1.在上一文章基础上修改,文章网址
ESP32-Ethernet-04-CSDN博客
2.基本代码
/* Ethernet Basic Example
This example code is in the Public Domain (or CC0 licensed, at your option.)
Unless required by applicable law or agreed to in writing, this
software is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
CONDITIONS OF ANY KIND, either express or implied.
*/
#include <stdio.h>
#include <string.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_netif.h"
#include "esp_eth.h"
#include "esp_event.h"
#include "esp_log.h"
#include "ethernet_init.h"
#include "sdkconfig.h"
#include "nvs_flash.h"
#include "lwip/err.h"
#include "lwip/sockets.h"
#include "lwip/sys.h"
#include <lwip/netdb.h>
static const char *TAG = "eth_example";
/** Event handler for Ethernet events */
static void eth_event_handler(void *arg, esp_event_base_t event_base,
int32_t event_id, void *event_data)
{
uint8_t mac_addr[6] = {0};
/* we can get the ethernet driver handle from event data */
esp_eth_handle_t eth_handle = *(esp_eth_handle_t *)event_data;
esp_netif_t *eth_netif=(esp_netif_t *)arg;
switch (event_id) {
case ETHERNET_EVENT_CONNECTED:
esp_netif_dhcpc_stop(eth_netif);
esp_netif_ip_info_t eth;
esp_netif_set_ip4_addr(ð.ip,192, 168, 1, 22);
esp_netif_set_ip4_addr(ð.gw,192, 168, 1, 1);
esp_netif_set_ip4_addr(ð.netmask, 255, 255, 255, 0);
esp_netif_set_ip_info(eth_netif,ð);
esp_eth_ioctl(eth_handle, ETH_CMD_G_MAC_ADDR, mac_addr);
ESP_LOGI(TAG, "Ethernet Link Up");
ESP_LOGI(TAG, "Ethernet HW Addr %02x:%02x:%02x:%02x:%02x:%02x",
mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
break;
case ETHERNET_EVENT_DISCONNECTED:
ESP_LOGI(TAG, "Ethernet Link Down");
break;
case ETHERNET_EVENT_START:
ESP_LOGI(TAG, "Ethernet Started");
break;
case ETHERNET_EVENT_STOP:
ESP_LOGI(TAG, "Ethernet Stopped");
break;
default:
break;
}
}
//IP_EVENT_ETH_GOT_IP的事件处理程序
static void got_ip_event_handler(void *arg, esp_event_base_t event_base,
int32_t event_id, void *event_data)
{
ip_event_got_ip_t *event = (ip_event_got_ip_t *) event_data;
const esp_netif_ip_info_t *ip_info = &event->ip_info;
ESP_LOGI(TAG, "Ethernet Got IP Address");
ESP_LOGI(TAG, "~~~~~~~~~~~");
ESP_LOGI(TAG, "ETHIP:" IPSTR, IP2STR(&ip_info->ip));
ESP_LOGI(TAG, "ETHMASK:" IPSTR, IP2STR(&ip_info->netmask));
ESP_LOGI(TAG, "ETHGW:" IPSTR, IP2STR(&ip_info->gw));
ESP_LOGI(TAG, "~~~~~~~~~~~");
}
//UDP 接受任务函数
static void udp_recv_task(void* arg)
{
char rx_buffer[128];
int sock = *(int *)arg;
struct sockaddr_in source_addr; // Large enough for both IPv4 or IPv6
