介绍:
本文以图书为例来实现一个顺序表以及顺序表的一些基本操作
一、存储结构
#define MAXSIZE 100 //图书可能达到的最大长度
//定义图书数据的存储结构,此为BOOK类型
typedef struct{//图书的信息
char no[20];//图书ISBN
char name[50];//书名
float price;//价格
}Book;
typedef Book ElemType;//定义顺序表中的元素类型
//定义顺序表的存储结构
typedef struct {
ElemType *elem;//存储空间的基地址
int Length;//记录图书当前的数量
}SqList;
二、初始化一个空的顺序表
/*
* @brief 顺序表初始化
* @param None
* @retval 1或0;
*/
Status SqList_Init(SqList *L)
{
L->elem = new ElemType[MAXSIZE];//为顺序表分配一个大小为MAXSIZE的数组空间。
if(!L->elem)return 0;//存储分配失败则返回0
L->Length = 0;//初始化图书数量为0。
return OK;
}
三、添加图书操作(添加顺序表元素)
/*
* @brief 添加图书
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的ISBN,例如: (char*)"1234"
* @param 书名,例如: (char*)"book"
* @param 价格,例如: 9.9
* @retval 1或0;
*/
void AddBook(SqList *L,char *no,char *name,float price)
{
int i;
for(i=0;i<strlen(no);i++)
{
L->elem[L->Length].no[i]=no[i];
}
L->elem[L->Length].no[i]='\0';
for(i=0;i<strlen(name);i++)
{
L->elem[L->Length].name[i]=name[i];
}
L->elem[L->Length].name[i]='\0';
L->elem[L->Length].price=price;
L->Length++;
}
四、顺序表的取值
/*
* @brief 获取图书信息图书信息
* @param 此处传入顺序表的地址,例如: &L
* @param 第i本图书,例如: 1
* @param 用于存储获取的图书信息,例如: &e
* @retval 1或0;
*/
Status GetElem(SqList L,int i, ElemType *e)
{
if(i<1 || i>L.Length)return 0;
*e=L.elem[i-1];
return OK;
}
五、顺序表的查找
/*
* @brief 查找指定图书
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的ISBN
* @param 书名
* @param 价格
* @retval 0(未找到)或大于0的数(图书的位置)
*/
Status LcateElem(SqList L,char *no,char *name,float price)
{
for(int i=0;i<L.Length;i++)
{
if( (strcmp(L.elem[i].name,name) && strcmp(L.elem[i].no,no) && !(L.elem[i].price == price)) ==0 )
return (i+1);
}
return 0;
}
六、打印顺序表中的元素
/*
* @brief 打印图书信息
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的位置(1,2,3......)
* @retval None
*/
void Print_BookIfo(SqList L,int location)
{
if(location<1 || location>L.Length)
{
printf("The location is beyond the defined range!");
}
else
{
printf("book%d:\n",location);
printf("no:%s\n",L.elem[location-1].no);
printf("name:%s\n",L.elem[location-1].name);
printf("price:%2.1f\n\n",L.elem[location-1].price);
}
}
七、删除图书
/*
* @brief 删除指定图书
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的位置(1,2,3......)
* @retval 0(Location的值不正确),1(删除成功)
*/
Status ListDelete(SqList *L,int Location)
{
if((Location<1) || (Location>L->Length))return 0;//判断Location的值是否合理
for(int i=Location;i<L->Length-1;i++)
{
L->elem[i-1]=L->elem[i];
}
L->Length--;
return OK;
}
八、插入
/*
* @brief 插入指定图书
* @param 此处传入顺序表的地址,例如: &L
* @param 要插入的图书位置(1,2,3......)
