pip install opencv-python
import cv2
import numpy as np
def get_face(path):
face_cascade = cv2.CascadeClassifier('../data/head/xml/haarcascade_frontalface_default.xml')
img = cv2.imread(path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(20, 20),
flags=cv2.CASCADE_SCALE_IMAGE)
if len(faces) > 0:
faces = faces[np.argsort(faces[:, 1])]
return faces[0], img
else:
print('没有识别到人脸')
if __name__ == '__main__':
image = get_face('./test.jpg')
if image is not None:
x, y, w, h = image[0]
img = image[1]
img_w = img.shape[0]
img_h = img.shape[1]
min_wh = int(min(min(x * 2 + w, y * 2 + h + 6), min(img_w, img_h)))
save_x = max(x - int((min_wh - w) / 2), 0)
save_y = max(y - int((min_wh - h) / 2) - 6, 0)
save_img = np.copy(img)[save_y: save_y + min_wh, save_x: save_x + min_wh]
cv2.imwrite('./test_out.jpg', save_img)
rect_img = cv2.rectangle(img, (x, y), (x + w, y + h), (255, 0, 0), 2)
rect_img = cv2.rectangle(img, (save_x, save_y), (save_x + min_wh, save_y + min_wh), (0, 0, 255), 2)
cv2.imshow('test_out.jpg', img)
cv2.waitKey(0)
- 原图
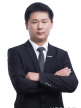
- 截取头像
