功能:通过PC机的串口调试助手发送数据给串口A,串口B接收到串口A发送的数据,再由串口B将接收到的数据返回给PC机的串口调试助手。
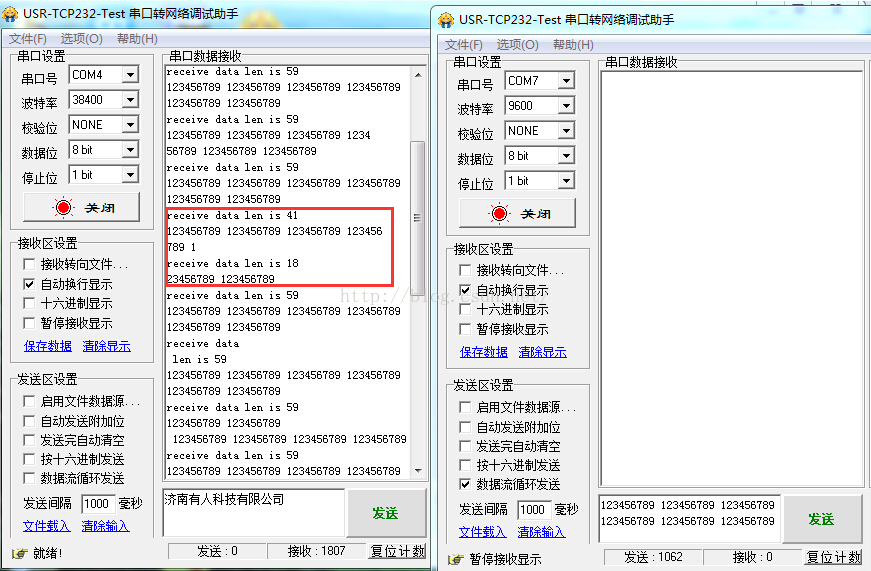
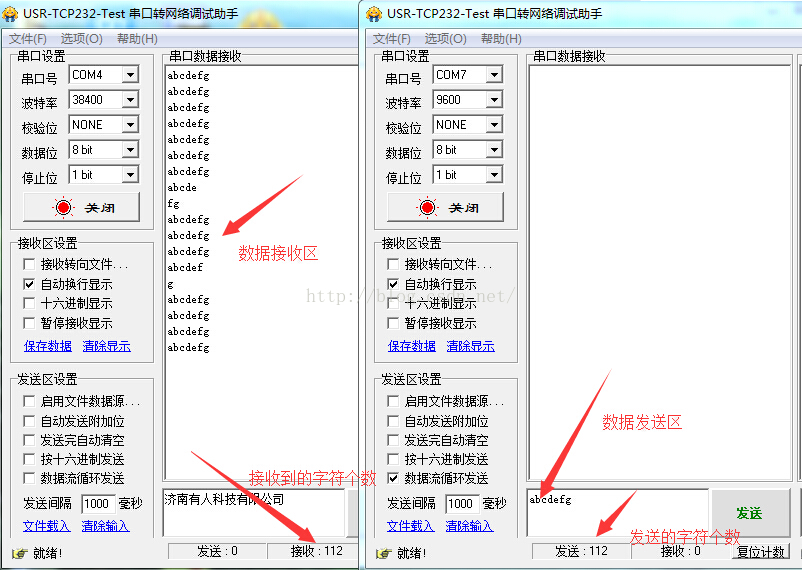
PC->串口A->串口B->PC。
实验平台:stm32f407
/*********************************************************************
本平台具有六个串口:
com1 485
com2
com3 232 需一个管脚控制DTU
com4 485
com5 调试串口 TTL
com6 485
*********************************************************************/
本实验用的的串口A对应为com1,串口B对应为com4。
本程序的设计思路:
通过PC机串口调试助手发送数据,当串口A发现有数据过来时,产生串口接收中断,把数据保存到自定义的接收缓冲区中,然后串口接收函数去缓冲区中去取数据保存在发送缓冲区中,再将数据通过串口B发送给串口调试助手。
代码:
multiple_serial.h
-
#ifndef multiple_serial_H
-
#define multiple_serial_H
- #define MAX_RECV_BUF_LEN (64)
-
#define MAX_RECV_DATA_MASK (0x3f)
-
#define MAX_COM_COUNT (6)
-
-
typedef signed char int8_t;
-
typedef signed short intint16_t;
-
typedef signed int int32_t;
-
-
typedef unsigned char uint8_t;
-
typedef unsigned short intuint16_t;
-
typedef unsigned int uint32_t;
-
-
#define NEXTAI_COM1_NAME"COM1"
-
#define NEXTAI_COM2_NAME"COM2"
-
#define NEXTAI_COM3_NAME"COM3"
-
#define NEXTAI_COM4_NAME"COM4"
-
#define NEXTAI_COM5_NAME"COM5"
-
#define NEXTAI_COM6_NAME"COM6"
-
-
enum{
-
NEXTAI_COM1_ID=1,
-
NEXTAI_COM2_ID,
-
NEXTAI_COM3_ID,
-
NEXTAI_COM4_ID,
-
NEXTAI_COM5_ID,
-
NEXTAI_COM6_ID
-
};
-
-
typedef struct _NEXTAI_CHANNEL_INFO_
-
{
-
uint8_t local_com_id;
-
//nextai_uint8 *databuf;
-
//nextai_uint16 data_read_p;
-
//nextai_uint16 data_write_p;
-
-
uint8_t used_flag;
-
}NEXTAI_CHANNEL_INFO;
-
-
/*******************************************************************************
-
* local_channel_data : 通道数据结构
-
* data_recv_flag : 是否有数据flag,1表示
-
*******************************************************************************/
-
typedef struct _NEXTAI_MGR_INFO_
-
{
-
NEXTAI_CHANNEL_INFO local_channel_data[MAX_COM_COUNT];
-
uint8_t data_recv_flag;
-
}NEXTAI_MGR_INFO;
-
-
static NEXTAI_MGR_INFO* local_com_mgr_p=0;
-
void serial_nvic_Configuration(uint8_t comID);
-
static void serial_gpio_configuration(uint8_t comID);
-
static void serial_rcc_configuration(uint8_t comID);
-
uint8_t serial_Configuration(uint8_t*comName);
-
uint8_t serial_send(uint8_t comID,uint8_t*databuf,uint32_t datalen);
-
uint32_t serial_recv(uint8_t comID,uint8_t*databuf,uint32_t datalen);
-
void clean_rebuff(void);
-
#endif
multiple_serial.c
-
#include"multiple_serial.h"
-
#include"stm32f4xx_gpio.h"
-
#include"stm32f4xx_usart.h"
-
#include<string.h>
-
-
unsigned char uart_buf[MAX_RECV_BUF_LEN]={0};
-
-
uint8_t com1_recv_buf[MAX_RECV_BUF_LEN]={0};
-
