402. Remove K Digits
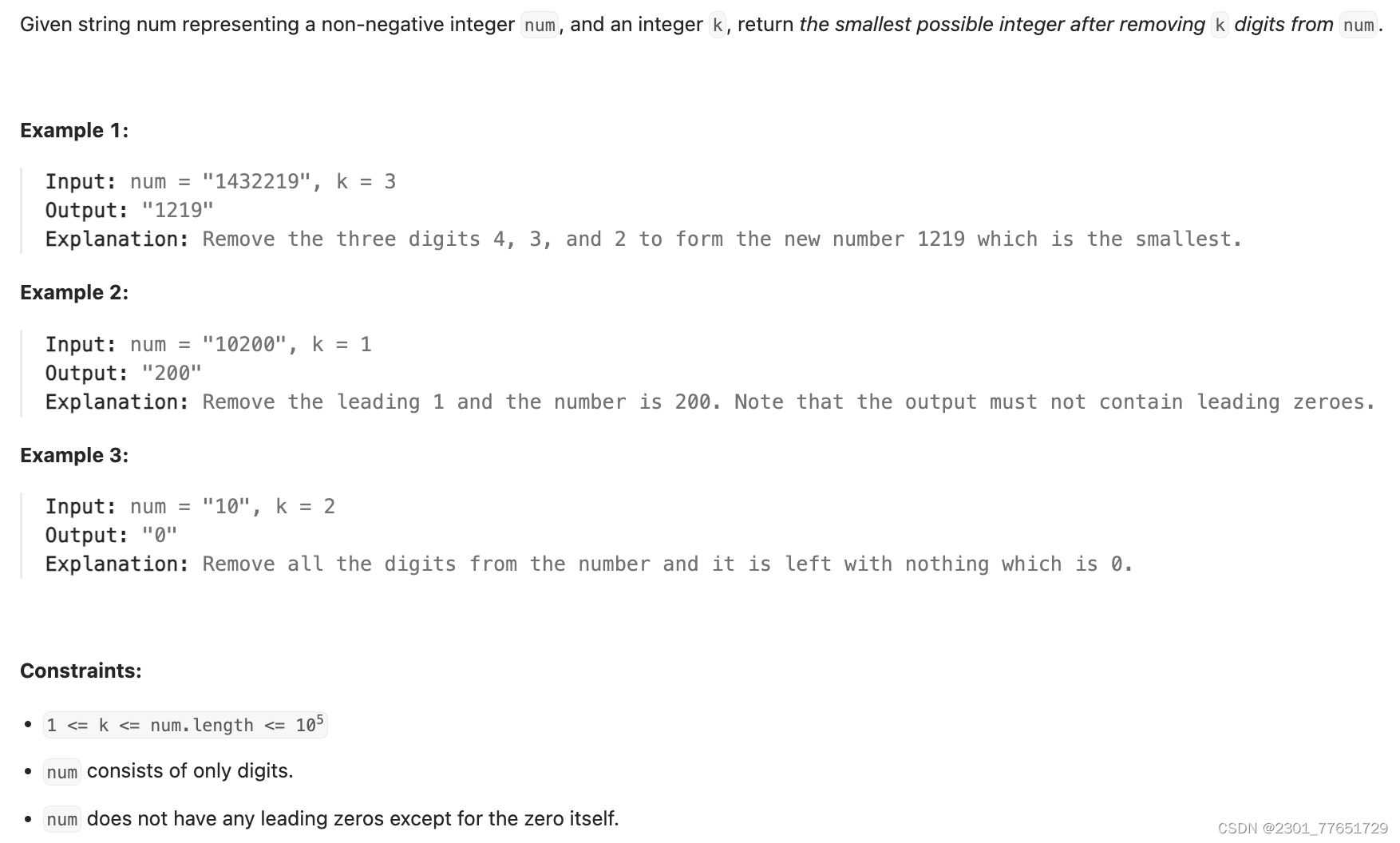
class Solution {
public String removeKdigits(String num, int k) {
if(k >= num.length()) {
return "0";
}
Stack<Character> stack = new Stack();
// 单调栈 保持整个num是升序
for(char digit : num.toCharArray()) {
while(k > 0 && !stack.isEmpty() && stack.peek() > digit) {
stack.pop();
k --;
}
stack.push(digit);
}
// 如果整个升序完成,升序的过程是删除了一些digit的,k还没有减完变成0,
// 那么移除掉末尾的digit,因为这个时候num是升序的,越末尾数字越大
while(k > 0) {
stack.pop();
k --;
}
StringBuilder str = new StringBuilder();
while(!stack.isEmpty()) {
str.insert(0, stack.pop());
// str.append(stack.pop());
}
// 去掉leading zero,
int index = 0;
while( index < str.length() && str.charAt(index) == '0') {
index++;
}
str.delete(0, index); // delete method do not include index
return str.length() == 0 ? "0" : str.toString();
// str.reverse().toString();
}
}
682. Baseball Game
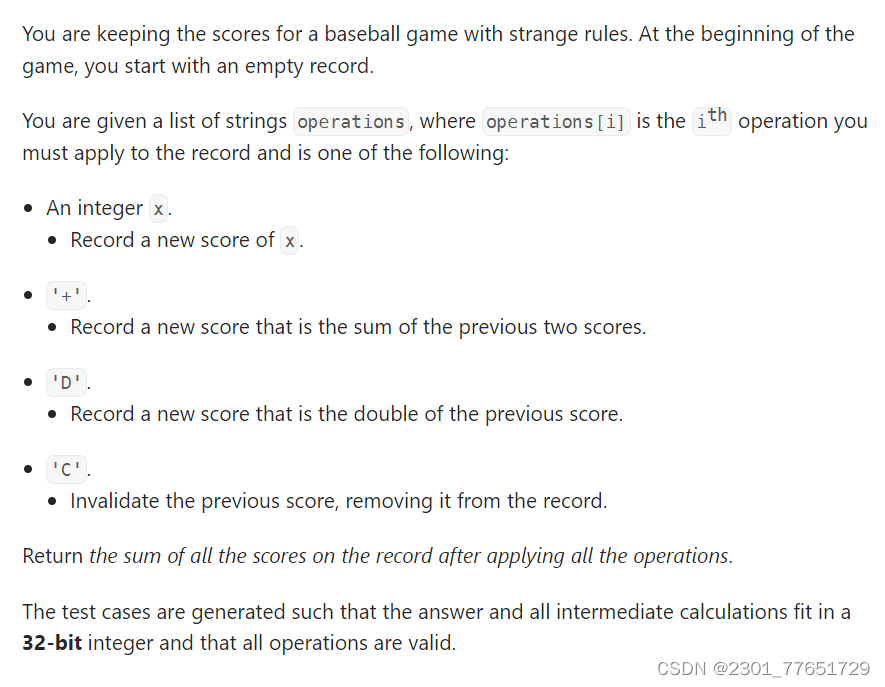
class Solution {
public int calPoints(String[] op) {
List<Integer> li = new ArrayList();
int res = 0;
for(int i = 0; i < op.length; i++) {
