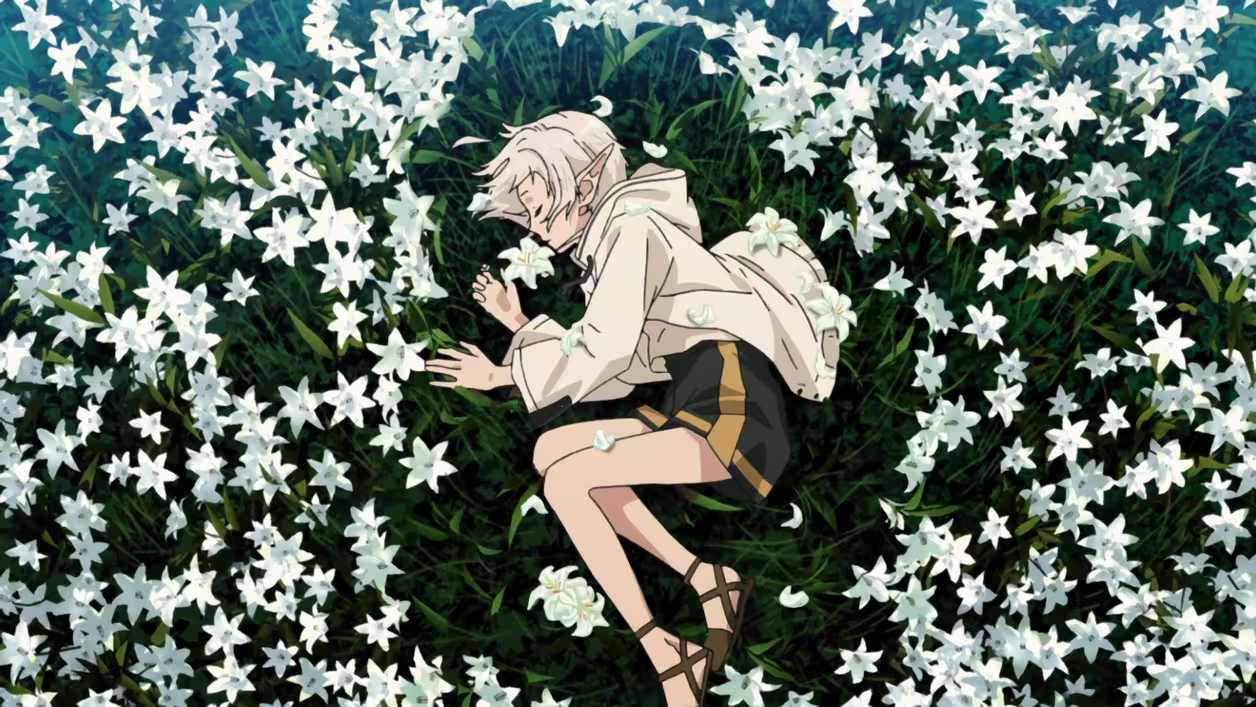
什么是红黑树?
红黑树
红黑树(Red-Black Tree)是一种自平衡的二叉搜索树,用于保持树的平衡,以确保在最坏情况下基本操作(如插入、删除和查找)的时间复杂度仍为 O(log n)。红黑树的每个节点都包含一个额外的颜色位,即红色或黑色。红黑树通过严格的平衡条件来维持树的平衡。
红黑树的性质
- 节点是红色或黑色的:每个节点都有颜色属性,红色或黑色。
- 根是黑色的:红黑树的根节点必须是黑色的。
- 叶节点(NIL 节点)是黑色的:红黑树中的所有叶节点(这里的叶节点是指为空的 NIL 节点,而不是实际的元素节点)都是黑色的。
- 红色节点的子节点必须是黑色的(即红色节点不能有红色子节点,也叫“红黑相间”):红色节点不能连续连接在一起。
- 从任何节点到其每个叶子的所有简单路径都必须包含相同数量的黑色节点:这确保了树的平衡性。
定义红黑树
enum Color
{
RED,
BLACK,
};
template<class K, class V>
struct RBTreeNode
{
pair<K, V> _kv;
RBTreeNode<K, V>* _left;
RBTreeNode<K, V>* _right;
RBTreeNode<K, V>* _parent;
Color _col;
RBTreeNode(const pair<K, V>& kv)
:_kv(kv)
,_left(nullptr)
,_right(nullptr)
,_parent(nullptr)
{}
};
template<class K,class V>
class RBTree
{
public:
typedef RBTreeNode<K, V> Node;
private:
Node* _root = nullptr;
};
红黑树的操作
insert
红黑树的逻辑是在普通的二叉搜索树上进行实现的,在普通的二叉搜索树中,我们不需要调节平衡,但是在红黑树当中我们需要根据红黑树的性质来调整数的颜色和高度,首先我们来看看第一种情况:
cur是插入节点,由于红色节点不能连续,所以这里已经破坏了平衡,所以我们应该进行调整颜色,我们不可能插入cur是黑色节点,因为如果插入黑色节点的话代价很大,每条路上都会多出来一个黑色节点,这样很不好维护,所以插入红色节点是代价最小的,插入红色节点之后,破坏了规则,所以p应该变成黑色,由于p这条路多了一个黑色节点,所以u应该也变成黑色节点,由于这棵树有可能是某个子树,这两条路多出来一个黑色节点为了保证黑色节点不多,所以g节点应该也变成红色,这样做完之后继续往上调整,将cur赋值到g节点,继续往上调整颜色。
这样就算调整完毕了。
第二种情况:当u节点不存在时
对于这种情况我们先准备调色,首先我们先将p改为黑色节点,然后将g改为红色节点之后,我们会发现,左边这条路多出来一个黑色节点,这样是不行的。
上面这个是不行的,左边已经多出来了一个黑色节点了,但是右边没有黑色节点,所以这种情况我们应该进行旋转。
对g节点进行右旋:
进行右旋之后将p改为黑色节点,将g改为红色节点,这样两边都保持平衡了。
还有一种情况:u存在但是为黑色
最下面的那个红色节点是插入节点:
这种情况我们先向上调整:
调整成这样之后,我们就发现,u是黑色节点,不管我们怎么调整,左边都会多出一个黑色节点,所以这里我们应该旋转:
绕着g节点进行旋转之后我们可以发现调整颜色就会平衡:
还有双旋的情况,双旋的情况,我们只说一种:
左旋将p和cur的位置交换,然后再对g进行右旋,就可以达到平衡的效果。
左旋接口:
void RotateL(Node* parent)
{
Node* subR = parent->_right;
Node* subRL = subR->_left;
parent->_right = subRL;
if (subRL)subRL->_parent = parent;
Node* parentParent = parent->_parent;
subR->_left = parent;
parent->_parent = subR;
if (parentParent == nullptr)
{
_root = subR;
subR->_parent = nullptr;
}
else
{
if (parent == parentParent->_left)parentParent->_left = subR;
else parentParent->_right = subR;
subR->_parent = parentParent;
}
}
右旋接口:
void RotateR(Node* parent)
{
Node* subL = parent->_left;
Node* subLR = subL->_right;
parent->_left = subLR;
if (subLR)subLR->_parent = parent;
Node* parentParent = parent->_parent;
subL->_right = parent;
parent->_parent = subL;
if (parentParent == nullptr)
{
_root = subL;
subL->_parent = nullptr;
}
else
{
if (parentParent->_left == parent)parentParent->_left = subL;
else parentParent->_right = subL;
subL->_parent = parentParent;
}
}
insert的代码:
bool Insert(const pair<K, V>& kv)
{
if (_root == nullptr)
{
_root = new Node(kv);
_root->_col = BLACK;
return true;
}
Node* cur = _root;
Node* parent = nullptr;
while (cur)
{