int com1_gloabl_p=0;
-
int com1_read_p=0;
-
-
uint8_t com4_recv_buf[MAX_RECV_BUF_LEN]={0};
-
int com4_gloabl_p=0;
-
int com4_read_p=0;
-
-
uint8_t com5_recv_buf[MAX_RECV_BUF_LEN]={0};
-
int com5_gloabl_p=0;
-
int com5_read_p=0;
-
-
uint8_t com6_recv_buf[MAX_RECV_BUF_LEN]={0};
-
int com6_gloabl_p=0;
-
int com6_read_p=0;
-
-
//串口gpio的配置
-
static void serial_gpio_configuration(uint8_t comID)
-
{
-
GPIO_InitTypeDef GPIO_InitStructure;
-
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
-
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOC, ENABLE);
-
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOD, ENABLE);
-
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOF, ENABLE);
-
-
/* Configure USART Tx and Rx as alternate function push-pull */
-
if(comID == NEXTAI_COM1_ID)
-
{
-
GPIO_StructInit(&GPIO_InitStructure);
-
-
GPIO_PinAFConfig(GPIOA, GPIO_PinSource9, GPIO_AF_USART1);
-
GPIO_PinAFConfig(GPIOA, GPIO_PinSource10, GPIO_AF_USART1);
-
-
GPIO_InitStructure.GPIO_Mode= GPIO_Mode_AF;
-
GPIO_InitStructure.GPIO_Speed= GPIO_Speed_100MHz;
-
GPIO_InitStructure.GPIO_OType= GPIO_OType_PP;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_9;
-
GPIO_Init(GPIOA,&GPIO_InitStructure);
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_10;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
GPIO_Init(GPIOA,&GPIO_InitStructure);
-
-
GPIO_StructInit(&GPIO_InitStructure);
-
GPIO_InitStructure.GPIO_Mode= GPIO_Mode_OUT;
-
GPIO_InitStructure.GPIO_Speed= GPIO_Speed_100MHz;
-
GPIO_InitStructure.GPIO_OType= GPIO_OType_PP;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_4;
-
-
GPIO_Init(GPIOF,&GPIO_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM4_ID)
-
{
-
GPIO_StructInit(&GPIO_InitStructure);
-
-
GPIO_PinAFConfig(GPIOA, GPIO_PinSource0, GPIO_AF_UART4);
-
GPIO_PinAFConfig(GPIOA, GPIO_PinSource1, GPIO_AF_UART4);
-
-
GPIO_InitStructure.GPIO_Mode= GPIO_Mode_AF;
-
GPIO_InitStructure.GPIO_Speed= GPIO_Speed_50MHz;
-
GPIO_InitStructure.GPIO_OType= GPIO_OType_PP;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_1;
-
GPIO_Init(GPIOA,&GPIO_InitStructure);
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_0;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
GPIO_Init(GPIOA,&GPIO_InitStructure);
-
-
GPIO_StructInit(&GPIO_InitStructure);
-
GPIO_InitStructure.GPIO_Mode= GPIO_Mode_OUT;
-
GPIO_InitStructure.GPIO_Speed= GPIO_Speed_50MHz;
-
GPIO_InitStructure.GPIO_OType= GPIO_OType_PP;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_7;
-
-