if(op[i].equals("+")) {
int sum = li.get(li.size()-1) + li.get(li.size()-2);
li.add(sum);
} else if(op[i].equals("D")) {
li.add(2 * li.get(li.size() - 1));
} else if(op[i].equals("C")) {
li.remove(li.size() - 1);
} else {
li.add(Integer.parseInt(op[i]));
}
}
for(int i = 0; i < li.size(); i++) {
res += li.get(i);
}
return res;
}
}
class Solution {
public int calPoints(String[] operations) {
Stack<Integer> stack = new Stack<>();
for (String s : operations) {
if (s.equals("+")) {
int a = stack.pop();
int newScore = a + stack.peek();
stack.push(a);
stack.push(newScore);
}
else if (s.equals("D")) {
stack.push(2 * stack.peek());
}
else if (s.equals("C")) {
stack.pop();
}
else stack.push(Integer.parseInt(s));
}
int totalScore = 0;
while (!stack.isEmpty()) totalScore += stack.pop();
return totalScore;
}
}
20. Valid Parentheses
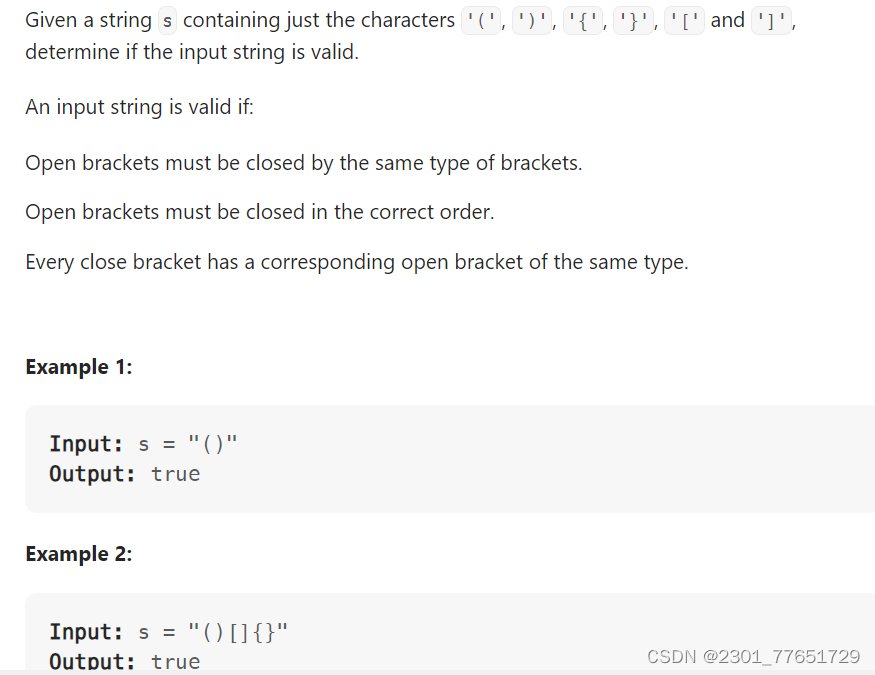
class Solution {
public boolean isValid(String s) {
Stack<Character> mat = new Stack<>();
char[] ss = s.toCharArray();
for(int i = 0; i < ss.length; i++) {
char a = ss[i];