* @param 被插入的元素
* @retval 0(Location的值不正确),1(删除成功)
*/
Status ListInsert(SqList *L,int Location,ElemType e)
{
int i=0;
if((Location<1) || (Location>L->Length+1))return 0;
if(L->Length==MAXSIZE)return 0;
for(i=L->Length-1;i>=Location-1;i--)
{
L->elem[i+1] = L->elem[i];
}
L->elem[i+1] = e;
L->Length++;
return OK;
}
九、以上是顺序表的一些基本操作,还有其它的一些操作在这里未实现,会在以后继续完成
以下是全部代码,包括测试代码
#include <stdio.h>
#include <string.h>
typedef unsigned int Status;
#define MAXSIZE 100 //图书可能达到的最大长度
#define OK 1
//定义图书数据的存储结构,此为BOOK类型
typedef struct{//图书的信息
char no[20];//图书ISBN
char name[50];//书名
float price;//价格
}Book;
typedef Book ElemType;//定义顺序表中的元素类型
//定义顺序表的存储结构
typedef struct {
ElemType *elem;//存储空间的基地址
int Length;//记录图书当前的数量
}SqList;
/*
* @brief 顺序表初始化
* @param None
* @retval 1或0;
*/
Status SqList_Init(SqList *L)
{
L->elem = new ElemType[MAXSIZE];//为顺序表分配一个大小为MAXSIZE的数组空间。
if(!L->elem)return 0;//存储分配失败则返回0
L->Length = 0;//初始化图书数量为0。
return OK;
}
/*
* @brief 添加图书
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的ISBN,例如: (char*)"1234"
* @param 书名,例如: (char*)"book"
* @param 价格,例如: 9.9
* @retval 1或0;
*/
void AddBook(SqList *L,char *no,char *name,float price)
{
int i;
for(i=0;i<strlen(no);i++)
{
L->elem[L->Length].no[i]=no[i];
}
L->elem[L->Length].no[i]='\0';
for(i=0;i<strlen(name);i++)
{
L->elem[L->Length].name[i]=name[i];
}
L->elem[L->Length].name[i]='\0';
L->elem[L->Length].price=price;
L->Length++;
}
/*
* @brief 获取图书信息图书信息
* @param 此处传入顺序表的地址,例如: &L
* @param 第i本图书,例如: 1
* @param 用于存储获取的图书信息,例如: &e
* @retval 1或0;
*/
Status GetElem(SqList L,int i, ElemType *e)
{
if(i<1 || i>L.Length)return 0;
*e=L.elem[i-1];
return OK;
}
/*
* @brief 查找指定图书
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的ISBN
* @param 书名
* @param 价格
* @retval 0(未找到)或大于0的数(图书的位置)
*/
Status LcateElem(SqList L,char *no,char *name,float price)
{
for(int i=0;i<L.Length;i++)
{
if( (strcmp(L.elem[i].name,name) && strcmp(L.elem[i].no,no) && !(L.elem[i].price == price)) ==0 )
return (i+1);
}
return 0;
}
/*
* @brief 打印图书信息
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的位置(1,2,3......)
* @retval None
*/
void Print_BookIfo(SqList L,int location)
{
if(location<1 || location>L.Length)
{
printf("The location is beyond the defined range!");
}
else
{
printf("book%d:\n",location);
printf("no:%s\n",L.elem[location-1].no);
printf("name:%s\n",L.elem[location-1].name);
printf("price:%2.1f\n\n",L.elem[location-1].price);
}
}
/*
* @brief 删除指定图书
* @param 此处传入顺序表的地址,例如: &L
* @param 图书的位置(1,2,3......)
* @retval 0(Location的值不正确),1(删除成功)
*/
Status ListDelete(SqList *L,int Location)
{
if((Location<1) || (Location>L->Length))return 0;//判断Location的值是否合理
for(int i=Location;i<=L->Length-1;i++)
{
L->elem[i-1]=L->elem[i];
}
L->Length--;
return OK;
}
/*
* @brief 插入指定图书
* @param 此处传入顺序表的地址,例如: &L
* @param 要插入的图书位置(1,2,3......)
