pic_wrapper {
display: inline-block;
overflow: hidden;
cursor: move;
position: relative;
background: #ccc;
}
img {
position: absolute;
z-index: 1;
}
.pic_show {
position: absolute;
left: 0;
top: 0;
z-index: 2;
pointer-events: none;
}
.translate{
display: block;
height: 35px;
margin-top: 3px;
}
.pic_draw{
display: none;
}
- 逻辑代码部分
let pic_wrapper = document.querySelector(".pic_wrapper")
let img = pic_wrapper.querySelector("img")
let translate = document.querySelector(".translate")
let pic_show = pic_wrapper.querySelector(".pic_show")
let ctx = pic_show.getContext("2d")
let initWidth,initHeight,disXO, disYO, maxWidth, maxHeight, wrapHeight
let computedUnit = 200
let wrapWidth = 500
let edgeMax = 10
img.onload = function () {
initWidth = img.width
initHeight = img.height
maxWidth = img.width * 2
maxHeight = img.height * 2
wrapHeight = parseInt(img.height / img.width * wrapWidth)
pic_wrapper.style.width = wrapWidth+"px"
pic_wrapper.style.height = wrapHeight+"px"
pic_show.width = wrapWidth
pic_show.height = wrapHeight
img.style.width = img.width +"px"
img.style.height = img.height +"px"
translate.style.width = wrapWidth +"px"
img.style.top = 0
img.style.left = 0
ctx.beginPath();
ctx.fillStyle = "rgba(255,255,255,0.3)"
ctx.arc(wrapWidth / 2, wrapHeight / 2, wrapHeight / 2, 0, Math.PI / 180 * 360, false);
ctx.fill();
}
let mousedownFn = function (e) {
e.preventDefault();
img.removeEventListener('mousemove', mousemoveFn);
img.addEventListener("mousemove", mousemoveFn)
disXO = e.clientX - pic_wrapper.offsetLeft - parseInt(img.style.left ? img.style.left : 0);
disYO = e.clientY - pic_wrapper.offsetTop - parseInt(img.style.top ? img.style.top : 0);
}
let mouseupFn = function (e) {
img.removeEventListener('mousemove', mousemoveFn);
}
let mouseleaveFn = function (e) {
img.removeEventListener('mousemove', mousemoveFn);
}
let mousewheelFn = function (e) {
e.preventDefault()
e.stopPropagation()
let {
width: wWidth,
height: wHeight
} = pic_wrapper.getBoundingClientRect()
let wheelDelta = e.wheelDelta
if (wheelDelta > 0) {
let lv = Math.abs(wheelDelta) / 120
let newWidth = img.width - lv * computedUnit
let newHeight = img.height - lv * computedUnit
if (newWidth > wWidth / 2) {
img.style.width = newWidth + "px"
} else {
img.style.width = wWidth / 2 + "px"
}
if (newHeight > wHeight / 2) {
img.style.height = newHeight + "px"
} else {
img.style.height = wHeight / 2 + "px"
}
} else {
let lv = Math.abs(wheelDelta) / 120
let newWidth = img.width + lv * computedUnit
let newHeight = img.height + lv * computedUnit
if (newWidth < maxWidth) {
img.style.width = newWidth + "px"
} else {
img.style.width = maxWidth + "px"
}
if (newHeight < maxHeight) {
img.style.height = newHeight + "px"
} else {
img.style.height = maxHeight + "px"
}
}
}
let mousemoveFn = function (e) {
e.preventDefault();
let {
width: wWidth,
height: wHeight
} = pic_wrapper.getBoundingClientRect()
let disXT = e.clientX - pic_wrapper.offsetLeft;
let disYT = e.clientY - pic_wrapper.offsetTop;
let disX = disXT - disXO
let disY = disYT - disYO
console.log(disX, disY);
if (wWidth - disX < edgeMax) {
disX = wWidth-edgeMax;
} else if (disX + img.width < edgeMax) {
disX = edgeMax-img.width;
}
if (wHeight - disY < edgeMax) {
disY = wHeight-edgeMax;
} else if (disY + img.height < edgeMax) {
disY = edgeMax-img.height;
}
img.style.left = disX + "px"
img.style.top = disY + "px"
}
img.addEventListener("mousedown", mousedownFn)
img.addEventListener("mouseleave", mouseleaveFn)
img.addEventListener("mouseup", mouseupFn)
img.addEventListener("mousewheel", mousewheelFn)
translate.addEventListener("click",function(){
const width = img.style.width
const height = img.style.height
const top = img.style.top
const left = img.style.left
console.table(width,height,top,left);
let pic_draw = document.querySelector(".pic_draw")
pic_draw.width = wrapHeight
pic_draw.height = wrapHeight
let ctx1 = pic_draw.getContext("2d")
ctx1.arc(wrapHeight/2, wrapHeight/2, wrapHeight/2, 0, Math.PI / 180 * 360, false);
ctx1.strokeStyle = '#FFFFFF';
ctx1.stroke();
ctx1.clip();
let lvW = initWidth/img.width
let lvH = initHeight/img.height
ctx1.drawImage(img,
(wrapWidth/2-wrapHeight/2 - parseInt(left))*lvW,-parseInt(top)*lvH,
(wrapHeight)*lvW,wrapHeight*lvH,
0,0, wrapHeight, wrapHeight)
console.log(pic_draw.toDataURL('image/png'))
},false)
- 备注
拖拽图片是需要阻止默认事件
想要看到截取的效果可以将类pic_draw修改
放大缩小的单位为200,可修改
初始化默认使用图片原始大小