if(a == '(' || a == '[' || a == '{') {
mat.push(a);
} else if(mat.empty()) {
// no matched brackets
return false;
} else if(a == ')' && mat.pop() != '(') {
return false;
} else if(a == ']' && mat.pop() != '[') {
return false;
} else if(a == '}' && mat.pop() != '{') {
return false;
}
}
return mat.empty();
}
}
2048. Next Greater Numerically Balanced Number
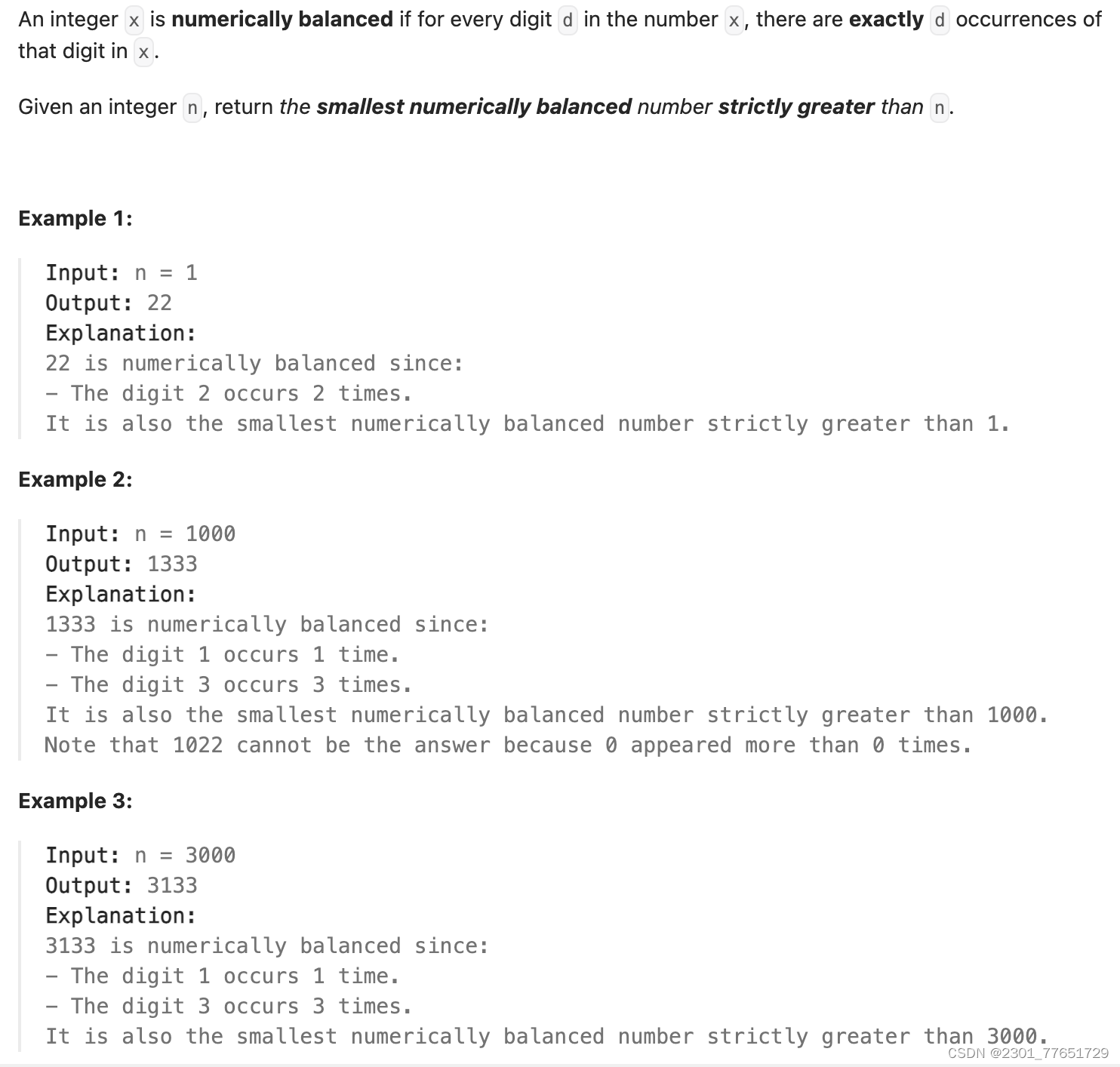
class Solution {
private boolean isBN(int num) {
int[] occurence = new int[10];
while(num > 0) {
int digit = num % 10;
occurence[digit]++;
num /= 10;
}
for(int i = 0; i < 10; i++) {
if(occurence[i] != 0 && occurence[i] != i) {
return false;
}
}
return true;
}
public int nextBeautifulNumber(int n) {
// ➕1操作,加1后check是否符合,符合返回 不符合再加1
// 使用数组或者map来存储出现次数,方便和digit的值是否相等
int num = n + 1;
while(! isBN(num)) {
num++;
}
return num;
}
}
2454. Next Greater Element IV
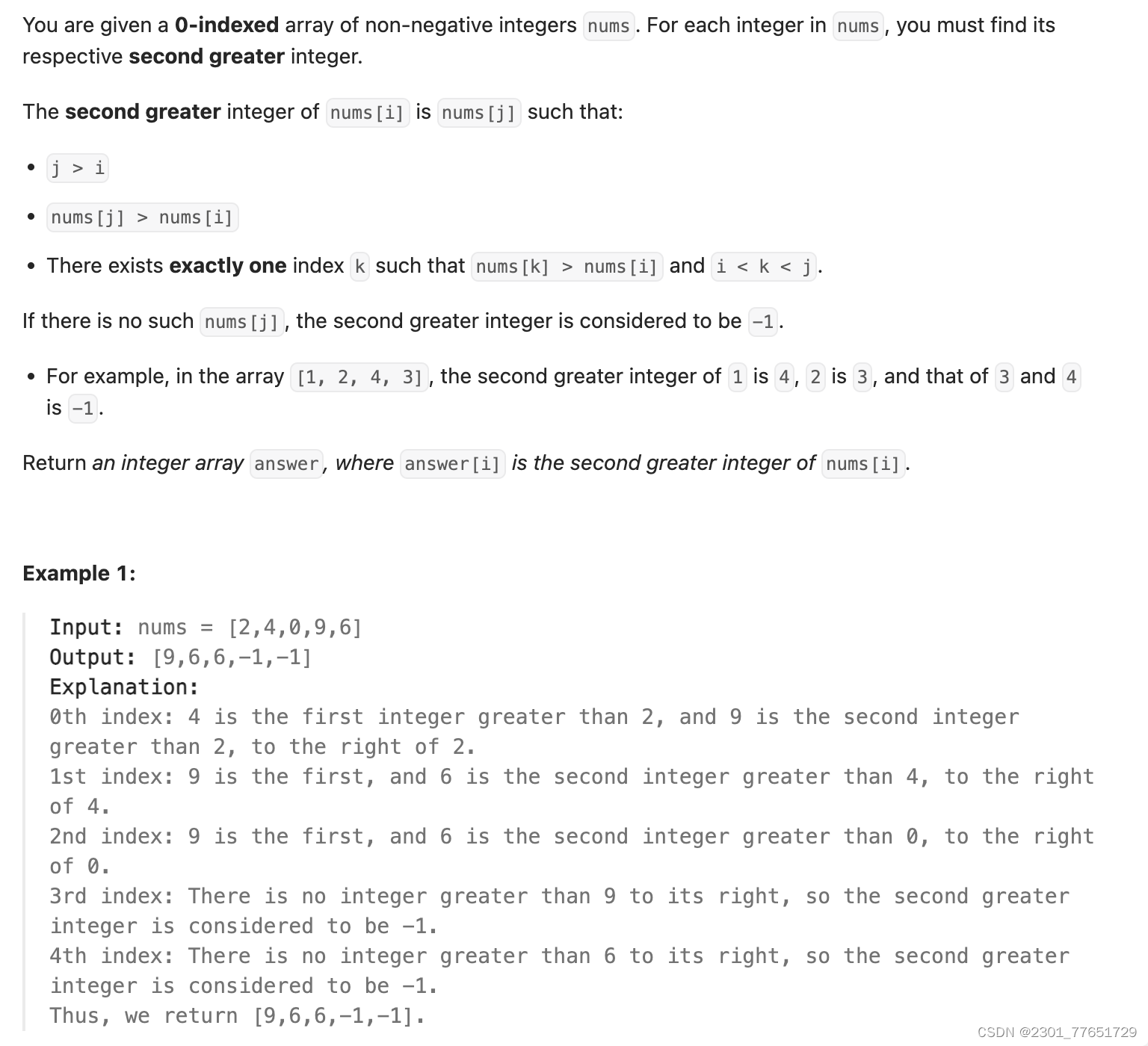
class Solution {
public int[] secondGreaterElement(int[] nums) {
int len = nums.length, res[] = new int[len];
Arrays.fill(res, -1);
