lambda表达式实现了函数式接口的实例对象,并实现了接口里的方法,
apple -> {
return apple.color.equals("green");
}
apple是方法的形参,后面是实现体
-
一些Functionnal Interface:定义lambda表达式的一些基础函数
predicate判断是与否,consumer是输入输出,function是转化,supplier是new新对象
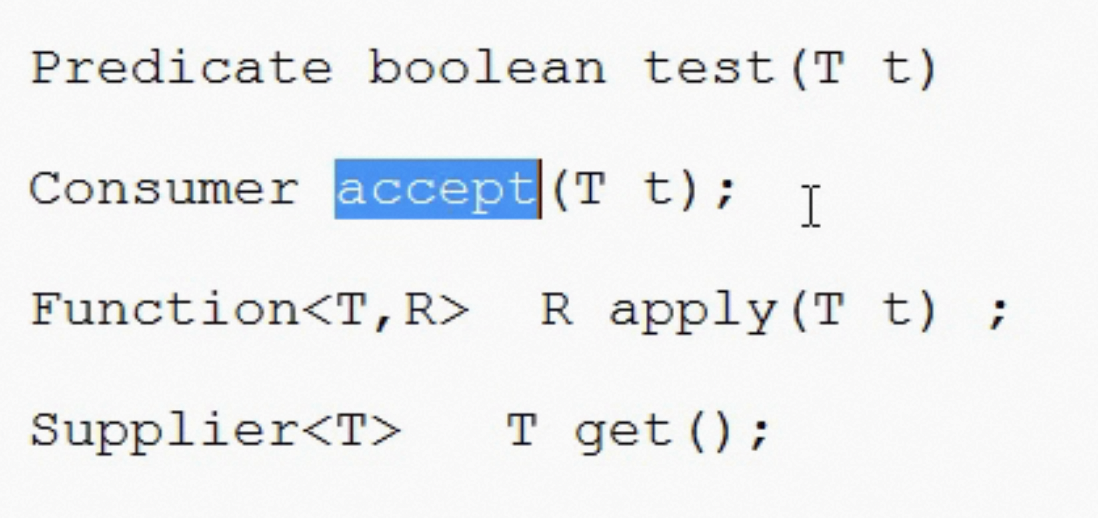
2、 源码:
@FunctionalInterface
public interface Predicate<T> {
/**
* Evaluates this predicate on the given argument.
*
* @param t the input argument
* @return {@code true} if the input argument matches the predicate,
* otherwise {@code false}
*/
boolean test(T t);
@FunctionalInterface
public interface Consumer<T> {
/**
* Performs this operation on the given argument.
*
* @param t the input argument
*/
void accept(T t);
@FunctionalInterface
public interface Function<T, R> {
/**
* Applies this function to the given argument.
*
* @param t the function argument
* @return the function result
*/
R apply(T t);
@FunctionalInterface
public interface Comparator<T> {
int compare(T o1, T o2);
3、案例:
package java8;
import org.apache.commons.compress.utils.Lists;
import scala.App;
import java.util.List;
import java.util.function.*;
public class LambdaUsage1 {
public static void main(String[] args) {
Apple greenApple = new Apple("green", 150);
Apple redApple = new Apple("red", 105);
List<Apple> appleList = Lists.newArrayList();
appleList.add(greenApple);
appleList.add(redApple);
//filter、filterByWeight、filterByBiPredicate、printByConsumer、convertByFunction这些例子中函数式接口的类型和接口实现的调用是在定义时确定的,具体实现是在lambda表达式实现的
//这个自定义的函数filter就类似于下面的list.filter(apple -> {return apple.color.equals("green");}))的filter
//Predicate:断言,有入参
//boolean test(T t);
filter(appleList, (apple -> {
return apple.color.equals("green");
})).forEach(System.out::print);
appleList.stream().filter(apple -> {
return apple.color.equals("green");
}).forEach(System.out::print);
filterByWeight(appleList, (appleWeight -> {
return appleWeight > 100;
})).forEach(System.out::print);
filterByBiPredicate(appleList, (color, appleWeight) -> {
return color.equals("green") && appleWeight > 100;
}).forEach(System.out::print);
//Consumer
//void accept(T t);
printByConsumer(appleList, (Apple apple) -> {
System.out.println(apple.getColor()+"::"+apple.getWeight());
});
//Function
//R apply(T t);
convertByFunction(appleList, (Apple apple)->{
apple.setColor(apple.getColor()+"##");
return apple;
}).forEach(System.out::print);
//Supplier
//T get();
Supplier<String> stringSupplier = String::new;
System.out.println(stringSupplier.get().getClass());
Apple supplyApple = supplyApple(() -> {
return new Apple("red", 001);
});
System.out.println(supplyApple);
}
private static List<Apple> filter(List<Apple> appleList, Predicate<Apple> predicate) {
List<Apple> result = Lists.newArrayList();
for (Apple apple : appleList) {
if (predicate.test(apple)) {
result.add(apple);
}
}
return result;
}
private static List<Apple> filterByWeight(List<Apple> appleList, LongPredicate predicate) {
List<Apple> result = Lists.newArrayList();
for (Apple apple : appleList) {
if (predicate.test(apple.getWeight())) {
result.add(apple);
}
}
return result;
}
private static List<Apple> filterByBiPredicate(List<Apple> appleList, BiPredicate<String, Integer> biPredicate) {
List<Apple> result = Lists.newArrayList();
for (Apple apple : appleList) {
if (biPredicate.test(apple.getColor(), apple.getWeight())) {
result.add(apple);
}
}
return result;
}
private static void printByConsumer(List<Apple> appleList, Consumer<Apple> appleConsumer) {
for (Apple apple : appleList) {
appleConsumer.accept(apple);
}
}
private static List<Apple> convertByFunction(List<Apple> appleList, Function<Apple,Apple> appleFunction) {
List<Apple> result = Lists.newArrayList();
for (Apple apple : appleList) {
Apple restmp = appleFunction.apply(apple);
result.add(restmp);
}
return result;
}
private static Apple supplyApple(Supplier<Apple> appleSupplier) {
return appleSupplier.get();
}
}