找色块游戏实现思路:
通过行列数创建所有色块,为了让每个色块均匀分布在游戏容器上,需要计算他的边距值margin,再去确定单一色块宽度,高度和宽度一样。点击开始游戏按钮,通过for循环创建色块,每次都要创建一个特殊色块,给特殊色块添加opacity属性,值小于1即可。具体通过难度来改变,难度越高opacity值越高,越不容易分辨(此功能未添加进代码中)。点击特殊色块,即为成功。行列数加1,得分加1。其他普通色块的opacity都为1,点击普通色块即为失败,会立即结束游戏,保存当前得分并上传到Bmob。点击结束游戏或者时间走到0都会立即结束游戏,并保存当前分数上传到Bmob。玩家还可通过得分进行pk(此功能目前还不完善)。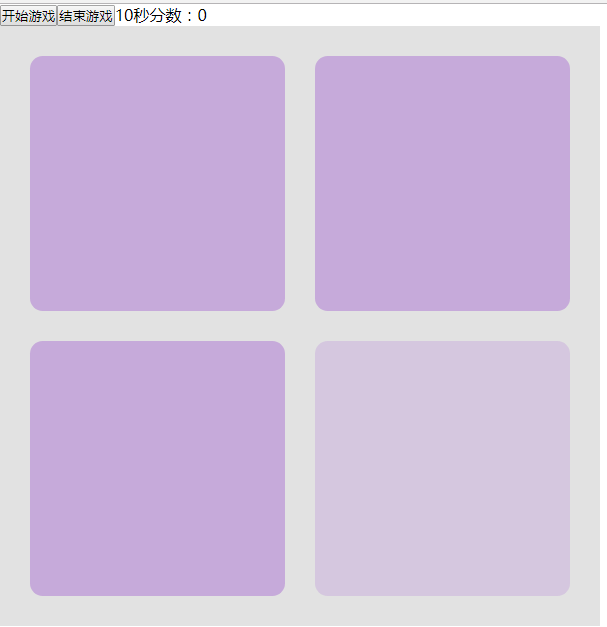
代码部分
index.html代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<style>
/*设置内外边距默认样式*/
*{
margin:0;
padding:0;
}
</style>
<body>
<!--//引入js文件-->
<script src="bmob-min.js"></script>
<script src="colorView.js"></script>
<script src="game.js"></script>
<script src="app.js"></script>
</body>
</html>
colorView.js代码:
// 声明findColor 定义在它上面的方法 可以在其他js文件中调用
window.findColor=window.findColor||{};
(function () {
/*
* info 字面量形式
* background 背景颜色
* opacity 不透明度
* row 行数
* containerWidth 容器宽度
* */
// 创建单一色块方法 需要传入一个样式对象
function ColorView(info) {
// 添加一个判断,在用户不传参数的时候也能继续运行
var _info=info||{};
// 创建单一色块
this.view=document.createElement("div");
// 宽度、高度、外边距、背景颜色、不透明度、浮动
// 间距比行数多1
var row=_info.row;
// 间距的数量
var spaceNum=row+1;
// 设置间距:多少行总宽度就平均分成多少份再除以合适的数字
var space=_info.containerWidth/row/10;
// 小方块的宽度=(容器总宽度-所有间距的宽度)/行数
var width=(_info.containerWidth-space*(spaceNum))/row;
// 设置单一色块样式
this.view.style.cssText="background-color:"+_info.background+";opacity:"+_info.opacity+";float:left;width:"+width+"px;height:"+width+"px;margin:"+space+"px 0 0 "+space+"px;border-radius:5%";
}
// 把ColorView定义在findColor上
findColor.ColorView=ColorView;
})();
game.js代码:
window.findColor=window.findColor||{};
(function () {
// 创建游戏类
function Game() {
// 调用控制工具函数
this.toolView();
// 调用游戏初始化方法
this.init();
// 定义timer为空
this.timer=null;
}
// 创建游戏初始化的方法
Game.prototype.init=function () {
// 定义变量最好是 创建属性 方便在其他函数中调用
// 创建row属性 默认为2行
this.row=2;
// 创建当前时间属性(定时器中用到)
this.currentTime=10;
// 创建得分属性
this.score=0;
// 在界面上显示当前时间
this.timeView.textContent=this.currentTime+"秒";
// 在界面上显示当前分数
this.scoreView.textContent="分数:"+this.score;
// 调用加载游戏数据方法
this.loadView();
};
// 创建控制工具方法
Game.prototype.toolView=function () {
// 创建工具容器
var toolContainer=document.createElement("div");
// 创建开始游戏按钮
var startButton=document.createElement("button");
// 创建结束游戏按钮
var stopButton=document.createElement("button");
// 给开始、结束按钮添加文本内容
startButton.textContent="开始游戏";
stopButton.textContent="结束游戏";
// 定义变量self替代this,改变this指向
var self=this;
// 给开始游戏按钮添加点击事件
startButton.onclick=function () {
// 调用开始游戏方法
self.start();
};
// 给结束游戏按钮添加点击事件
stopButton.onclick=function () {
// 调用结束游戏方法
self.stop();
};
// 将按钮添加到工具容器中
toolContainer.appendChild(startButton);
toolContainer.appendChild(stopButton);
// 将工具容器添加到body中
document.body.appendChild(toolContainer);
// 创建一个在页面上显示时间的变量
var timeView=document.createElement("span");
// 创建一个在页面上显示分数的变量
var scoreView=document.createElement("span");
// 添加到工具容器中
toolContainer.appendChild(timeView);
toolContainer.appendChild(scoreView);
// 定义两个属性接收创建的变量 (优化代码)
this.timeView=timeView;
this.scoreView=scoreView;
};
// 创建加载游戏数据的方法(核心)
Game.prototype.loadView=function () {
// 判断 如果有this.gameContent则清除它(调试时候发现的bug 否则点击一次就会按顺序排列下去)
if(this.gameContent){
this.gameContent.parentNode.removeChild(this.gameContent);
}
// 创建随机的颜色
var color = "rgb("+parseInt(Math.random()*255)+","+parseInt(Math.random()*255)+","+parseInt(Math.random()*255)+")";