Stack<Integer> s1 = new Stack(), s2 = new Stack(), temp = new Stack();
for(int i = 0; i < len; i++) {
while(!s2.isEmpty() && nums[s2.peek()] < nums[i]) {
res[s2.pop()] = nums[i];
}
while(!s1.isEmpty() && nums[s1.peek()] < nums[i]) {
temp.push(s1.pop()); // 使用缓存栈平移到声s2中,不然直接移动顺序会有问题
}
while(!temp.isEmpty()) {
s2.push(temp.pop());
}
s1.push(i);
}
return res;
}
}
556. Next Greater Element III
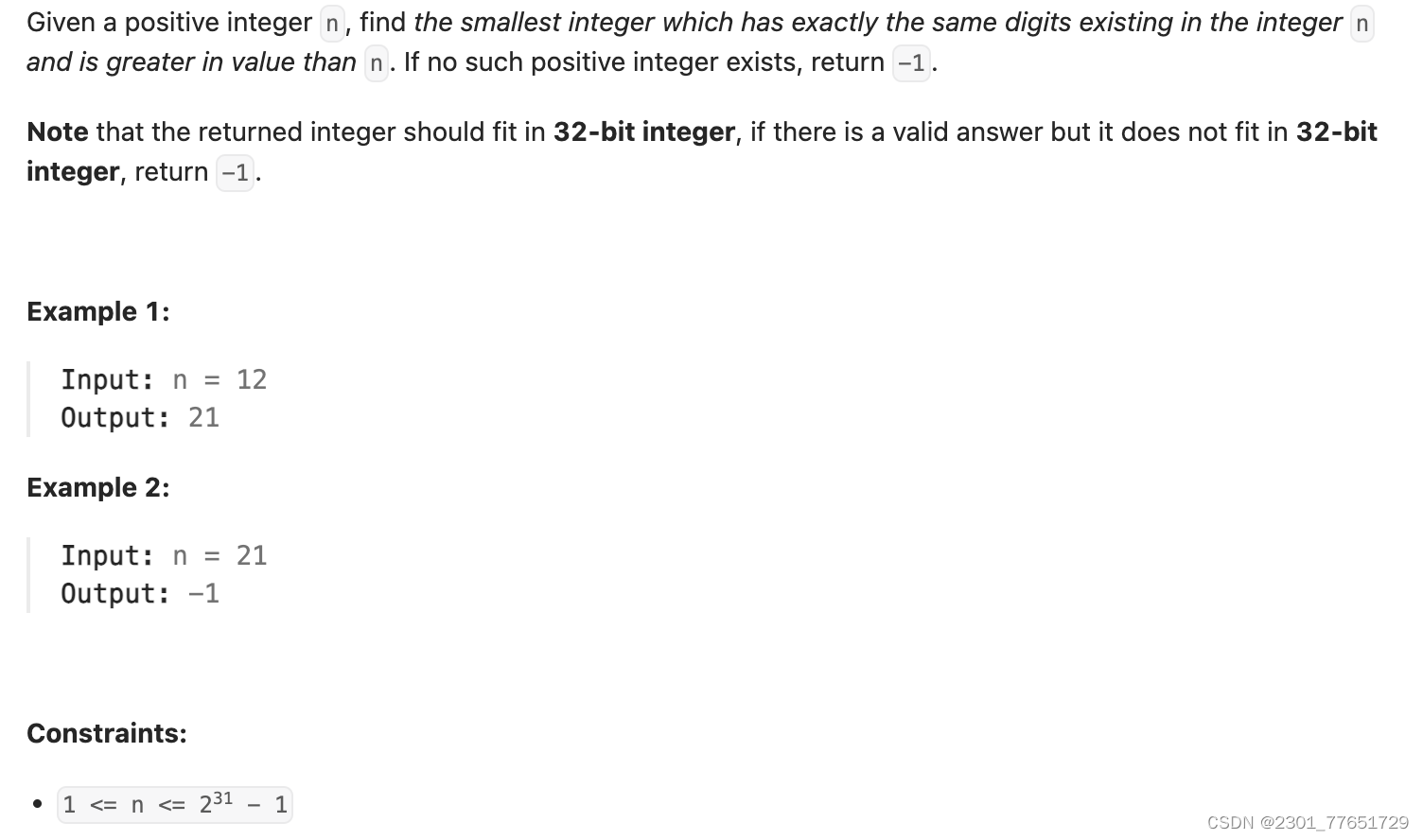
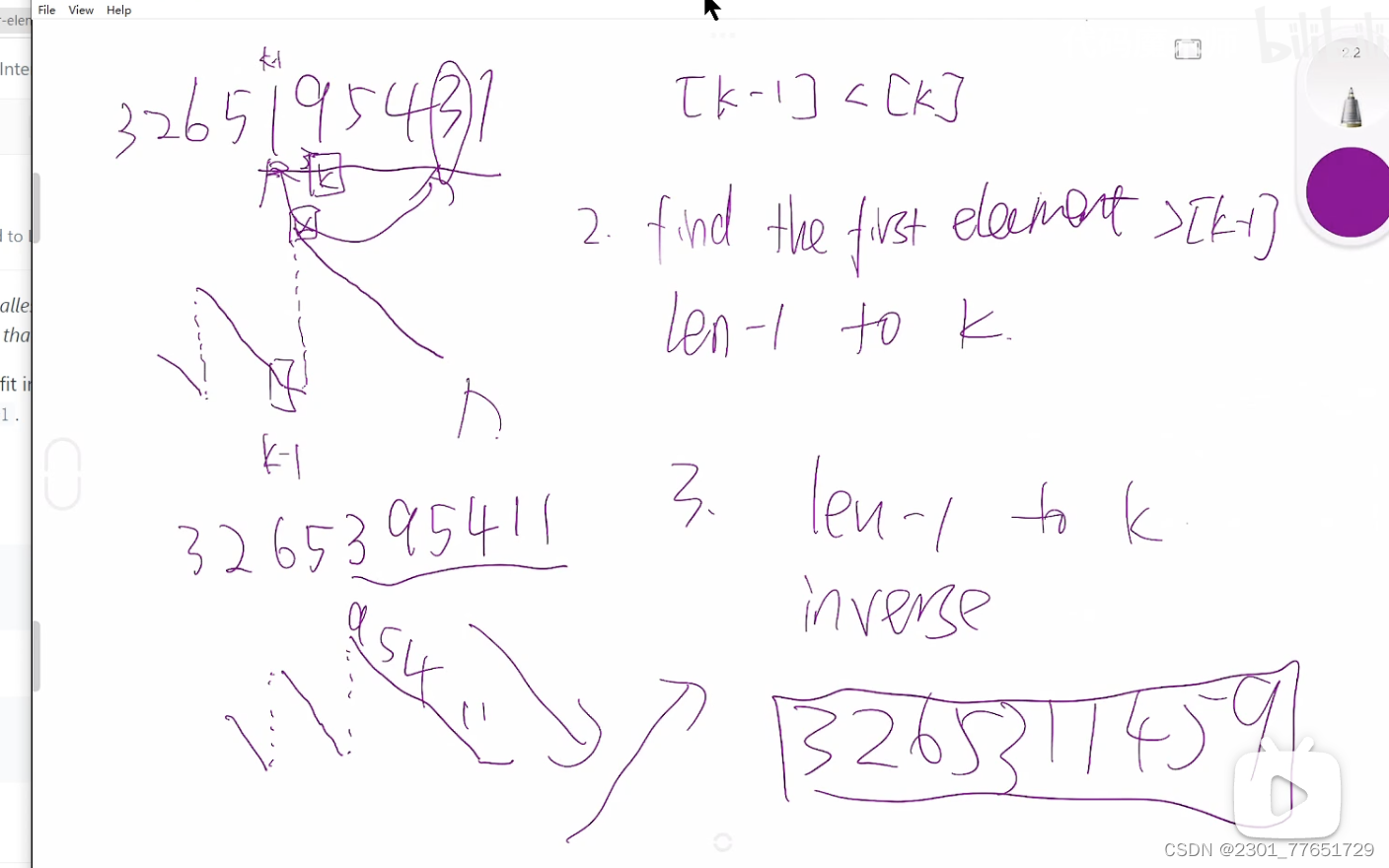
class Solution {
public int nextGreaterElement(int n) {
// find last position [k - 1] < [k]
// find first element > [k - 1] and exchange their position (from len - 1 to k)
// reverse the elements from k to len - 1
//将int转换成 string 在转换成char数组
char[] ch = String.valueOf(n).toCharArray();
int len = ch.length;
int i = len - 2;
// 找到第一个最后一个转折点开始降序19这里 3265195431
while(i >= 0 && ch[i + 1] <= ch[i]) { // 等于必须有
i--;
}
// 54321这样的特列没有nge
if(i < 0) {
return -1;
}
// 从3265195431后面开始找到第一个大于1的数
int j = len - 1;
while(j >= 0 && ch[j] <= ch[i]) {
j--;
}
swap(ch, i, j);
reverse(ch, i + 1);
