一、Values资源
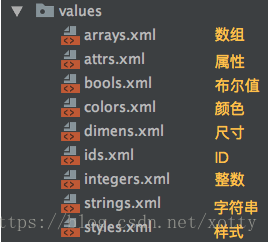
1.定义和使用方法一览表
资源种类 | 定义 | in XML | in Java |
String | <string name=”xxx【1】”>Value【2】</string> | @string/xxx | getResources.getString(R.string.xxx) getResources.getText(R.string.xxx) |
Integer | <integer name=”xxx”>Value</integer> | @integer/xxx | getResources.getInteger(R.integer.xxx) |
Bool | <bool name=”xxx”>Value</bool> | @bool/xxx | getResources.getBoolean(R.bool.xxx) |
Color | <color name=”xxx”Value</color> <drawable name=”xxx”Value</drawable> | @color/xxx @drawable/xxx | getResources.getColor(R.color.xxx) getDrawable(R.drawable.xxx) |
Dimension | <dimen name=”xxx”>Value</dimen> | @dimen/xxx | getResources.getDimension(R.dimen.xxx) |
Array | <string-array name=”xxx” <item>Value1</item> <item>Value2</item> </string-array> <integer-array name=”xxx” <item>Value1</item> <item>Value2</item> </integer-array> <array name=”xxx” <item>Reference Value1【3】</item> <item>Reference Value2</item> </array> | @array/xxx | getResources().getStringArray(R.array.xxx) getResources().getIntArray(R.array.xxx)
TypedArray typedArray= getResources().obtainTypedArray(R.array.xxx) typedArray.getResourceId(index ,defaultValue) |
ID | <item type=”id” name=”xxx”/> | @id/xxx | findViewbyId(R.id.xxx) mView.setId(R.id.xxx) |
Attribute | <attr name=”aaa1”【4】 format=”fff”【5】/> <attr name=”aaa2” format=”fff”/>
<declare-styleable name=”xxx” <attr name=”aaa1” format=”fff”/> <attr name=”aaa2”> <enum name=”eee1”【7】value=”v1”【7】/> <enum name=”eee2” value=”v2”/> </attr> </declare-styleable> | ?attr/aaa1 ?attr/aaa2
app:aaa1 app:aaa2
| int[] mAttrs = {R.attr.aaa1, R.attr.aaa2}; TypedArray mAttributes = obtainStyledAttributes(attributeSet【6】, mAttrs);
TypedArray mAttributes= obtainStyledAttributes(R.style.yyy, R.styleable.xxx)
mAttributes.getXXX(index,defaultValue)
mAttributes.recycle() |
Style | <style name=”yyy”【8】 <item name=”aaa1”>Value</item> <item name=”aaa2”>Reference Value</item> </style> <style name=”xxx” parent=”Theme”【9】 <item name=”aaa”>Value</item> </style> | @style/xxx【10】 | 属性值的引用方法之一参见上面Attribute内容 new ContextThemeWrapper(context, R.style.yyy)
主题的引用方法如下: requestWindowFeature(R.style.xxx);</LinearLayout> </ScrollView> |
2.示例
1)String
XML定义:
<resources> <string name="app_name">ResourcesDemo</string> <string name="string_xml">String XML </string> <string name="string_code">String Code</string> </resources>
XML引用:
<Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="@string/string_xml"/>
Java引用:
((Button) btn).setText(getString(R.string.string_code)); ((Button) btn).setText(getText(R.string.string_code));
2)Integer
XML定义:
<resources> <integer name="max_speed">75</integer> <integer name="min_speed">5</integer> </resources>
XML引用:
<Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="@integer/max_speed"/>
Java引用:
((Button) btn).setText("" + getResources().getInteger(R.integer.min_speed));
3)Bool
XML定义:
<resources> <bool name="bool_xml">true</bool> <bool name="bool_code">false</bool> </resources>
XML引用:
<Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="@bool/bool_xml"/>
Java引用:
((Button) btn).setText("" + getResources().getBoolean(R.bool.bool_code));
4)Color
XML定义:
<resources> <color name="c_red">#a00</color> <color name="c_blue">#0000ff</color> <drawable name="d_green">#f0f0</color> <drawable name="d_yellow">#ffffff00</color> </resources>
XML引用:
<Button android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@drawable/d_green" android:textColor="@color/c_red/>
Java引用:
(((Button)btn).setBackgroundColor(getResources().getColor(R.color.c_grey)); ((Button) btn).setBackground(getDrawable(R.drawable.d_grey));
颜色值格式(A代表透明度):
- #RGB,红、绿、蓝(只支持0~f共16级颜色)
- #ARGB,透明度(只支持0~f共16级透明度)+红、绿、蓝(只支持0~f这16级颜色)
- #RRGGBB,红、绿、蓝(支持00~ff共256级颜色)
- #AARRGGBB,透明度(支持00~ff共256级透明度)+红、绿、蓝(支持00~ff共256级颜色)
5)Dimension
XML定义:
<resources> <dimen name="text_margin_small">8dp</dimen> <dimen name="text_margin_very_small">4dp</dimen> <dimen name="text_size_large">32sp</dimen> <dimen name="text_size_normal">20sp</dimen> </resources>