* @param 被插入的元素
* @retval 0(Location的值不正确),1(删除成功)
*/
Status ListInsert(SqList *L,int Location,ElemType e)
{
int i=0;
if((Location<1) || (Location>L->Length+1))return 0;
if(L->Length==MAXSIZE)return 0;
for(i=L->Length-1;i>=Location-1;i--)
{
L->elem[i+1] = L->elem[i];
}
L->elem[i+1] = e;
L->Length++;
return OK;
}
int main(void)
{
SqList L;
ElemType e1,e2,e3,e4,e5;
char no[20]="2024";
char name[50]="abcd5";
float price=3.3;
int Location=0;
if(SqList_Init(&L) == 0)SqList_Init(&L);
printf("添加图书\n");
AddBook(&L,(char*)"1234",(char*)"CPP",9.9);
AddBook(&L,(char*)"6666",(char*)"JAVA",8.9);
AddBook(&L,(char*)"999",(char*)"Python",7.9);
AddBook(&L,(char*)"1433",(char*)"vocal",6.6);
printf("Number of book:%d\n ",L.Length);
//初始化Book类型的变量e5
for(int i=0;i<strlen(no)+1;i++)
{
e5.no[i]=no[i];
}
for(int i=0;i<strlen(name)+1;i++)
{
e5.name[i]=name[i];
}
e5.price=price;
//将e5插入顺序表中的第2个位置
printf("开始插入:\n");
if(ListInsert(&L,2,e5)==OK)
{
//插入成功则打印这条语句,并显示插入后的元素数量 5
printf("Successfully Insert.\nnumber of book is:%d\n ",L.Length);
}
printf("******************************获取图书信息*******************************************\n");
if(GetElem(L,1,&e1) == OK)
{
printf("book1:\n");
printf("no:%s\n",e1.no);
printf("name:%s\n",e1.name);
printf("price:%2.1f\n\n",e1.price);
}
if(GetElem(L,2,&e2) == OK)
{
printf("book2:\n");
printf("no:%s\n",e2.no);
printf("name:%s\n",e2.name);
printf("price:%2.1f\n\n",e2.price);
}
if(GetElem(L,3,&e3) == OK)
{
printf("book3:\n");
printf("no:%s\n",e3.no);
printf("name:%s\n",e3.name);
printf("price:%2.1f\n\n",e3.price);
}
if(GetElem(L,4,&e4) == OK)
{