// 将最终ch数组转化成int 但有可能超出整数最大范围,所以改成long类型
long res = Long.parseLong(new String(ch));
return (res <= Integer.MAX_VALUE ? (int)res : -1);
}
private void reverse(char[] ch, int i) {
int start = i;
int end = ch.length - 1;
while(start < end) {
swap(ch, start++, end--);
}
}
private void swap(char[] ch, int i, int j) {
char temp = ch[i];
ch[i] = ch[j];
ch[j] = temp;
}
}
503. Next Greater Element II
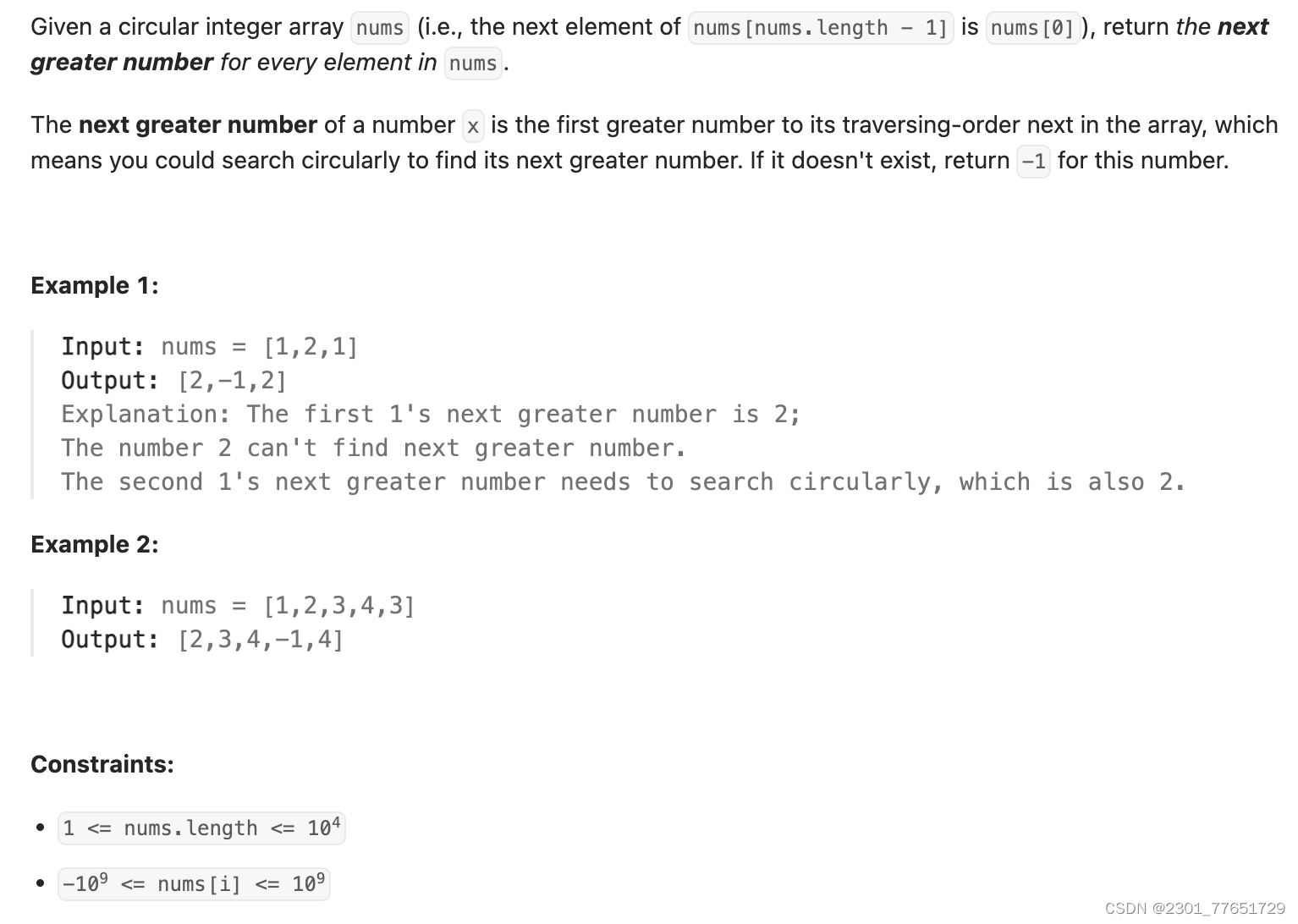
class Solution {
public int[] nextGreaterElements(int[] nums) {
// 是一个circle 1 2 1 相当于在后面加了一遍 1 2 1 1 2 1
int len = nums.length;
int res[] = new int[len];
Arrays.fill(res, -1);
Stack<Integer> stack = new Stack();
for(int i = 0; i < 2 * len; i++) {
int num = nums[i % len]; // 技巧: 计算后面的值
while(!stack.isEmpty() && nums[stack.peek()] < num) { //注意写num不是 nums[i]
res[stack.pop()] = num; // 这里也注意是stack.pop(),不是stack.peep(),不然会timeout,还有num,不然会下标越界
}
if(i < len) {
stack.push(i);
}
}
return res;
}
}
496. Next Greater Element I
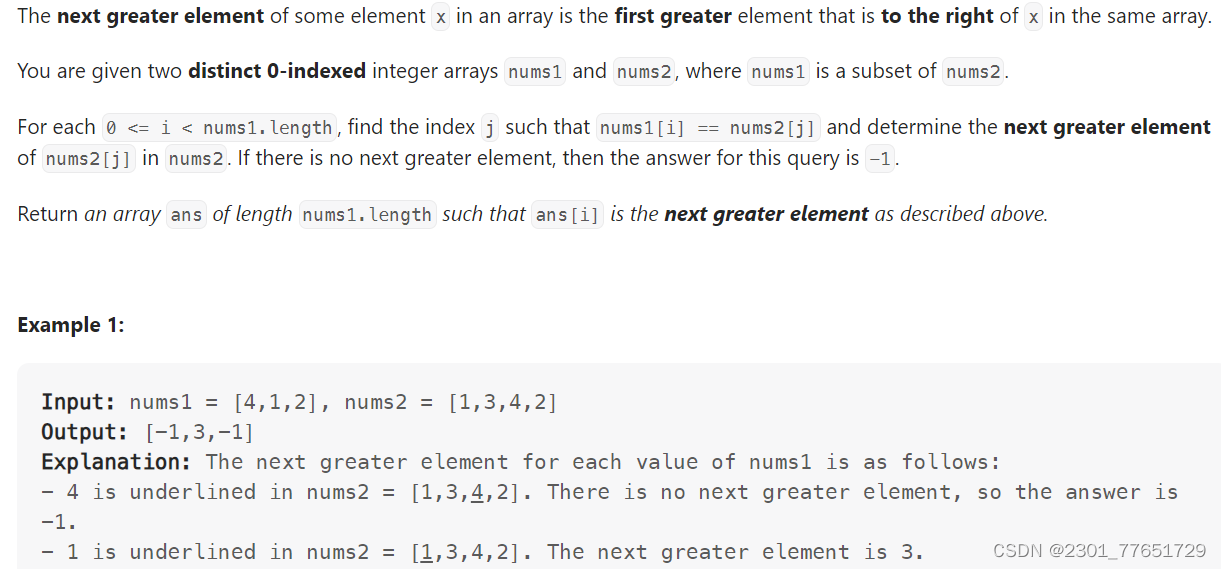
class Solution {