if (cur->_kv.first < kv.first)
{
parent = cur;
cur = cur->_right;
}
else if (cur->_kv.first > kv.first)
{
parent = cur;
cur = cur->_left;
}
else return false;
}
cur = new Node(kv);
cur->_col = RED;
//插入到右边
if (parent->_kv.first < kv.first)parent->_right = cur;
else parent->_left = cur;
cur->_parent = parent;
//调整颜色或旋转
//parent不为空并且parent指向的颜色是红色才进行调整
while (parent && parent->_col == RED)
{
Node* grandparent = parent->_parent;
if (grandparent->_left == parent)
{
Node* uncle = grandparent->_right;
//uncle存在且是红色
if (uncle && uncle->_col == RED)
{
parent->_col = uncle->_col = BLACK;
grandparent->_col = RED;
cur = grandparent;
parent = cur->_parent;
}
//uncle不存在或者存在为黑色
else
{
//判断cur是parent的左子树还是右子树:
//右单旋
if (cur == parent->_left)
{
RotateR(grandparent);
grandparent->_col = RED;
parent->_col = BLACK;
}
else
{
RotateL(parent);
RotateR(grandparent);
cur->_col = BLACK;
grandparent->_col = RED;
}
break;
}
}
else
{
Node* uncle = grandparent->_left;
//uncle存在且是红色
if (uncle && uncle->_col == RED)
{
parent->_col = uncle->_col = BLACK;
grandparent->_col = RED;
cur = grandparent;
parent = cur->_parent;
}
//uncle不存在或者存在为黑色
else
{
//判断cur是parent的左子树还是右子树:
//右单旋
if (cur == parent->_right)
{
RotateL(grandparent);
grandparent->_col = RED;
parent->_col = BLACK;
}
else
{
RotateR(parent);
RotateL(grandparent);
cur->_col = BLACK;
grandparent->_col = RED;
}
break;
}
}
}
_root->_col = BLACK;
return true;
}
inorder
void InOrder()
{
_InOrder(_root);
}
void _InOrder(Node* root)
{
if (root == nullptr)return;
_InOrder(root->_left);
cout << root->_kv.first << ':' << root->_kv.second << endl;
_InOrder(root->_right);
}
find
bool Find(const K& k)
{
Node* cur = _root;
while (cur)
{
if (cur->_kv.first < k) cur = cur->_right;
else if (cur->_kv.first > k) cur = cur->_left;
else return true;
}
return false;
}
height
int height()
{
return _height(_root);
}
int _height(Node* root)
{
if (root == nullptr)return 0;
int leftheight = _height(root->_left);
int rightheight = _height(root->_right);
return max(leftheight, rightheight) + 1;
}
size
int size()
{
return _size(_root);
}
int _size(Node* root)
{
if (root == nullptr)return 0;
int left = _size(root->_left);
int right = _size(root->_right);
return left + right + 1;
}
构造函数
//默认构造
RBTree() = default;
//拷贝构造
RBTree(const RBTree<K, V>& t)
{
_root = Copy(t._root);
}
Node* Copy(Node* root)
{
if (root == nullptr)return nullptr;
Node* newnode = new Node(root->_kv);
newnode->_left = Copy(root->_left);
newnode->_right = Copy(root->_right);
return newnode;
}
析构函数
~RBTree()
{
Destroy(_root);
_root = nullptr;
}
void Destroy(Node* root)
{
if (root == nullptr)return;
Destroy(root->_left);