XML引用:
<Button android:layout_width="match_parent" android:layout_height="@dimen/button_height"/>
Java引用:
float density=getResources().getDisplayMetrics().scaledDensity float fontSize = getResources().getDimension(R.dimen.text_size_small) / density; ((Button) btn).setTextSize(fontSize);
尺寸值单位:
- in(英寸): 屏幕的物理尺寸, 每英寸等于2.54厘米。
- mm(毫米): 屏幕的物理尺寸。
- pt(点): 屏幕的物理尺寸。1/72英寸。
- dp/dip: 与密度无关的象素,一种基于屏幕密度的抽象单位。在每英寸160点的显示器上,1dp =1px。但dp和px的比例会随着屏幕密度的变化而改变,不同设备有不同的显示效果。
- sp: 与刻度无关的象素,主要用于字体显示,作为和文字相关大小单位。
6)Array
XML定义:
<resources> <string-array name="planets"> <item>Mercury</item> <item>Venus</item> <item>@string/earth</item> <item>Mars</item> </string-array> <integer-array name="SCMBit"> <item>8</item> <item>16</item> <item>@integer/max_bits</item> </integer-array> <array name="scenes"> <item>@drawable/wrapper_img1</item> <item>@drawable/wrapper_img2</item> <item>@drawable/wrapper_img3</item> <item>@drawable/wrapper_img4</item> </array> </resources>
XML引用:
<Spinner android:id="@+id/spn_array" android:layout_width="@dimen/button_width_small" android:layout_height="@dimen/button_height" android:entries="@array/planets"/>
Java引用:
int scmbits[] = getResources().getIntArray(R.array.SCMBit);
7)ID
XML定义:
<resources> <item type="id" name="raw_demo"/> <item type="id" name="drawble_demo"/> <item type="id" name="animation_demo"/> </resources>
XML引用:
<TextView android:id="@id/txv_array" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Array:"/>
Java引用:
TextView txv=findViewbyId(R.id.txv_array)
TypedArray typedArray = getResources().obtainTypedArray(R.array.scenes); drawables=new Drawable[typedArray.length()]; drawables[i] = getDrawable(typedArray.getResourceId(i ,R.drawable.wrapper_img1));
8)Attribute
XML定义:
<resources> <attr name="mPercent" format="float"/> <attr name="mColor" format="color|reference"/> <declare-styleable name="DraggableDot"> <attr name="radius" format="dimension" /> <attr name="legend" format="string" /> <attr name="anr"> <enum name="none" value="0" /> <enum name="thumbnail" value="1" /> <enum name="drop" value="2" /> </attr> <attr name="textStyle"> <flag name="normal" value="0" /> <flag name="bold" value="1" /> <flag name="italic" value="2" /> </attr> </declare-styleable> </resources>
XML引用:
<org.xottys.ResourcesDemo.PieView android:id="@+id/pieview_attr_style" android:layout_width="100dp" android:layout_height="100dp" app:mColor="@color/c_red" app:mPercent="0.6" /> <Button android:id="@+id/btn_attr_style" android:layout_width="match_parent" android:layout_height="@dimen/button_height" android:textColor="?attr/mColor"/>
Java引用:
TypedArray styledAttributes = obtainStyledAttributes(R.style.pie_para, R.styleable.pieView); int color = styledAttributes.getColor(0, 0xffa4d5e6); float percent = styledAttributes.getFloat(1 + 0, 0.75f); styledAttributes.recycle();
9)Style
XML定义:
<resources> <style name="pie_para"> <item name="mColor">@color/c_yellow</item> <item name="mPercent">0.4</item> </style>
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> <item name="mColor">@color/c_green</item> <item name="android:actionBarSize">40dp</item> <item name="actionBarSize">40dp</item> </style> </resources>
XML引用:
<Button android:layout_width="match_parent" android:layout_height="wrap_content" android:text="@string/string_xml" style="@style/button_style"/>
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="org.xottys.ResourcesDemo"> <application android:theme="@style/AppTheme"> <activity ...... </activity> </application> </manifest>
Java引用:
textView.setTextAppearance(this, R.style.text_style); imageView = new ImageView(new ContextThemeWrapper(context, R.style.img), null, 0);
requestWindowFeature(R.style.AppTheme);
二、ColorStateList资源
在res/color文件夹下,用来在控件不同状态时呈现不同颜色。
资源种类 | 定义 | in XML | in Java |
