1.该实例包含两个activity,一个是MainActivi.java和布局文件activity_main.xml,主要用来收集用户的注册信息,另一个是SecondActivity.java与second.xml用来显示第一个activity中提交的结果。(本示例参考疯狂android讲义),下面分别是各个示例的代码和布局文件。
//MainActivity.java
package com.dragon.testevent
import android.app.Activity
import android.content.Intent
import android.os.Bundle
import android.view.View
import android.widget.Button
import android.widget.EditText
import android.widget.RadioButton
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
Button bn = (Button) findViewById(R.id.bn)
bn.setOnClickListener(new View.OnClickListener(){
public void onClick(View v){
EditText name = (EditText) findViewById(R.id.name)
EditText passwd = (EditText) findViewById(R.id.passwd)
RadioButton male = (RadioButton) findViewById(R.id.male)
String gender = male.isChecked()?"male":"female"
Person p = new Person(name.getText().toString(),passwd.getText().toString(),gender)
Bundle data = new Bundle()
data.putSerializable("person",p)
Intent intent = new Intent(MainActivity.this,SecondActivity.class)
intent.putExtras(data)
startActivity(intent)
}
})
}
}
//activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.dragon.testevent.MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="please input your information"
android:textSize="20sp"
/>
<TableRow>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="user name:"
android:textSize="20sp"
/>
<EditText
android:id="@+id/name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="please input you account"
android:selectAllOnFocus="true"
/>
</TableRow>
<TableRow>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="password"
android:textSize="16sp"
/>
<EditText
android:id="@+id/passwd"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:password="true"
android:selectAllOnFocus="true"/>
</TableRow>
<TableRow>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="gender:"
android:textSize="16sp"/>
<RadioGroup>
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
<RadioButton
android:id="@+id/male"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="male"
android:textSize="16sp"
/>
<RadioButton
android:id="@+id/female"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="female"
android:textSize="16sp"/>
</RadioGroup>
</TableRow>
<Button
android:id="@+id/bn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="regster"
android:textSize="16sp"
/>
</TableLayout>
package com.dragon.testevent;
import java.io.Serializable;
/**
* This file created by dragon on 2016/7/4 21:26,belong to com.dragon.testevent .
*/
public class Person implements Serializable {
private Integer id;
private String name;
private String passwd;
private String gender;
public Person(String name,String passwd,String gender){
this.name = name;
this.passwd = passwd;
this.gender = gender;
}
public Integer getId(){
return id;
}
public void setId(Integer id){
this.id =id;
}
public String getName(){
return name;
}
public void setName(String name){
this.name = name;
}
public String getPasswd(){
return passwd;
}
public void setPasswd(String passwd){
this.passwd = passwd;
}
public String getGender(){
return gender;
}
public void setGender(String gender){
this.gender= gender;
}
}
package com.dragon.testevent;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.widget.TextView;
/**
* This file created by dragon on 2016/7/4 19:46,belong to com.dragon.testevent .
*/
public class SecondActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
setContentView(R.layout.second);
TextView name = (TextView) findViewById(R.id.name);
TextView passwd =(TextView) findViewById(R.id.passwd);
TextView gender = (TextView) findViewById(R.id.gender);
Intent intent = getIntent();
Person p = (Person) intent.getSerializableExtra("person");
name.setText("your user name is :"+ p.getName());
passwd.setText("your password is :"+p.getPasswd());
gender.setText("your gender is :"+p.getGender());
}
}
//second.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp"/>
<TextView
android:id="@+id/passwd"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp"/>
<TextView
android:id="@+id/gender"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp"/>
</LinearLayout>
2.效果展示:
第一个activity
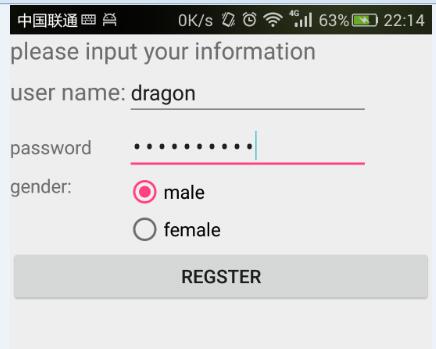
第二个activity
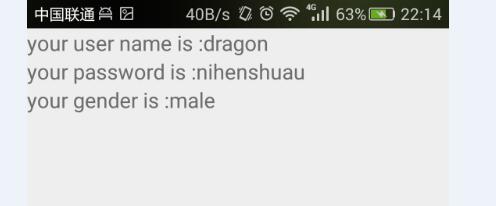