#include <curl/curl.h>
#include <iostream>
#include <fstream>
#include <string>
#include <functional>
using namespace std;
struct CURLFunctionHelper
{
CURLFunctionHelper(CURL* curl
, CURLoption opt_function, CURLoption opt_data)
{
curl_easy_setopt(curl, opt_function, inner_function);
curl_easy_setopt(curl, opt_data, this);
}
template <typename Fn>
void Bind(Fn&& fn)
{
_callback = std::move(fn);
}
template <typename Fn, typename C>
void BindMember(Fn&& fn, C* c)
{
_callback = MakeMemberCallBack(fn, c);
}
private:
typedef std::function <size_t(char* , size_t, size_t)> CURLCallback;
template <typename Fn, typename C>
CURLCallback MakeMemberCallBack(Fn&& fn, C* c)
{
return std::bind(C::on_write, c, std::placeholders::_1
, std::placeholders::_2
, std::placeholders::_3);
}
static size_t inner_function(char* data, size_t size
, size_t nmemb, void* userdata)
{
auto self = static_cast <CURLFunctionHelper*> (userdata);
return self->_callback(data, size, nmemb);
}
CURLCallback _callback;
};
struct StdOutputer
{
size_t on_write(char* data, size_t size, size_t nmemb)
{
std::string line(data, size*nmemb);
cout << line;
return size*nmemb;
}
};
int main()
{
curl_global_init(CURL_GLOBAL_DEFAULT);
CURL* handle = curl_easy_init();
string url = "ftp://d2school:123456@127.0.0.1/:21";
curl_easy_setopt(handle, CURLOPT_URL, url.c_str());
CURLFunctionHelper helper(handle, CURLOPT_HEADERFUNCTION, CURLOPT_HEADERDATA);
//绑定一个函数对象,注意它的入参和返回值
StdOutputer outputer;
helper.Bind(std::bind(StdOutputer::on_write
, &outputer//this指针
, std::placeholders::_1, std::placeholders::_2
, std::placeholders::_3));
curl_easy_perform(handle);
curl_easy_cleanup(handle);
curl_global_cleanup();
return 0;
}
第13章 网络 13.6.3 实现多样化回调 02用模板实现更强大的回调 绑定非静态成员函数
于 2023-11-27 21:00:29 首次发布
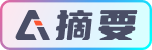