ColorStateList | <selector xmlns:android= "http://schemas.android.com/apk/res/android" > <item android:color="hex_color" android:state_pressed=["true" | "false"] android:state_focused=["true" | "false"] android:state_selected=["true" | "false"] android:state_checkable=["true" | "false"] android:state_checked=["true" | "false"] android:state_enabled=["true" | "false"] android:state_window_focused=["true" | "false"] /> </selector> | @color/xxx | getResources(). getColorStateList(R.color.filename【1】);
|
注解: 【1】颜色状态xml文件名称
XML定义示例(my_colorlist.xml):
<selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_pressed="true" android:color="@color/c_red" /> <item android:color="#e6e67b" /> </selector>
XML引用示例:
<Button android:id="@+id/btn_color" android:layout_width="match_parent" android:layout_height="wrap_content" android:textColor="@color/my_colorlist"/>
Java引用示例:
ColorStateList csl=getResources().getColorStateList(R.color.my_colorlist); ((Button)btn).setTextColor(csl);
三、XML资源
在res/xml文件夹下,用来提供自定义xml格式的数据,只能在代码中用xml解析器来解析引用。
XML引用示例(data.xml):
<?xml version="1.0" encoding="utf-8"?> <root> <title>Hello XML!</title> </root>
Java引用示例:
XmlResourceParser xmlResourceParser = getResources().getXml(R.xml.data); try { xmlResourceParser.next(); int eventType = xmlResourceParser.getEventType(); while (eventType != XmlPullParser.END_DOCUMENT) { if (eventType == XmlPullParser.START_TAG) { if (xmlResourceParser.getName().equals("title")) { xmlBtn.setText(xmlResourceParser.nextText()); } } eventType = xmlResourceParser.next(); } } catch (org.xmlpull.v1.XmlPullParserException e1) { e1.printStackTrace(); } catch (IOException e2) { e2.printStackTrace(); }
四、图标资源
在res/mipmap文件夹下,用来为程序提供图标(png文件),只能在AndroidManifest.xml 中引用作为图标使用。
XML引用示例(ic_launcher.png):
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="org.xottys.ResourcesDemo"> <application android:icon="@mipmap/ic_launcher"> <activity ...... </activity> </application> </manifest>
五、Menu资源
在res/menu文件夹下,用来为程序提供菜单项。
资源种类 | 定义 | in XML | in Java |
Menu | <menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@[+][package:]id/resource_name" android:title="string" android:titleCondensed="string" android:icon="@[package:]drawable/drawable_resource_name" android:onClick="method name" android:showAsAction=["ifRoom" | "never" | "withText" | "always" | "collapseActionView"] android:actionLayout="@[package:]layout/layout_resource_name" android:actionViewClass="class name" android:actionProviderClass="class name" android:alphabeticShortcut="string" android:alphabeticModifiers=["META" | "CTRL" | "ALT" | "SHIFT" | "SYM" | "FUNCTION"] android:numericShortcut="string" android:numericModifiers=["META" | "CTRL" | "ALT" | "SHIFT" | "SYM" | "FUNCTION"] android:checkable=["true" | "false"] android:visible=["true" | "false"] android:enabled=["true" | "false"] android:menuCategory=["container" | "system" | "secondary" | "alternative"] android:orderInCategory="integer" /> <group android:id="@[+][package:]id/resource name" android:checkableBehavior=["none" | "all" | "single"] android:visible=["true" | "false"] android:enabled=["true" | "false"] android:menuCategory=["container" | "system" | "secondary" | "alternative"] android:orderInCategory="integer" > </group> <item > </menu> | N/A | getMenuInflater(). inflate(R.menu.filename, menu); |
XML定义示例(menu_main.xml):
<menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@id/drawble_demo" android:title="@string/drawable_demo" /> <item android:id="@id/raw_demo" android:title="@string/raw_demo" /> <item android:id="@id/animation_demo" android:title="@string/anim_demo" /> </menu>
Java引用示例:
@Override public boolean onCreateOptionsMenu(Menu menu) { getMenuInflater().inflate(R.menu.menu_main, menu); return true; }
六、Layout资源
在res/layout文件夹下,用来为程序提供UI视图。
资源种类 | 定义 | in XML | in Java |
