一、线性回归
### --- 线性回归
~~~ 线性回归是在给定一个或多个其他变量(数据点)的值的情况下,预测一个连续变量的值的问题。
~~~ 例如,根据房屋的占地面积,预测房屋的售价。
~~~ 在这个示例中,您可以将已知特征及其关联的标签绘制在简单的线性图上,
~~~ 如x, y 散点图,并在此基础上拟合一条直线 。
~~~ 然后,可以读取对应于该图的x 范围内的任何特征值的标签。
~~~ 但是,线性回归问题多数情况下涉及多个特征,我们称之为多元线性回归。
~~~ 在这种情况下,不是一条数据的线,而是一个平面(两个特征)或一个超平面(两个以上特征)。
~~~ 备注:特征也称为预测变量或自变量。 标签也称为响应变量或因变量。
### --- 通过TensorFlow 实现线性回归
~~~ 构建了一个人工数据集,我们将通过使用 TensorFlow 拟合这条线。
~~~ 执行以下操作-在导入和初始化之后,我们进入一个循环。
~~~ 在此循环内,我们计算总损失(定义为点的数据集y 的均方误差)。
~~~ 然后,我们根据我们的权重和偏置来得出这种损失的导数,
~~~ 这将用于调整权重和偏差从而降低损失的值, 这就是所谓的梯度下降。
~~~ 通过多次重复此循环,我们可以将损失降低到尽可能低的程度,并且可以使用训练好的模型进行预测。
~~~ # 导入所需的模块:
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
~~~ # 初始化重要常量:
n_examples = 1000 # 样本个数
training_steps = 1000 # 迭代次数(梯度下降)
display_step = 100 # 显示loss频率
learning_rate = 0.01 # 学习率
m, c = 6, -5 # 设置m、c,满足不同的线性 y = m*x + c
~~~ # 给定weight 和bias ( m 和c )的函数,用于计算预测的y
~~~ # 生成数据
def train_data(n, m, c):
x = tf.random.normal([n]) # 正态分布下的随机数
noise = tf.random.normal([n])
y = m*x + c + noise # 散点
return x, y
~~~ # 预测y
def prediction(x, weight, bias): #weight回归系数(m)、bias为截距(c)
return weight*x + bias
~~~ # 以预估值和实际值之间的均方误差作为损失(loss)
def loss(x, y, weights, biases):
error = prediction(x, weights, biases) - y #误差值
squared_error = tf.square(error) #求平方
return tf.reduce_mean(input_tensor=squared_error) #计算均值,得到均方误差
~~~ # 使用TensorFlow下的GradientTape() 的类,
~~~ # 我们可以编写一个函数来计算相对于weights 和bias 的损失的导数(梯度)
~~~ # 对均方误差求导
def grad(x, y, weights, biases):
with tf.GradientTape() as tape:
loss_ = loss(x, y, weights, biases)
return tape.gradient(loss_, [weights, biases])
### --- 显示构建的散点图
x, y = train_data(n_examples,m,c) # 得到x和y
plt.scatter(x,y)
plt.xlabel("x")
plt.ylabel("y")
plt.title("Figure 1: Training Data")
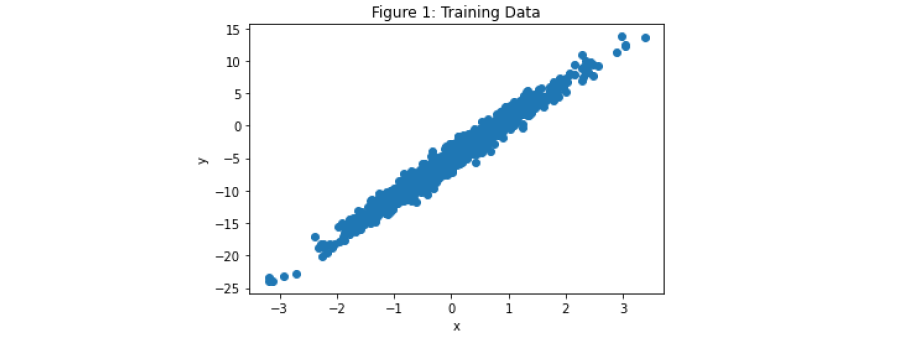
~~~ # 计算初始的均方误差
W = tf.Variable(np.random.randn()) #初始化预测的值weight (m)
B = tf.Variable(np.random.randn()) #初始化预测的值bias (c)
print("Initial loss: {:.3f}".format(loss(x, y, W, B))) #得到误差