GPIO_Init(GPIOA,&GPIO_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM5_ID)
-
{
-
GPIO_StructInit(&GPIO_InitStructure);
-
-
GPIO_PinAFConfig(GPIOC, GPIO_PinSource12, GPIO_AF_UART5);
-
GPIO_PinAFConfig(GPIOD, GPIO_PinSource2, GPIO_AF_UART5);
-
-
GPIO_InitStructure.GPIO_Mode= GPIO_Mode_AF;
-
GPIO_InitStructure.GPIO_Speed= GPIO_Speed_50MHz;
-
GPIO_InitStructure.GPIO_OType= GPIO_OType_PP;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_2;
-
GPIO_Init(GPIOD,&GPIO_InitStructure);
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_12;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
GPIO_Init(GPIOC,&GPIO_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM6_ID)
-
{
-
GPIO_StructInit(&GPIO_InitStructure);
-
-
GPIO_PinAFConfig(GPIOC, GPIO_PinSource6, GPIO_AF_USART6);
-
GPIO_PinAFConfig(GPIOC, GPIO_PinSource7, GPIO_AF_USART6);
-
-
GPIO_InitStructure.GPIO_Mode= GPIO_Mode_AF;
-
GPIO_InitStructure.GPIO_Speed= GPIO_Speed_100MHz;
-
GPIO_InitStructure.GPIO_OType= GPIO_OType_PP;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_6;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
GPIO_Init(GPIOC,&GPIO_InitStructure);
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_7;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
GPIO_Init(GPIOC,&GPIO_InitStructure);
-
-
GPIO_StructInit(&GPIO_InitStructure);
-
GPIO_InitStructure.GPIO_Mode= GPIO_Mode_OUT;
-
GPIO_InitStructure.GPIO_Speed= GPIO_Speed_50MHz;
-
GPIO_InitStructure.GPIO_OType= GPIO_OType_PP;
-
GPIO_InitStructure.GPIO_PuPd= GPIO_PuPd_UP;
-
-
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_8;
-
GPIO_Init(GPIOC,&GPIO_InitStructure);
-
}
-
}
-
-
//串口时钟的配置
-
static void serial_rcc_configuration(uint8_t comID)
-
{
- /* Enable USART clock */
-
if(comID== NEXTAI_COM1_ID)
-
{
-
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
-
}
-
elseif(comID== NEXTAI_COM4_ID)
-
{
-
RCC_APB1PeriphClockCmd(RCC_APB1Periph_UART4,ENABLE);
-
}
-
elseif(comID== NEXTAI_COM5_ID)
-
{
-
RCC_APB1PeriphClockCmd(RCC_APB1Periph_UART5,ENABLE);
-
}
-
elseif(comID== NEXTAI_COM6_ID)
-
{
-
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART6, ENABLE);
-
}
-
}
-
-
//配置各个串口的中断处理函数,优先级相同
-
void serial_nvic_Configuration(uint8_t comID)
-
{
-
NVIC_InitTypeDef NVIC_InitStructure;
-
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
-
-
if(comID== NEXTAI_COM1_ID)
-
{
-
NVIC_InitStructure.NVIC_IRQChannel= USART1_IRQn;
-
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelSubPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelCmd= ENABLE;