public int[] nextGreaterElement(int[] nums1, int[] nums2) {
Deque<Integer> stack = new ArrayDeque();
Map<Integer, Integer> map = new HashMap();
int[] res = new int[nums1.length];
for(int num : nums2) {
while(!stack.isEmpty() && stack.peek() < num) {
map.put(stack.pop(), num);
}
stack.push(num);
}
for(int i = 0; i < nums1.length; i++) {
res[i] = map.getOrDefault(nums1[i], -1);
}
return res;
}
}
345. Reverse Vowels of a String
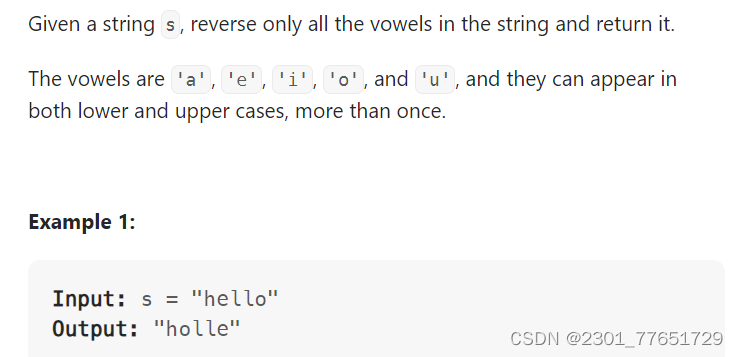
class Solution {
public String reverseVowels(String s) {
Deque<Character> stack = new ArrayDeque();
for(char c : s.toCharArray()) {
if(c == 'a' || c == 'e' || c == 'i'|| c == 'o' || c == 'u'|| c == 'A' || c == 'E'|| c == 'I' || c == 'O'|| c == 'U') {
stack.addLast(c);
}
}
StringBuilder str = new StringBuilder();
for(char c : s.toCharArray()) {
if(c == 'a' || c == 'e' || c == 'i'|| c == 'o' || c == 'u'|| c == 'A' || c == 'E'|| c == 'I' || c == 'O'|| c == 'U') {
str.append(stack.pollLast());
} else {
str.append(c);
}
}
return str.toString();
}
}
32. Longest Valid Parentheses