Destroy(root->_right);
delete root;
}
赋值拷贝
RBTree<K, V>& operator=(RBTree<K, V> t)
{
swap(_root, t._root);
return *this;
}
判断红黑树
bool IsBalance()
{
//空树也是搜索树
if (_root == nullptr) return true;
//根节点不能为红色
if (_root->_col == RED) return false;
//参考值
int refNum = 0;
Node* cur = _root;
while (cur)
{
//遇到黑色++
if (cur->_col == BLACK) refNum++;
cur = cur->_left;
}
return Check(_root, 0, refNum);
}
bool Check(Node* root, int blackNum, const int refNum)
{
//走到空之后就算出一条路径的数量了
if (root == nullptr)
{
if (blackNum != refNum)
{
cout << "存在黑色节点不相等的路径" << endl;
return false;
}
return true;
}
//存在连续的红色节点
if (root->_col == RED && root->_parent->_col == RED)return false;
if (root->_col == BLACK)blackNum++;
return Check(root->_left, blackNum, refNum) && Check(root->_right, blackNum, refNum);
}
全部代码
#pragma once
#include<iostream>
#include<assert.h>
using namespace std;
enum Color
{
RED,
BLACK,
};
template<class K, class V>
struct RBTreeNode
{
pair<K, V> _kv;
RBTreeNode<K, V>* _left;
RBTreeNode<K, V>* _right;
RBTreeNode<K, V>* _parent;
Color _col;
RBTreeNode(const pair<K, V>& kv)
:_kv(kv)
,_left(nullptr)
,_right(nullptr)
,_parent(nullptr)
{}
};
template<class K,class V>
class RBTree
{
public:
typedef RBTreeNode<K, V> Node;
//默认构造
RBTree() = default;
//拷贝构造
RBTree(const RBTree<K, V>& t)
{
_root = Copy(t._root);
}
//赋值拷贝
RBTree<K, V>& operator=(RBTree<K, V> t)
{
swap(_root, t._root);
return *this;
}
//析构函数
~RBTree()
{
Destroy(_root);
_root = nullptr;
}
//查找
bool Find(const K& k)
{
Node* cur = _root;
while (cur)
{
if (cur->_kv.first < k) cur = cur->_right;
else if (cur->_kv.first > k) cur = cur->_left;
else return true;
}
return false;
}
//中序遍历
void InOrder()
{
_InOrder(_root);
}
//插入
bool Insert(const pair<K, V>& kv)
{
if (_root == nullptr)
{
_root = new Node(kv);
_root->_col = BLACK;
return true;
}
Node* cur = _root;
Node* parent = nullptr;
while (cur)
{
if (cur->_kv.first < kv.first)
{
parent = cur;
cur = cur->_right;
}
else if (cur->_kv.first > kv.first)
{
parent = cur;
cur = cur->_left;
}
else return false;
}
cur = new Node(kv);
cur->_col = RED;
//插入到右边
if (parent->_kv.first < kv.first)parent->_right = cur;
else parent->_left = cur;
cur->_parent = parent;
//调整颜色或旋转
//parent不为空并且parent指向的颜色是红色才进行调整
while (parent && parent->_col == RED)
{
Node* grandparent = parent->_parent;
if (grandparent->_left == parent)
{
Node* uncle = grandparent->_right;
//uncle存在且是红色
if (uncle && uncle->_col == RED)
{
parent->_col = uncle->_col = BLACK;
grandparent->_col = RED;
cur = grandparent;
parent = cur->_parent;
}
//uncle不存在或者存在为黑色
else
{
//判断cur是parent的左子树还是右子树:
//右单旋
if (cur == parent->_left)
{
RotateR(grandparent);
