- 通过路径来绘制二维形状平面。简单理解就是在一个平面上用不规则的线连接成一个图形。
- 想在
three.js
中展示需要使用ExtrudeGeometry,ShapeGeometry
来生成几何体。 - 它有一个
.holes
属性,用于在形状平面中挖洞。.holes
值是一个THREE.Path()
数组,定义了二维路径。
常用绘图函数
moveTo(x, y)
将绘图点移动到指定的 x、y 坐标处。lineTo(x, y)
从当前位置创建一条到 x、y 坐标的线。quadricCurveTo(cpx, cpy, x, y)
创建一条到x、y 坐标的二次曲线。bezierCurveTo(cpx1, cpy1, cpx2, cpy2, x, y)
创建一条到x、y 坐标的贝塞尔曲线。splineThru(points)
沿着参数指定的坐标集合绘制一条光滑的样条曲线。
- 从一个或多个路径形状中创建一个单面多边形几何体。
new THREE.ShapeGeometry(shapes, curveSegments)
两个参数:
shapes
一个或多个形状(THREE.Shape对象)
。curveSegments
形状的分段数。
- 将一个二维图形拉伸成三维图形。
new THREE.ExtrudeGeometry(shapes, options)
两个参数:
shapes
一个或多个形状(THREE.Shape对象)
。options
拉伸成三维图形的配置参数。
绘制不规则图形
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>学习</title>
</head>
<body>
<canvas id="c2d" class="c2d" width="1000" height="500"></canvas>
<script type="module">
import * as THREE from './file/three.js-dev/build/three.module.js'
import { OrbitControls } from './file/three.js-dev/examples/jsm/controls/OrbitControls.js'
const canvas = document.querySelector('#c2d')
const renderer = new THREE.WebGLRenderer({ canvas })
const fov = 40
const aspect = 2
const near = 0.1
const far = 10000
const camera = new THREE.PerspectiveCamera(fov, aspect, near, far)
camera.position.set(0, 10, 10)
camera.lookAt(0, 0, 0)
const controls = new OrbitControls(camera, canvas)
controls.update()
const scene = new THREE.Scene()
function render() {
renderer.render(scene, camera)
requestAnimationFrame(render)
}
requestAnimationFrame(render)
</script>