~~~ # 根据learning_rate将weights和bias进行微调,
~~~ # 以将损失逐步降低至我们已经收敛到最佳拟合线的程度(梯度下降)。
for step in range(training_steps): #迭代次数training_steps(1000次)
deltaW, deltaB = grad(x, y, W, B) # 得到weights、bias的均方误差(导)
# 进行微调
change_W = deltaW * learning_rate
change_B = deltaB * learning_rate
W.assign_sub(change_W) # 变量W变更为减去change_W后的值
B.assign_sub(change_B) # 变量B变更为减去change_B后的值
if step==0 or step % display_step == 0:
print("Loss at step {:02d}: {:.6f}".format(step, loss(x, y, W, B)))
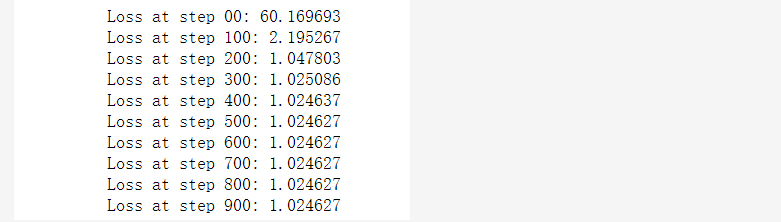
~~~ # 拟合最优直线
print("Final loss: {:.3f}".format(loss(x, y, W, B))) #最终损失
print("W = {}, B = {}".format(W.numpy(), B.numpy()))
~~~ # 对比原始的W和B
print("Compared with m = {:.1f}, c = {:.1f}".format(m, c)," of the original
line")
Final loss: 1.083
W = 5.989720821380615, B = -5.057075023651123
Compared with m = 6.0, c = -5.0 of the original line
x, y = train_data(n_examples,m,c)
plt.scatter(x,y)
xs = np.linspace(-3, 4, 50)
ys = W.numpy()*xs + B.numpy()
plt.scatter(xs,ys)
plt.xlabel("x")
plt.ylabel("y")
plt.title("Figure 2: Line of Best Fit")
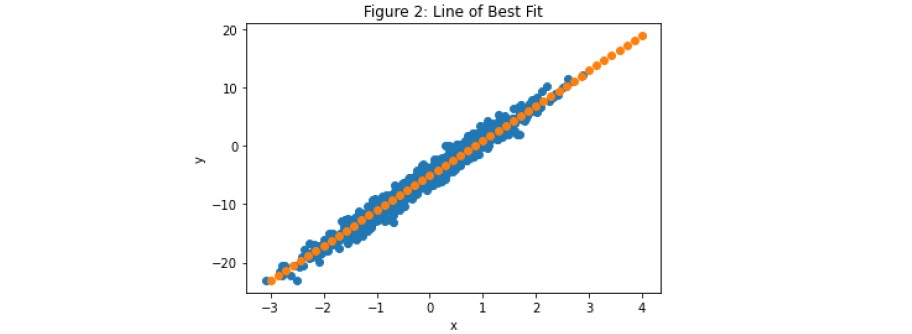
### --- 通过TensorFlow 预测房价
~~~ 这里使用波士顿住房数据集,涉及 13 个特征。
~~~ 根据到这 13 个特征,这里的回归问题是预测波士顿郊区的房价中位数。
~~~ # 导入相关包
import tensorflow as tf
from sklearn.datasets import load_boston
# 标准化处理-将每一列特征标准化为标准正太分布
from sklearn.preprocessing import scale
import numpy as np
~~~ # 初始化变量
learning_rate = 0.01 # 学习率
epochs = 10000 # 迭代次数
display_epoch = epochs//20 # 控制训练过程中数据显示的频率
n_train = 300 # 60%训练集
n_valid = 100 # 20%验证集
# 读取数据
features, prices = load_boston(True)
n_test = len(features) - n_train - n_valid # 20%测试集
~~~ # 切分数据集&数据处理
# 训练集-#总样本的前60%
train_features = tf.cast(scale(features[:n_train]), dtype=tf.float32)
train_prices = prices[:n_train]
# 验证集样本-总样本的中间20%
valid_features = tf.cast(scale(features[n_train:n_train+n_valid]),
dtype=tf.float32)
valid_prices = prices[n_train:n_train+n_valid]