socklen_t socklen = sizeof(source_addr);
for (;;)
{
//清空缓存
memset(rx_buffer, 0, sizeof(rx_buffer));
//接受数据
int len = recvfrom(sock, rx_buffer, sizeof(rx_buffer), 0, (struct sockaddr *)&source_addr, &socklen);
//发送数据
int err = sendto(sock, rx_buffer, len, 0, (struct sockaddr *)&source_addr, sizeof(source_addr));
if(err < 0)
{
printf("Error occurred during sending: errno %d", errno);
}
}
}
void app_main(void)
{
esp_err_t ret = nvs_flash_init();
if (ret == ESP_ERR_NVS_NO_FREE_PAGES || ret == ESP_ERR_NVS_NEW_VERSION_FOUND) {
ESP_ERROR_CHECK(nvs_flash_erase());
ret = nvs_flash_init();
}
//初始化TCP/IP网络接口
esp_netif_init();
//创建一个在后台运行的默认事件循环
esp_event_loop_create_default();
//初始化MAC和PHY默认配置
eth_mac_config_t mac_config = ETH_MAC_DEFAULT_CONFIG();
eth_phy_config_t phy_config = ETH_PHY_DEFAULT_CONFIG();
//PHY地址和复位引脚配置
phy_config.phy_addr = CONFIG_EXAMPLE_ETH_PHY_ADDR;
phy_config.reset_gpio_num = CONFIG_EXAMPLE_ETH_PHY_RST_GPIO;
//初始化EMAC默认配置
eth_esp32_emac_config_t esp32_emac_config = ETH_ESP32_EMAC_DEFAULT_CONFIG();
//MDC的信号引脚
esp32_emac_config.smi_mdc_gpio_num = CONFIG_EXAMPLE_ETH_MDC_GPIO;
//MDIO的信号引脚
esp32_emac_config.smi_mdio_gpio_num = CONFIG_EXAMPLE_ETH_MDIO_GPIO;
//创建MAC实例
esp_eth_mac_t *mac = esp_eth_mac_new_esp32(&esp32_emac_config, &mac_config);
//创建PHY实例
esp_eth_phy_t *phy = esp_eth_phy_new_lan87xx(&phy_config);
//驱动程序安装完毕后,将得到驱动程序的句柄
esp_eth_handle_t eth_handle = NULL;
//应用默认驱动程序配置
esp_eth_config_t config = ETH_DEFAULT_CONFIG(mac, phy);
//安装驱动程序
esp_eth_driver_install(&config, ð_handle);
//应用以太网默认的网络接口配置
esp_netif_config_t cfg = ESP_NETIF_DEFAULT_ETH();
//为以太网驱动程序创建网络接口
esp_netif_t *eth_netif = esp_netif_new(&cfg);
//将以太网驱动程序连接至TCP/IP协议栈
esp_netif_attach(eth_netif, esp_eth_new_netif_glue(eth_handle));
//注册以太网事件处理程序
esp_event_handler_register(ETH_EVENT, ESP_EVENT_ANY_ID, ð_event_handler, eth_netif);
//注册用户定义的IP事件处理程序
esp_event_handler_register(IP_EVENT, IP_EVENT_ETH_GOT_IP, &got_ip_event_handler, eth_netif);
//启动以太网驱动程序状态机
esp_eth_start(eth_handle);
//新建socket
int sock = socket(AF_INET, SOCK_DGRAM, IPPROTO_IP);
//绑定本机地址的IP和端口
struct sockaddr_in dest_addr;
dest_addr.sin_family = AF_INET;
dest_addr.sin_addr.s_addr = htonl(INADDR_ANY);
dest_addr.sin_port = htons(10000);
//绑定本机IP
int err = bind(sock, (struct sockaddr *)&dest_addr, sizeof(dest_addr));
if(err < 0)
{
printf("Socket unable to bind: errno %d", errno);
close(sock);
}
//创建任务
xTaskCreate(udp_recv_task, "udp_recv_task", 2048, &sock, 10, NULL);
}
3.运行和测试结果
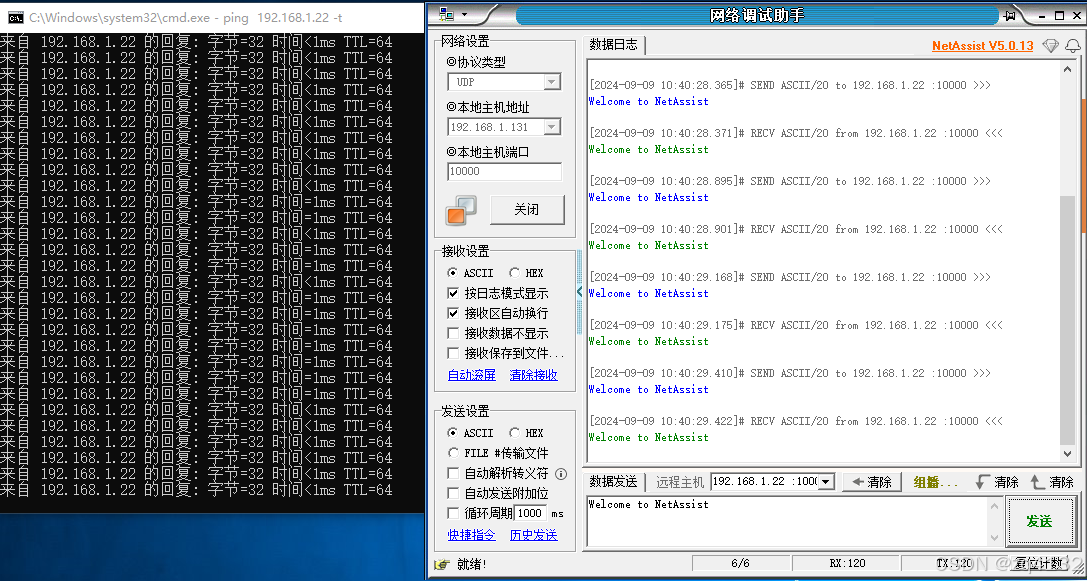