// createDocumentFragment 创建文档的碎片元素 在使用循环 添加子元素到父元素的时候 可以使用它 节省没戏给真实父元素 添加子元素的开销(优化代码)
var temp=document.createDocumentFragment();
// 创建色块的个数:行数*列数(行数)
var num=this.row*this.row;
// 创建一个num范围内的随机数
var id=parseInt(Math.random()*num);
var self=this;
// 通过for循环创建单一色块
for(var i=0;i<num;i++){
// 定义变量
var item=null;
// 通过判断 创建一个特殊色块
if(i===id){
// 特殊色块
// 创建单一色块对象ColorView() 传入参数,由于是特殊色块,不透明度要小于1,具体要根据难度改变
item=new findColor.ColorView({background:color,opacity:0.5,row:this.row,containerWidth:600}).view;
// 添加点击事件
item.onclick=function () {
// 成功
// 判断如果没有开启定时器 直接跳出(调试出现的bug,否则游戏结束后仍有定时器存在,导致游戏继续运行)
if(!self.timer){return}
// 行数加1
++self.row;
// 得分加1
++self.score;
// 调用更新时间和分数的方法
self.updateView();
// 调用加载游戏数据的方法
self.loadView();
}
}else {
// 普通色块
item=new findColor.ColorView({background:color,opacity:1,row:this.row,containerWidth:600}).view;
item.onclick=function () {
// 失败
if(!self.timer){return}
self.updateView();
// 失败后调用结束游戏方法
self.stop();
}
}
// 将创建出来的单一色块添加到碎片元素上面
temp.appendChild(item);
}
// 创建游戏容器
this.gameContent=document.createElement("div");
// 设置样式
this.gameContent.style.cssText="width:600px;height:600px;background:#e2e2e2";
// 将碎片元素添加到游戏容器上
this.gameContent.appendChild(temp);
// 将游戏容器添加到body上
document.body.appendChild(this.gameContent);
};
// 游戏开始的方法
Game.prototype.start=function () {
// 判断如果有定时器直接跳出
if(this.timer){return}
// 开启定时器
this.timer=setInterval(function () {
// 每秒钟减少当前时间-1
--this.currentTime;
// 调用更新时间和分数的方法
this.updateView();
// 判断如果当前时间为0,结束游戏
if(this.currentTime==0){
this.stop();
}
// 绑定this
}.bind(this),1000)
};
// 游戏结束的方法
Game.prototype.stop=function () {
// 清除定时器
clearInterval(this.timer);
// 将定时器对象设为null(释放)
this.timer=null;
// 调用保存分数的方法
this.saveScore();
// 调用与其他玩家pk的方法
this.PK();
// 调用游戏初始化方法:将界面还原为初始界面
this.init();
};
// 更新当前时间和当前分数的方法
Game.prototype.updateView=function () {
this.timeView.textContent=this.currentTime+"秒";
this.scoreView.textContent="分数:"+this.score;
};
// 与其他玩家pk分数的方法 代码参考Bmob文档中查询数据的方法,具体查询条件见文档 此方法目前还不完善
Game.prototype.PK=function () {
// var self = this;
var GameScore = Bmob.Object.extend("GameScore");
var query = new Bmob.Query(GameScore);
query.equalTo("userID",Bmob.User.current().id);
query.descending("score");
// 查询所有数据
query.find({
success: function(results) {
console.log(results);
var obj = results[0].attributes;
self.score >obj.score?alert("你赢了!"):alert("你输了!");
},
error: function(error) {
alert("查询失败: " + error.code + " " + error.message);
}
});
};
// 保存分数的方法 代码参考Bmob文档中添加数据的方法
Game.prototype.saveScore=function () {
var GameScore = Bmob.Object.extend("GameScore");
var gameScore = new GameScore();
gameScore.save({score:this.score,userID:Bmob.User.current().id}, {
success: function(gameScore) {
alert("上传分数成功")
},
error: function(gameScore, error) {
alert('添加数据失败,返回错误信息:' + error.description);
}
});
};
// 将Game定义在findColor上
findColor.Game=Game;
})();
app.js代码:
// 创建初始化游戏的函数
function init() {
// Bmob的操作方法具体查看Bmob文档
// Bmob初始化方法
Bmob.initialize("e867d11b0972f8f34b77ab79d6618cc9","eded1275a6165be15297521e9f209c1f");
// Bmob注册方法
new Bmob.User().signUp({username:"zhanghao",password:"11111"});
// Bomb登录方法
Bmob.User.logIn("zhanghao", "11111");
// 创建找色块游戏对象
new findColor.Game();
}
init();