-
NVIC_Init(&NVIC_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM4_ID)
-
{
-
NVIC_InitStructure.NVIC_IRQChannel= UART4_IRQn;
-
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelSubPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelCmd= ENABLE;
-
NVIC_Init(&NVIC_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM5_ID)
-
{
-
NVIC_InitStructure.NVIC_IRQChannel= UART5_IRQn;
-
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelSubPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelCmd= ENABLE;
-
NVIC_Init(&NVIC_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM6_ID)
-
{
-
NVIC_InitStructure.NVIC_IRQChannel= USART6_IRQn;
-
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelSubPriority=1;
-
NVIC_InitStructure.NVIC_IRQChannelCmd= ENABLE;
-
NVIC_Init(&NVIC_InitStructure);
-
}
-
}
-
-
-
/*串口参数配置*/
-
uint8_t serial_Configuration(uint8_t*comName)
-
{
-
USART_InitTypeDef USART_InitStructure;
-
uint8_t comID =0;
-
-
if(strcmp(NEXTAI_COM1_NAME,(constchar*)comName)==0)
-
{
-
comID= NEXTAI_COM1_ID;
-
}
-
elseif(strcmp(NEXTAI_COM4_NAME,(constchar*)comName)==0)
-
{
-
comID= NEXTAI_COM4_ID;
-
}
-
elseif(strcmp(NEXTAI_COM5_NAME,(constchar*)comName)==0)
-
{
-
comID= NEXTAI_COM5_ID;
-
}
-
elseif(strcmp(NEXTAI_COM6_NAME,(constchar*)comName)==0)
-
{
-
comID= NEXTAI_COM6_ID;
-
}
-
-
/* System Clocks Configuration */
-
serial_rcc_configuration(comID);
-
-
/* Configure the GPIO ports */
-
serial_gpio_configuration(comID);
-
-
USART_InitStructure.USART_WordLength= USART_WordLength_8b;
-
USART_InitStructure.USART_Parity= USART_Parity_No;
-
USART_InitStructure.USART_HardwareFlowControl= USART_HardwareFlowControl_None;
-
USART_InitStructure.USART_Mode= USART_Mode_Rx| USART_Mode_Tx;
-
-
if(comID== NEXTAI_COM1_ID)
-
{
-
USART_InitStructure.USART_BaudRate=9600;
-
USART_InitStructure.USART_StopBits= USART_StopBits_1;
-
USART_Init(USART1,&USART_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM4_ID)
-
{
-
USART_InitStructure.USART_BaudRate=38400;
-
USART_InitStructure.USART_StopBits= USART_StopBits_1;
-
USART_Init(UART4,&USART_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM5_ID)
-
{
-
USART_InitStructure.USART_BaudRate=115200;
-
USART_InitStructure.USART_StopBits= USART_StopBits_1;
-
USART_Init(UART5,&USART_InitStructure);
-
}
-
elseif(comID== NEXTAI_COM6_ID)
-
{
-
USART_InitStructure.USART_BaudRate=9600;
-
USART_InitStructure.USART_StopBits= USART_StopBits_1;
-
USART_Init(USART6,&USART_InitStructure);
-
}
-