printf("book4:\n");
printf("no:%s\n",e4.no);
printf("name:%s\n",e4.name);
printf("price:%2.1f\n\n",e4.price);
}
if(GetElem(L,5,&e5) == OK)
{
printf("book5:\n");
printf("no:%s\n",e5.no);
printf("name:%s\n",e5.name);
printf("price:%2.1f\n\n",e5.price);
}
printf("*******************************查找图书位置*******************************************\n");
printf("CPP:%d\n",LcateElem(L,(char*)"1234",(char*)"CPP",9.9));
printf("JAVA:%d\n",LcateElem(L,(char*)"6666",(char*)"JAVA",8.9));
printf("Python:%d\n",LcateElem(L,(char*)"999",(char*)"Python",7.9));
printf("vocal:%d\n",LcateElem(L,(char*)"1433",(char*)"vocal",6.6));
printf("abcd5:%d\n",LcateElem(L,(char*)"2024",(char*)"abcd5",3.3));
printf("*******************************通过图书位置获取图书信息*******************************************\n");
Print_BookIfo(L,LcateElem(L,(char*)"6666",(char*)"JAVA",8.9));
Print_BookIfo(L,LcateElem(L,(char*)"1234",(char*)"CPP",9.9));
Print_BookIfo(L,LcateElem(L,(char*)"999",(char*)"Python",7.9));
Print_BookIfo(L,LcateElem(L,(char*)"1433",(char*)"vocal",6.6));
Print_BookIfo(L,LcateElem(L,(char*)"2024",(char*)"abcd5",3.3));
printf("*******************************删除第4本书后查找*******************************************\n");
if(ListDelete(&L,4)==OK)
{
printf("Successfully Delete !\n");
}
printf("Number of book:%d\n ",L.Length);
if(LcateElem(L,(char*)"999",(char*)"Python",7.9) == 0)
{
printf("NO books found!\n");
}
else
{
printf("book is found!\n");
}
Print_BookIfo(L,LcateElem(L,(char*)"999",(char*)"Python",7.9));
return 0;
}
运行情况如下
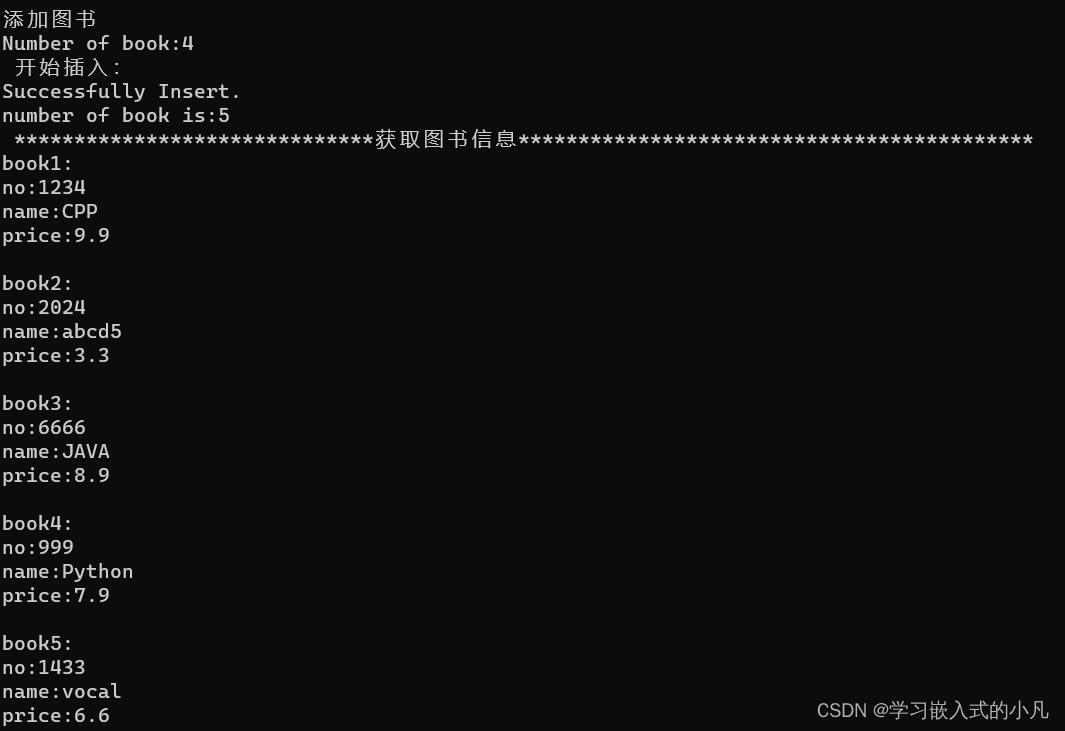
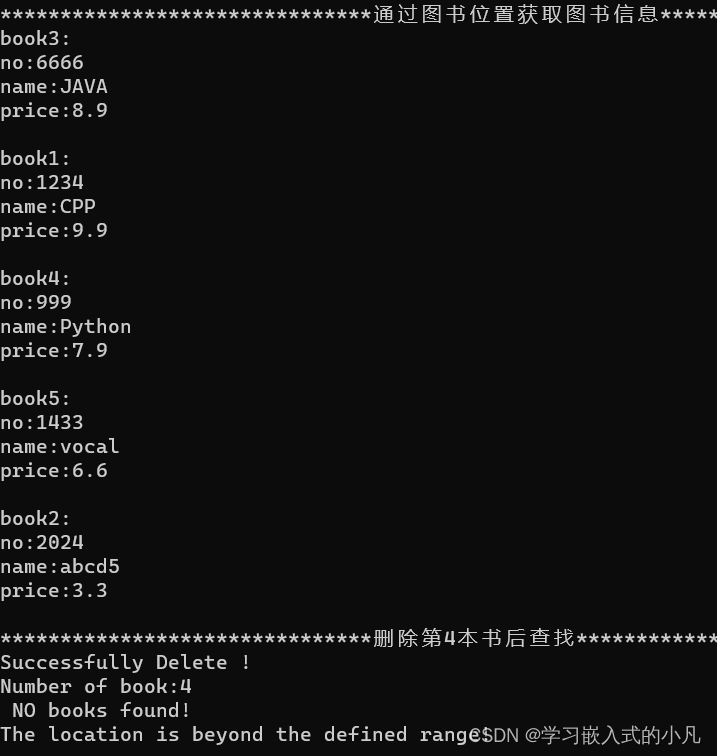