</body>
</html>
const heartShape = new THREE.Shape()
heartShape.moveTo(0, 1.5)
heartShape.bezierCurveTo(2, 3.5, 4, 1.5, 2, -0.5)
heartShape.lineTo(0, -2.5)
heartShape.lineTo(-2, -0.5)
heartShape.bezierCurveTo(-4, 1.5, -2, 3.5, 0, 1.5)
心形平面
const geometry = new THREE.ShapeGeometry(heartShape)
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00, side: THREE.DoubleSide })
const mesh = new THREE.Mesh(geometry, material)
scene.add(mesh)
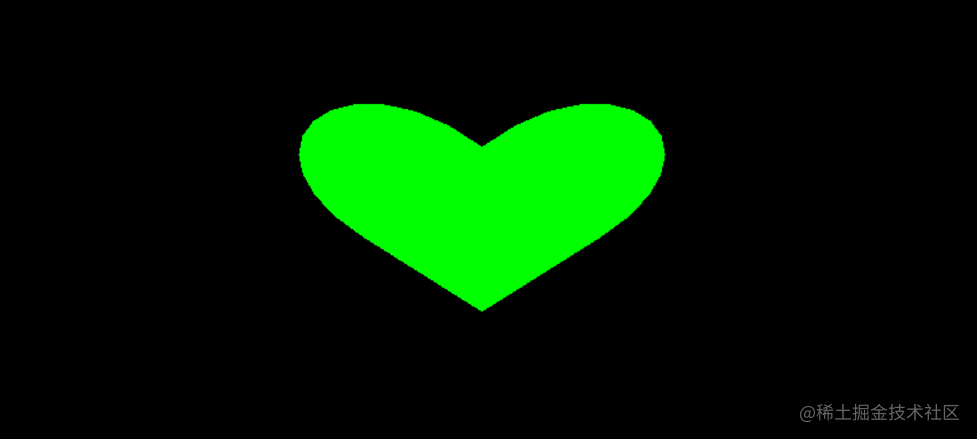
拉伸三维图形
- 使用
.ExtrudeGeometry
绘制三维图形。 - 增强立体感,在几何体上下都添加灯光。
{
const color = 0xffffff
const intensity = 1
const light = new THREE.DirectionalLight(color, intensity)
light.position.set(-1, 10, 4)
scene.add(light)
}
{
const color = 0xffffff
const intensity = 1
const light = new THREE.DirectionalLight(color, intensity)
light.position.set(-1, -10, -4)
scene.add(light)
}
const extrudeSettings = {
steps: 2,
depth: 3
}
- 使用
.ExtrudeGeometry
,拉伸为三维图形。
const geometry = new THREE.ExtrudeGeometry(heartShape, extrudeSettings)
const material = new THREE.MeshPhongMaterial({ color: 0x00ff00, side: THREE.DoubleSide })
const mesh = new THREE.Mesh(geometry, material)
scene.add(mesh)
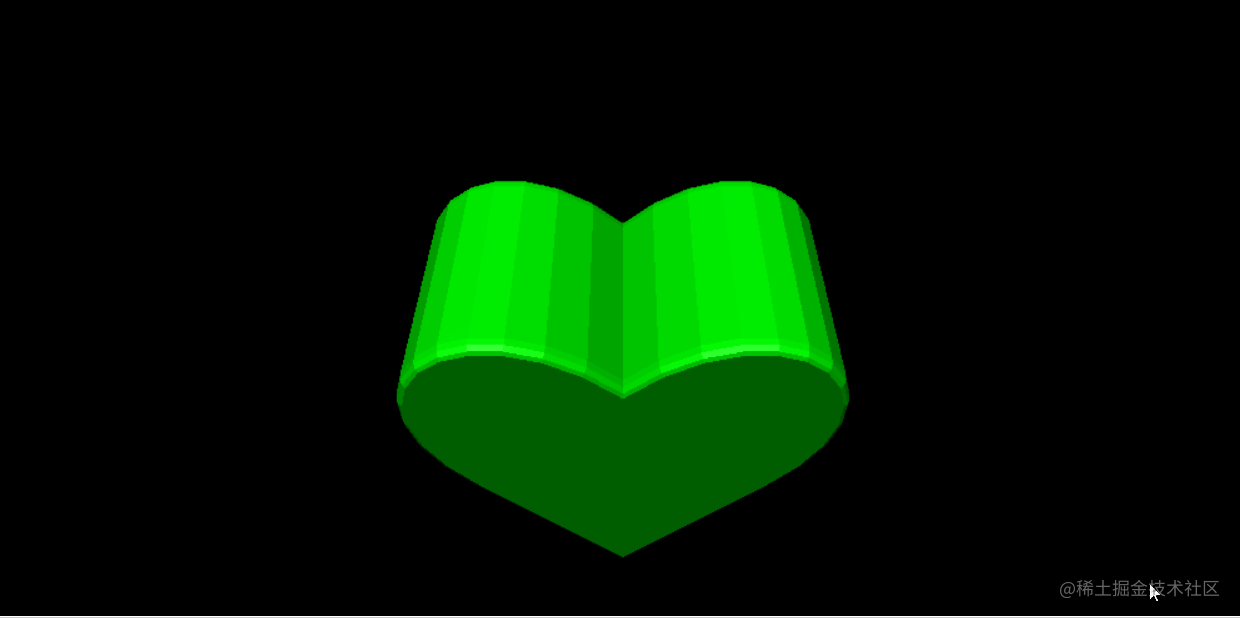
挖洞
- 使用
.Path
创建路径。放入形状的.holes
中。
const shape_c = new THREE.Path()
shape_c.moveTo(-1, 1)
shape_c.lineTo(-1, -1)
shape_c.lineTo(1, -1)
shape_c.lineTo(1, 1)
shape_c.lineTo(-1, 1)
heartShape.holes.push(shape_c)
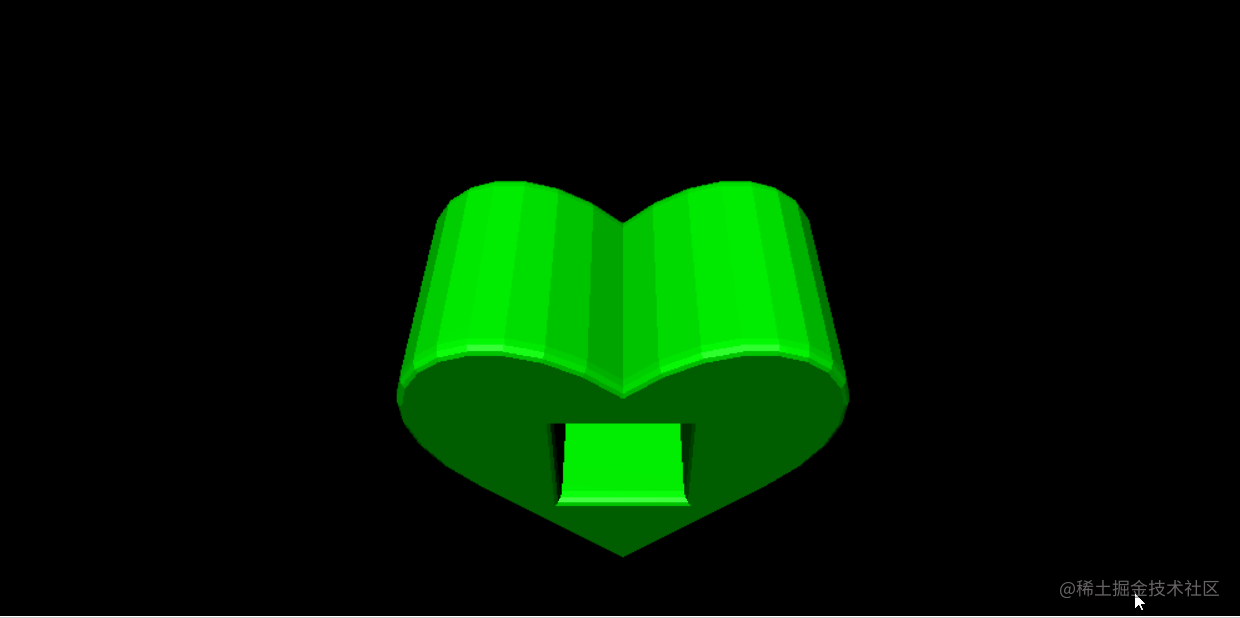
.ExtrudeGeometry
的配置参数有很多,需要详细了解的可以在官网查看后自己使用。