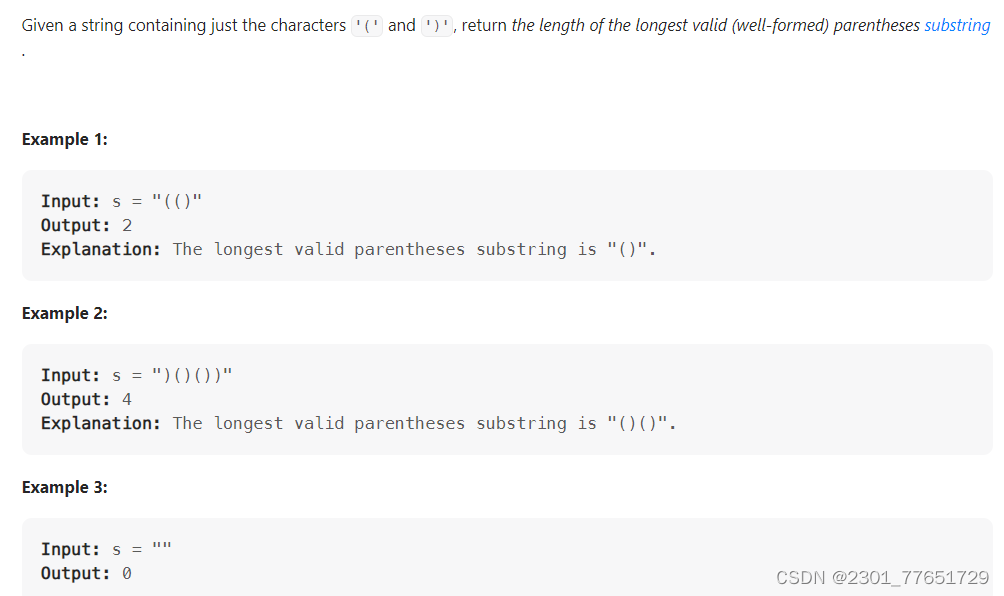
class Solution {
public int longestValidParentheses(String s) {
Stack<Integer> st = new Stack();
st.push(-1);
int n = 0;
for(int i = 0; i < s.length(); i++) {
if(s.charAt(i) == '(') {
st.push(i);
} else if(s.charAt(i) == ')') {
st.pop();
if(st.empty()) {
st.push(i);
} else {
n = Math.max(n, i - st.peek());
}
}
}
return n;
}
}
150. Evaluate Reverse Polish Notation
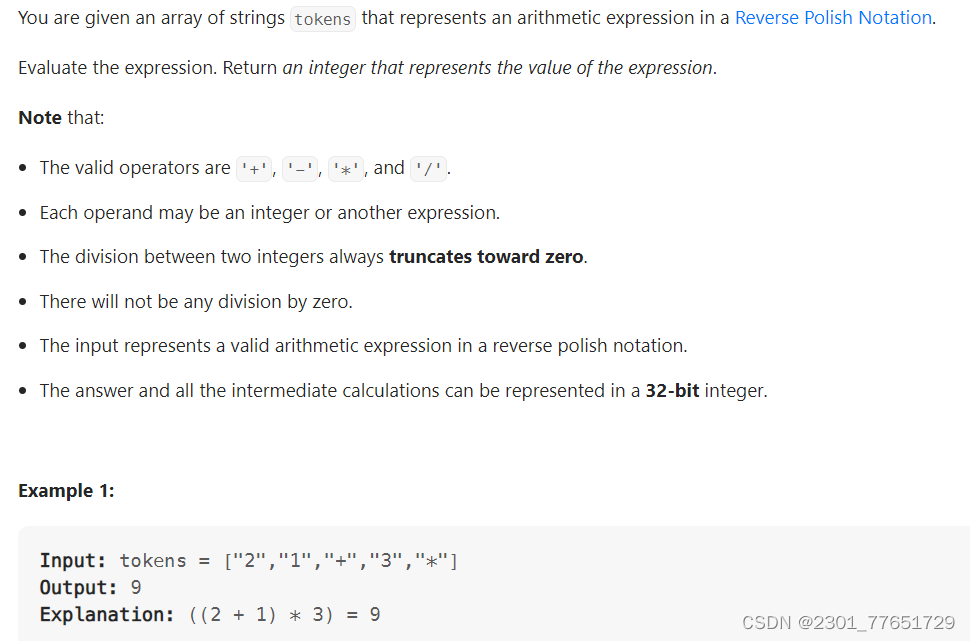
class Solution {
public int evalRPN(String[] tokens) {
Stack<Integer> st = new Stack();
for(String s : tokens) {
if(s.equals("+")) {
int x = st.pop();
int y = st.pop();
st.push(x + y);
} else if(s.equals("*")) {
int x = st.pop();
int y = st.pop();
st.push(y * x);
} else if(s.equals("-")) {
int x = st.pop();
int y = st.pop();
st.push(y - x);
} else if(s.equals("/")) {
int x = st.pop();
int y = st.pop();
st.push(y / x);
} else {
st.push(Integer.parseInt(s));
}
}
return st.peek();
}
}
636. Exclusive Time of Functions
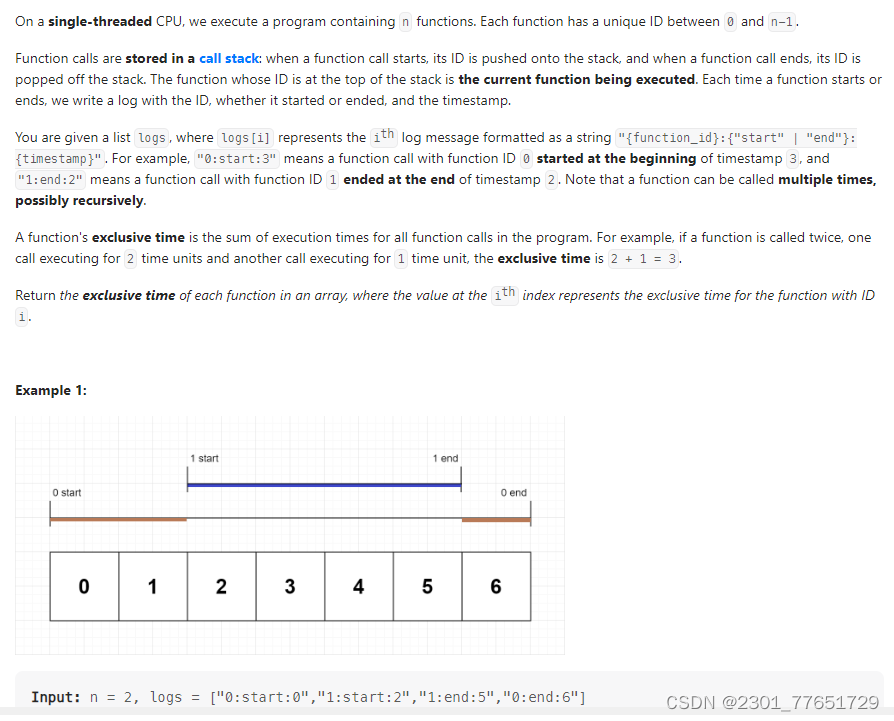
class Solution {
public int[] exclusiveTime(int n, List<String> logs) {