-
serial_nvic_Configuration(comID);
-
-
if(comID== NEXTAI_COM1_ID)
-
{
-
USART_Cmd(USART1, ENABLE);
-
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
-
}
-
elseif(comID== NEXTAI_COM4_ID)
-
{
-
USART_Cmd(UART4, ENABLE);
-
USART_ITConfig(UART4, USART_IT_RXNE, ENABLE);
-
}
-
elseif(comID== NEXTAI_COM5_ID)
-
{
-
USART_Cmd(UART5, ENABLE);
-
USART_ITConfig(UART5, USART_IT_RXNE, ENABLE);
-
}
-
elseif(comID== NEXTAI_COM6_ID)
-
{
-
USART_Cmd(USART6, ENABLE);
-
USART_ITConfig(USART6, USART_IT_RXNE, ENABLE);
-
}
-
-
return comID;
-
}
-
-
/*******************************************************************************
-
* 函数名 : serial_send
-
* 描 述 : 发送某个端口的数据
-
* 输 入 : comID: 串口标识号
-
databuf: 发送数据缓冲区
-
datalen: 发送数据长度
-
* 输 出 : None
-
* 返 回 : None
-
*******************************************************************************/
-
uint8_t serial_send(uint8_t comID,uint8_t*databuf,uint32_t datalen)
-
{
-
int i =0;
-
uint8_t nextai_timer =0;
-
-
if(comID== NEXTAI_COM1_ID)
-
{
-
GPIO_SetBits(GPIOF, GPIO_Pin_4);
-
-
//清除标志位,否则第1位数据会丢失
-
USART_GetFlagStatus(USART1, USART_FLAG_TC);
-
-
for(i=0; i< datalen; i++)
-
{
-
//485 需添加超时检测
-
USART_SendData(USART1,databuf[i]);
-
-
while(USART_GetFlagStatus(USART1, USART_FLAG_TC)== RESET)
-
{
-
nextai_timer++;
-
if(nextai_timer>>8)
-
return0;
-
}
-
}
-
GPIO_ResetBits(GPIOF, GPIO_Pin_4);
-
}
-
elseif(comID== NEXTAI_COM4_ID)
-
{
-
GPIO_SetBits(GPIOA, GPIO_Pin_7);
-
-
//清除标志位,否则第1位数据会丢失
-
USART_GetFlagStatus(UART4, USART_FLAG_TC);
-
-
for(i=0; i< datalen; i++)
-
{
-
//485
-
USART_SendData(UART4,databuf[i]);
-
while(USART_GetFlagStatus(UART4, USART_FLAG_TC)== RESET)
-
{
-
nextai_timer++;
-
if(nextai_timer>>8)
-
return0;
-
}
-
}
-
GPIO_ResetBits(GPIOA, GPIO_Pin_7);
-
}
-
elseif(comID== NEXTAI_COM5_ID)
-
{
-
//清除标志位,否则第1位数据会丢失
-
USART_GetFlagStatus(UART5,USART_FLAG_TC);
-
for(i=0; i< datalen; i++)
-
{
- while(USART_GetFlagStatus(UART5, USART_FLAG_TC)== RESET);
-
USART_SendData(UART5,databuf[i]);
-
while(USART_GetFlagStatus(UART5, USART_FLAG_TC)== RESET)
-
{
-
nextai_timer++;
-
if(nextai_timer>>8)
-
return0;
-
}
-
}
-
}
-
elseif(comID== NEXTAI_COM6_ID)
-
{
-
GPIO_SetBits(GPIOC, GPIO_Pin_8);
-
-
//清除标志位,否则第1位数据会丢失
-
USART_GetFlagStatus(USART6,USART_FLAG_TC);
-
for(i=0; i< datalen; i++)
-
{
- //485
-
USART_SendData(USART6,databuf[i]);
-
while(USART_GetFlagStatus(USART6, USART_FLAG_TC)== RESET)
-
{
-
nextai_timer++;
-
if(nextai_timer>>8)
-
return0;
-
}
-
}
-
GPIO_ResetBits(GPIOC, GPIO_Pin_8);
-
}
-
-
return datalen;
-
}
-
-