Layout | <ViewGroup xmlns:android="http://schemas.android.com/apk/res/android" android:id="@[+][package:]id/resource_name" android:layout_height=["dimension" | "match_parent" | "wrap_content"] android:layout_width=["dimension" | "match_parent" | "wrap_content"] [ViewGroup-specific attributes] > <View android:id="@[+][package:]id/resource_name" android:layout_height=["dimension" | "match_parent" | "wrap_content"] android:layout_width=["dimension" | "match_parent" | "wrap_content"] [View-specific attributes] > <requestFocus/> </View> <ViewGroup > <View /> </ViewGroup> <include layout="@layout/layout_resource|merge_resource"/> </ViewGroup> 可复用布局模块: <merge xmlns:android="http://schemas.android.com/apk/res/android"> <View android:id="@[+][package:]id/resource_name" android:layout_height=["dimension" | "match_parent" | "wrap_content"] android:layout_width=["dimension" | "match_parent" | "wrap_content"] [View-specific attributes] > <requestFocus/> </View> </merge> | @layout/ filename | setContentView(R.layout.filename) getLayoutInflater(). inflate(R.layout.filename, null) |
<?xml version="1.0" encoding="utf-8"?> <ScrollView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="15dp"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="30dp" android:text="res/values res/color res/xml" android:textSize="@dimen/text_size_small" /> <LinearLayout android:id="@+id/lnl_string" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:orientation="horizontal"> <include layout="@layout/mymerge"/> </LinearLayout> <LinearLayout android:id="@+id/lnl_integer" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp" android:orientation="horizontal"> <include layout="@layout/mymerge"/> </LinearLayout> </LinearLayout> </ScrollView>
<?xml version="1.0" encoding="utf-8"?> <merge xmlns:android="http://schemas.android.com/apk/res/android"> <TextView android:id="@+id/txv" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="String:" android:textSize="@dimen/text_size_normal" /> <Button android:id="@+id/btn" android:layout_width="match_parent" android:layout_height="@dimen/button_height" android:background="@drawable/d_blue" android:onClick="clickButton" android:text="@string/string_xml" android:textAllCaps="false" android:textColor="@color/my_colorstatelist" android:textSize="@dimen/text_size_normal" /> </merge>
Java引用示例:
setContentView(R.layout.activity_main)
TextView txvInteger=lnlInteger.findViewById(R.id.txv); txvInteger.setText("Integer:"); Button btnInteger=lnlInteger.findViewById(R.id.btn); btnInteger.setText(""+getResources().getInteger(R.integer.max_speed)); btnInteger.setId(R.id.btn_integer);
七、Font资源
Android 8.0新增在res/font文件夹下,可以为程序提供自定义字体。字体文件可以是ttf(TrueType)或otf(OpenType)格式,可以直接引用字体文件,也用一xml格式font-family文档间接引用字体文件。
资源种类 | 定义 | in XML | in Java |
Font | <?xml version="1.0" encoding="utf-8"?> <font-family xmlns:android="http://schemas.android.com/apk/res/android"> <fontandroid:fontStyle=""normal|bold|italic" android:fontWeight="100|200|300|400|500|600|700|800|900" android:font="@font/fontfile" /> </font-family> | @font/filename | Typeface typeface= getResources().getFont(Rfont.filename); textView.setTypeface(typeface);
|
XML定义示例(myfont.xml):
<?xml version="1.0" encoding="utf-8"?> <font-family xmlns:android="http://schemas.android.com/apk/res/android"> <font android:fontStyle="normal" android:fontWeight="400" android:font="@font/fzlx" /> <font. android:fontStyle="italic" android:fontWeight="400" android:font="@font/fzlx" /> </font-family>
XML引用示例:
<Button android:id="@+id/btn_font" android:layout_width="match_parent" android:layout_height="80dp" android:onClick="clickButton" android:text="@string/font_text1" android:fontFamily="@font/myfont"/>
Java引用示例:
Typeface typeface = getResources().getFont(R.font.myfont); ((Button)findViewById(R.id.btn_font)).setTypeface(typeface); ((Button)findViewById(R.id.btn_font)).setText(R.string.font_text2);
八、Demo源码
Resource Demo是将所有Android资源集合在一起,对其定义、引用和应用进行充分完全的演示,并提供了详细的注释和说明,不仅可供学习时使用,也可作为模版在开发中直接拷贝需要的代码,以加快开发进度。该Demo在Android Studio 3.0 和Android SDK5.0以上版本可直接运行,欢迎索取使用。有需要者请发邮件到:xottys@163.com 即可。
该部分内容APK的运行效果在上一篇博文中可见,也可点击后显示。建议直接下载安装APK:Android ResourceDemo,演示效果会更好。该APK可在Android5.0以上版本正常运行。