int[] res = new int[n];
if(n == 0 || logs == null || logs.size() == 0) {
return res;
}
Deque<Integer> stack = new ArrayDeque();
int preT = 0;
for(String log : logs) {
String[] logP = log.split(":");
int curT = Integer.parseInt(logP[2]);
if("start".equals(logP[1])) {
if(!stack.isEmpty()) {
res[stack.peek()] += curT - preT;
}
stack.push(Integer.parseInt(logP[0]));
preT = curT;
} else {
res[stack.pop()] += curT - preT + 1;
preT = curT + 1;
}
}
return res;
}
}
844. Backspace String Compare
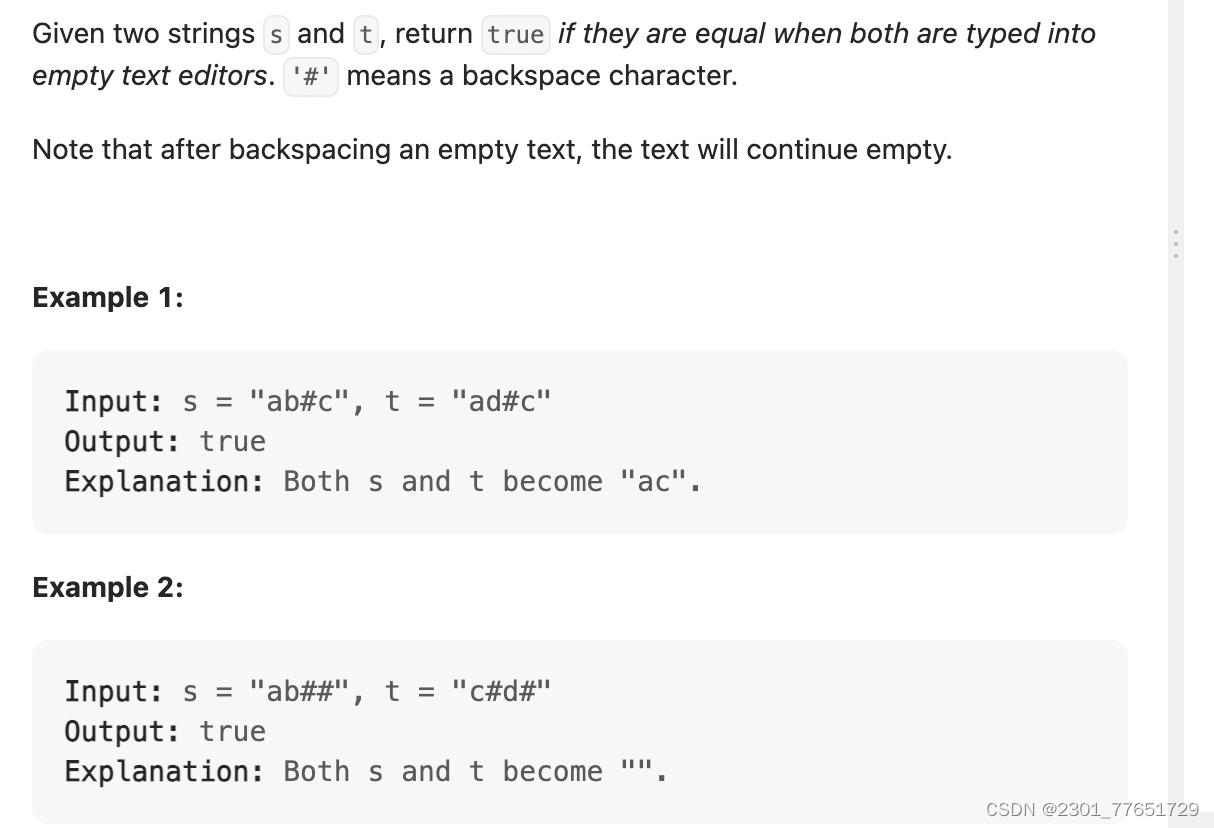
class Solution {
public boolean backspaceCompare(String s, String t) {
Stack<Character> stacks = new Stack();
Stack<Character> stackt = new Stack();
for(int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if(!stacks.isEmpty() && c == '#') {
stacks.pop();
} else {
if(c != '#')
stacks.push(c);
}
}
for(int i = 0; i < t.length(); i++) {
char c = t.charAt(i);
if(!stackt.isEmpty() && c == '#') {
stackt.pop();
} else {
if(c != '#')
stackt.push(c);
}
}
return stacks.equals(stackt);
}
}