/*******************************************************************************
-
* 函数名 : serial_recv
-
* 描 述 : 接收一定长度的数据(从内存中读取数据)
-
* 输 入 : comID: 串口标识号
-
databuf: 接收数据缓冲区
-
datalen: 需要接收数据长度
-
* 输 出 : None
-
* 返 回 : 实际接收到的数据长度
-
*******************************************************************************/
-
uint32_t serial_recv(uint8_t comID,uint8_t*databuf,uint32_t datalen)
-
{
-
uint16_t i =0, nextai_indx=0, j=0;
-
NEXTAI_MGR_INFO*me= local_com_mgr_p;
-
-
if((comID> NEXTAI_COM6_ID)||(comID< NEXTAI_COM1_ID))
-
return 0xffffffff;
-
nextai_indx= comID- NEXTAI_COM1_ID;
-
-
if(me->local_channel_data[nextai_indx].used_flag==0)
-
return 0xffffffff;
-
-
if(!(me->data_recv_flag&(1<< nextai_indx)))
-
return 0xffffffff;
-
-
if(comID== NEXTAI_COM1_ID)
-
{
-
i=0;
-
while(1)
-
{
-
if(com1_read_p== com1_gloabl_p)
-
break;
-
databuf[i]= com1_recv_buf[com1_read_p];
-
com1_read_p++;
-
com1_read_p&= MAX_RECV_DATA_MASK;
-
i++;
-
if(i>= datalen)
-
break;
-
for(j=0; j<100; j++);
-
}
-
me->data_recv_flag= me->data_recv_flag&(~(1<< nextai_indx));
-
}
-
-
if(comID== NEXTAI_COM4_ID)
-
{
-
i=0;
-
while(1)
-
{
-
if(com4_read_p== com4_gloabl_p)
-
break;
-
databuf[i]= com4_recv_buf[com4_read_p];
-
com4_read_p++;
-
com4_read_p&= MAX_RECV_DATA_MASK;
-
i++;
-
if(i>= datalen)
-
break;
-
for(j=0; j<100; j++);
-
}
-
me->data_recv_flag= me->data_recv_flag&(~(1<< nextai_indx));
-
}
-
-
if(comID== NEXTAI_COM5_ID)
-
{
-
i=0;
-
while(1)
-
{
-
if(com5_read_p== com5_gloabl_p)
-
break;
-
databuf[i]= com5_recv_buf[com5_read_p];
-
com5_read_p++;
-
com5_read_p&= MAX_RECV_DATA_MASK;
-
i++;
-
if(i>= datalen)
-
break;
-
for(j=0; j<100; j++);
-
}
-
me->data_recv_flag= me->data_recv_flag&(~(1<< nextai_indx));
-
}
-
-
if(comID== NEXTAI_COM6_ID)
-
{
-
i=0;
-
while(1)
-
{
-
if(com6_read_p== com6_gloabl_p)
-
break;
-
databuf[i]= com6_recv_buf[com6_read_p];
-
com6_read_p++;
-
com6_read_p&= MAX_RECV_DATA_MASK;
-
i++;
-
if(i>= datalen)
-
break;
-
for(j=0; j<100; j++);
-
}
-
me->data_recv_flag= me->data_recv_flag&(~(1<< nextai_indx));
-
}
-
-
return i;
-
}
-
-
//中断处理函数只是将发送过来的数据保存到内存
-
void USART1_IRQHandler(void)
-
{
-
NEXTAI_MGR_INFO*me= local_com_mgr_p;
-
-
if(USART_GetITStatus(USART1, USART_IT_RXNE)!= RESET)
-
{
-
USART_ClearITPendingBit(USART1,USART_IT_RXNE);
-
com1_recv_buf[com1_gloabl_p]= USART_ReceiveData(USART1);
-
com1_gloabl_p++;
-
com1_gloabl_p&= MAX_RECV_DATA_MASK;
-
}
-
-
me->data_recv_flag|=0x01;
- }
-
- //void UART5_IRQHandler(void)
-
//{
-
// uint8_t temp;
-
