开发工具: MyEclipse2013 , 数据库: MySql
1.首先, 在数据库中创建数据库 , 我使用的数据库工具是SQLyog.
创建如下数据库:
数据库创建完成后打开MyEclispe
2.创建Web Project
2.1: 第一项: 导包
需要导入如下包:(这些包在网上都可以找到, 我也会共享在我得资源里)
2.2 : 编写配置文件:hibernate.cfg.xml
<hibernate-configuration>
<!-- 配置数据库各个参数 -->
<session-factory>
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="connection.url">jdbc:mysql://localhost:3306/hibertest?characterEncoding=utf-8</property>
<property name="connection.username">root</property>
<property name="connection.password">sasa</property>
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- 显示sql语句 -->
<property name="show_sql">true</property>
<!-- 格式化代码 -->
<property name="format_sql">true</property>
<mapping resource="com/entity/Dept.hbm.xml" />
</session-factory>
</hibernate-configuration>
2.3: 创建一个实体类 , 并给get/set方法 , 有参无参构造
package com.entity;
import java.io.Serializable;
public class Dept implements Serializable {
private int deptno;
private String deptname;
private String loc;
public int getDeptno() {
return deptno;
}
public void setDeptno(int deptno) {
this.deptno = deptno;
}
public String getDeptname() {
return deptname;
}
public void setDeptname(String deptname) {
this.deptname = deptname;
}
public String getLoc() {
return loc;
}
public void setLoc(String loc) {
this.loc = loc;
}
public Dept(int deptno, String deptname, String loc) {
super();
this.deptno = deptno;
this.deptname = deptname;
this.loc = loc;
}
public Dept() {
super();
// TODO Auto-generated constructor stub
}
}
实体包里需创建Dept.hbm.xml文件;
代码如下:
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.entity.Dept" table="dept">
<!-- 主键映射 -->
<id name="deptno" column="deptno">
<!-- 主键产生的值得策略 -->
<generator class="native"></generator>
</id>
<!-- 此处的name值对应实体类中属性 -->
<property name="deptname" column="deptname"></property>
<property name="loc" column="loc"></property>
</class>
</hibernate-m
下面编写test类:
代码如下:
package com.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
import org.hibernate.service.ServiceRegistry;
import org.hibernate.service.ServiceRegistryBuilder;
import com.entity.Dept;
import junit.framework.TestCase;
public class Test extends TestCase {
// 增加部门
public void testAdd() {
// 读取hibernate配置文件
Configuration config = new Configuration()
.configure("hibernate.cfg.xml");
// 所有的配置都要向一个类中注册
ServiceRegistry sr = new ServiceRegistryBuilder().applySettings(
config.getProperties()).buildServiceRegistry();
// 获得sessionfactory
SessionFactory sf = config.buildSessionFactory(sr);
// 获得session,操作数据库的接口
Session session = sf.openSession();
// 创建一个部门对象
Dept dept = new Dept();
dept.setDeptname("开发部");
dept.setLoc("武汉");
// 开启事物
Transaction tran = session.beginTransaction();
// 执行插入操作
//手动try catch 选中-右键 -surround with
try {
session.save(dept);
tran.commit();
} catch (Exception e) {
tran.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
}
总体图如下: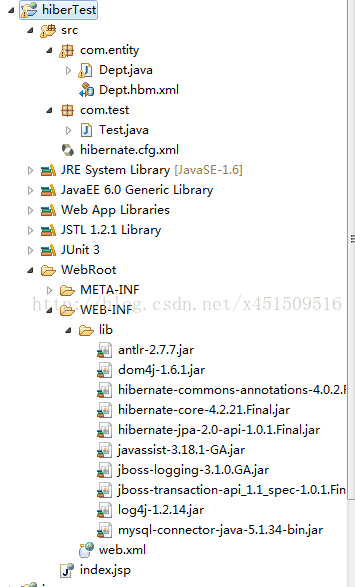
运行test类里的testAdd()方法:
控制台会打印出sql语句如下:
Hibernate: insert into dept (deptname, loc) values (?, ?)
数据库数据添加结果:
![]()