# 测试集样本-总样本的后20%
test_features = tf.cast(scale(features[n_train+n_valid:n_train+n_valid+n_test]),
dtype=tf.float32)
test_prices = prices[n_train + n_valid : n_train + n_valid + n_test]
### --- 定义相关函数
~~~ # 构建回归模型,返回预测值y
def prediction(x, weights, bias):
return tf.add(tf.matmul(x,weights), bias)
~~~ # 定义损失函数(均方根误差)
def loss(x, y, weights, bias):
error = prediction(x, weights, bias) - y #误差值
squared_error = tf.square(error) #计算平方
return tf.sqrt(tf.reduce_mean(input_tensor=squared_error)) #计算均值,得到均方根误差
~~~ # 定义梯度函数
# 样本数据[x,y]在参数[w,b]点上的梯度
def gradient(x, y, weights, bias):
with tf.GradientTape() as tape:
loss_value = loss(x, y, weights, bias)
return tape.gradient(loss_value, [weights, bias])
#
### --- 训练模型
# 初始化W、B
W = tf.Variable(tf.random.normal([13, 1]))
B = tf.Variable(tf.zeros(1) , dtype = tf.float32)print(W,B)
print("Initial loss: {:.3f}".format(loss(train_features, train_prices,W, B)))
# 训练模型
for e in range(epochs):
deltaW, deltaB = gradient(train_features, train_prices, W, B) # 得到weights、bias的均方根误差(导)
# 计算当前样本数据[x,y]在参数[w,b]点上的梯度
change_W = deltaW * learning_rate
change_B = deltaB * learning_rate
W.assign_sub(change_W) # 变量W变更为减去change_W后的值
B.assign_sub(change_B) # 变量B变更为减去change_B后的值
# 显示训练过程
if e==0 or e % display_epoch == 0:
# 验证集的均方根误差
print("Validation loss after epoch {:02d}: {:.3f}".format(e,loss(valid_features, valid_prices, W, B)))
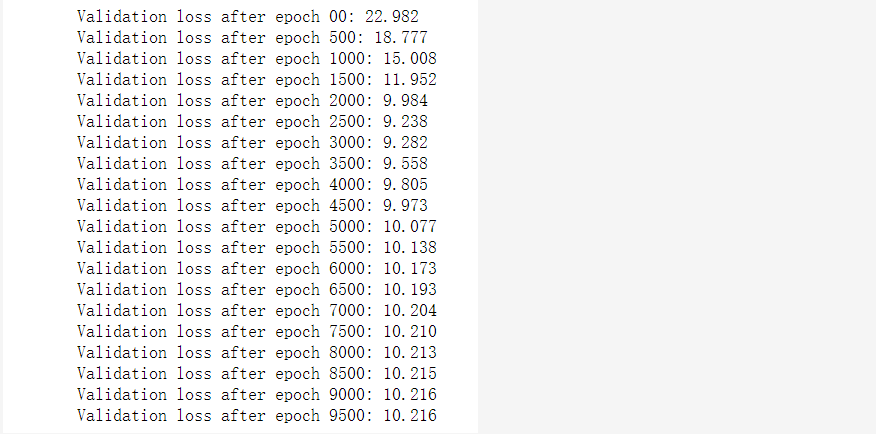
~~~ # 训练集的均方根误差
print("Final validation loss: {:.3f}".format(loss(train_features, train_prices,W, B)))
~~~ # 测试集的均方误差
print("Final test loss: {:.3f}".format(loss(test_features, test_prices, W, B)))
~~~ # 输出训练后的W和B
print("W = {}, B = {}".format(W.numpy(), B.numpy()))
~~~ # 预测值与真实值进行比对
example_house = 80
y = test_prices[example_house]
y_pred = prediction(test_features,W.numpy(),B.numpy())[example_house]
print("Actual Value",y," in $10K")
print("Predicted Value",y_pred.numpy()," in $10K")
Actual Value 23.0 in $10K
Predicted Value [25.39715] in $10K