// if(USART_GetITStatus(UART5, USART_IT_RXNE)!= RESET)
-
// {
-
// temp= USART_ReceiveData(UART5);
-
// USART_SendData(UART5,temp);
-
// }
-
//}
-
-
void UART4_IRQHandler(void)
-
{
-
NEXTAI_MGR_INFO*me= local_com_mgr_p;
-
-
if(USART_GetITStatus(UART4, USART_IT_RXNE)!= RESET)
-
{
-
com4_recv_buf[com4_gloabl_p++]= USART_ReceiveData(UART4);
-
com4_gloabl_p&= MAX_RECV_DATA_MASK;
-
}
-
-
me->data_recv_flag|=0x08;
-
}
-
-
void UART5_IRQHandler(void)
-
{
-
NEXTAI_MGR_INFO *me = local_com_mgr_p;
-
-
if(USART_GetITStatus(UART5, USART_IT_RXNE) != RESET)
-
{
-
com5_recv_buf[com5_gloabl_p++] = USART_ReceiveData(UART5);
-
com5_gloabl_p &= MAX_RECV_DATA_MASK;
-
}
-
-
me->data_recv_flag |= 0x10;
-
}
-
-
void USART6_IRQHandler(void)
-
{
-
NEXTAI_MGR_INFO*me= local_com_mgr_p;
-
-
if(USART_GetITStatus(USART6, USART_IT_RXNE)!= RESET)
-
{
-
com6_recv_buf[com6_gloabl_p++]= USART_ReceiveData(USART6);
-
com6_gloabl_p&= MAX_RECV_DATA_MASK;
-
}
-
-
me->data_recv_flag|=0x20;
-
}
main.c
-
#include"multiple_serial.h"
-
#include"stm32f4xx_gpio.h"
-
#include"stm32f4xx_usart.h"
-
#include<string.h>
-
#include<stdio.h>
-
-
int main()
-
{
-
char*COM1="COM1";
-
char*COM4="COM4";
-
-
serial_Configuration((uint8_t*)COM1);
-
serial_Configuration((uint8_t*)COM4);
-
-
extern unsigned char uart_buf[64];
-
-
int i=0,j;
-
-
unsigned char printf_buf[128]={0};
-
-
uint8_t flag=0;
-
-
while(1)
-
{
-
flag= serial_recv(1, uart_buf,64);
-
//延时让serial_recv函数接收完PC机串口调试助手发来的全部数据
-
for(i=10000;i!=0;i--)
-
for(j=3000;j!=0;j--);
-
-
if(flag>0)
-
{
-
sprintf((char*)printf_buf,"receive data len is %d\n", flag);//将字符串写入printf_buf中
-
serial_send(4, printf_buf, strlen((char*)printf_buf));//发送printf_buf中的内容到串口
-
-
serial_send(4, uart_buf, flag+1);
-
-
}
-
-
}
-
-
return 0;
-
}
测试结果如下图所示:
刚才改了下程序:
main.c
-
#include"multiple_serial.h"
-
#include"stm32f4xx_gpio.h"
-
#include"stm32f4xx_usart.h"
-
#include<string.h>
-
#include<stdio.h>
-
-
int main()
-
{
-
char*COM1="COM1";
-
char*COM4="COM4";
-
char*COM5="COM5";
-
char*COM6="COM6";
-
-
serial_Configuration((uint8_t*)COM1);
-
serial_Configuration((uint8_t*)COM6);
-
extern unsigned char uart_buf[64];
- int i=0,j;
-
unsigned char printf_buf[128]={0};
- uint8_t flag=0;
-
-
while(1)
-
{
-
//从串口调试助手发送数据,串口A接收到数据后产生中断,在中断函数中将数据保存到com6_recv_buf中,
-
//然后通过serial_recv函数将数据从com6_recv_buf中保存到接收uart_buf中,最后调用serial_send回传给串口调试助手。
-
flag= serial_recv(1, uart_buf,64);
-
-
if(flag>0)
-
{
-
serial_send(4, uart_buf, flag+1);
-
}
-
}
-
-
return0;
-
}
测试结果如下图所示:
通过发送和接收的数据对比,发现程序设计的还是比较稳定的,没有出现数据丢失的现象。