grandparent->_col = RED;
parent->_col = BLACK;
}
else
{
RotateL(parent);
RotateR(grandparent);
cur->_col = BLACK;
grandparent->_col = RED;
}
break;
}
}
else
{
Node* uncle = grandparent->_left;
//uncle存在且是红色
if (uncle && uncle->_col == RED)
{
parent->_col = uncle->_col = BLACK;
grandparent->_col = RED;
cur = grandparent;
parent = cur->_parent;
}
//uncle不存在或者存在为黑色
else
{
//判断cur是parent的左子树还是右子树:
//右单旋
if (cur == parent->_right)
{
RotateL(grandparent);
grandparent->_col = RED;
parent->_col = BLACK;
}
else
{
RotateR(parent);
RotateL(grandparent);
cur->_col = BLACK;
grandparent->_col = RED;
}
break;
}
}
}
_root->_col = BLACK;
return true;
}
//大小
int size()
{
return _size(_root);
}
//树的高度
int height()
{
return _height(_root);
}
private:
int _height(Node* root)
{
if (root == nullptr)return 0;
int leftheight = _height(root->_left);
int rightheight = _height(root->_right);
return max(leftheight, rightheight) + 1;
}
int _size(Node* root)
{
if (root == nullptr)return 0;
int left = _size(root->_left);
int right = _size(root->_right);
return left + right + 1;
}
void RotateL(Node* parent)
{
Node* subR = parent->_right;
Node* subRL = subR->_left;
parent->_right = subRL;
if (subRL)subRL->_parent = parent;
Node* parentParent = parent->_parent;
subR->_left = parent;
parent->_parent = subR;
if (parentParent == nullptr)
{
_root = subR;
subR->_parent = nullptr;
}
else
{
if (parent == parentParent->_left)parentParent->_left = subR;
else parentParent->_right = subR;
subR->_parent = parentParent;
}
}
void RotateR(Node* parent)
{
Node* subL = parent->_left;
Node* subLR = subL->_right;
parent->_left = subLR;
if (subLR)subLR->_parent = parent;
Node* parentParent = parent->_parent;
subL->_right = parent;
parent->_parent = subL;
if (parentParent == nullptr)
{
_root = subL;
subL->_parent = nullptr;
}
else
{
if (parentParent->_left == parent)parentParent->_left = subL;
else parentParent->_right = subL;
subL->_parent = parentParent;
}
}
void _InOrder(Node* root)
{
if (root == nullptr)return;
_InOrder(root->_left);
cout << root->_kv.first << ':' << root->_kv.second << endl;
_InOrder(root->_right);
}
void Destroy(Node* root)
{
if (root == nullptr)return;
Destroy(root->_left);
Destroy(root->_right);
delete root;
}
Node* Copy(Node* root)
{
if (root == nullptr)return nullptr;
Node* newnode = new Node(root->_kv);
newnode->_left = Copy(root->_left);
newnode->_right = Copy(root->_right);
return newnode;
}
Node* _root = nullptr;
};
总结
红黑树作为一种自平衡二叉搜索树,通过严格的颜色和旋转规则,保证了在最坏情况下仍能提供高效的查找、插入和删除操作。其特有的平衡机制使得红黑树在实际应用中被广泛采用,尤其在需要频繁增删节点的数据结构中表现出色。掌握红黑树的原理和操作,不仅有助于理解复杂数据结构的实现,也为开发高效算法奠定了坚实基础。希望通过本篇博客,大家能够对红黑树有更加深入的认识,并在实际编程中灵活应用。